Meta Programming - dynamic method
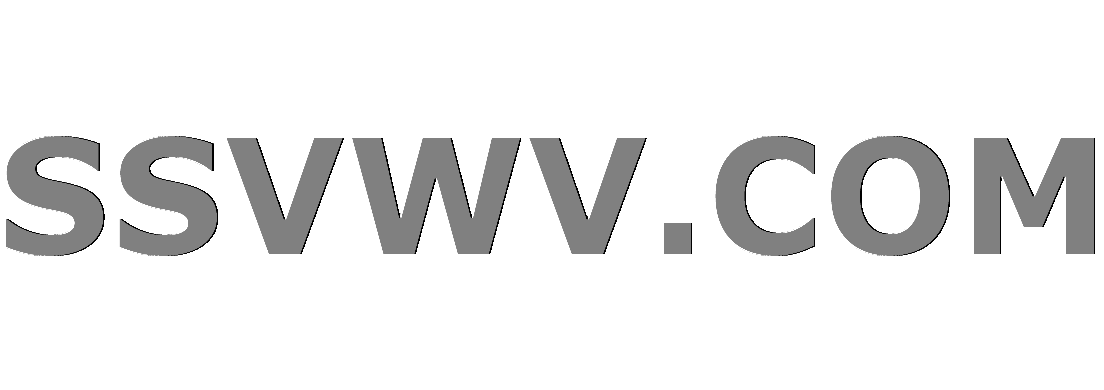
Multi tool use
I have 2 versions of Computer class here. I'm seeing that Computer2 is much better than Computer1. Both Computer classes yield the same result. How the Computer2 class varies from Computer1 class?
class DataSource
def cpu_info(computer_id)
"#{computer_id}'s CPU"
end
def cpu_price(computer_id)
12
end
def mouse_info(computer_id)
"#{computer_id}'s mouse"
end
def mouse_price(computer_id)
27
end
def monitor_info(computer_id)
"#{computer_id}'s monitor"
end
def monitor_price(computer_id)
33
end
end
# Step 1
class Computer1
def initialize(computer_id, data_source)
@id = computer_id
@ds = data_source
end
def cpu
get_info :cpu
end
def mouse
get_info :mouse
end
def monitor
get_info :monitor
end
def get_info(component)
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
end
end
computer = Computer1.new(1, DataSource.new)
computer.cpu #The work station 1's CPU is about 12$
# Step 2 - Further simplified
class Computer2
def initialize(computer_id, data_source)
@id = computer_id
@ds = data_source
end
def self.get_info(component)
define_method(component) do
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
end
end
get_info :mouse
get_info :cpu
get_info :monitor
end
computer = Computer2.new(1, DataSource.new)
computer.cpu #The work station 1's CPU is about 12$
My question is how the following is possible as there is no instance cpu method in Computer2 class
computer = Computer2.new(1, DataSource.new)
computer.cpu
ruby-on-rails ruby
add a comment |
I have 2 versions of Computer class here. I'm seeing that Computer2 is much better than Computer1. Both Computer classes yield the same result. How the Computer2 class varies from Computer1 class?
class DataSource
def cpu_info(computer_id)
"#{computer_id}'s CPU"
end
def cpu_price(computer_id)
12
end
def mouse_info(computer_id)
"#{computer_id}'s mouse"
end
def mouse_price(computer_id)
27
end
def monitor_info(computer_id)
"#{computer_id}'s monitor"
end
def monitor_price(computer_id)
33
end
end
# Step 1
class Computer1
def initialize(computer_id, data_source)
@id = computer_id
@ds = data_source
end
def cpu
get_info :cpu
end
def mouse
get_info :mouse
end
def monitor
get_info :monitor
end
def get_info(component)
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
end
end
computer = Computer1.new(1, DataSource.new)
computer.cpu #The work station 1's CPU is about 12$
# Step 2 - Further simplified
class Computer2
def initialize(computer_id, data_source)
@id = computer_id
@ds = data_source
end
def self.get_info(component)
define_method(component) do
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
end
end
get_info :mouse
get_info :cpu
get_info :monitor
end
computer = Computer2.new(1, DataSource.new)
computer.cpu #The work station 1's CPU is about 12$
My question is how the following is possible as there is no instance cpu method in Computer2 class
computer = Computer2.new(1, DataSource.new)
computer.cpu
ruby-on-rails ruby
add a comment |
I have 2 versions of Computer class here. I'm seeing that Computer2 is much better than Computer1. Both Computer classes yield the same result. How the Computer2 class varies from Computer1 class?
class DataSource
def cpu_info(computer_id)
"#{computer_id}'s CPU"
end
def cpu_price(computer_id)
12
end
def mouse_info(computer_id)
"#{computer_id}'s mouse"
end
def mouse_price(computer_id)
27
end
def monitor_info(computer_id)
"#{computer_id}'s monitor"
end
def monitor_price(computer_id)
33
end
end
# Step 1
class Computer1
def initialize(computer_id, data_source)
@id = computer_id
@ds = data_source
end
def cpu
get_info :cpu
end
def mouse
get_info :mouse
end
def monitor
get_info :monitor
end
def get_info(component)
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
end
end
computer = Computer1.new(1, DataSource.new)
computer.cpu #The work station 1's CPU is about 12$
# Step 2 - Further simplified
class Computer2
def initialize(computer_id, data_source)
@id = computer_id
@ds = data_source
end
def self.get_info(component)
define_method(component) do
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
end
end
get_info :mouse
get_info :cpu
get_info :monitor
end
computer = Computer2.new(1, DataSource.new)
computer.cpu #The work station 1's CPU is about 12$
My question is how the following is possible as there is no instance cpu method in Computer2 class
computer = Computer2.new(1, DataSource.new)
computer.cpu
ruby-on-rails ruby
I have 2 versions of Computer class here. I'm seeing that Computer2 is much better than Computer1. Both Computer classes yield the same result. How the Computer2 class varies from Computer1 class?
class DataSource
def cpu_info(computer_id)
"#{computer_id}'s CPU"
end
def cpu_price(computer_id)
12
end
def mouse_info(computer_id)
"#{computer_id}'s mouse"
end
def mouse_price(computer_id)
27
end
def monitor_info(computer_id)
"#{computer_id}'s monitor"
end
def monitor_price(computer_id)
33
end
end
# Step 1
class Computer1
def initialize(computer_id, data_source)
@id = computer_id
@ds = data_source
end
def cpu
get_info :cpu
end
def mouse
get_info :mouse
end
def monitor
get_info :monitor
end
def get_info(component)
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
end
end
computer = Computer1.new(1, DataSource.new)
computer.cpu #The work station 1's CPU is about 12$
# Step 2 - Further simplified
class Computer2
def initialize(computer_id, data_source)
@id = computer_id
@ds = data_source
end
def self.get_info(component)
define_method(component) do
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
end
end
get_info :mouse
get_info :cpu
get_info :monitor
end
computer = Computer2.new(1, DataSource.new)
computer.cpu #The work station 1's CPU is about 12$
My question is how the following is possible as there is no instance cpu method in Computer2 class
computer = Computer2.new(1, DataSource.new)
computer.cpu
ruby-on-rails ruby
ruby-on-rails ruby
asked Dec 27 at 13:16
Sam
1,78182759
1,78182759
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
When your class Computer2
is loaded, self calls get_info
class method with argument :cpu
which is later used to define instance method cpu
for your Computer2 instance.
Read define_method
add a comment |
In the Computer2 class, you call the method :get_info:
get_info :mouse
get_info :cpu
get_info :monitor
This methods, call the ruby function :define_method (https://apidock.com/ruby/Module/define_method), this method creates a named function for you instance in this case, and after call get_info(:cpu)
you have the .cpu
method to call, with the giving block:
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945739%2fmeta-programming-dynamic-method%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
When your class Computer2
is loaded, self calls get_info
class method with argument :cpu
which is later used to define instance method cpu
for your Computer2 instance.
Read define_method
add a comment |
When your class Computer2
is loaded, self calls get_info
class method with argument :cpu
which is later used to define instance method cpu
for your Computer2 instance.
Read define_method
add a comment |
When your class Computer2
is loaded, self calls get_info
class method with argument :cpu
which is later used to define instance method cpu
for your Computer2 instance.
Read define_method
When your class Computer2
is loaded, self calls get_info
class method with argument :cpu
which is later used to define instance method cpu
for your Computer2 instance.
Read define_method
answered Dec 27 at 13:26


ray
949113
949113
add a comment |
add a comment |
In the Computer2 class, you call the method :get_info:
get_info :mouse
get_info :cpu
get_info :monitor
This methods, call the ruby function :define_method (https://apidock.com/ruby/Module/define_method), this method creates a named function for you instance in this case, and after call get_info(:cpu)
you have the .cpu
method to call, with the giving block:
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
add a comment |
In the Computer2 class, you call the method :get_info:
get_info :mouse
get_info :cpu
get_info :monitor
This methods, call the ruby function :define_method (https://apidock.com/ruby/Module/define_method), this method creates a named function for you instance in this case, and after call get_info(:cpu)
you have the .cpu
method to call, with the giving block:
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
add a comment |
In the Computer2 class, you call the method :get_info:
get_info :mouse
get_info :cpu
get_info :monitor
This methods, call the ruby function :define_method (https://apidock.com/ruby/Module/define_method), this method creates a named function for you instance in this case, and after call get_info(:cpu)
you have the .cpu
method to call, with the giving block:
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
In the Computer2 class, you call the method :get_info:
get_info :mouse
get_info :cpu
get_info :monitor
This methods, call the ruby function :define_method (https://apidock.com/ruby/Module/define_method), this method creates a named function for you instance in this case, and after call get_info(:cpu)
you have the .cpu
method to call, with the giving block:
component_info = @ds.send("#{component}_info", @id)
component_price = @ds.send("#{component}_price", @id)
data = "The work station #{component_info} is about #{component_price}$"
return data;
answered Dec 27 at 13:26


Dimitrius Lachi
32510
32510
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945739%2fmeta-programming-dynamic-method%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
P j,odyik OgCiUfkvdNmQElaFb p4t9 Hza,ZzAdhTL Qw IX,UNg,fj3VnI0Ybc40kXRVIPSqdtJuTI8E9X gfK98 kW3BFfcjbSVpHU55Ph