How to align View component to left and right equally in react-native
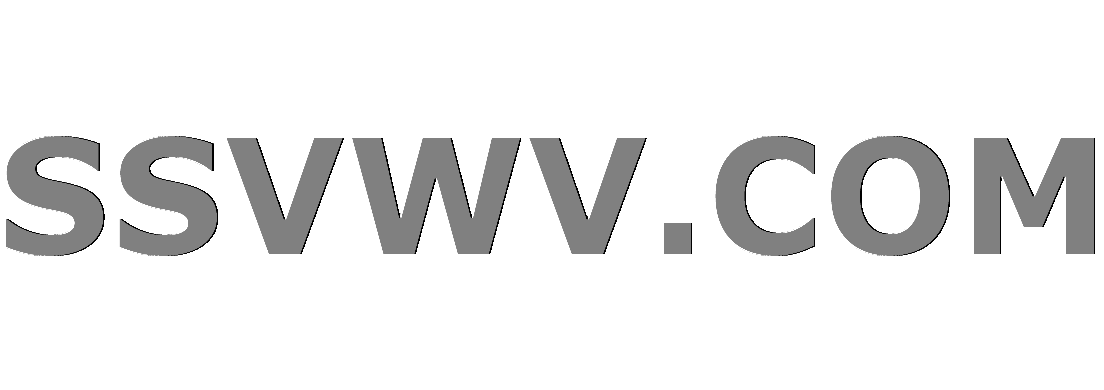
Multi tool use
I am building a cross platform mobile application using react-native. I am implementing a functionality where I am displaying the data in Accordion. I want the data to be aligned to the left and right in the Accordion Header and Accordion Body but I am not able to achieve that.
Here is my code-
import React, { Component } from 'react';
import {
Switch,
ScrollView,
StyleSheet,
Text,
Image,
View,
TouchableOpacity,
} from 'react-native';
import * as Animatable from 'react-native-animatable';
import Collapsible from 'react-native-collapsible';
import Accordion from 'react-native-collapsible/Accordion';
const axios = require('axios');
import moment from 'moment'
import Dialog, { SlideAnimation, DialogContent } from 'react-native-popup-dialog';
export default class AccordionScreen extends Component {
constructor (){
super();
this.state = {
activeSections: ,
collapsed: true,
multipleSelect: false,
newData:,
visible: false
};
}
componentDidMount(){
this.fetchData();
}
fetchData() {
axios({
method: "POST",
url: "URL",
headers: {
"Content-Type": "application/json",
"Authorization": this.props.navigation.getParam('authorizationToken', undefined)
},
data: {}
})
.then (response => {
if (response.status === 200 ){
if (response.data.newData.length > 0 ){
this.setState({
newData: response.data.newData
});
//console.log(activeSections);
}
else {
return <h1>No Data!</h1>
}
}
else{
throw "Request resulted in NOT 200";
}
})
.catch(error => {
console.log(error);
});
}
_renderSectionTitle = section => {
return (
<View style={styles.titleHeader}>
<Text>{moment(section.eventDate).format('ll')}</Text>
</View>
);
};
toggleExpanded = () => {
this.setState({ collapsed: !this.state.collapsed });
};
setSections = sections => {
this.setState({
activeSections: sections.includes(undefined) ? : sections,
});
};
_renderHeader(section, index, isActive, sections) {
return (
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</Animatable.View>
);
}
_renderContent(section, i, isActive, sections) {
return (
<Animatable.View
duration={300}
transition="backgroundColor"
style={[styles.accordionCont, isActive ? styles.active : styles.inactive]}
transition="backgroundColor"
>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Insurer Address
</Animatable.Text>
<Animatable.Text
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
{section.addressStreet}
</Animatable.Text>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Policy Number
</Animatable.Text>
<Animatable.Text
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
{section.policyNumber}
</Animatable.Text>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Additional Details
</Animatable.Text>
</Animatable.View>
);
}
_updateSections = activeSections => {
this.setState({ activeSections });
};
render() {
const { multipleSelect, activeSections, poiHistoryData } = this.state;
return (
<View style={styles.container}>
<ScrollView contentContainerStyle={{ paddingTop: 30 }}>
<TouchableOpacity onPress={this.toggleExpanded}>
<View style={styles.header}>
<Text style={styles.headerText}>View Data</Text>
</View>
</TouchableOpacity>
<Accordion
sections={newData}
activeSections={activeSections}
touchableComponent={TouchableOpacity}
expandMultiple={multipleSelect}
renderSectionTitle={this._renderSectionTitle}
renderHeader={this._renderHeader}
renderContent={this._renderContent}
duration={400}
onChange={this._updateSections}
/>
</ScrollView>
</View>
) }
}
If you see the Heading 'Name' and 'City', these are the headings and I am displaying the value just below it. But right now everything is stacked and aligned to the left. I want 'Other Insurer' and its value to be appear on the left of the screen and 'Other Insured' and its value to be aligned to the right of the screen.
CSS that I am using-
headerCont: {
fontWeight:'bold'
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'flex-end',
alignItems: 'center'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
flex: 1,
flexDirection: 'row'
}
Screenshot-
Can someone please suggest me how can I handle this gracefully. Thanks in advance.
css react-native react-native-stylesheet
add a comment |
I am building a cross platform mobile application using react-native. I am implementing a functionality where I am displaying the data in Accordion. I want the data to be aligned to the left and right in the Accordion Header and Accordion Body but I am not able to achieve that.
Here is my code-
import React, { Component } from 'react';
import {
Switch,
ScrollView,
StyleSheet,
Text,
Image,
View,
TouchableOpacity,
} from 'react-native';
import * as Animatable from 'react-native-animatable';
import Collapsible from 'react-native-collapsible';
import Accordion from 'react-native-collapsible/Accordion';
const axios = require('axios');
import moment from 'moment'
import Dialog, { SlideAnimation, DialogContent } from 'react-native-popup-dialog';
export default class AccordionScreen extends Component {
constructor (){
super();
this.state = {
activeSections: ,
collapsed: true,
multipleSelect: false,
newData:,
visible: false
};
}
componentDidMount(){
this.fetchData();
}
fetchData() {
axios({
method: "POST",
url: "URL",
headers: {
"Content-Type": "application/json",
"Authorization": this.props.navigation.getParam('authorizationToken', undefined)
},
data: {}
})
.then (response => {
if (response.status === 200 ){
if (response.data.newData.length > 0 ){
this.setState({
newData: response.data.newData
});
//console.log(activeSections);
}
else {
return <h1>No Data!</h1>
}
}
else{
throw "Request resulted in NOT 200";
}
})
.catch(error => {
console.log(error);
});
}
_renderSectionTitle = section => {
return (
<View style={styles.titleHeader}>
<Text>{moment(section.eventDate).format('ll')}</Text>
</View>
);
};
toggleExpanded = () => {
this.setState({ collapsed: !this.state.collapsed });
};
setSections = sections => {
this.setState({
activeSections: sections.includes(undefined) ? : sections,
});
};
_renderHeader(section, index, isActive, sections) {
return (
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</Animatable.View>
);
}
_renderContent(section, i, isActive, sections) {
return (
<Animatable.View
duration={300}
transition="backgroundColor"
style={[styles.accordionCont, isActive ? styles.active : styles.inactive]}
transition="backgroundColor"
>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Insurer Address
</Animatable.Text>
<Animatable.Text
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
{section.addressStreet}
</Animatable.Text>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Policy Number
</Animatable.Text>
<Animatable.Text
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
{section.policyNumber}
</Animatable.Text>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Additional Details
</Animatable.Text>
</Animatable.View>
);
}
_updateSections = activeSections => {
this.setState({ activeSections });
};
render() {
const { multipleSelect, activeSections, poiHistoryData } = this.state;
return (
<View style={styles.container}>
<ScrollView contentContainerStyle={{ paddingTop: 30 }}>
<TouchableOpacity onPress={this.toggleExpanded}>
<View style={styles.header}>
<Text style={styles.headerText}>View Data</Text>
</View>
</TouchableOpacity>
<Accordion
sections={newData}
activeSections={activeSections}
touchableComponent={TouchableOpacity}
expandMultiple={multipleSelect}
renderSectionTitle={this._renderSectionTitle}
renderHeader={this._renderHeader}
renderContent={this._renderContent}
duration={400}
onChange={this._updateSections}
/>
</ScrollView>
</View>
) }
}
If you see the Heading 'Name' and 'City', these are the headings and I am displaying the value just below it. But right now everything is stacked and aligned to the left. I want 'Other Insurer' and its value to be appear on the left of the screen and 'Other Insured' and its value to be aligned to the right of the screen.
CSS that I am using-
headerCont: {
fontWeight:'bold'
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'flex-end',
alignItems: 'center'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
flex: 1,
flexDirection: 'row'
}
Screenshot-
Can someone please suggest me how can I handle this gracefully. Thanks in advance.
css react-native react-native-stylesheet
take the screen shot of your issue and attach.
– Malik Adil
Dec 29 '18 at 6:28
add a comment |
I am building a cross platform mobile application using react-native. I am implementing a functionality where I am displaying the data in Accordion. I want the data to be aligned to the left and right in the Accordion Header and Accordion Body but I am not able to achieve that.
Here is my code-
import React, { Component } from 'react';
import {
Switch,
ScrollView,
StyleSheet,
Text,
Image,
View,
TouchableOpacity,
} from 'react-native';
import * as Animatable from 'react-native-animatable';
import Collapsible from 'react-native-collapsible';
import Accordion from 'react-native-collapsible/Accordion';
const axios = require('axios');
import moment from 'moment'
import Dialog, { SlideAnimation, DialogContent } from 'react-native-popup-dialog';
export default class AccordionScreen extends Component {
constructor (){
super();
this.state = {
activeSections: ,
collapsed: true,
multipleSelect: false,
newData:,
visible: false
};
}
componentDidMount(){
this.fetchData();
}
fetchData() {
axios({
method: "POST",
url: "URL",
headers: {
"Content-Type": "application/json",
"Authorization": this.props.navigation.getParam('authorizationToken', undefined)
},
data: {}
})
.then (response => {
if (response.status === 200 ){
if (response.data.newData.length > 0 ){
this.setState({
newData: response.data.newData
});
//console.log(activeSections);
}
else {
return <h1>No Data!</h1>
}
}
else{
throw "Request resulted in NOT 200";
}
})
.catch(error => {
console.log(error);
});
}
_renderSectionTitle = section => {
return (
<View style={styles.titleHeader}>
<Text>{moment(section.eventDate).format('ll')}</Text>
</View>
);
};
toggleExpanded = () => {
this.setState({ collapsed: !this.state.collapsed });
};
setSections = sections => {
this.setState({
activeSections: sections.includes(undefined) ? : sections,
});
};
_renderHeader(section, index, isActive, sections) {
return (
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</Animatable.View>
);
}
_renderContent(section, i, isActive, sections) {
return (
<Animatable.View
duration={300}
transition="backgroundColor"
style={[styles.accordionCont, isActive ? styles.active : styles.inactive]}
transition="backgroundColor"
>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Insurer Address
</Animatable.Text>
<Animatable.Text
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
{section.addressStreet}
</Animatable.Text>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Policy Number
</Animatable.Text>
<Animatable.Text
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
{section.policyNumber}
</Animatable.Text>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Additional Details
</Animatable.Text>
</Animatable.View>
);
}
_updateSections = activeSections => {
this.setState({ activeSections });
};
render() {
const { multipleSelect, activeSections, poiHistoryData } = this.state;
return (
<View style={styles.container}>
<ScrollView contentContainerStyle={{ paddingTop: 30 }}>
<TouchableOpacity onPress={this.toggleExpanded}>
<View style={styles.header}>
<Text style={styles.headerText}>View Data</Text>
</View>
</TouchableOpacity>
<Accordion
sections={newData}
activeSections={activeSections}
touchableComponent={TouchableOpacity}
expandMultiple={multipleSelect}
renderSectionTitle={this._renderSectionTitle}
renderHeader={this._renderHeader}
renderContent={this._renderContent}
duration={400}
onChange={this._updateSections}
/>
</ScrollView>
</View>
) }
}
If you see the Heading 'Name' and 'City', these are the headings and I am displaying the value just below it. But right now everything is stacked and aligned to the left. I want 'Other Insurer' and its value to be appear on the left of the screen and 'Other Insured' and its value to be aligned to the right of the screen.
CSS that I am using-
headerCont: {
fontWeight:'bold'
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'flex-end',
alignItems: 'center'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
flex: 1,
flexDirection: 'row'
}
Screenshot-
Can someone please suggest me how can I handle this gracefully. Thanks in advance.
css react-native react-native-stylesheet
I am building a cross platform mobile application using react-native. I am implementing a functionality where I am displaying the data in Accordion. I want the data to be aligned to the left and right in the Accordion Header and Accordion Body but I am not able to achieve that.
Here is my code-
import React, { Component } from 'react';
import {
Switch,
ScrollView,
StyleSheet,
Text,
Image,
View,
TouchableOpacity,
} from 'react-native';
import * as Animatable from 'react-native-animatable';
import Collapsible from 'react-native-collapsible';
import Accordion from 'react-native-collapsible/Accordion';
const axios = require('axios');
import moment from 'moment'
import Dialog, { SlideAnimation, DialogContent } from 'react-native-popup-dialog';
export default class AccordionScreen extends Component {
constructor (){
super();
this.state = {
activeSections: ,
collapsed: true,
multipleSelect: false,
newData:,
visible: false
};
}
componentDidMount(){
this.fetchData();
}
fetchData() {
axios({
method: "POST",
url: "URL",
headers: {
"Content-Type": "application/json",
"Authorization": this.props.navigation.getParam('authorizationToken', undefined)
},
data: {}
})
.then (response => {
if (response.status === 200 ){
if (response.data.newData.length > 0 ){
this.setState({
newData: response.data.newData
});
//console.log(activeSections);
}
else {
return <h1>No Data!</h1>
}
}
else{
throw "Request resulted in NOT 200";
}
})
.catch(error => {
console.log(error);
});
}
_renderSectionTitle = section => {
return (
<View style={styles.titleHeader}>
<Text>{moment(section.eventDate).format('ll')}</Text>
</View>
);
};
toggleExpanded = () => {
this.setState({ collapsed: !this.state.collapsed });
};
setSections = sections => {
this.setState({
activeSections: sections.includes(undefined) ? : sections,
});
};
_renderHeader(section, index, isActive, sections) {
return (
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</Animatable.View>
);
}
_renderContent(section, i, isActive, sections) {
return (
<Animatable.View
duration={300}
transition="backgroundColor"
style={[styles.accordionCont, isActive ? styles.active : styles.inactive]}
transition="backgroundColor"
>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Insurer Address
</Animatable.Text>
<Animatable.Text
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
{section.addressStreet}
</Animatable.Text>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Policy Number
</Animatable.Text>
<Animatable.Text
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
{section.policyNumber}
</Animatable.Text>
<Animatable.Text
style={styles.headerCont}
duration={300}
easing="ease-out"
animation={isActive ? 'zoomIn' : false}>
Additional Details
</Animatable.Text>
</Animatable.View>
);
}
_updateSections = activeSections => {
this.setState({ activeSections });
};
render() {
const { multipleSelect, activeSections, poiHistoryData } = this.state;
return (
<View style={styles.container}>
<ScrollView contentContainerStyle={{ paddingTop: 30 }}>
<TouchableOpacity onPress={this.toggleExpanded}>
<View style={styles.header}>
<Text style={styles.headerText}>View Data</Text>
</View>
</TouchableOpacity>
<Accordion
sections={newData}
activeSections={activeSections}
touchableComponent={TouchableOpacity}
expandMultiple={multipleSelect}
renderSectionTitle={this._renderSectionTitle}
renderHeader={this._renderHeader}
renderContent={this._renderContent}
duration={400}
onChange={this._updateSections}
/>
</ScrollView>
</View>
) }
}
If you see the Heading 'Name' and 'City', these are the headings and I am displaying the value just below it. But right now everything is stacked and aligned to the left. I want 'Other Insurer' and its value to be appear on the left of the screen and 'Other Insured' and its value to be aligned to the right of the screen.
CSS that I am using-
headerCont: {
fontWeight:'bold'
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'flex-end',
alignItems: 'center'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
flex: 1,
flexDirection: 'row'
}
Screenshot-
Can someone please suggest me how can I handle this gracefully. Thanks in advance.
css react-native react-native-stylesheet
css react-native react-native-stylesheet
edited Dec 29 '18 at 7:35
techie questie
asked Dec 29 '18 at 6:24
techie questietechie questie
232419
232419
take the screen shot of your issue and attach.
– Malik Adil
Dec 29 '18 at 6:28
add a comment |
take the screen shot of your issue and attach.
– Malik Adil
Dec 29 '18 at 6:28
take the screen shot of your issue and attach.
– Malik Adil
Dec 29 '18 at 6:28
take the screen shot of your issue and attach.
– Malik Adil
Dec 29 '18 at 6:28
add a comment |
2 Answers
2
active
oldest
votes
Replace your Animatable view this
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<View style={styles.viewContainer}>
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</View>
</Animatable.View>
And your css
headerCont: {
fontWeight:'bold',
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems:'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'flex-end'
},
viewContainer:{
flex: 1,
flexDirection: 'row'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
}
I have tried this but it doesn't seem to be working.
– techie questie
Dec 29 '18 at 6:39
can you share your screen shot.
– Malik Adil
Dec 29 '18 at 6:42
updated screenshot. It moves my accordion icon to the left and also no equal spacing in between the left and right content.
– techie questie
Dec 29 '18 at 6:47
I have update the Answer hope this will work for you
– Malik Adil
Dec 29 '18 at 6:56
if you feel any other issue plz share the latest screen shot
– Malik Adil
Dec 29 '18 at 6:57
|
show 10 more comments
hopefully this is work for you
import React, { Component } from 'react';
import { AppRegistry, View,Text } from 'react-native';
export default class FlexDirectionBasics extends Component {
render() {
return (
// Try setting `flexDirection` to `column`.
<View style={{flex: 1, flexDirection: 'row'}}>
<View style={{flex:1, alignItems:'center', backgroundColor: 'steelblue'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
<View style={{flex:1, alignItems:'center', backgroundColor: 'red'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => FlexDirectionBasics);
it's work for me
– Malik Adil
Dec 29 '18 at 7:29
I test this on this site facebook.github.io/react-native/docs/flexbox
– Malik Adil
Dec 29 '18 at 7:29
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53967236%2fhow-to-align-view-component-to-left-and-right-equally-in-react-native%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Replace your Animatable view this
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<View style={styles.viewContainer}>
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</View>
</Animatable.View>
And your css
headerCont: {
fontWeight:'bold',
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems:'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'flex-end'
},
viewContainer:{
flex: 1,
flexDirection: 'row'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
}
I have tried this but it doesn't seem to be working.
– techie questie
Dec 29 '18 at 6:39
can you share your screen shot.
– Malik Adil
Dec 29 '18 at 6:42
updated screenshot. It moves my accordion icon to the left and also no equal spacing in between the left and right content.
– techie questie
Dec 29 '18 at 6:47
I have update the Answer hope this will work for you
– Malik Adil
Dec 29 '18 at 6:56
if you feel any other issue plz share the latest screen shot
– Malik Adil
Dec 29 '18 at 6:57
|
show 10 more comments
Replace your Animatable view this
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<View style={styles.viewContainer}>
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</View>
</Animatable.View>
And your css
headerCont: {
fontWeight:'bold',
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems:'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'flex-end'
},
viewContainer:{
flex: 1,
flexDirection: 'row'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
}
I have tried this but it doesn't seem to be working.
– techie questie
Dec 29 '18 at 6:39
can you share your screen shot.
– Malik Adil
Dec 29 '18 at 6:42
updated screenshot. It moves my accordion icon to the left and also no equal spacing in between the left and right content.
– techie questie
Dec 29 '18 at 6:47
I have update the Answer hope this will work for you
– Malik Adil
Dec 29 '18 at 6:56
if you feel any other issue plz share the latest screen shot
– Malik Adil
Dec 29 '18 at 6:57
|
show 10 more comments
Replace your Animatable view this
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<View style={styles.viewContainer}>
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</View>
</Animatable.View>
And your css
headerCont: {
fontWeight:'bold',
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems:'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'flex-end'
},
viewContainer:{
flex: 1,
flexDirection: 'row'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
}
Replace your Animatable view this
<Animatable.View
duration={400}
style={[styles.header, isActive ? styles.active : styles.inactive]}
transition="backgroundColor">
{
isActive ?
<Image source={require('../assets/images/upArrow.png')}
style={{width: 15, height: 15, alignSelf: 'flex-end'}}
/>
:
<Image source={require('../assets/images/downArrow.png')}
style={{width: 15, height: 15 , alignSelf: 'flex-end'}}
/>
}
<View style={styles.viewContainer}>
<Animatable.View style={styles.leftContainer}>
<Animatable.Text style={styles.headerCont}>Name </Animatable.Text>
<Animatable.Text>{section.name} </Animatable.Text>
</Animatable.View>
<Animatable.View style={styles.rightContainer} >
<Animatable.Text style={styles.headerCont}>City</Animatable.Text>
<Animatable.Text>{section.firstName} {section.lastName} </Animatable.Text>
</Animatable.View>
</View>
</Animatable.View>
And your css
headerCont: {
fontWeight:'bold',
},
leftContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems:'flex-start'
},
rightContainer: {
flex: 1,
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'flex-end'
},
viewContainer:{
flex: 1,
flexDirection: 'row'
},
header: {
backgroundColor: '#24abc1',
padding: 5,
marginLeft:5,
marginRight:5,
}
edited Dec 29 '18 at 6:56
answered Dec 29 '18 at 6:32
Malik AdilMalik Adil
648
648
I have tried this but it doesn't seem to be working.
– techie questie
Dec 29 '18 at 6:39
can you share your screen shot.
– Malik Adil
Dec 29 '18 at 6:42
updated screenshot. It moves my accordion icon to the left and also no equal spacing in between the left and right content.
– techie questie
Dec 29 '18 at 6:47
I have update the Answer hope this will work for you
– Malik Adil
Dec 29 '18 at 6:56
if you feel any other issue plz share the latest screen shot
– Malik Adil
Dec 29 '18 at 6:57
|
show 10 more comments
I have tried this but it doesn't seem to be working.
– techie questie
Dec 29 '18 at 6:39
can you share your screen shot.
– Malik Adil
Dec 29 '18 at 6:42
updated screenshot. It moves my accordion icon to the left and also no equal spacing in between the left and right content.
– techie questie
Dec 29 '18 at 6:47
I have update the Answer hope this will work for you
– Malik Adil
Dec 29 '18 at 6:56
if you feel any other issue plz share the latest screen shot
– Malik Adil
Dec 29 '18 at 6:57
I have tried this but it doesn't seem to be working.
– techie questie
Dec 29 '18 at 6:39
I have tried this but it doesn't seem to be working.
– techie questie
Dec 29 '18 at 6:39
can you share your screen shot.
– Malik Adil
Dec 29 '18 at 6:42
can you share your screen shot.
– Malik Adil
Dec 29 '18 at 6:42
updated screenshot. It moves my accordion icon to the left and also no equal spacing in between the left and right content.
– techie questie
Dec 29 '18 at 6:47
updated screenshot. It moves my accordion icon to the left and also no equal spacing in between the left and right content.
– techie questie
Dec 29 '18 at 6:47
I have update the Answer hope this will work for you
– Malik Adil
Dec 29 '18 at 6:56
I have update the Answer hope this will work for you
– Malik Adil
Dec 29 '18 at 6:56
if you feel any other issue plz share the latest screen shot
– Malik Adil
Dec 29 '18 at 6:57
if you feel any other issue plz share the latest screen shot
– Malik Adil
Dec 29 '18 at 6:57
|
show 10 more comments
hopefully this is work for you
import React, { Component } from 'react';
import { AppRegistry, View,Text } from 'react-native';
export default class FlexDirectionBasics extends Component {
render() {
return (
// Try setting `flexDirection` to `column`.
<View style={{flex: 1, flexDirection: 'row'}}>
<View style={{flex:1, alignItems:'center', backgroundColor: 'steelblue'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
<View style={{flex:1, alignItems:'center', backgroundColor: 'red'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => FlexDirectionBasics);
it's work for me
– Malik Adil
Dec 29 '18 at 7:29
I test this on this site facebook.github.io/react-native/docs/flexbox
– Malik Adil
Dec 29 '18 at 7:29
add a comment |
hopefully this is work for you
import React, { Component } from 'react';
import { AppRegistry, View,Text } from 'react-native';
export default class FlexDirectionBasics extends Component {
render() {
return (
// Try setting `flexDirection` to `column`.
<View style={{flex: 1, flexDirection: 'row'}}>
<View style={{flex:1, alignItems:'center', backgroundColor: 'steelblue'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
<View style={{flex:1, alignItems:'center', backgroundColor: 'red'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => FlexDirectionBasics);
it's work for me
– Malik Adil
Dec 29 '18 at 7:29
I test this on this site facebook.github.io/react-native/docs/flexbox
– Malik Adil
Dec 29 '18 at 7:29
add a comment |
hopefully this is work for you
import React, { Component } from 'react';
import { AppRegistry, View,Text } from 'react-native';
export default class FlexDirectionBasics extends Component {
render() {
return (
// Try setting `flexDirection` to `column`.
<View style={{flex: 1, flexDirection: 'row'}}>
<View style={{flex:1, alignItems:'center', backgroundColor: 'steelblue'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
<View style={{flex:1, alignItems:'center', backgroundColor: 'red'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => FlexDirectionBasics);
hopefully this is work for you
import React, { Component } from 'react';
import { AppRegistry, View,Text } from 'react-native';
export default class FlexDirectionBasics extends Component {
render() {
return (
// Try setting `flexDirection` to `column`.
<View style={{flex: 1, flexDirection: 'row'}}>
<View style={{flex:1, alignItems:'center', backgroundColor: 'steelblue'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
<View style={{flex:1, alignItems:'center', backgroundColor: 'red'}} >
<Text>Name</Text>
<Text>ABC</Text>
</View>
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => FlexDirectionBasics);
answered Dec 29 '18 at 7:29
Malik AdilMalik Adil
648
648
it's work for me
– Malik Adil
Dec 29 '18 at 7:29
I test this on this site facebook.github.io/react-native/docs/flexbox
– Malik Adil
Dec 29 '18 at 7:29
add a comment |
it's work for me
– Malik Adil
Dec 29 '18 at 7:29
I test this on this site facebook.github.io/react-native/docs/flexbox
– Malik Adil
Dec 29 '18 at 7:29
it's work for me
– Malik Adil
Dec 29 '18 at 7:29
it's work for me
– Malik Adil
Dec 29 '18 at 7:29
I test this on this site facebook.github.io/react-native/docs/flexbox
– Malik Adil
Dec 29 '18 at 7:29
I test this on this site facebook.github.io/react-native/docs/flexbox
– Malik Adil
Dec 29 '18 at 7:29
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53967236%2fhow-to-align-view-component-to-left-and-right-equally-in-react-native%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EiJ2LrQOIu,G2j95axXpcSpv
take the screen shot of your issue and attach.
– Malik Adil
Dec 29 '18 at 6:28