How do you write a Java program to authenticate to an Aurora MySQL database?
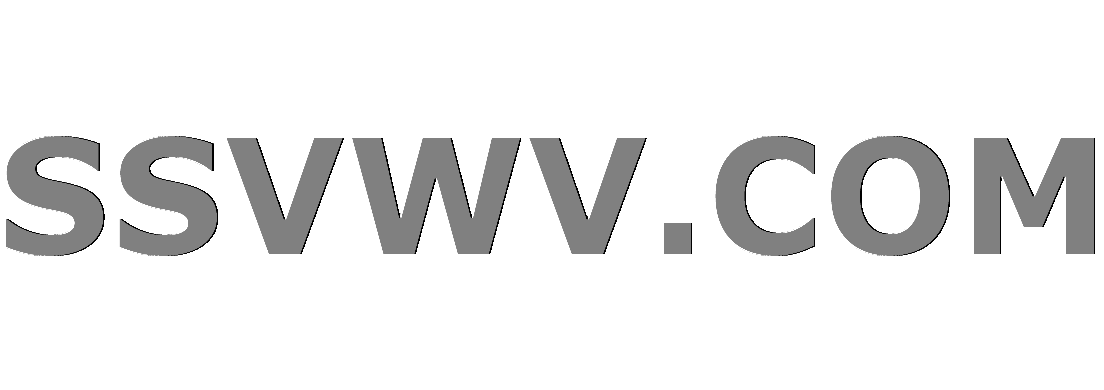
Multi tool use
I am having a problem writing a Java program that will run a SQL query on an Aurora database.
I know my database's name is goodnameofdb.
I run this command: aws rds describe-db-instances | grep DBInstanceIdentifier
I see this:
"ReadReplicaDBInstanceIdentifiers": ,
"DBInstanceIdentifier": "goodnameofdb"
"ReadReplicaDBInstanceIdentifiers": ,
"DBInstanceIdentifier": "goodnameofdb-us-west-2b"
I know my Aurora database's address. I run this command: aws rds describe-db-instances | grep goodnameofdb-us-west-2b.abcdef123456
I see this:
"Address": "goodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com"
My Java program compiles. I have the driver in the correct directory too.
I have a Java program with this as the code:
import java.sql.*;
class MysqlCon{
public static void main(String args){
try{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(
"jdbc:mysql://goodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com:3306/goodnameofdb","jdoe","securepassword");
Statement stmt=con.createStatement();
ResultSet rs=stmt.executeQuery("show tables");
while(rs.next())
System.out.println(rs.getInt(1)+" "+rs.getString(2)+" "+rs.getString(3));
con.close();
}catch(Exception e){ System.out.println(e);}
}
}
When I run the compiled program, it does not work. I see this error:
com.mysql.jdbc.exceptions.MySQLSyntaxErrorException: Unknown database 'goodnameofdb'
I am running my Java program from an EC-2 instance that is in the same VPC that the Aurora database is in. (I checked via AWS CLI commands.) I expect the program to connect to the Aurora database and authenticate.
What is wrong with my connection JDBC connection string? Or why am I getting this error?
Update: when I remove the "goodnameofdb" after the ":3306/" part of the string and recompile the program, I get an error when I run the program. The error that I receive is
java.sql.SQLException: No database selected
I therefore think that a database name must be there. My AWS CLI commands seem to tell me that I have the name correct.
java mysql amazon-web-services amazon-rds-aurora
|
show 1 more comment
I am having a problem writing a Java program that will run a SQL query on an Aurora database.
I know my database's name is goodnameofdb.
I run this command: aws rds describe-db-instances | grep DBInstanceIdentifier
I see this:
"ReadReplicaDBInstanceIdentifiers": ,
"DBInstanceIdentifier": "goodnameofdb"
"ReadReplicaDBInstanceIdentifiers": ,
"DBInstanceIdentifier": "goodnameofdb-us-west-2b"
I know my Aurora database's address. I run this command: aws rds describe-db-instances | grep goodnameofdb-us-west-2b.abcdef123456
I see this:
"Address": "goodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com"
My Java program compiles. I have the driver in the correct directory too.
I have a Java program with this as the code:
import java.sql.*;
class MysqlCon{
public static void main(String args){
try{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(
"jdbc:mysql://goodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com:3306/goodnameofdb","jdoe","securepassword");
Statement stmt=con.createStatement();
ResultSet rs=stmt.executeQuery("show tables");
while(rs.next())
System.out.println(rs.getInt(1)+" "+rs.getString(2)+" "+rs.getString(3));
con.close();
}catch(Exception e){ System.out.println(e);}
}
}
When I run the compiled program, it does not work. I see this error:
com.mysql.jdbc.exceptions.MySQLSyntaxErrorException: Unknown database 'goodnameofdb'
I am running my Java program from an EC-2 instance that is in the same VPC that the Aurora database is in. (I checked via AWS CLI commands.) I expect the program to connect to the Aurora database and authenticate.
What is wrong with my connection JDBC connection string? Or why am I getting this error?
Update: when I remove the "goodnameofdb" after the ":3306/" part of the string and recompile the program, I get an error when I run the program. The error that I receive is
java.sql.SQLException: No database selected
I therefore think that a database name must be there. My AWS CLI commands seem to tell me that I have the name correct.
java mysql amazon-web-services amazon-rds-aurora
Are you able to open the DB using mysql tools from your ec2 instance? (eg.mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword goodnameofdb
)
– Jason Braucht
Dec 28 '18 at 0:33
No. I get "ERROR 1049 (42000): Unknown database goodnameofdb"
– Ranjit
Dec 28 '18 at 0:48
Does it work withgoodnameofdb-us-west-2b
?
– Robert Bain
Dec 28 '18 at 1:02
No. Please note that the endpoint (as the AWS console shows) includes "abcdef123456". I tried variations of goodnameofdb (including using the endpoint that the AWS console showed). They all come back with "Unknown database..."
– Ranjit
Dec 28 '18 at 1:48
1
...amazonaws.com:3306/goodnameofdb
<< why isgoodnameofdb
here? This is where you specify the schema name you want to use as the default database schema for this connection. You are connecting to the server fine, then trying to use a database (schema) name that does not exist there. Replace this with the correct value, or eliminate it.
– Michael - sqlbot
Dec 28 '18 at 2:55
|
show 1 more comment
I am having a problem writing a Java program that will run a SQL query on an Aurora database.
I know my database's name is goodnameofdb.
I run this command: aws rds describe-db-instances | grep DBInstanceIdentifier
I see this:
"ReadReplicaDBInstanceIdentifiers": ,
"DBInstanceIdentifier": "goodnameofdb"
"ReadReplicaDBInstanceIdentifiers": ,
"DBInstanceIdentifier": "goodnameofdb-us-west-2b"
I know my Aurora database's address. I run this command: aws rds describe-db-instances | grep goodnameofdb-us-west-2b.abcdef123456
I see this:
"Address": "goodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com"
My Java program compiles. I have the driver in the correct directory too.
I have a Java program with this as the code:
import java.sql.*;
class MysqlCon{
public static void main(String args){
try{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(
"jdbc:mysql://goodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com:3306/goodnameofdb","jdoe","securepassword");
Statement stmt=con.createStatement();
ResultSet rs=stmt.executeQuery("show tables");
while(rs.next())
System.out.println(rs.getInt(1)+" "+rs.getString(2)+" "+rs.getString(3));
con.close();
}catch(Exception e){ System.out.println(e);}
}
}
When I run the compiled program, it does not work. I see this error:
com.mysql.jdbc.exceptions.MySQLSyntaxErrorException: Unknown database 'goodnameofdb'
I am running my Java program from an EC-2 instance that is in the same VPC that the Aurora database is in. (I checked via AWS CLI commands.) I expect the program to connect to the Aurora database and authenticate.
What is wrong with my connection JDBC connection string? Or why am I getting this error?
Update: when I remove the "goodnameofdb" after the ":3306/" part of the string and recompile the program, I get an error when I run the program. The error that I receive is
java.sql.SQLException: No database selected
I therefore think that a database name must be there. My AWS CLI commands seem to tell me that I have the name correct.
java mysql amazon-web-services amazon-rds-aurora
I am having a problem writing a Java program that will run a SQL query on an Aurora database.
I know my database's name is goodnameofdb.
I run this command: aws rds describe-db-instances | grep DBInstanceIdentifier
I see this:
"ReadReplicaDBInstanceIdentifiers": ,
"DBInstanceIdentifier": "goodnameofdb"
"ReadReplicaDBInstanceIdentifiers": ,
"DBInstanceIdentifier": "goodnameofdb-us-west-2b"
I know my Aurora database's address. I run this command: aws rds describe-db-instances | grep goodnameofdb-us-west-2b.abcdef123456
I see this:
"Address": "goodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com"
My Java program compiles. I have the driver in the correct directory too.
I have a Java program with this as the code:
import java.sql.*;
class MysqlCon{
public static void main(String args){
try{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection(
"jdbc:mysql://goodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com:3306/goodnameofdb","jdoe","securepassword");
Statement stmt=con.createStatement();
ResultSet rs=stmt.executeQuery("show tables");
while(rs.next())
System.out.println(rs.getInt(1)+" "+rs.getString(2)+" "+rs.getString(3));
con.close();
}catch(Exception e){ System.out.println(e);}
}
}
When I run the compiled program, it does not work. I see this error:
com.mysql.jdbc.exceptions.MySQLSyntaxErrorException: Unknown database 'goodnameofdb'
I am running my Java program from an EC-2 instance that is in the same VPC that the Aurora database is in. (I checked via AWS CLI commands.) I expect the program to connect to the Aurora database and authenticate.
What is wrong with my connection JDBC connection string? Or why am I getting this error?
Update: when I remove the "goodnameofdb" after the ":3306/" part of the string and recompile the program, I get an error when I run the program. The error that I receive is
java.sql.SQLException: No database selected
I therefore think that a database name must be there. My AWS CLI commands seem to tell me that I have the name correct.
java mysql amazon-web-services amazon-rds-aurora
java mysql amazon-web-services amazon-rds-aurora
edited Dec 28 '18 at 3:20
asked Dec 28 '18 at 0:19
Ranjit
113
113
Are you able to open the DB using mysql tools from your ec2 instance? (eg.mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword goodnameofdb
)
– Jason Braucht
Dec 28 '18 at 0:33
No. I get "ERROR 1049 (42000): Unknown database goodnameofdb"
– Ranjit
Dec 28 '18 at 0:48
Does it work withgoodnameofdb-us-west-2b
?
– Robert Bain
Dec 28 '18 at 1:02
No. Please note that the endpoint (as the AWS console shows) includes "abcdef123456". I tried variations of goodnameofdb (including using the endpoint that the AWS console showed). They all come back with "Unknown database..."
– Ranjit
Dec 28 '18 at 1:48
1
...amazonaws.com:3306/goodnameofdb
<< why isgoodnameofdb
here? This is where you specify the schema name you want to use as the default database schema for this connection. You are connecting to the server fine, then trying to use a database (schema) name that does not exist there. Replace this with the correct value, or eliminate it.
– Michael - sqlbot
Dec 28 '18 at 2:55
|
show 1 more comment
Are you able to open the DB using mysql tools from your ec2 instance? (eg.mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword goodnameofdb
)
– Jason Braucht
Dec 28 '18 at 0:33
No. I get "ERROR 1049 (42000): Unknown database goodnameofdb"
– Ranjit
Dec 28 '18 at 0:48
Does it work withgoodnameofdb-us-west-2b
?
– Robert Bain
Dec 28 '18 at 1:02
No. Please note that the endpoint (as the AWS console shows) includes "abcdef123456". I tried variations of goodnameofdb (including using the endpoint that the AWS console showed). They all come back with "Unknown database..."
– Ranjit
Dec 28 '18 at 1:48
1
...amazonaws.com:3306/goodnameofdb
<< why isgoodnameofdb
here? This is where you specify the schema name you want to use as the default database schema for this connection. You are connecting to the server fine, then trying to use a database (schema) name that does not exist there. Replace this with the correct value, or eliminate it.
– Michael - sqlbot
Dec 28 '18 at 2:55
Are you able to open the DB using mysql tools from your ec2 instance? (eg.
mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword goodnameofdb
)– Jason Braucht
Dec 28 '18 at 0:33
Are you able to open the DB using mysql tools from your ec2 instance? (eg.
mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword goodnameofdb
)– Jason Braucht
Dec 28 '18 at 0:33
No. I get "ERROR 1049 (42000): Unknown database goodnameofdb"
– Ranjit
Dec 28 '18 at 0:48
No. I get "ERROR 1049 (42000): Unknown database goodnameofdb"
– Ranjit
Dec 28 '18 at 0:48
Does it work with
goodnameofdb-us-west-2b
?– Robert Bain
Dec 28 '18 at 1:02
Does it work with
goodnameofdb-us-west-2b
?– Robert Bain
Dec 28 '18 at 1:02
No. Please note that the endpoint (as the AWS console shows) includes "abcdef123456". I tried variations of goodnameofdb (including using the endpoint that the AWS console showed). They all come back with "Unknown database..."
– Ranjit
Dec 28 '18 at 1:48
No. Please note that the endpoint (as the AWS console shows) includes "abcdef123456". I tried variations of goodnameofdb (including using the endpoint that the AWS console showed). They all come back with "Unknown database..."
– Ranjit
Dec 28 '18 at 1:48
1
1
...amazonaws.com:3306/goodnameofdb
<< why is goodnameofdb
here? This is where you specify the schema name you want to use as the default database schema for this connection. You are connecting to the server fine, then trying to use a database (schema) name that does not exist there. Replace this with the correct value, or eliminate it.– Michael - sqlbot
Dec 28 '18 at 2:55
...amazonaws.com:3306/goodnameofdb
<< why is goodnameofdb
here? This is where you specify the schema name you want to use as the default database schema for this connection. You are connecting to the server fine, then trying to use a database (schema) name that does not exist there. Replace this with the correct value, or eliminate it.– Michael - sqlbot
Dec 28 '18 at 2:55
|
show 1 more comment
2 Answers
2
active
oldest
votes
It seems that the database "goodnameofdb " is not exist.
You can check from mysql commond line.
mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword;
show databases;
If goodnameofdb is not exist, you need create a db withe the name goodnameofdb.
mysql> create DATABASE goodnameofdb;
Then, try again.
Our team uses aurora also, aurora is compatible with mysql.
add a comment |
When you create an Aurora cluster, you mention a database-name
name along with master-user
and master-user-password
. Here database refers to a default mysql database (also referred to schema
at times) and NOT your db cluster identifier (in your example "goodnameofdb").
In your case, it looks like the default database that you created is either NOT NAMED goodnameofdb
or you DIDN'T PROVIDE one explicitly, thereby making Aurora create some default database for you.
You cannot use AWS CLI to fetch the database name of your db instance. Use your master-user credentials, connect to the db via mysql cli (or something similar) and list the databases (show databases;
) that are there in your Aurora cluster.
I'm fairly sure you are trying to use a non-existent mysql database.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53952311%2fhow-do-you-write-a-java-program-to-authenticate-to-an-aurora-mysql-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
It seems that the database "goodnameofdb " is not exist.
You can check from mysql commond line.
mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword;
show databases;
If goodnameofdb is not exist, you need create a db withe the name goodnameofdb.
mysql> create DATABASE goodnameofdb;
Then, try again.
Our team uses aurora also, aurora is compatible with mysql.
add a comment |
It seems that the database "goodnameofdb " is not exist.
You can check from mysql commond line.
mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword;
show databases;
If goodnameofdb is not exist, you need create a db withe the name goodnameofdb.
mysql> create DATABASE goodnameofdb;
Then, try again.
Our team uses aurora also, aurora is compatible with mysql.
add a comment |
It seems that the database "goodnameofdb " is not exist.
You can check from mysql commond line.
mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword;
show databases;
If goodnameofdb is not exist, you need create a db withe the name goodnameofdb.
mysql> create DATABASE goodnameofdb;
Then, try again.
Our team uses aurora also, aurora is compatible with mysql.
It seems that the database "goodnameofdb " is not exist.
You can check from mysql commond line.
mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword;
show databases;
If goodnameofdb is not exist, you need create a db withe the name goodnameofdb.
mysql> create DATABASE goodnameofdb;
Then, try again.
Our team uses aurora also, aurora is compatible with mysql.
answered Dec 28 '18 at 5:18


user7378390
112
112
add a comment |
add a comment |
When you create an Aurora cluster, you mention a database-name
name along with master-user
and master-user-password
. Here database refers to a default mysql database (also referred to schema
at times) and NOT your db cluster identifier (in your example "goodnameofdb").
In your case, it looks like the default database that you created is either NOT NAMED goodnameofdb
or you DIDN'T PROVIDE one explicitly, thereby making Aurora create some default database for you.
You cannot use AWS CLI to fetch the database name of your db instance. Use your master-user credentials, connect to the db via mysql cli (or something similar) and list the databases (show databases;
) that are there in your Aurora cluster.
I'm fairly sure you are trying to use a non-existent mysql database.
add a comment |
When you create an Aurora cluster, you mention a database-name
name along with master-user
and master-user-password
. Here database refers to a default mysql database (also referred to schema
at times) and NOT your db cluster identifier (in your example "goodnameofdb").
In your case, it looks like the default database that you created is either NOT NAMED goodnameofdb
or you DIDN'T PROVIDE one explicitly, thereby making Aurora create some default database for you.
You cannot use AWS CLI to fetch the database name of your db instance. Use your master-user credentials, connect to the db via mysql cli (or something similar) and list the databases (show databases;
) that are there in your Aurora cluster.
I'm fairly sure you are trying to use a non-existent mysql database.
add a comment |
When you create an Aurora cluster, you mention a database-name
name along with master-user
and master-user-password
. Here database refers to a default mysql database (also referred to schema
at times) and NOT your db cluster identifier (in your example "goodnameofdb").
In your case, it looks like the default database that you created is either NOT NAMED goodnameofdb
or you DIDN'T PROVIDE one explicitly, thereby making Aurora create some default database for you.
You cannot use AWS CLI to fetch the database name of your db instance. Use your master-user credentials, connect to the db via mysql cli (or something similar) and list the databases (show databases;
) that are there in your Aurora cluster.
I'm fairly sure you are trying to use a non-existent mysql database.
When you create an Aurora cluster, you mention a database-name
name along with master-user
and master-user-password
. Here database refers to a default mysql database (also referred to schema
at times) and NOT your db cluster identifier (in your example "goodnameofdb").
In your case, it looks like the default database that you created is either NOT NAMED goodnameofdb
or you DIDN'T PROVIDE one explicitly, thereby making Aurora create some default database for you.
You cannot use AWS CLI to fetch the database name of your db instance. Use your master-user credentials, connect to the db via mysql cli (or something similar) and list the databases (show databases;
) that are there in your Aurora cluster.
I'm fairly sure you are trying to use a non-existent mysql database.
answered Dec 29 '18 at 3:12


Karthik Rajan
442316
442316
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53952311%2fhow-do-you-write-a-java-program-to-authenticate-to-an-aurora-mysql-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XA8pI41 UuSaTgu
Are you able to open the DB using mysql tools from your ec2 instance? (eg.
mysql -hgoodnameofdb-us-west-2b.abcdef123456.us-east-2.rds.amazonaws.com -ujdoe -psecurepassword goodnameofdb
)– Jason Braucht
Dec 28 '18 at 0:33
No. I get "ERROR 1049 (42000): Unknown database goodnameofdb"
– Ranjit
Dec 28 '18 at 0:48
Does it work with
goodnameofdb-us-west-2b
?– Robert Bain
Dec 28 '18 at 1:02
No. Please note that the endpoint (as the AWS console shows) includes "abcdef123456". I tried variations of goodnameofdb (including using the endpoint that the AWS console showed). They all come back with "Unknown database..."
– Ranjit
Dec 28 '18 at 1:48
1
...amazonaws.com:3306/goodnameofdb
<< why isgoodnameofdb
here? This is where you specify the schema name you want to use as the default database schema for this connection. You are connecting to the server fine, then trying to use a database (schema) name that does not exist there. Replace this with the correct value, or eliminate it.– Michael - sqlbot
Dec 28 '18 at 2:55