The most efficient method to get an object's attributes when the object is in a list in python?
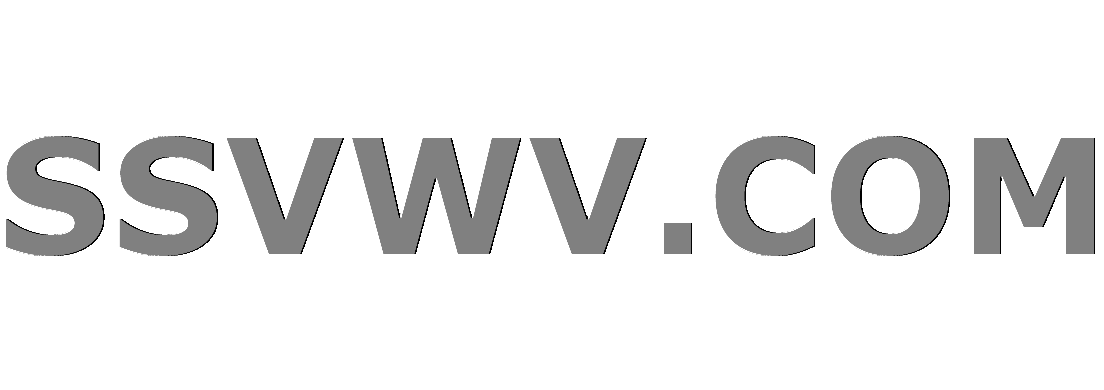
Multi tool use
For example:
import numpy as np
import datetime
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
l1 = [Test(i,i+1) for i in range(1000000)]
My solution is:
start_time = datetime.datetime.now()
l11 = np.array([v.atti1 for v in l1])
l12 = np.array([v.atti2 for v in l1])
print(datetime.datetime.now()-start_time)
It costs 0:00:00.234735 in my macbookpro2017.
It there a more efficient method to make it in python?
---edit1
It is not's not necessary to use numpy.Here is another solution:
l11 =
l12 =
start_time = datetime.datetime.now()
for v in l1:
l11.append(v.atti1)
l12.append(v.atti2)
print(datetime.datetime.now()-start_time)
It costs 0:00:00.225412
---edit2
Here is a bad solution:
l11 = np.array()
l12 = np.array()
start_time = datetime.datetime.now()
for v in l1:
l11 = np.append(l11,v.atti1)
l12 = np.append(l12,v.atti2)
print(datetime.datetime.now()-start_time)
python python-3.x
|
show 1 more comment
For example:
import numpy as np
import datetime
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
l1 = [Test(i,i+1) for i in range(1000000)]
My solution is:
start_time = datetime.datetime.now()
l11 = np.array([v.atti1 for v in l1])
l12 = np.array([v.atti2 for v in l1])
print(datetime.datetime.now()-start_time)
It costs 0:00:00.234735 in my macbookpro2017.
It there a more efficient method to make it in python?
---edit1
It is not's not necessary to use numpy.Here is another solution:
l11 =
l12 =
start_time = datetime.datetime.now()
for v in l1:
l11.append(v.atti1)
l12.append(v.atti2)
print(datetime.datetime.now()-start_time)
It costs 0:00:00.225412
---edit2
Here is a bad solution:
l11 = np.array()
l12 = np.array()
start_time = datetime.datetime.now()
for v in l1:
l11 = np.append(l11,v.atti1)
l12 = np.append(l12,v.atti2)
print(datetime.datetime.now()-start_time)
python python-3.x
1
I'm confused. What exactly are you trying to do? And if you're trying to evaluate how a bit of Python code performs, don't usedatetime.now()
, use thetimeit
module, which is designed for this purpose.
– Daniel Pryden
Dec 27 at 12:57
1
1. What are you actually trying to achieve? 2. What is the significance of usingnumpy
here? 3. Why do you think that0:00:00.234735
is slow for a list with 1 million objects? Anyway, 1 method for improving this code is to fetch both attributes in a single pass instead of iterating twice over the entire list
– DeepSpace
Dec 27 at 12:58
@DanielPryden Many modules' function return a list of object.I want to know what is the best way to get object's attitudes.
– DachuanZhao
Dec 27 at 13:05
@DeepSpace That's not necessary.I will edit the question.Thanks.
– DachuanZhao
Dec 27 at 13:07
@DachuanZhao: Two points of English terminology that I want to clarify. 1. You say "attitudes" but I assume you actually mean "attributes". Is that correct? If so, please clarify it in the question. 2. You ask for the "most effective method". Do you really mean "effective" (as in, able to produce the desired effect) or do you intend something like "efficient" (as in, producing the effect with the smallest amount of work expended)? Please clarify that as well, as that will affect what the answer should be.
– Daniel Pryden
Dec 27 at 13:11
|
show 1 more comment
For example:
import numpy as np
import datetime
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
l1 = [Test(i,i+1) for i in range(1000000)]
My solution is:
start_time = datetime.datetime.now()
l11 = np.array([v.atti1 for v in l1])
l12 = np.array([v.atti2 for v in l1])
print(datetime.datetime.now()-start_time)
It costs 0:00:00.234735 in my macbookpro2017.
It there a more efficient method to make it in python?
---edit1
It is not's not necessary to use numpy.Here is another solution:
l11 =
l12 =
start_time = datetime.datetime.now()
for v in l1:
l11.append(v.atti1)
l12.append(v.atti2)
print(datetime.datetime.now()-start_time)
It costs 0:00:00.225412
---edit2
Here is a bad solution:
l11 = np.array()
l12 = np.array()
start_time = datetime.datetime.now()
for v in l1:
l11 = np.append(l11,v.atti1)
l12 = np.append(l12,v.atti2)
print(datetime.datetime.now()-start_time)
python python-3.x
For example:
import numpy as np
import datetime
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
l1 = [Test(i,i+1) for i in range(1000000)]
My solution is:
start_time = datetime.datetime.now()
l11 = np.array([v.atti1 for v in l1])
l12 = np.array([v.atti2 for v in l1])
print(datetime.datetime.now()-start_time)
It costs 0:00:00.234735 in my macbookpro2017.
It there a more efficient method to make it in python?
---edit1
It is not's not necessary to use numpy.Here is another solution:
l11 =
l12 =
start_time = datetime.datetime.now()
for v in l1:
l11.append(v.atti1)
l12.append(v.atti2)
print(datetime.datetime.now()-start_time)
It costs 0:00:00.225412
---edit2
Here is a bad solution:
l11 = np.array()
l12 = np.array()
start_time = datetime.datetime.now()
for v in l1:
l11 = np.append(l11,v.atti1)
l12 = np.append(l12,v.atti2)
print(datetime.datetime.now()-start_time)
python python-3.x
python python-3.x
edited Dec 27 at 13:15
asked Dec 27 at 12:55
DachuanZhao
509
509
1
I'm confused. What exactly are you trying to do? And if you're trying to evaluate how a bit of Python code performs, don't usedatetime.now()
, use thetimeit
module, which is designed for this purpose.
– Daniel Pryden
Dec 27 at 12:57
1
1. What are you actually trying to achieve? 2. What is the significance of usingnumpy
here? 3. Why do you think that0:00:00.234735
is slow for a list with 1 million objects? Anyway, 1 method for improving this code is to fetch both attributes in a single pass instead of iterating twice over the entire list
– DeepSpace
Dec 27 at 12:58
@DanielPryden Many modules' function return a list of object.I want to know what is the best way to get object's attitudes.
– DachuanZhao
Dec 27 at 13:05
@DeepSpace That's not necessary.I will edit the question.Thanks.
– DachuanZhao
Dec 27 at 13:07
@DachuanZhao: Two points of English terminology that I want to clarify. 1. You say "attitudes" but I assume you actually mean "attributes". Is that correct? If so, please clarify it in the question. 2. You ask for the "most effective method". Do you really mean "effective" (as in, able to produce the desired effect) or do you intend something like "efficient" (as in, producing the effect with the smallest amount of work expended)? Please clarify that as well, as that will affect what the answer should be.
– Daniel Pryden
Dec 27 at 13:11
|
show 1 more comment
1
I'm confused. What exactly are you trying to do? And if you're trying to evaluate how a bit of Python code performs, don't usedatetime.now()
, use thetimeit
module, which is designed for this purpose.
– Daniel Pryden
Dec 27 at 12:57
1
1. What are you actually trying to achieve? 2. What is the significance of usingnumpy
here? 3. Why do you think that0:00:00.234735
is slow for a list with 1 million objects? Anyway, 1 method for improving this code is to fetch both attributes in a single pass instead of iterating twice over the entire list
– DeepSpace
Dec 27 at 12:58
@DanielPryden Many modules' function return a list of object.I want to know what is the best way to get object's attitudes.
– DachuanZhao
Dec 27 at 13:05
@DeepSpace That's not necessary.I will edit the question.Thanks.
– DachuanZhao
Dec 27 at 13:07
@DachuanZhao: Two points of English terminology that I want to clarify. 1. You say "attitudes" but I assume you actually mean "attributes". Is that correct? If so, please clarify it in the question. 2. You ask for the "most effective method". Do you really mean "effective" (as in, able to produce the desired effect) or do you intend something like "efficient" (as in, producing the effect with the smallest amount of work expended)? Please clarify that as well, as that will affect what the answer should be.
– Daniel Pryden
Dec 27 at 13:11
1
1
I'm confused. What exactly are you trying to do? And if you're trying to evaluate how a bit of Python code performs, don't use
datetime.now()
, use the timeit
module, which is designed for this purpose.– Daniel Pryden
Dec 27 at 12:57
I'm confused. What exactly are you trying to do? And if you're trying to evaluate how a bit of Python code performs, don't use
datetime.now()
, use the timeit
module, which is designed for this purpose.– Daniel Pryden
Dec 27 at 12:57
1
1
1. What are you actually trying to achieve? 2. What is the significance of using
numpy
here? 3. Why do you think that 0:00:00.234735
is slow for a list with 1 million objects? Anyway, 1 method for improving this code is to fetch both attributes in a single pass instead of iterating twice over the entire list– DeepSpace
Dec 27 at 12:58
1. What are you actually trying to achieve? 2. What is the significance of using
numpy
here? 3. Why do you think that 0:00:00.234735
is slow for a list with 1 million objects? Anyway, 1 method for improving this code is to fetch both attributes in a single pass instead of iterating twice over the entire list– DeepSpace
Dec 27 at 12:58
@DanielPryden Many modules' function return a list of object.I want to know what is the best way to get object's attitudes.
– DachuanZhao
Dec 27 at 13:05
@DanielPryden Many modules' function return a list of object.I want to know what is the best way to get object's attitudes.
– DachuanZhao
Dec 27 at 13:05
@DeepSpace That's not necessary.I will edit the question.Thanks.
– DachuanZhao
Dec 27 at 13:07
@DeepSpace That's not necessary.I will edit the question.Thanks.
– DachuanZhao
Dec 27 at 13:07
@DachuanZhao: Two points of English terminology that I want to clarify. 1. You say "attitudes" but I assume you actually mean "attributes". Is that correct? If so, please clarify it in the question. 2. You ask for the "most effective method". Do you really mean "effective" (as in, able to produce the desired effect) or do you intend something like "efficient" (as in, producing the effect with the smallest amount of work expended)? Please clarify that as well, as that will affect what the answer should be.
– Daniel Pryden
Dec 27 at 13:11
@DachuanZhao: Two points of English terminology that I want to clarify. 1. You say "attitudes" but I assume you actually mean "attributes". Is that correct? If so, please clarify it in the question. 2. You ask for the "most effective method". Do you really mean "effective" (as in, able to produce the desired effect) or do you intend something like "efficient" (as in, producing the effect with the smallest amount of work expended)? Please clarify that as well, as that will affect what the answer should be.
– Daniel Pryden
Dec 27 at 13:11
|
show 1 more comment
2 Answers
2
active
oldest
votes
There's no need to use numpy here, and generally list comprehension is good enough. I.e. l11 = [v.atti1 for v in lst]
is totally fine.
Conceptually, you must iterate over all objects and access attribute of every single one.
Metrics on "why you should not overengineer":
# numpy array builder
np.array([v.atti1 for v in lst])
np.array([v.atti2 for v in lst])
215 ms ± 3.69 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
This is slowing because you first building list with comprehension, and then re-allocate memory for np array and copy
# single list iteration with appending
l1 =
l2 =
for v in lst:
l1.append(v.atti1)
l2.append(v.atti2)
174 ms ± 384 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
Better, but you have a lot of function calls for .append
and eventually you're re-allocating and copying list.
# thing that you always start with, no pre-mature optimizations
l1 = [v.atti1 for v in lst]
l2 = [v.atti2 for v in lst]
99.3 ms ± 982 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
This is more readable, pythonic, does exactly what it says, and its faster. Internally, its faster because of low-level optimizations of comprehension.
Side note, CPython (that you're most probably using) starting from 3.5 (iirc) uses shared-key dictionaries to store object attributes, and starting from 3.6 it's merged with compact dict implementation. Both working great together — memory efficiency gives a huge boost to you raw performance.
Not sure if VM actually leverages shared dict when running comprehensions (probably not) but this must be left to VM optimizations in 99% of cases. High-level abstracted languages (like python) is really not about microoptimizations.
add a comment |
You can use self.__dict__
to return the dictionary of attributes and their values in Python.
import numpy as np
import datetime
import pandas as pd
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
def getAttr(self):
return self.__dict__
l1 = [Test(i,i+1).getAttr() for i in range(1000000)]
l1 = pd.DataFrame(l1)
l11 = list(l1['atti1'])
l12 = list(l1['atti2'])
How does this relate to question? OP is asking for lists with raw attributes, not dicts
– Slam
Dec 27 at 13:31
The ultimate goal, as I understand, is to get two different lists for two different attributes. This method creates a list of dictionaries of object attributes. A list comprehension or amap
or evenpandas.DataFrame
can be used to create 2 different lists later.
– Rishabh Mishra
Dec 27 at 13:51
Solution with pandas is slower by magnitude, literally.
– Slam
Dec 27 at 14:21
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945460%2fthe-most-efficient-method-to-get-an-objects-attributes-when-the-object-is-in-a%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
There's no need to use numpy here, and generally list comprehension is good enough. I.e. l11 = [v.atti1 for v in lst]
is totally fine.
Conceptually, you must iterate over all objects and access attribute of every single one.
Metrics on "why you should not overengineer":
# numpy array builder
np.array([v.atti1 for v in lst])
np.array([v.atti2 for v in lst])
215 ms ± 3.69 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
This is slowing because you first building list with comprehension, and then re-allocate memory for np array and copy
# single list iteration with appending
l1 =
l2 =
for v in lst:
l1.append(v.atti1)
l2.append(v.atti2)
174 ms ± 384 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
Better, but you have a lot of function calls for .append
and eventually you're re-allocating and copying list.
# thing that you always start with, no pre-mature optimizations
l1 = [v.atti1 for v in lst]
l2 = [v.atti2 for v in lst]
99.3 ms ± 982 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
This is more readable, pythonic, does exactly what it says, and its faster. Internally, its faster because of low-level optimizations of comprehension.
Side note, CPython (that you're most probably using) starting from 3.5 (iirc) uses shared-key dictionaries to store object attributes, and starting from 3.6 it's merged with compact dict implementation. Both working great together — memory efficiency gives a huge boost to you raw performance.
Not sure if VM actually leverages shared dict when running comprehensions (probably not) but this must be left to VM optimizations in 99% of cases. High-level abstracted languages (like python) is really not about microoptimizations.
add a comment |
There's no need to use numpy here, and generally list comprehension is good enough. I.e. l11 = [v.atti1 for v in lst]
is totally fine.
Conceptually, you must iterate over all objects and access attribute of every single one.
Metrics on "why you should not overengineer":
# numpy array builder
np.array([v.atti1 for v in lst])
np.array([v.atti2 for v in lst])
215 ms ± 3.69 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
This is slowing because you first building list with comprehension, and then re-allocate memory for np array and copy
# single list iteration with appending
l1 =
l2 =
for v in lst:
l1.append(v.atti1)
l2.append(v.atti2)
174 ms ± 384 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
Better, but you have a lot of function calls for .append
and eventually you're re-allocating and copying list.
# thing that you always start with, no pre-mature optimizations
l1 = [v.atti1 for v in lst]
l2 = [v.atti2 for v in lst]
99.3 ms ± 982 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
This is more readable, pythonic, does exactly what it says, and its faster. Internally, its faster because of low-level optimizations of comprehension.
Side note, CPython (that you're most probably using) starting from 3.5 (iirc) uses shared-key dictionaries to store object attributes, and starting from 3.6 it's merged with compact dict implementation. Both working great together — memory efficiency gives a huge boost to you raw performance.
Not sure if VM actually leverages shared dict when running comprehensions (probably not) but this must be left to VM optimizations in 99% of cases. High-level abstracted languages (like python) is really not about microoptimizations.
add a comment |
There's no need to use numpy here, and generally list comprehension is good enough. I.e. l11 = [v.atti1 for v in lst]
is totally fine.
Conceptually, you must iterate over all objects and access attribute of every single one.
Metrics on "why you should not overengineer":
# numpy array builder
np.array([v.atti1 for v in lst])
np.array([v.atti2 for v in lst])
215 ms ± 3.69 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
This is slowing because you first building list with comprehension, and then re-allocate memory for np array and copy
# single list iteration with appending
l1 =
l2 =
for v in lst:
l1.append(v.atti1)
l2.append(v.atti2)
174 ms ± 384 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
Better, but you have a lot of function calls for .append
and eventually you're re-allocating and copying list.
# thing that you always start with, no pre-mature optimizations
l1 = [v.atti1 for v in lst]
l2 = [v.atti2 for v in lst]
99.3 ms ± 982 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
This is more readable, pythonic, does exactly what it says, and its faster. Internally, its faster because of low-level optimizations of comprehension.
Side note, CPython (that you're most probably using) starting from 3.5 (iirc) uses shared-key dictionaries to store object attributes, and starting from 3.6 it's merged with compact dict implementation. Both working great together — memory efficiency gives a huge boost to you raw performance.
Not sure if VM actually leverages shared dict when running comprehensions (probably not) but this must be left to VM optimizations in 99% of cases. High-level abstracted languages (like python) is really not about microoptimizations.
There's no need to use numpy here, and generally list comprehension is good enough. I.e. l11 = [v.atti1 for v in lst]
is totally fine.
Conceptually, you must iterate over all objects and access attribute of every single one.
Metrics on "why you should not overengineer":
# numpy array builder
np.array([v.atti1 for v in lst])
np.array([v.atti2 for v in lst])
215 ms ± 3.69 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
This is slowing because you first building list with comprehension, and then re-allocate memory for np array and copy
# single list iteration with appending
l1 =
l2 =
for v in lst:
l1.append(v.atti1)
l2.append(v.atti2)
174 ms ± 384 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
Better, but you have a lot of function calls for .append
and eventually you're re-allocating and copying list.
# thing that you always start with, no pre-mature optimizations
l1 = [v.atti1 for v in lst]
l2 = [v.atti2 for v in lst]
99.3 ms ± 982 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
This is more readable, pythonic, does exactly what it says, and its faster. Internally, its faster because of low-level optimizations of comprehension.
Side note, CPython (that you're most probably using) starting from 3.5 (iirc) uses shared-key dictionaries to store object attributes, and starting from 3.6 it's merged with compact dict implementation. Both working great together — memory efficiency gives a huge boost to you raw performance.
Not sure if VM actually leverages shared dict when running comprehensions (probably not) but this must be left to VM optimizations in 99% of cases. High-level abstracted languages (like python) is really not about microoptimizations.
edited Dec 27 at 13:29
answered Dec 27 at 13:10
Slam
3,69712033
3,69712033
add a comment |
add a comment |
You can use self.__dict__
to return the dictionary of attributes and their values in Python.
import numpy as np
import datetime
import pandas as pd
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
def getAttr(self):
return self.__dict__
l1 = [Test(i,i+1).getAttr() for i in range(1000000)]
l1 = pd.DataFrame(l1)
l11 = list(l1['atti1'])
l12 = list(l1['atti2'])
How does this relate to question? OP is asking for lists with raw attributes, not dicts
– Slam
Dec 27 at 13:31
The ultimate goal, as I understand, is to get two different lists for two different attributes. This method creates a list of dictionaries of object attributes. A list comprehension or amap
or evenpandas.DataFrame
can be used to create 2 different lists later.
– Rishabh Mishra
Dec 27 at 13:51
Solution with pandas is slower by magnitude, literally.
– Slam
Dec 27 at 14:21
add a comment |
You can use self.__dict__
to return the dictionary of attributes and their values in Python.
import numpy as np
import datetime
import pandas as pd
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
def getAttr(self):
return self.__dict__
l1 = [Test(i,i+1).getAttr() for i in range(1000000)]
l1 = pd.DataFrame(l1)
l11 = list(l1['atti1'])
l12 = list(l1['atti2'])
How does this relate to question? OP is asking for lists with raw attributes, not dicts
– Slam
Dec 27 at 13:31
The ultimate goal, as I understand, is to get two different lists for two different attributes. This method creates a list of dictionaries of object attributes. A list comprehension or amap
or evenpandas.DataFrame
can be used to create 2 different lists later.
– Rishabh Mishra
Dec 27 at 13:51
Solution with pandas is slower by magnitude, literally.
– Slam
Dec 27 at 14:21
add a comment |
You can use self.__dict__
to return the dictionary of attributes and their values in Python.
import numpy as np
import datetime
import pandas as pd
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
def getAttr(self):
return self.__dict__
l1 = [Test(i,i+1).getAttr() for i in range(1000000)]
l1 = pd.DataFrame(l1)
l11 = list(l1['atti1'])
l12 = list(l1['atti2'])
You can use self.__dict__
to return the dictionary of attributes and their values in Python.
import numpy as np
import datetime
import pandas as pd
class Test():
def __init__(self,atti1,atti2):
self.atti1 = atti1
self.atti2 = atti2
def getAttr(self):
return self.__dict__
l1 = [Test(i,i+1).getAttr() for i in range(1000000)]
l1 = pd.DataFrame(l1)
l11 = list(l1['atti1'])
l12 = list(l1['atti2'])
edited Dec 27 at 13:57
answered Dec 27 at 13:18


Rishabh Mishra
365110
365110
How does this relate to question? OP is asking for lists with raw attributes, not dicts
– Slam
Dec 27 at 13:31
The ultimate goal, as I understand, is to get two different lists for two different attributes. This method creates a list of dictionaries of object attributes. A list comprehension or amap
or evenpandas.DataFrame
can be used to create 2 different lists later.
– Rishabh Mishra
Dec 27 at 13:51
Solution with pandas is slower by magnitude, literally.
– Slam
Dec 27 at 14:21
add a comment |
How does this relate to question? OP is asking for lists with raw attributes, not dicts
– Slam
Dec 27 at 13:31
The ultimate goal, as I understand, is to get two different lists for two different attributes. This method creates a list of dictionaries of object attributes. A list comprehension or amap
or evenpandas.DataFrame
can be used to create 2 different lists later.
– Rishabh Mishra
Dec 27 at 13:51
Solution with pandas is slower by magnitude, literally.
– Slam
Dec 27 at 14:21
How does this relate to question? OP is asking for lists with raw attributes, not dicts
– Slam
Dec 27 at 13:31
How does this relate to question? OP is asking for lists with raw attributes, not dicts
– Slam
Dec 27 at 13:31
The ultimate goal, as I understand, is to get two different lists for two different attributes. This method creates a list of dictionaries of object attributes. A list comprehension or a
map
or even pandas.DataFrame
can be used to create 2 different lists later.– Rishabh Mishra
Dec 27 at 13:51
The ultimate goal, as I understand, is to get two different lists for two different attributes. This method creates a list of dictionaries of object attributes. A list comprehension or a
map
or even pandas.DataFrame
can be used to create 2 different lists later.– Rishabh Mishra
Dec 27 at 13:51
Solution with pandas is slower by magnitude, literally.
– Slam
Dec 27 at 14:21
Solution with pandas is slower by magnitude, literally.
– Slam
Dec 27 at 14:21
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945460%2fthe-most-efficient-method-to-get-an-objects-attributes-when-the-object-is-in-a%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5sO1LapEC XjOmJCLvQPaiDdDjh5VtGUxr c,tu73ymvpvbnsq58FOfwX3VlCGPRwy1HIhCgZ19WeNfAe
1
I'm confused. What exactly are you trying to do? And if you're trying to evaluate how a bit of Python code performs, don't use
datetime.now()
, use thetimeit
module, which is designed for this purpose.– Daniel Pryden
Dec 27 at 12:57
1
1. What are you actually trying to achieve? 2. What is the significance of using
numpy
here? 3. Why do you think that0:00:00.234735
is slow for a list with 1 million objects? Anyway, 1 method for improving this code is to fetch both attributes in a single pass instead of iterating twice over the entire list– DeepSpace
Dec 27 at 12:58
@DanielPryden Many modules' function return a list of object.I want to know what is the best way to get object's attitudes.
– DachuanZhao
Dec 27 at 13:05
@DeepSpace That's not necessary.I will edit the question.Thanks.
– DachuanZhao
Dec 27 at 13:07
@DachuanZhao: Two points of English terminology that I want to clarify. 1. You say "attitudes" but I assume you actually mean "attributes". Is that correct? If so, please clarify it in the question. 2. You ask for the "most effective method". Do you really mean "effective" (as in, able to produce the desired effect) or do you intend something like "efficient" (as in, producing the effect with the smallest amount of work expended)? Please clarify that as well, as that will affect what the answer should be.
– Daniel Pryden
Dec 27 at 13:11