How to avoid creating a class attribute by accident
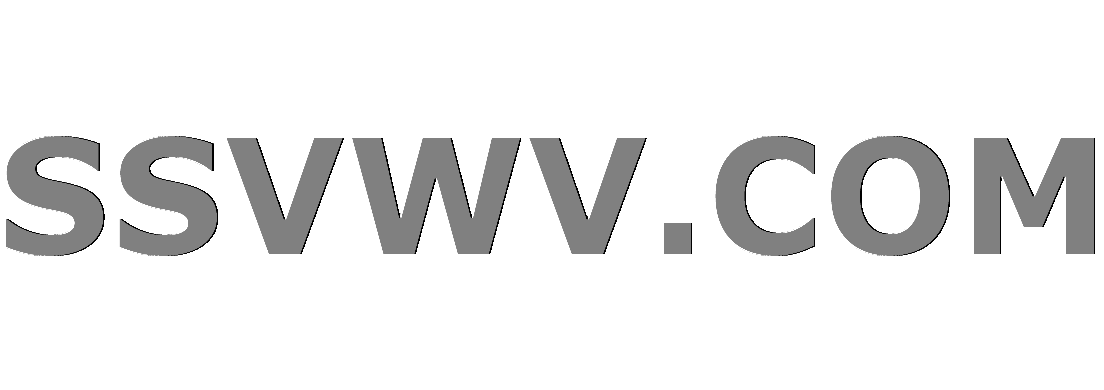
Multi tool use
I know the motto is "we're all consenting adults around here."
but here is a problem I spent a day on. I got passed a class with over 100 attributes. I had specified one of them was to be called "run_count". The front-end had a place to enter run_count.
Somehow, the front-end/back-end package people decided to call it "run_iterations" instead.
So, my problem is I am writing unit test software, and I did this:
passed_parameters.run_count = 100
result = do_the_thing(passed_parameters)
assert result == 99.75
Now, the problem, of course, is that Python willingly let me set this "new" attribute called "run_count". But, after delving 10 levels down into the code, I discover that the function "do_the_thing" (obviously) never looks at "run_count", but uses "passed_paramaters.run_iterations" instead.
Is there some simple way to avoid allowing yourself to create a new attribute in a class, or a new entry in a dictionary, when you naievely assume you know the attribute name (or the dict key), and accidentally create a new entry that never gets looked at?
In an ideal world, no matter how dynamic, Python would allow you to "lock" and object or instance of one. Then, trying to set a new value for an attribute that doesn't exist would raise an attribute error, letting you know you are trying to change something that doesn't exist, rather than letting you create a new attribute that never gets used.
python-3.x
add a comment |
I know the motto is "we're all consenting adults around here."
but here is a problem I spent a day on. I got passed a class with over 100 attributes. I had specified one of them was to be called "run_count". The front-end had a place to enter run_count.
Somehow, the front-end/back-end package people decided to call it "run_iterations" instead.
So, my problem is I am writing unit test software, and I did this:
passed_parameters.run_count = 100
result = do_the_thing(passed_parameters)
assert result == 99.75
Now, the problem, of course, is that Python willingly let me set this "new" attribute called "run_count". But, after delving 10 levels down into the code, I discover that the function "do_the_thing" (obviously) never looks at "run_count", but uses "passed_paramaters.run_iterations" instead.
Is there some simple way to avoid allowing yourself to create a new attribute in a class, or a new entry in a dictionary, when you naievely assume you know the attribute name (or the dict key), and accidentally create a new entry that never gets looked at?
In an ideal world, no matter how dynamic, Python would allow you to "lock" and object or instance of one. Then, trying to set a new value for an attribute that doesn't exist would raise an attribute error, letting you know you are trying to change something that doesn't exist, rather than letting you create a new attribute that never gets used.
python-3.x
1
It seems you are looking for an statically typed language
– Josué Cortina
Dec 29 '18 at 6:28
No, just looking for a way to avoid wasted time on complex projects. As I noted, most of the dat went into tracking down what attribute - other than the one I had asked for - got specified on the front-end and used deep in the back-end, where it was not what I expected. This is a nightmare for large projects: suppose you develop a class, someone redefines an attribute or method for it that changes it but their code relies on it, but that def changes later. It would be a nightmare to track down that what got lost was someone's redefinition you never knew of.
– eSurfsnake
Dec 29 '18 at 6:31
add a comment |
I know the motto is "we're all consenting adults around here."
but here is a problem I spent a day on. I got passed a class with over 100 attributes. I had specified one of them was to be called "run_count". The front-end had a place to enter run_count.
Somehow, the front-end/back-end package people decided to call it "run_iterations" instead.
So, my problem is I am writing unit test software, and I did this:
passed_parameters.run_count = 100
result = do_the_thing(passed_parameters)
assert result == 99.75
Now, the problem, of course, is that Python willingly let me set this "new" attribute called "run_count". But, after delving 10 levels down into the code, I discover that the function "do_the_thing" (obviously) never looks at "run_count", but uses "passed_paramaters.run_iterations" instead.
Is there some simple way to avoid allowing yourself to create a new attribute in a class, or a new entry in a dictionary, when you naievely assume you know the attribute name (or the dict key), and accidentally create a new entry that never gets looked at?
In an ideal world, no matter how dynamic, Python would allow you to "lock" and object or instance of one. Then, trying to set a new value for an attribute that doesn't exist would raise an attribute error, letting you know you are trying to change something that doesn't exist, rather than letting you create a new attribute that never gets used.
python-3.x
I know the motto is "we're all consenting adults around here."
but here is a problem I spent a day on. I got passed a class with over 100 attributes. I had specified one of them was to be called "run_count". The front-end had a place to enter run_count.
Somehow, the front-end/back-end package people decided to call it "run_iterations" instead.
So, my problem is I am writing unit test software, and I did this:
passed_parameters.run_count = 100
result = do_the_thing(passed_parameters)
assert result == 99.75
Now, the problem, of course, is that Python willingly let me set this "new" attribute called "run_count". But, after delving 10 levels down into the code, I discover that the function "do_the_thing" (obviously) never looks at "run_count", but uses "passed_paramaters.run_iterations" instead.
Is there some simple way to avoid allowing yourself to create a new attribute in a class, or a new entry in a dictionary, when you naievely assume you know the attribute name (or the dict key), and accidentally create a new entry that never gets looked at?
In an ideal world, no matter how dynamic, Python would allow you to "lock" and object or instance of one. Then, trying to set a new value for an attribute that doesn't exist would raise an attribute error, letting you know you are trying to change something that doesn't exist, rather than letting you create a new attribute that never gets used.
python-3.x
python-3.x
asked Dec 29 '18 at 6:21
eSurfsnakeeSurfsnake
279110
279110
1
It seems you are looking for an statically typed language
– Josué Cortina
Dec 29 '18 at 6:28
No, just looking for a way to avoid wasted time on complex projects. As I noted, most of the dat went into tracking down what attribute - other than the one I had asked for - got specified on the front-end and used deep in the back-end, where it was not what I expected. This is a nightmare for large projects: suppose you develop a class, someone redefines an attribute or method for it that changes it but their code relies on it, but that def changes later. It would be a nightmare to track down that what got lost was someone's redefinition you never knew of.
– eSurfsnake
Dec 29 '18 at 6:31
add a comment |
1
It seems you are looking for an statically typed language
– Josué Cortina
Dec 29 '18 at 6:28
No, just looking for a way to avoid wasted time on complex projects. As I noted, most of the dat went into tracking down what attribute - other than the one I had asked for - got specified on the front-end and used deep in the back-end, where it was not what I expected. This is a nightmare for large projects: suppose you develop a class, someone redefines an attribute or method for it that changes it but their code relies on it, but that def changes later. It would be a nightmare to track down that what got lost was someone's redefinition you never knew of.
– eSurfsnake
Dec 29 '18 at 6:31
1
1
It seems you are looking for an statically typed language
– Josué Cortina
Dec 29 '18 at 6:28
It seems you are looking for an statically typed language
– Josué Cortina
Dec 29 '18 at 6:28
No, just looking for a way to avoid wasted time on complex projects. As I noted, most of the dat went into tracking down what attribute - other than the one I had asked for - got specified on the front-end and used deep in the back-end, where it was not what I expected. This is a nightmare for large projects: suppose you develop a class, someone redefines an attribute or method for it that changes it but their code relies on it, but that def changes later. It would be a nightmare to track down that what got lost was someone's redefinition you never knew of.
– eSurfsnake
Dec 29 '18 at 6:31
No, just looking for a way to avoid wasted time on complex projects. As I noted, most of the dat went into tracking down what attribute - other than the one I had asked for - got specified on the front-end and used deep in the back-end, where it was not what I expected. This is a nightmare for large projects: suppose you develop a class, someone redefines an attribute or method for it that changes it but their code relies on it, but that def changes later. It would be a nightmare to track down that what got lost was someone's redefinition you never knew of.
– eSurfsnake
Dec 29 '18 at 6:31
add a comment |
1 Answer
1
active
oldest
votes
Use __setattr__
, and check the attribute exists, otherwise, throw an error. If you do this, you will receive an error when you define those attributes inside __init__
, so you have to workaround that situation. I found 4 ways of doing that. First, define those attributes inside the class, that way, when you try to set their initial value they will already be defined. Second, call object.__setattr__
directly. Third, add a fourth boolean param to __setattr__
indicating whether to bypass checking or not. Fourth, define the previous boolean flag as class-wide, set it to True
, initialize the fields and set the flag back to False
. Here is the code:
Code
class A:
f = 90
a = None
bypass_check = False
def __init__(self, a, b, c, d1, d2, d3, d4):
# 1st workaround
self.a = a
# 2nd workaround
object.__setattr__(self, 'b', b)
# 3rd workaround
self.__setattr__('c', c, True)
# 4th workaround
self.bypass_check = True
self.d1 = d1
self.d2 = d2
self.d3 = d3
self.d4 = d4
self.bypass_check = False
def __setattr__(self, attr, value, bypass=False):
if bypass or self.bypass_check or hasattr(self, attr):
object.__setattr__(self, attr, value)
else:
# Throw some error
print('Attribute %s not found' % attr)
a = A(1, 2, 3, 4, 5, 6, 7)
a.f = 100
a.d1 = -1
a.g = 200
print(a.f, a.a, a.d1, a.d4)
Output
Attribute g not found
100 1 -1 7
In the right area, but how to avoid accidentally creating new attributes, not calling for ones that don't exiist?
– eSurfsnake
Dec 29 '18 at 6:39
It seems there ought to be a __setattr__(), and one could put a hook in there. Something like: def __set_attr__(attr, value): if not __get_attr__(attr) raise <proper error> else <go and__setattr__()>
– eSurfsnake
Dec 29 '18 at 6:40
eSurfsnake, I edited the code... that should work
– Josué Cortina
Dec 29 '18 at 6:49
yes, exactly what I was looking for. Thanks!.
– eSurfsnake
Dec 29 '18 at 6:50
@eSurfsnake please, remember to mark my answer as accepted (green checkmark) and up vote it if this worked for you. Thanks in advance!
– Josué Cortina
Dec 29 '18 at 17:02
|
show 3 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53967220%2fhow-to-avoid-creating-a-class-attribute-by-accident%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use __setattr__
, and check the attribute exists, otherwise, throw an error. If you do this, you will receive an error when you define those attributes inside __init__
, so you have to workaround that situation. I found 4 ways of doing that. First, define those attributes inside the class, that way, when you try to set their initial value they will already be defined. Second, call object.__setattr__
directly. Third, add a fourth boolean param to __setattr__
indicating whether to bypass checking or not. Fourth, define the previous boolean flag as class-wide, set it to True
, initialize the fields and set the flag back to False
. Here is the code:
Code
class A:
f = 90
a = None
bypass_check = False
def __init__(self, a, b, c, d1, d2, d3, d4):
# 1st workaround
self.a = a
# 2nd workaround
object.__setattr__(self, 'b', b)
# 3rd workaround
self.__setattr__('c', c, True)
# 4th workaround
self.bypass_check = True
self.d1 = d1
self.d2 = d2
self.d3 = d3
self.d4 = d4
self.bypass_check = False
def __setattr__(self, attr, value, bypass=False):
if bypass or self.bypass_check or hasattr(self, attr):
object.__setattr__(self, attr, value)
else:
# Throw some error
print('Attribute %s not found' % attr)
a = A(1, 2, 3, 4, 5, 6, 7)
a.f = 100
a.d1 = -1
a.g = 200
print(a.f, a.a, a.d1, a.d4)
Output
Attribute g not found
100 1 -1 7
In the right area, but how to avoid accidentally creating new attributes, not calling for ones that don't exiist?
– eSurfsnake
Dec 29 '18 at 6:39
It seems there ought to be a __setattr__(), and one could put a hook in there. Something like: def __set_attr__(attr, value): if not __get_attr__(attr) raise <proper error> else <go and__setattr__()>
– eSurfsnake
Dec 29 '18 at 6:40
eSurfsnake, I edited the code... that should work
– Josué Cortina
Dec 29 '18 at 6:49
yes, exactly what I was looking for. Thanks!.
– eSurfsnake
Dec 29 '18 at 6:50
@eSurfsnake please, remember to mark my answer as accepted (green checkmark) and up vote it if this worked for you. Thanks in advance!
– Josué Cortina
Dec 29 '18 at 17:02
|
show 3 more comments
Use __setattr__
, and check the attribute exists, otherwise, throw an error. If you do this, you will receive an error when you define those attributes inside __init__
, so you have to workaround that situation. I found 4 ways of doing that. First, define those attributes inside the class, that way, when you try to set their initial value they will already be defined. Second, call object.__setattr__
directly. Third, add a fourth boolean param to __setattr__
indicating whether to bypass checking or not. Fourth, define the previous boolean flag as class-wide, set it to True
, initialize the fields and set the flag back to False
. Here is the code:
Code
class A:
f = 90
a = None
bypass_check = False
def __init__(self, a, b, c, d1, d2, d3, d4):
# 1st workaround
self.a = a
# 2nd workaround
object.__setattr__(self, 'b', b)
# 3rd workaround
self.__setattr__('c', c, True)
# 4th workaround
self.bypass_check = True
self.d1 = d1
self.d2 = d2
self.d3 = d3
self.d4 = d4
self.bypass_check = False
def __setattr__(self, attr, value, bypass=False):
if bypass or self.bypass_check or hasattr(self, attr):
object.__setattr__(self, attr, value)
else:
# Throw some error
print('Attribute %s not found' % attr)
a = A(1, 2, 3, 4, 5, 6, 7)
a.f = 100
a.d1 = -1
a.g = 200
print(a.f, a.a, a.d1, a.d4)
Output
Attribute g not found
100 1 -1 7
In the right area, but how to avoid accidentally creating new attributes, not calling for ones that don't exiist?
– eSurfsnake
Dec 29 '18 at 6:39
It seems there ought to be a __setattr__(), and one could put a hook in there. Something like: def __set_attr__(attr, value): if not __get_attr__(attr) raise <proper error> else <go and__setattr__()>
– eSurfsnake
Dec 29 '18 at 6:40
eSurfsnake, I edited the code... that should work
– Josué Cortina
Dec 29 '18 at 6:49
yes, exactly what I was looking for. Thanks!.
– eSurfsnake
Dec 29 '18 at 6:50
@eSurfsnake please, remember to mark my answer as accepted (green checkmark) and up vote it if this worked for you. Thanks in advance!
– Josué Cortina
Dec 29 '18 at 17:02
|
show 3 more comments
Use __setattr__
, and check the attribute exists, otherwise, throw an error. If you do this, you will receive an error when you define those attributes inside __init__
, so you have to workaround that situation. I found 4 ways of doing that. First, define those attributes inside the class, that way, when you try to set their initial value they will already be defined. Second, call object.__setattr__
directly. Third, add a fourth boolean param to __setattr__
indicating whether to bypass checking or not. Fourth, define the previous boolean flag as class-wide, set it to True
, initialize the fields and set the flag back to False
. Here is the code:
Code
class A:
f = 90
a = None
bypass_check = False
def __init__(self, a, b, c, d1, d2, d3, d4):
# 1st workaround
self.a = a
# 2nd workaround
object.__setattr__(self, 'b', b)
# 3rd workaround
self.__setattr__('c', c, True)
# 4th workaround
self.bypass_check = True
self.d1 = d1
self.d2 = d2
self.d3 = d3
self.d4 = d4
self.bypass_check = False
def __setattr__(self, attr, value, bypass=False):
if bypass or self.bypass_check or hasattr(self, attr):
object.__setattr__(self, attr, value)
else:
# Throw some error
print('Attribute %s not found' % attr)
a = A(1, 2, 3, 4, 5, 6, 7)
a.f = 100
a.d1 = -1
a.g = 200
print(a.f, a.a, a.d1, a.d4)
Output
Attribute g not found
100 1 -1 7
Use __setattr__
, and check the attribute exists, otherwise, throw an error. If you do this, you will receive an error when you define those attributes inside __init__
, so you have to workaround that situation. I found 4 ways of doing that. First, define those attributes inside the class, that way, when you try to set their initial value they will already be defined. Second, call object.__setattr__
directly. Third, add a fourth boolean param to __setattr__
indicating whether to bypass checking or not. Fourth, define the previous boolean flag as class-wide, set it to True
, initialize the fields and set the flag back to False
. Here is the code:
Code
class A:
f = 90
a = None
bypass_check = False
def __init__(self, a, b, c, d1, d2, d3, d4):
# 1st workaround
self.a = a
# 2nd workaround
object.__setattr__(self, 'b', b)
# 3rd workaround
self.__setattr__('c', c, True)
# 4th workaround
self.bypass_check = True
self.d1 = d1
self.d2 = d2
self.d3 = d3
self.d4 = d4
self.bypass_check = False
def __setattr__(self, attr, value, bypass=False):
if bypass or self.bypass_check or hasattr(self, attr):
object.__setattr__(self, attr, value)
else:
# Throw some error
print('Attribute %s not found' % attr)
a = A(1, 2, 3, 4, 5, 6, 7)
a.f = 100
a.d1 = -1
a.g = 200
print(a.f, a.a, a.d1, a.d4)
Output
Attribute g not found
100 1 -1 7
edited Dec 30 '18 at 20:03
answered Dec 29 '18 at 6:36


Josué CortinaJosué Cortina
1,286212
1,286212
In the right area, but how to avoid accidentally creating new attributes, not calling for ones that don't exiist?
– eSurfsnake
Dec 29 '18 at 6:39
It seems there ought to be a __setattr__(), and one could put a hook in there. Something like: def __set_attr__(attr, value): if not __get_attr__(attr) raise <proper error> else <go and__setattr__()>
– eSurfsnake
Dec 29 '18 at 6:40
eSurfsnake, I edited the code... that should work
– Josué Cortina
Dec 29 '18 at 6:49
yes, exactly what I was looking for. Thanks!.
– eSurfsnake
Dec 29 '18 at 6:50
@eSurfsnake please, remember to mark my answer as accepted (green checkmark) and up vote it if this worked for you. Thanks in advance!
– Josué Cortina
Dec 29 '18 at 17:02
|
show 3 more comments
In the right area, but how to avoid accidentally creating new attributes, not calling for ones that don't exiist?
– eSurfsnake
Dec 29 '18 at 6:39
It seems there ought to be a __setattr__(), and one could put a hook in there. Something like: def __set_attr__(attr, value): if not __get_attr__(attr) raise <proper error> else <go and__setattr__()>
– eSurfsnake
Dec 29 '18 at 6:40
eSurfsnake, I edited the code... that should work
– Josué Cortina
Dec 29 '18 at 6:49
yes, exactly what I was looking for. Thanks!.
– eSurfsnake
Dec 29 '18 at 6:50
@eSurfsnake please, remember to mark my answer as accepted (green checkmark) and up vote it if this worked for you. Thanks in advance!
– Josué Cortina
Dec 29 '18 at 17:02
In the right area, but how to avoid accidentally creating new attributes, not calling for ones that don't exiist?
– eSurfsnake
Dec 29 '18 at 6:39
In the right area, but how to avoid accidentally creating new attributes, not calling for ones that don't exiist?
– eSurfsnake
Dec 29 '18 at 6:39
It seems there ought to be a __setattr__(), and one could put a hook in there. Something like: def __set_attr__(attr, value): if not __get_attr__(attr) raise <proper error> else <go and__setattr__()>
– eSurfsnake
Dec 29 '18 at 6:40
It seems there ought to be a __setattr__(), and one could put a hook in there. Something like: def __set_attr__(attr, value): if not __get_attr__(attr) raise <proper error> else <go and__setattr__()>
– eSurfsnake
Dec 29 '18 at 6:40
eSurfsnake, I edited the code... that should work
– Josué Cortina
Dec 29 '18 at 6:49
eSurfsnake, I edited the code... that should work
– Josué Cortina
Dec 29 '18 at 6:49
yes, exactly what I was looking for. Thanks!.
– eSurfsnake
Dec 29 '18 at 6:50
yes, exactly what I was looking for. Thanks!.
– eSurfsnake
Dec 29 '18 at 6:50
@eSurfsnake please, remember to mark my answer as accepted (green checkmark) and up vote it if this worked for you. Thanks in advance!
– Josué Cortina
Dec 29 '18 at 17:02
@eSurfsnake please, remember to mark my answer as accepted (green checkmark) and up vote it if this worked for you. Thanks in advance!
– Josué Cortina
Dec 29 '18 at 17:02
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53967220%2fhow-to-avoid-creating-a-class-attribute-by-accident%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fOeY4iqEob
1
It seems you are looking for an statically typed language
– Josué Cortina
Dec 29 '18 at 6:28
No, just looking for a way to avoid wasted time on complex projects. As I noted, most of the dat went into tracking down what attribute - other than the one I had asked for - got specified on the front-end and used deep in the back-end, where it was not what I expected. This is a nightmare for large projects: suppose you develop a class, someone redefines an attribute or method for it that changes it but their code relies on it, but that def changes later. It would be a nightmare to track down that what got lost was someone's redefinition you never knew of.
– eSurfsnake
Dec 29 '18 at 6:31