Java Pong game bounce ball from paddle
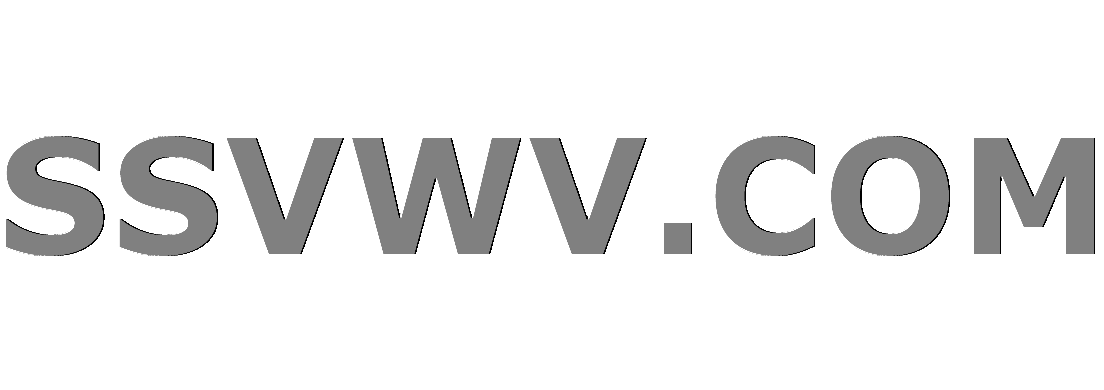
Multi tool use
I'm trying to make a Pong game in Java and I have a little problem with bouncing the ball from the paddle.
I have two paddles: left paddle1, right paddle2.
My idea is the following: ball_Y
should be between the height of the paddle and ball_X
touching point should be paddle width (PP1_width or PP2_width) +/- ball_radius
Paddle2 is working OK, but the ball passes through paddle1 and I cannot see my mistake.
Here is the video of how it looks like;
https://streamable.com/bzrud
Here is the paddle bouncing part:
if(ball_Y > P1_Y && ball_Y < P1_Hit_Y){
if(ball_X < P1_Hit_X){
ball_Velocity_X *= -1;
ball_X = P1_Hit_X;
}
}
if (ball_Y > P2_Y && ball_Y < P2_Hit_Y){
if(ball_X > P2_Hit_X){
ball_Velocity_X *= -1;
ball_X = P2_Hit_X;
}
}
Here is all the code:
import java.awt.*;
import java.awt.geom.*;
import javax.swing.*;
import java.awt.event.*;
public class PongGame extends GameEngine{
public static void main(String args) {
// Warning - don't edit this function.
// Create a new game.
createGame(new PongGame());
}
int game_width, game_height;
double PP1_width, PP1_height, PP2_width, PP2_height;
double P1_X, P1_Y, P2_X, P2_Y;
double PP_Velocity;
boolean PP1_UP, PP1_DOWN, PP2_UP, PP2_DOWN;
double ball_X, ball_Y, ball_Radius, ball_Velocity_X, ball_Velocity_Y;
double P1_Hit_X, P1_Hit_Y, P2_Hit_X, P2_Hit_Y;
AudioClip wall_bounce;
//TODO: Create paddles and a ball
//Two paddles for two players: They will be controlled with W-S and UP-DOWN Keys
public void paddle1(){
drawSolidRectangle(P1_X, P1_Y, PP1_width, PP1_height);
}
public void paddle2(){
drawSolidRectangle(P2_X, P2_Y, PP2_width, PP2_height);
}
public void ball(){
drawSolidCircle(ball_X, ball_Y, ball_Radius);
}
//TODO: Update Paddles
//DONE: Sources from PhysicsDemo lecture
/**
* These methods allow us the update the window with each framerate so we will be able to move the
* paddles
*/
public void paddle1_update(double dt){
// Paddle 1 Up with W
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
}
}
}
public void paddle2_update(double dt){
// Paddle 2 Up with Up Key
if(PP2_UP == true){
P2_Y -= PP_Velocity * dt;
// Check paddle inside wall
if((P2_Y < 0) ) {
P2_Y = 0;
}
}
// Paddle 2 Down with Down Key
if(PP2_DOWN == true){
P2_Y += PP_Velocity * dt;
// Check paddle inside wall
if(P2_Y > game_height-PP2_height){
P2_Y = game_height-PP2_height;
}
}
}
public void ball_update(double dt){
ball_X += ball_Velocity_X * dt;
ball_Y += ball_Velocity_Y * dt;
// Bouncing the ball from edge of the wals
if(ball_Y < ball_Radius) {
ball_Velocity_Y *= -1;
//Play wall bounce sound
playAudio(wall_bounce);
}else if (ball_Y > (game_height - ball_Radius)){
ball_Velocity_Y *= -1;
//Play wall bounce sound
playAudio(wall_bounce);
}
}
// Background
//TODO: Override init method in GameEngine
public void init(){
//Initialise Window size
game_width = 750;
game_height = 450;
//Position for Paddles
PP_Velocity = 250;
PP1_width = 10;
PP1_height = 100;
P1_X = 0;
P1_Y = 100;
PP2_width = 10;
PP2_height = 100;
P2_X = game_width - PP2_width;
P2_Y = 100;
//Initialise Keyboard variables
PP1_UP = false;
PP1_DOWN = false;
PP2_UP = false;
PP2_DOWN= false;
//Initialise Ball
ball_X = 250;
ball_Y = 250;
ball_Radius = 10;
ball_Velocity_X = 200;
ball_Velocity_Y = 200;
//Paddle ball bounce point
P1_Hit_X = PP1_width + ball_Radius;
P1_Hit_Y = P1_Y + PP1_height;
P2_Hit_X = P2_X - ball_Radius;
P2_Hit_Y = P2_Y + PP2_height;
/* NOTE: Sound Resources
* https://freesound.org/people/NoiseCollector/sounds/4391/
* https://freesound.org/people/NoiseCollector/sounds/4386/
*/
wall_bounce = loadAudio("Audio/wall_bounce.wav");
// paddle = loadAudio("Audio/paddle_bounce.wav");
//background = loadAudio("Audio/background.wav");
}
// Override abstract method update(double) in GameEngine
public void update(double dt){
paddle1_update(dt);
paddle2_update(dt);
ball_update(dt);
if(ball_Y > P1_Y && ball_Y < P1_Hit_Y){
if(ball_X < P1_Hit_X){
ball_Velocity_X *= -1;
ball_X = P1_Hit_X;
}
}
if (ball_Y > P2_Y && ball_Y < P2_Hit_Y){
if(ball_X > P2_Hit_X){
ball_Velocity_X *= -1;
ball_X = P2_Hit_X;
}
}
}
public void paintComponent() {
// Clear the background to black
setWindowSize(game_width, game_height);
changeBackgroundColor(black);
clearBackground(game_width, game_height);
changeColor(white);
drawLine(game_width/2, 0, game_width/2, game_height);
paddle1();
paddle2();
ball();
// Draw in black
changeColor(black);
}
//TODO: Key Listener for paddles ()
//DONE: KeyListener -> Space game example
// KeyPressed Event
public void keyPressed(KeyEvent event) {
// Left Arrow
if(event.getKeyCode() == KeyEvent.VK_W) {
PP1_UP = true;
}
// Right Arrow
if(event.getKeyCode() == KeyEvent.VK_S) {
PP1_DOWN = true;
}
// Space Button
if(event.getKeyCode() == KeyEvent.VK_UP) {
PP2_UP = true;
}
if(event.getKeyCode() == KeyEvent.VK_DOWN) {
PP2_DOWN = true;
}
}
// KeyReleased Event
public void keyReleased(KeyEvent event) {
// Left Arrow
if(event.getKeyCode() == KeyEvent.VK_W) {
PP1_UP = false;
}
// Right Arrow
if(event.getKeyCode() == KeyEvent.VK_S) {
PP1_DOWN = false;
}
// Space Button
if(event.getKeyCode() == KeyEvent.VK_UP) {
PP2_UP = false;
}
if(event.getKeyCode() == KeyEvent.VK_DOWN) {
PP2_DOWN = false;
}
}
}
java game-physics
add a comment |
I'm trying to make a Pong game in Java and I have a little problem with bouncing the ball from the paddle.
I have two paddles: left paddle1, right paddle2.
My idea is the following: ball_Y
should be between the height of the paddle and ball_X
touching point should be paddle width (PP1_width or PP2_width) +/- ball_radius
Paddle2 is working OK, but the ball passes through paddle1 and I cannot see my mistake.
Here is the video of how it looks like;
https://streamable.com/bzrud
Here is the paddle bouncing part:
if(ball_Y > P1_Y && ball_Y < P1_Hit_Y){
if(ball_X < P1_Hit_X){
ball_Velocity_X *= -1;
ball_X = P1_Hit_X;
}
}
if (ball_Y > P2_Y && ball_Y < P2_Hit_Y){
if(ball_X > P2_Hit_X){
ball_Velocity_X *= -1;
ball_X = P2_Hit_X;
}
}
Here is all the code:
import java.awt.*;
import java.awt.geom.*;
import javax.swing.*;
import java.awt.event.*;
public class PongGame extends GameEngine{
public static void main(String args) {
// Warning - don't edit this function.
// Create a new game.
createGame(new PongGame());
}
int game_width, game_height;
double PP1_width, PP1_height, PP2_width, PP2_height;
double P1_X, P1_Y, P2_X, P2_Y;
double PP_Velocity;
boolean PP1_UP, PP1_DOWN, PP2_UP, PP2_DOWN;
double ball_X, ball_Y, ball_Radius, ball_Velocity_X, ball_Velocity_Y;
double P1_Hit_X, P1_Hit_Y, P2_Hit_X, P2_Hit_Y;
AudioClip wall_bounce;
//TODO: Create paddles and a ball
//Two paddles for two players: They will be controlled with W-S and UP-DOWN Keys
public void paddle1(){
drawSolidRectangle(P1_X, P1_Y, PP1_width, PP1_height);
}
public void paddle2(){
drawSolidRectangle(P2_X, P2_Y, PP2_width, PP2_height);
}
public void ball(){
drawSolidCircle(ball_X, ball_Y, ball_Radius);
}
//TODO: Update Paddles
//DONE: Sources from PhysicsDemo lecture
/**
* These methods allow us the update the window with each framerate so we will be able to move the
* paddles
*/
public void paddle1_update(double dt){
// Paddle 1 Up with W
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
}
}
}
public void paddle2_update(double dt){
// Paddle 2 Up with Up Key
if(PP2_UP == true){
P2_Y -= PP_Velocity * dt;
// Check paddle inside wall
if((P2_Y < 0) ) {
P2_Y = 0;
}
}
// Paddle 2 Down with Down Key
if(PP2_DOWN == true){
P2_Y += PP_Velocity * dt;
// Check paddle inside wall
if(P2_Y > game_height-PP2_height){
P2_Y = game_height-PP2_height;
}
}
}
public void ball_update(double dt){
ball_X += ball_Velocity_X * dt;
ball_Y += ball_Velocity_Y * dt;
// Bouncing the ball from edge of the wals
if(ball_Y < ball_Radius) {
ball_Velocity_Y *= -1;
//Play wall bounce sound
playAudio(wall_bounce);
}else if (ball_Y > (game_height - ball_Radius)){
ball_Velocity_Y *= -1;
//Play wall bounce sound
playAudio(wall_bounce);
}
}
// Background
//TODO: Override init method in GameEngine
public void init(){
//Initialise Window size
game_width = 750;
game_height = 450;
//Position for Paddles
PP_Velocity = 250;
PP1_width = 10;
PP1_height = 100;
P1_X = 0;
P1_Y = 100;
PP2_width = 10;
PP2_height = 100;
P2_X = game_width - PP2_width;
P2_Y = 100;
//Initialise Keyboard variables
PP1_UP = false;
PP1_DOWN = false;
PP2_UP = false;
PP2_DOWN= false;
//Initialise Ball
ball_X = 250;
ball_Y = 250;
ball_Radius = 10;
ball_Velocity_X = 200;
ball_Velocity_Y = 200;
//Paddle ball bounce point
P1_Hit_X = PP1_width + ball_Radius;
P1_Hit_Y = P1_Y + PP1_height;
P2_Hit_X = P2_X - ball_Radius;
P2_Hit_Y = P2_Y + PP2_height;
/* NOTE: Sound Resources
* https://freesound.org/people/NoiseCollector/sounds/4391/
* https://freesound.org/people/NoiseCollector/sounds/4386/
*/
wall_bounce = loadAudio("Audio/wall_bounce.wav");
// paddle = loadAudio("Audio/paddle_bounce.wav");
//background = loadAudio("Audio/background.wav");
}
// Override abstract method update(double) in GameEngine
public void update(double dt){
paddle1_update(dt);
paddle2_update(dt);
ball_update(dt);
if(ball_Y > P1_Y && ball_Y < P1_Hit_Y){
if(ball_X < P1_Hit_X){
ball_Velocity_X *= -1;
ball_X = P1_Hit_X;
}
}
if (ball_Y > P2_Y && ball_Y < P2_Hit_Y){
if(ball_X > P2_Hit_X){
ball_Velocity_X *= -1;
ball_X = P2_Hit_X;
}
}
}
public void paintComponent() {
// Clear the background to black
setWindowSize(game_width, game_height);
changeBackgroundColor(black);
clearBackground(game_width, game_height);
changeColor(white);
drawLine(game_width/2, 0, game_width/2, game_height);
paddle1();
paddle2();
ball();
// Draw in black
changeColor(black);
}
//TODO: Key Listener for paddles ()
//DONE: KeyListener -> Space game example
// KeyPressed Event
public void keyPressed(KeyEvent event) {
// Left Arrow
if(event.getKeyCode() == KeyEvent.VK_W) {
PP1_UP = true;
}
// Right Arrow
if(event.getKeyCode() == KeyEvent.VK_S) {
PP1_DOWN = true;
}
// Space Button
if(event.getKeyCode() == KeyEvent.VK_UP) {
PP2_UP = true;
}
if(event.getKeyCode() == KeyEvent.VK_DOWN) {
PP2_DOWN = true;
}
}
// KeyReleased Event
public void keyReleased(KeyEvent event) {
// Left Arrow
if(event.getKeyCode() == KeyEvent.VK_W) {
PP1_UP = false;
}
// Right Arrow
if(event.getKeyCode() == KeyEvent.VK_S) {
PP1_DOWN = false;
}
// Space Button
if(event.getKeyCode() == KeyEvent.VK_UP) {
PP2_UP = false;
}
if(event.getKeyCode() == KeyEvent.VK_DOWN) {
PP2_DOWN = false;
}
}
}
java game-physics
You have not included them class GameEngine.
– Just another Java programmer
Dec 27 at 14:12
1
If you have a new problem, post a new question. It's not fair to invalidate existing answers.
– Makoto
2 days ago
Right I have not thought from that perspective. I thought might I can make tidy. Thanks for comment
– Axis
2 days ago
add a comment |
I'm trying to make a Pong game in Java and I have a little problem with bouncing the ball from the paddle.
I have two paddles: left paddle1, right paddle2.
My idea is the following: ball_Y
should be between the height of the paddle and ball_X
touching point should be paddle width (PP1_width or PP2_width) +/- ball_radius
Paddle2 is working OK, but the ball passes through paddle1 and I cannot see my mistake.
Here is the video of how it looks like;
https://streamable.com/bzrud
Here is the paddle bouncing part:
if(ball_Y > P1_Y && ball_Y < P1_Hit_Y){
if(ball_X < P1_Hit_X){
ball_Velocity_X *= -1;
ball_X = P1_Hit_X;
}
}
if (ball_Y > P2_Y && ball_Y < P2_Hit_Y){
if(ball_X > P2_Hit_X){
ball_Velocity_X *= -1;
ball_X = P2_Hit_X;
}
}
Here is all the code:
import java.awt.*;
import java.awt.geom.*;
import javax.swing.*;
import java.awt.event.*;
public class PongGame extends GameEngine{
public static void main(String args) {
// Warning - don't edit this function.
// Create a new game.
createGame(new PongGame());
}
int game_width, game_height;
double PP1_width, PP1_height, PP2_width, PP2_height;
double P1_X, P1_Y, P2_X, P2_Y;
double PP_Velocity;
boolean PP1_UP, PP1_DOWN, PP2_UP, PP2_DOWN;
double ball_X, ball_Y, ball_Radius, ball_Velocity_X, ball_Velocity_Y;
double P1_Hit_X, P1_Hit_Y, P2_Hit_X, P2_Hit_Y;
AudioClip wall_bounce;
//TODO: Create paddles and a ball
//Two paddles for two players: They will be controlled with W-S and UP-DOWN Keys
public void paddle1(){
drawSolidRectangle(P1_X, P1_Y, PP1_width, PP1_height);
}
public void paddle2(){
drawSolidRectangle(P2_X, P2_Y, PP2_width, PP2_height);
}
public void ball(){
drawSolidCircle(ball_X, ball_Y, ball_Radius);
}
//TODO: Update Paddles
//DONE: Sources from PhysicsDemo lecture
/**
* These methods allow us the update the window with each framerate so we will be able to move the
* paddles
*/
public void paddle1_update(double dt){
// Paddle 1 Up with W
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
}
}
}
public void paddle2_update(double dt){
// Paddle 2 Up with Up Key
if(PP2_UP == true){
P2_Y -= PP_Velocity * dt;
// Check paddle inside wall
if((P2_Y < 0) ) {
P2_Y = 0;
}
}
// Paddle 2 Down with Down Key
if(PP2_DOWN == true){
P2_Y += PP_Velocity * dt;
// Check paddle inside wall
if(P2_Y > game_height-PP2_height){
P2_Y = game_height-PP2_height;
}
}
}
public void ball_update(double dt){
ball_X += ball_Velocity_X * dt;
ball_Y += ball_Velocity_Y * dt;
// Bouncing the ball from edge of the wals
if(ball_Y < ball_Radius) {
ball_Velocity_Y *= -1;
//Play wall bounce sound
playAudio(wall_bounce);
}else if (ball_Y > (game_height - ball_Radius)){
ball_Velocity_Y *= -1;
//Play wall bounce sound
playAudio(wall_bounce);
}
}
// Background
//TODO: Override init method in GameEngine
public void init(){
//Initialise Window size
game_width = 750;
game_height = 450;
//Position for Paddles
PP_Velocity = 250;
PP1_width = 10;
PP1_height = 100;
P1_X = 0;
P1_Y = 100;
PP2_width = 10;
PP2_height = 100;
P2_X = game_width - PP2_width;
P2_Y = 100;
//Initialise Keyboard variables
PP1_UP = false;
PP1_DOWN = false;
PP2_UP = false;
PP2_DOWN= false;
//Initialise Ball
ball_X = 250;
ball_Y = 250;
ball_Radius = 10;
ball_Velocity_X = 200;
ball_Velocity_Y = 200;
//Paddle ball bounce point
P1_Hit_X = PP1_width + ball_Radius;
P1_Hit_Y = P1_Y + PP1_height;
P2_Hit_X = P2_X - ball_Radius;
P2_Hit_Y = P2_Y + PP2_height;
/* NOTE: Sound Resources
* https://freesound.org/people/NoiseCollector/sounds/4391/
* https://freesound.org/people/NoiseCollector/sounds/4386/
*/
wall_bounce = loadAudio("Audio/wall_bounce.wav");
// paddle = loadAudio("Audio/paddle_bounce.wav");
//background = loadAudio("Audio/background.wav");
}
// Override abstract method update(double) in GameEngine
public void update(double dt){
paddle1_update(dt);
paddle2_update(dt);
ball_update(dt);
if(ball_Y > P1_Y && ball_Y < P1_Hit_Y){
if(ball_X < P1_Hit_X){
ball_Velocity_X *= -1;
ball_X = P1_Hit_X;
}
}
if (ball_Y > P2_Y && ball_Y < P2_Hit_Y){
if(ball_X > P2_Hit_X){
ball_Velocity_X *= -1;
ball_X = P2_Hit_X;
}
}
}
public void paintComponent() {
// Clear the background to black
setWindowSize(game_width, game_height);
changeBackgroundColor(black);
clearBackground(game_width, game_height);
changeColor(white);
drawLine(game_width/2, 0, game_width/2, game_height);
paddle1();
paddle2();
ball();
// Draw in black
changeColor(black);
}
//TODO: Key Listener for paddles ()
//DONE: KeyListener -> Space game example
// KeyPressed Event
public void keyPressed(KeyEvent event) {
// Left Arrow
if(event.getKeyCode() == KeyEvent.VK_W) {
PP1_UP = true;
}
// Right Arrow
if(event.getKeyCode() == KeyEvent.VK_S) {
PP1_DOWN = true;
}
// Space Button
if(event.getKeyCode() == KeyEvent.VK_UP) {
PP2_UP = true;
}
if(event.getKeyCode() == KeyEvent.VK_DOWN) {
PP2_DOWN = true;
}
}
// KeyReleased Event
public void keyReleased(KeyEvent event) {
// Left Arrow
if(event.getKeyCode() == KeyEvent.VK_W) {
PP1_UP = false;
}
// Right Arrow
if(event.getKeyCode() == KeyEvent.VK_S) {
PP1_DOWN = false;
}
// Space Button
if(event.getKeyCode() == KeyEvent.VK_UP) {
PP2_UP = false;
}
if(event.getKeyCode() == KeyEvent.VK_DOWN) {
PP2_DOWN = false;
}
}
}
java game-physics
I'm trying to make a Pong game in Java and I have a little problem with bouncing the ball from the paddle.
I have two paddles: left paddle1, right paddle2.
My idea is the following: ball_Y
should be between the height of the paddle and ball_X
touching point should be paddle width (PP1_width or PP2_width) +/- ball_radius
Paddle2 is working OK, but the ball passes through paddle1 and I cannot see my mistake.
Here is the video of how it looks like;
https://streamable.com/bzrud
Here is the paddle bouncing part:
if(ball_Y > P1_Y && ball_Y < P1_Hit_Y){
if(ball_X < P1_Hit_X){
ball_Velocity_X *= -1;
ball_X = P1_Hit_X;
}
}
if (ball_Y > P2_Y && ball_Y < P2_Hit_Y){
if(ball_X > P2_Hit_X){
ball_Velocity_X *= -1;
ball_X = P2_Hit_X;
}
}
Here is all the code:
import java.awt.*;
import java.awt.geom.*;
import javax.swing.*;
import java.awt.event.*;
public class PongGame extends GameEngine{
public static void main(String args) {
// Warning - don't edit this function.
// Create a new game.
createGame(new PongGame());
}
int game_width, game_height;
double PP1_width, PP1_height, PP2_width, PP2_height;
double P1_X, P1_Y, P2_X, P2_Y;
double PP_Velocity;
boolean PP1_UP, PP1_DOWN, PP2_UP, PP2_DOWN;
double ball_X, ball_Y, ball_Radius, ball_Velocity_X, ball_Velocity_Y;
double P1_Hit_X, P1_Hit_Y, P2_Hit_X, P2_Hit_Y;
AudioClip wall_bounce;
//TODO: Create paddles and a ball
//Two paddles for two players: They will be controlled with W-S and UP-DOWN Keys
public void paddle1(){
drawSolidRectangle(P1_X, P1_Y, PP1_width, PP1_height);
}
public void paddle2(){
drawSolidRectangle(P2_X, P2_Y, PP2_width, PP2_height);
}
public void ball(){
drawSolidCircle(ball_X, ball_Y, ball_Radius);
}
//TODO: Update Paddles
//DONE: Sources from PhysicsDemo lecture
/**
* These methods allow us the update the window with each framerate so we will be able to move the
* paddles
*/
public void paddle1_update(double dt){
// Paddle 1 Up with W
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
}
}
}
public void paddle2_update(double dt){
// Paddle 2 Up with Up Key
if(PP2_UP == true){
P2_Y -= PP_Velocity * dt;
// Check paddle inside wall
if((P2_Y < 0) ) {
P2_Y = 0;
}
}
// Paddle 2 Down with Down Key
if(PP2_DOWN == true){
P2_Y += PP_Velocity * dt;
// Check paddle inside wall
if(P2_Y > game_height-PP2_height){
P2_Y = game_height-PP2_height;
}
}
}
public void ball_update(double dt){
ball_X += ball_Velocity_X * dt;
ball_Y += ball_Velocity_Y * dt;
// Bouncing the ball from edge of the wals
if(ball_Y < ball_Radius) {
ball_Velocity_Y *= -1;
//Play wall bounce sound
playAudio(wall_bounce);
}else if (ball_Y > (game_height - ball_Radius)){
ball_Velocity_Y *= -1;
//Play wall bounce sound
playAudio(wall_bounce);
}
}
// Background
//TODO: Override init method in GameEngine
public void init(){
//Initialise Window size
game_width = 750;
game_height = 450;
//Position for Paddles
PP_Velocity = 250;
PP1_width = 10;
PP1_height = 100;
P1_X = 0;
P1_Y = 100;
PP2_width = 10;
PP2_height = 100;
P2_X = game_width - PP2_width;
P2_Y = 100;
//Initialise Keyboard variables
PP1_UP = false;
PP1_DOWN = false;
PP2_UP = false;
PP2_DOWN= false;
//Initialise Ball
ball_X = 250;
ball_Y = 250;
ball_Radius = 10;
ball_Velocity_X = 200;
ball_Velocity_Y = 200;
//Paddle ball bounce point
P1_Hit_X = PP1_width + ball_Radius;
P1_Hit_Y = P1_Y + PP1_height;
P2_Hit_X = P2_X - ball_Radius;
P2_Hit_Y = P2_Y + PP2_height;
/* NOTE: Sound Resources
* https://freesound.org/people/NoiseCollector/sounds/4391/
* https://freesound.org/people/NoiseCollector/sounds/4386/
*/
wall_bounce = loadAudio("Audio/wall_bounce.wav");
// paddle = loadAudio("Audio/paddle_bounce.wav");
//background = loadAudio("Audio/background.wav");
}
// Override abstract method update(double) in GameEngine
public void update(double dt){
paddle1_update(dt);
paddle2_update(dt);
ball_update(dt);
if(ball_Y > P1_Y && ball_Y < P1_Hit_Y){
if(ball_X < P1_Hit_X){
ball_Velocity_X *= -1;
ball_X = P1_Hit_X;
}
}
if (ball_Y > P2_Y && ball_Y < P2_Hit_Y){
if(ball_X > P2_Hit_X){
ball_Velocity_X *= -1;
ball_X = P2_Hit_X;
}
}
}
public void paintComponent() {
// Clear the background to black
setWindowSize(game_width, game_height);
changeBackgroundColor(black);
clearBackground(game_width, game_height);
changeColor(white);
drawLine(game_width/2, 0, game_width/2, game_height);
paddle1();
paddle2();
ball();
// Draw in black
changeColor(black);
}
//TODO: Key Listener for paddles ()
//DONE: KeyListener -> Space game example
// KeyPressed Event
public void keyPressed(KeyEvent event) {
// Left Arrow
if(event.getKeyCode() == KeyEvent.VK_W) {
PP1_UP = true;
}
// Right Arrow
if(event.getKeyCode() == KeyEvent.VK_S) {
PP1_DOWN = true;
}
// Space Button
if(event.getKeyCode() == KeyEvent.VK_UP) {
PP2_UP = true;
}
if(event.getKeyCode() == KeyEvent.VK_DOWN) {
PP2_DOWN = true;
}
}
// KeyReleased Event
public void keyReleased(KeyEvent event) {
// Left Arrow
if(event.getKeyCode() == KeyEvent.VK_W) {
PP1_UP = false;
}
// Right Arrow
if(event.getKeyCode() == KeyEvent.VK_S) {
PP1_DOWN = false;
}
// Space Button
if(event.getKeyCode() == KeyEvent.VK_UP) {
PP2_UP = false;
}
if(event.getKeyCode() == KeyEvent.VK_DOWN) {
PP2_DOWN = false;
}
}
}
java game-physics
java game-physics
edited 2 days ago
Makoto
80.6k15125173
80.6k15125173
asked Dec 27 at 13:31


Axis
1,1081922
1,1081922
You have not included them class GameEngine.
– Just another Java programmer
Dec 27 at 14:12
1
If you have a new problem, post a new question. It's not fair to invalidate existing answers.
– Makoto
2 days ago
Right I have not thought from that perspective. I thought might I can make tidy. Thanks for comment
– Axis
2 days ago
add a comment |
You have not included them class GameEngine.
– Just another Java programmer
Dec 27 at 14:12
1
If you have a new problem, post a new question. It's not fair to invalidate existing answers.
– Makoto
2 days ago
Right I have not thought from that perspective. I thought might I can make tidy. Thanks for comment
– Axis
2 days ago
You have not included them class GameEngine.
– Just another Java programmer
Dec 27 at 14:12
You have not included them class GameEngine.
– Just another Java programmer
Dec 27 at 14:12
1
1
If you have a new problem, post a new question. It's not fair to invalidate existing answers.
– Makoto
2 days ago
If you have a new problem, post a new question. It's not fair to invalidate existing answers.
– Makoto
2 days ago
Right I have not thought from that perspective. I thought might I can make tidy. Thanks for comment
– Axis
2 days ago
Right I have not thought from that perspective. I thought might I can make tidy. Thanks for comment
– Axis
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
I think you coded this very neatly and it took me quite a while to find out what was the problem. However, I think it is caused by not updating P1_Hit_Y and P2_Hit_Y in the paddle update methods. So:
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
P1_Hit_Y -= PP_Velocity *dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
P1_Hit_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
P1_Hit_Y += PP_Velocity *dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
P1_Hit_Y = game_height;
}
}
And the same for paddle2.
Hope this helps!
New contributor
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
That's right, I saw your point. Thank you @Samson Daniel. I should play on it because still I have missing point when the paddle on top of the window (paddle == 0) paddle missing the ball (Also a few times random missing in the middle. Weird). I could not find why? Might they are different problem but If you have an idea I would like to hear
– Axis
Dec 27 at 15:01
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945933%2fjava-pong-game-bounce-ball-from-paddle%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you coded this very neatly and it took me quite a while to find out what was the problem. However, I think it is caused by not updating P1_Hit_Y and P2_Hit_Y in the paddle update methods. So:
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
P1_Hit_Y -= PP_Velocity *dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
P1_Hit_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
P1_Hit_Y += PP_Velocity *dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
P1_Hit_Y = game_height;
}
}
And the same for paddle2.
Hope this helps!
New contributor
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
That's right, I saw your point. Thank you @Samson Daniel. I should play on it because still I have missing point when the paddle on top of the window (paddle == 0) paddle missing the ball (Also a few times random missing in the middle. Weird). I could not find why? Might they are different problem but If you have an idea I would like to hear
– Axis
Dec 27 at 15:01
add a comment |
I think you coded this very neatly and it took me quite a while to find out what was the problem. However, I think it is caused by not updating P1_Hit_Y and P2_Hit_Y in the paddle update methods. So:
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
P1_Hit_Y -= PP_Velocity *dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
P1_Hit_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
P1_Hit_Y += PP_Velocity *dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
P1_Hit_Y = game_height;
}
}
And the same for paddle2.
Hope this helps!
New contributor
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
That's right, I saw your point. Thank you @Samson Daniel. I should play on it because still I have missing point when the paddle on top of the window (paddle == 0) paddle missing the ball (Also a few times random missing in the middle. Weird). I could not find why? Might they are different problem but If you have an idea I would like to hear
– Axis
Dec 27 at 15:01
add a comment |
I think you coded this very neatly and it took me quite a while to find out what was the problem. However, I think it is caused by not updating P1_Hit_Y and P2_Hit_Y in the paddle update methods. So:
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
P1_Hit_Y -= PP_Velocity *dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
P1_Hit_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
P1_Hit_Y += PP_Velocity *dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
P1_Hit_Y = game_height;
}
}
And the same for paddle2.
Hope this helps!
New contributor
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I think you coded this very neatly and it took me quite a while to find out what was the problem. However, I think it is caused by not updating P1_Hit_Y and P2_Hit_Y in the paddle update methods. So:
if(PP1_UP == true){
P1_Y -= PP_Velocity * dt;
P1_Hit_Y -= PP_Velocity *dt;
// Check paddle inside wall
if((P1_Y < 0) ) {
P1_Y = 0;
P1_Hit_Y = 0;
}
}
// Paddle 1 Down with S
if(PP1_DOWN == true){
P1_Y += PP_Velocity * dt;
P1_Hit_Y += PP_Velocity *dt;
// Check paddle inside wall
if(P1_Y > game_height-PP1_height){
P1_Y = game_height-PP1_height;
P1_Hit_Y = game_height;
}
}
And the same for paddle2.
Hope this helps!
New contributor
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Dec 27 at 14:13
Samson Daniel
313
313
New contributor
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Samson Daniel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
That's right, I saw your point. Thank you @Samson Daniel. I should play on it because still I have missing point when the paddle on top of the window (paddle == 0) paddle missing the ball (Also a few times random missing in the middle. Weird). I could not find why? Might they are different problem but If you have an idea I would like to hear
– Axis
Dec 27 at 15:01
add a comment |
That's right, I saw your point. Thank you @Samson Daniel. I should play on it because still I have missing point when the paddle on top of the window (paddle == 0) paddle missing the ball (Also a few times random missing in the middle. Weird). I could not find why? Might they are different problem but If you have an idea I would like to hear
– Axis
Dec 27 at 15:01
That's right, I saw your point. Thank you @Samson Daniel. I should play on it because still I have missing point when the paddle on top of the window (paddle == 0) paddle missing the ball (Also a few times random missing in the middle. Weird). I could not find why? Might they are different problem but If you have an idea I would like to hear
– Axis
Dec 27 at 15:01
That's right, I saw your point. Thank you @Samson Daniel. I should play on it because still I have missing point when the paddle on top of the window (paddle == 0) paddle missing the ball (Also a few times random missing in the middle. Weird). I could not find why? Might they are different problem but If you have an idea I would like to hear
– Axis
Dec 27 at 15:01
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945933%2fjava-pong-game-bounce-ball-from-paddle%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
j,YoTsveo,yV os wqQvPGZ8C,bGeKDbsVmwTHk3oZqgejEDeN FaKXrx ZvJZ9q1,kUr
You have not included them class GameEngine.
– Just another Java programmer
Dec 27 at 14:12
1
If you have a new problem, post a new question. It's not fair to invalidate existing answers.
– Makoto
2 days ago
Right I have not thought from that perspective. I thought might I can make tidy. Thanks for comment
– Axis
2 days ago