Node Express js Query inside a loop
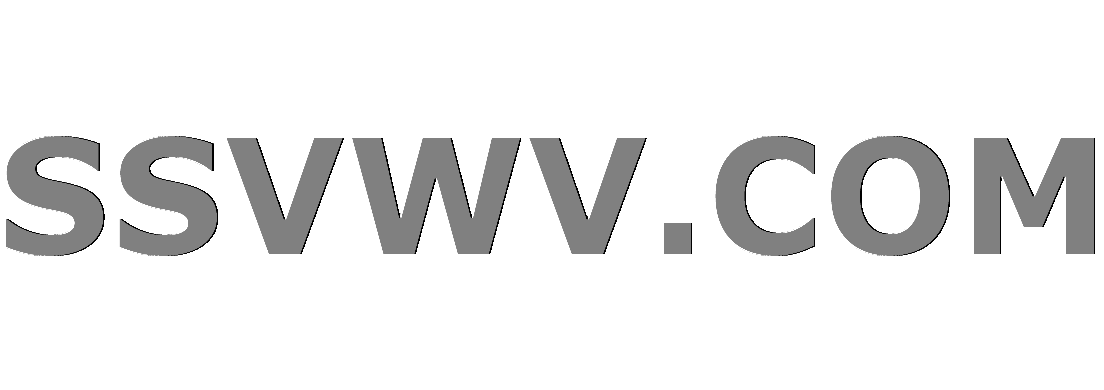
Multi tool use
I am new in node js / express I am trying to query, foreach id in an array, some data from the sqlite3 database. My for loop looks like as follows
response.patients = results;
for (var i = 0; i < results.length; i++) {
response.patients[i].check = "false";
var patient = response.patients[i];
db.each("SELECT visit_id FROM patient_visits where patient_id='"+patient.id+"' AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", function(err, row) {
if (row) {
response.patients[i].check = "true";
}
});
}
res.send(response);
the problem is that the for loop continues before the query is finished. Is there a way to check if the query has finished?
I only need to set a flag true / false.
node.js express
add a comment |
I am new in node js / express I am trying to query, foreach id in an array, some data from the sqlite3 database. My for loop looks like as follows
response.patients = results;
for (var i = 0; i < results.length; i++) {
response.patients[i].check = "false";
var patient = response.patients[i];
db.each("SELECT visit_id FROM patient_visits where patient_id='"+patient.id+"' AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", function(err, row) {
if (row) {
response.patients[i].check = "true";
}
});
}
res.send(response);
the problem is that the for loop continues before the query is finished. Is there a way to check if the query has finished?
I only need to set a flag true / false.
node.js express
I don’t know Node, so someone else will have to help you with the syntax, but if your goal is to just find if patients exist, I would consolidate this into a single query ofselect patient_id from patient_visits where patient_id in ( PATIENT ID LIST FROM RESPONSE GOES HERE ) and visitdate >= DATE GOES HERE
. The idea is perform your query using a list of ids, and basically ask for a list of valid ids back from the database. Once you have your array of integers, you can loop throughresponse.patients
and setcheck
based on whether the id is in your list that you just got from the query.
– Nate
Dec 29 '18 at 6:29
The more you can consolidate queries when it makes sense, the more performant your system will be.
– Nate
Dec 29 '18 at 6:30
add a comment |
I am new in node js / express I am trying to query, foreach id in an array, some data from the sqlite3 database. My for loop looks like as follows
response.patients = results;
for (var i = 0; i < results.length; i++) {
response.patients[i].check = "false";
var patient = response.patients[i];
db.each("SELECT visit_id FROM patient_visits where patient_id='"+patient.id+"' AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", function(err, row) {
if (row) {
response.patients[i].check = "true";
}
});
}
res.send(response);
the problem is that the for loop continues before the query is finished. Is there a way to check if the query has finished?
I only need to set a flag true / false.
node.js express
I am new in node js / express I am trying to query, foreach id in an array, some data from the sqlite3 database. My for loop looks like as follows
response.patients = results;
for (var i = 0; i < results.length; i++) {
response.patients[i].check = "false";
var patient = response.patients[i];
db.each("SELECT visit_id FROM patient_visits where patient_id='"+patient.id+"' AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", function(err, row) {
if (row) {
response.patients[i].check = "true";
}
});
}
res.send(response);
the problem is that the for loop continues before the query is finished. Is there a way to check if the query has finished?
I only need to set a flag true / false.
node.js express
node.js express
edited Dec 29 '18 at 6:53
Prateek Vora
asked Dec 29 '18 at 6:06
Prateek VoraPrateek Vora
11
11
I don’t know Node, so someone else will have to help you with the syntax, but if your goal is to just find if patients exist, I would consolidate this into a single query ofselect patient_id from patient_visits where patient_id in ( PATIENT ID LIST FROM RESPONSE GOES HERE ) and visitdate >= DATE GOES HERE
. The idea is perform your query using a list of ids, and basically ask for a list of valid ids back from the database. Once you have your array of integers, you can loop throughresponse.patients
and setcheck
based on whether the id is in your list that you just got from the query.
– Nate
Dec 29 '18 at 6:29
The more you can consolidate queries when it makes sense, the more performant your system will be.
– Nate
Dec 29 '18 at 6:30
add a comment |
I don’t know Node, so someone else will have to help you with the syntax, but if your goal is to just find if patients exist, I would consolidate this into a single query ofselect patient_id from patient_visits where patient_id in ( PATIENT ID LIST FROM RESPONSE GOES HERE ) and visitdate >= DATE GOES HERE
. The idea is perform your query using a list of ids, and basically ask for a list of valid ids back from the database. Once you have your array of integers, you can loop throughresponse.patients
and setcheck
based on whether the id is in your list that you just got from the query.
– Nate
Dec 29 '18 at 6:29
The more you can consolidate queries when it makes sense, the more performant your system will be.
– Nate
Dec 29 '18 at 6:30
I don’t know Node, so someone else will have to help you with the syntax, but if your goal is to just find if patients exist, I would consolidate this into a single query of
select patient_id from patient_visits where patient_id in ( PATIENT ID LIST FROM RESPONSE GOES HERE ) and visitdate >= DATE GOES HERE
. The idea is perform your query using a list of ids, and basically ask for a list of valid ids back from the database. Once you have your array of integers, you can loop through response.patients
and set check
based on whether the id is in your list that you just got from the query.– Nate
Dec 29 '18 at 6:29
I don’t know Node, so someone else will have to help you with the syntax, but if your goal is to just find if patients exist, I would consolidate this into a single query of
select patient_id from patient_visits where patient_id in ( PATIENT ID LIST FROM RESPONSE GOES HERE ) and visitdate >= DATE GOES HERE
. The idea is perform your query using a list of ids, and basically ask for a list of valid ids back from the database. Once you have your array of integers, you can loop through response.patients
and set check
based on whether the id is in your list that you just got from the query.– Nate
Dec 29 '18 at 6:29
The more you can consolidate queries when it makes sense, the more performant your system will be.
– Nate
Dec 29 '18 at 6:30
The more you can consolidate queries when it makes sense, the more performant your system will be.
– Nate
Dec 29 '18 at 6:30
add a comment |
1 Answer
1
active
oldest
votes
The problem is that db.each is a callback function. This function executes and the response takes some time to be retrieved, however the system doesn't stop and the for loop continues. When it ends, res.send is called while the responses from the db.each are still being processed.
You can try
db.all(
SELECT visit_id FROM patient_visits where patient_id IN ?
AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", response.patients,
function(err, rows) {
if (err) {
throw err;
}
rows.forEach((row) => {
response.patients[row.visit_id].check = "true";
});
res.send(response);
});
I'm not sure about the content of row variable to get the visit_id but before print the variable and check if it works.
If you have any doubt let me know. And I strongly advise you to read some content about callbacks and how they work and then promises and async/await because callbacks aren't used now, with async await, your problem would be solved easily.
I have solved my problem using sql query LEFT JOIN. but thank you so much for your help.
– Prateek Vora
Dec 31 '18 at 7:45
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53967146%2fnode-express-js-query-inside-a-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The problem is that db.each is a callback function. This function executes and the response takes some time to be retrieved, however the system doesn't stop and the for loop continues. When it ends, res.send is called while the responses from the db.each are still being processed.
You can try
db.all(
SELECT visit_id FROM patient_visits where patient_id IN ?
AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", response.patients,
function(err, rows) {
if (err) {
throw err;
}
rows.forEach((row) => {
response.patients[row.visit_id].check = "true";
});
res.send(response);
});
I'm not sure about the content of row variable to get the visit_id but before print the variable and check if it works.
If you have any doubt let me know. And I strongly advise you to read some content about callbacks and how they work and then promises and async/await because callbacks aren't used now, with async await, your problem would be solved easily.
I have solved my problem using sql query LEFT JOIN. but thank you so much for your help.
– Prateek Vora
Dec 31 '18 at 7:45
add a comment |
The problem is that db.each is a callback function. This function executes and the response takes some time to be retrieved, however the system doesn't stop and the for loop continues. When it ends, res.send is called while the responses from the db.each are still being processed.
You can try
db.all(
SELECT visit_id FROM patient_visits where patient_id IN ?
AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", response.patients,
function(err, rows) {
if (err) {
throw err;
}
rows.forEach((row) => {
response.patients[row.visit_id].check = "true";
});
res.send(response);
});
I'm not sure about the content of row variable to get the visit_id but before print the variable and check if it works.
If you have any doubt let me know. And I strongly advise you to read some content about callbacks and how they work and then promises and async/await because callbacks aren't used now, with async await, your problem would be solved easily.
I have solved my problem using sql query LEFT JOIN. but thank you so much for your help.
– Prateek Vora
Dec 31 '18 at 7:45
add a comment |
The problem is that db.each is a callback function. This function executes and the response takes some time to be retrieved, however the system doesn't stop and the for loop continues. When it ends, res.send is called while the responses from the db.each are still being processed.
You can try
db.all(
SELECT visit_id FROM patient_visits where patient_id IN ?
AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", response.patients,
function(err, rows) {
if (err) {
throw err;
}
rows.forEach((row) => {
response.patients[row.visit_id].check = "true";
});
res.send(response);
});
I'm not sure about the content of row variable to get the visit_id but before print the variable and check if it works.
If you have any doubt let me know. And I strongly advise you to read some content about callbacks and how they work and then promises and async/await because callbacks aren't used now, with async await, your problem would be solved easily.
The problem is that db.each is a callback function. This function executes and the response takes some time to be retrieved, however the system doesn't stop and the for loop continues. When it ends, res.send is called while the responses from the db.each are still being processed.
You can try
db.all(
SELECT visit_id FROM patient_visits where patient_id IN ?
AND visitdate >='"+moment().format('YYYY-MM-DD')+"'", response.patients,
function(err, rows) {
if (err) {
throw err;
}
rows.forEach((row) => {
response.patients[row.visit_id].check = "true";
});
res.send(response);
});
I'm not sure about the content of row variable to get the visit_id but before print the variable and check if it works.
If you have any doubt let me know. And I strongly advise you to read some content about callbacks and how they work and then promises and async/await because callbacks aren't used now, with async await, your problem would be solved easily.
answered Dec 29 '18 at 13:05


Pedro SilvaPedro Silva
651215
651215
I have solved my problem using sql query LEFT JOIN. but thank you so much for your help.
– Prateek Vora
Dec 31 '18 at 7:45
add a comment |
I have solved my problem using sql query LEFT JOIN. but thank you so much for your help.
– Prateek Vora
Dec 31 '18 at 7:45
I have solved my problem using sql query LEFT JOIN. but thank you so much for your help.
– Prateek Vora
Dec 31 '18 at 7:45
I have solved my problem using sql query LEFT JOIN. but thank you so much for your help.
– Prateek Vora
Dec 31 '18 at 7:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53967146%2fnode-express-js-query-inside-a-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
H1oM,eFUwL1 n76GEmxHJI 1wYeF,ruy
I don’t know Node, so someone else will have to help you with the syntax, but if your goal is to just find if patients exist, I would consolidate this into a single query of
select patient_id from patient_visits where patient_id in ( PATIENT ID LIST FROM RESPONSE GOES HERE ) and visitdate >= DATE GOES HERE
. The idea is perform your query using a list of ids, and basically ask for a list of valid ids back from the database. Once you have your array of integers, you can loop throughresponse.patients
and setcheck
based on whether the id is in your list that you just got from the query.– Nate
Dec 29 '18 at 6:29
The more you can consolidate queries when it makes sense, the more performant your system will be.
– Nate
Dec 29 '18 at 6:30