parsing signed and encrypted email
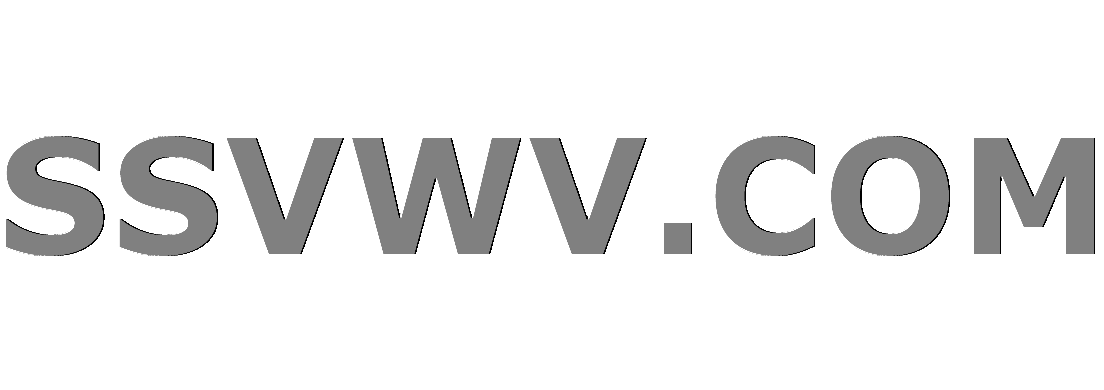
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am writing an application that must be able to read signed and encrypted emails and parse through their contents. I am able to get everything working fine for emails that are only encrypted, but do not know what to do when I get an email that is also signed. Once I decrypt this email instead of having an anticipated 4 parts in a Multipart object, I have only 1 part in a MimePart object with the file name smime.p7m. I do not know how to break this file up or verify the signature. I have found the documentation on verifying a signature (http://www.mimekit.net/docs/html/Working-With-SMime.htm#Verify), but I don't see how this does anything. Obviously there is something that I am just not understanding at this point.
Below is a sample of the code that I am using. Note that this will be refactored after I get everything figured out, but this code is thus far working fine for all emails that I have tested so far that are not signed (may or may not be encrypted).
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
//If the SecureMimeType has not been set as it should, set it to EnvelopedData
if (pkcs7.SecureMimeType == SecureMimeType.Unknown)
{
var content = new MemoryStream();
pkcs7.Content.DecodeTo(content);
content.Position = 0;
pkcs7 = new ApplicationPkcs7Mime(SecureMimeType.EnvelopedData, content);
}
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
////do stuff with decrypted Email
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//do stuff with decrypted Email
}
}
}
encryption digital-signature smime mimekit
add a comment |
I am writing an application that must be able to read signed and encrypted emails and parse through their contents. I am able to get everything working fine for emails that are only encrypted, but do not know what to do when I get an email that is also signed. Once I decrypt this email instead of having an anticipated 4 parts in a Multipart object, I have only 1 part in a MimePart object with the file name smime.p7m. I do not know how to break this file up or verify the signature. I have found the documentation on verifying a signature (http://www.mimekit.net/docs/html/Working-With-SMime.htm#Verify), but I don't see how this does anything. Obviously there is something that I am just not understanding at this point.
Below is a sample of the code that I am using. Note that this will be refactored after I get everything figured out, but this code is thus far working fine for all emails that I have tested so far that are not signed (may or may not be encrypted).
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
//If the SecureMimeType has not been set as it should, set it to EnvelopedData
if (pkcs7.SecureMimeType == SecureMimeType.Unknown)
{
var content = new MemoryStream();
pkcs7.Content.DecodeTo(content);
content.Position = 0;
pkcs7 = new ApplicationPkcs7Mime(SecureMimeType.EnvelopedData, content);
}
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
////do stuff with decrypted Email
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//do stuff with decrypted Email
}
}
}
encryption digital-signature smime mimekit
1
FWIW, MimeKit 2.1.2 (released a few days ago) fixes the whole SecureMimeType.Unknown thing, so that work-around is not needed anymore. That said, what is the value ofdecrypted.ContentType.MimeType
? Doesdecrypted.GetType()
returnMimeKit.Cryptography.ApplicationPkcs7Mime
? I would imagine that the decrypted part is anotherapplication/pkcs7-mime
part that you should callVerify()
on, but that's just a guess.
– jstedfast
Jan 4 at 13:45
add a comment |
I am writing an application that must be able to read signed and encrypted emails and parse through their contents. I am able to get everything working fine for emails that are only encrypted, but do not know what to do when I get an email that is also signed. Once I decrypt this email instead of having an anticipated 4 parts in a Multipart object, I have only 1 part in a MimePart object with the file name smime.p7m. I do not know how to break this file up or verify the signature. I have found the documentation on verifying a signature (http://www.mimekit.net/docs/html/Working-With-SMime.htm#Verify), but I don't see how this does anything. Obviously there is something that I am just not understanding at this point.
Below is a sample of the code that I am using. Note that this will be refactored after I get everything figured out, but this code is thus far working fine for all emails that I have tested so far that are not signed (may or may not be encrypted).
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
//If the SecureMimeType has not been set as it should, set it to EnvelopedData
if (pkcs7.SecureMimeType == SecureMimeType.Unknown)
{
var content = new MemoryStream();
pkcs7.Content.DecodeTo(content);
content.Position = 0;
pkcs7 = new ApplicationPkcs7Mime(SecureMimeType.EnvelopedData, content);
}
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
////do stuff with decrypted Email
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//do stuff with decrypted Email
}
}
}
encryption digital-signature smime mimekit
I am writing an application that must be able to read signed and encrypted emails and parse through their contents. I am able to get everything working fine for emails that are only encrypted, but do not know what to do when I get an email that is also signed. Once I decrypt this email instead of having an anticipated 4 parts in a Multipart object, I have only 1 part in a MimePart object with the file name smime.p7m. I do not know how to break this file up or verify the signature. I have found the documentation on verifying a signature (http://www.mimekit.net/docs/html/Working-With-SMime.htm#Verify), but I don't see how this does anything. Obviously there is something that I am just not understanding at this point.
Below is a sample of the code that I am using. Note that this will be refactored after I get everything figured out, but this code is thus far working fine for all emails that I have tested so far that are not signed (may or may not be encrypted).
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
//If the SecureMimeType has not been set as it should, set it to EnvelopedData
if (pkcs7.SecureMimeType == SecureMimeType.Unknown)
{
var content = new MemoryStream();
pkcs7.Content.DecodeTo(content);
content.Position = 0;
pkcs7 = new ApplicationPkcs7Mime(SecureMimeType.EnvelopedData, content);
}
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
////do stuff with decrypted Email
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//do stuff with decrypted Email
}
}
}
encryption digital-signature smime mimekit
encryption digital-signature smime mimekit
asked Jan 3 at 21:53
dmoore1181dmoore1181
371521
371521
1
FWIW, MimeKit 2.1.2 (released a few days ago) fixes the whole SecureMimeType.Unknown thing, so that work-around is not needed anymore. That said, what is the value ofdecrypted.ContentType.MimeType
? Doesdecrypted.GetType()
returnMimeKit.Cryptography.ApplicationPkcs7Mime
? I would imagine that the decrypted part is anotherapplication/pkcs7-mime
part that you should callVerify()
on, but that's just a guess.
– jstedfast
Jan 4 at 13:45
add a comment |
1
FWIW, MimeKit 2.1.2 (released a few days ago) fixes the whole SecureMimeType.Unknown thing, so that work-around is not needed anymore. That said, what is the value ofdecrypted.ContentType.MimeType
? Doesdecrypted.GetType()
returnMimeKit.Cryptography.ApplicationPkcs7Mime
? I would imagine that the decrypted part is anotherapplication/pkcs7-mime
part that you should callVerify()
on, but that's just a guess.
– jstedfast
Jan 4 at 13:45
1
1
FWIW, MimeKit 2.1.2 (released a few days ago) fixes the whole SecureMimeType.Unknown thing, so that work-around is not needed anymore. That said, what is the value of
decrypted.ContentType.MimeType
? Does decrypted.GetType()
return MimeKit.Cryptography.ApplicationPkcs7Mime
? I would imagine that the decrypted part is another application/pkcs7-mime
part that you should call Verify()
on, but that's just a guess.– jstedfast
Jan 4 at 13:45
FWIW, MimeKit 2.1.2 (released a few days ago) fixes the whole SecureMimeType.Unknown thing, so that work-around is not needed anymore. That said, what is the value of
decrypted.ContentType.MimeType
? Does decrypted.GetType()
return MimeKit.Cryptography.ApplicationPkcs7Mime
? I would imagine that the decrypted part is another application/pkcs7-mime
part that you should call Verify()
on, but that's just a guess.– jstedfast
Jan 4 at 13:45
add a comment |
1 Answer
1
active
oldest
votes
I have finally figured this out using a combination of the comment from @jstedfast and the information I found in Unable to decrypt p7m using MimeKit. The following is the resulting code to fix this issue:
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
if (decrypted != null && decrypted is MimePart && ((MimePart)decrypted).FileName == "smime.p7m")
{
//We need to verify the signature
var signedDecryptedEntity = decrypted as ApplicationPkcs7Mime;
signedDecryptedEntity.Verify(ctx, out decrypted); //the real decrypted data
}
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
//Do Something with email (decryptedEmailMessage)
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//Do Something with email (decryptedEmailMessage)
}
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030317%2fparsing-signed-and-encrypted-email%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I have finally figured this out using a combination of the comment from @jstedfast and the information I found in Unable to decrypt p7m using MimeKit. The following is the resulting code to fix this issue:
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
if (decrypted != null && decrypted is MimePart && ((MimePart)decrypted).FileName == "smime.p7m")
{
//We need to verify the signature
var signedDecryptedEntity = decrypted as ApplicationPkcs7Mime;
signedDecryptedEntity.Verify(ctx, out decrypted); //the real decrypted data
}
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
//Do Something with email (decryptedEmailMessage)
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//Do Something with email (decryptedEmailMessage)
}
}
}
add a comment |
I have finally figured this out using a combination of the comment from @jstedfast and the information I found in Unable to decrypt p7m using MimeKit. The following is the resulting code to fix this issue:
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
if (decrypted != null && decrypted is MimePart && ((MimePart)decrypted).FileName == "smime.p7m")
{
//We need to verify the signature
var signedDecryptedEntity = decrypted as ApplicationPkcs7Mime;
signedDecryptedEntity.Verify(ctx, out decrypted); //the real decrypted data
}
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
//Do Something with email (decryptedEmailMessage)
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//Do Something with email (decryptedEmailMessage)
}
}
}
add a comment |
I have finally figured this out using a combination of the comment from @jstedfast and the information I found in Unable to decrypt p7m using MimeKit. The following is the resulting code to fix this issue:
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
if (decrypted != null && decrypted is MimePart && ((MimePart)decrypted).FileName == "smime.p7m")
{
//We need to verify the signature
var signedDecryptedEntity = decrypted as ApplicationPkcs7Mime;
signedDecryptedEntity.Verify(ctx, out decrypted); //the real decrypted data
}
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
//Do Something with email (decryptedEmailMessage)
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//Do Something with email (decryptedEmailMessage)
}
}
}
I have finally figured this out using a combination of the comment from @jstedfast and the information I found in Unable to decrypt p7m using MimeKit. The following is the resulting code to fix this issue:
public void decryptAndSendEmails()
{
List<EmailMessage> emails = getEmails();
foreach (var email in emails)
{
var decryptedEmailMessage = new EmailMessage(service);
MimeMessage message;
using (var stream = new MemoryStream(email.MimeContent.Content, false))
{
message = MimeMessage.Load(stream);
}
var pkcs7 = message.BodyParts.OfType<ApplicationPkcs7Mime>().FirstOrDefault();
if (pkcs7 != null)
{
using (var ctx = new TemporarySecureMimeContext())
{
using (var stream = File.OpenRead(ConfigurationManager.AppSettings["certLocation"]))
{
ctx.Import(stream, ConfigurationManager.AppSettings["certPassword"]);
}
var decrypted = pkcs7.Decrypt(ctx);
if (decrypted != null && decrypted is MimePart && ((MimePart)decrypted).FileName == "smime.p7m")
{
//We need to verify the signature
var signedDecryptedEntity = decrypted as ApplicationPkcs7Mime;
signedDecryptedEntity.Verify(ctx, out decrypted); //the real decrypted data
}
var decryptedParts = new List<MimePart>();
if (decrypted is Multipart)
{
decryptedParts = breakMultiPart((Multipart)decrypted);
}
else if (decrypted is MimePart)
{
decryptedParts.Add((MimePart)decrypted);
}
else
{
throw new InvalidOperationException("Unknown Mime part found");
}
var textParts = decryptedParts.Where(r => r is TextPart);
var htmlParts = textParts.Where(x => ((TextPart)x).IsHtml);
var textBodyParts = textParts.Where(x => !((TextPart)x).IsHtml);
var attachmentParts = decryptedParts.Where(r => !(r is TextPart));
if (htmlParts.Any())
{
if (htmlParts.Count() > 1)
{
throw new InvalidOperationException("multiple html body parts.");
}
var htmlPart = (TextPart)htmlParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.HTML, htmlPart.Text);
}
else
{
//Text body
if (textBodyParts.Count() > 1)
{
throw new InvalidOperationException("multiple text body parts.");
}
var textPart = (TextPart)textBodyParts.First();
decryptedEmailMessage.Body = new MessageBody(BodyType.Text, textPart.Text);
}
foreach (var part in attachmentParts)
{
var content = new MemoryStream();
part.Content.DecodeTo(content);
content.Position = 0;
decryptedEmailMessage.Attachments.AddFileAttachment(part.FileName, content);
if (!part.IsAttachment)
{
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).IsInline = true;
decryptedEmailMessage.Attachments.First(r => r.Name == part.FileName).ContentId = part.ContentId;
}
}
}
//Do Something with email (decryptedEmailMessage)
}
else
{
//The email is not encrypted
decryptedEmailMessage = email;
//Do Something with email (decryptedEmailMessage)
}
}
}
answered Jan 4 at 15:58
dmoore1181dmoore1181
371521
371521
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030317%2fparsing-signed-and-encrypted-email%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BjCrl4Z5x2Nx7nTKY90afHCuf Cp xUA3pyOX fkrBNmf,GIVOJC,wC RIp7
1
FWIW, MimeKit 2.1.2 (released a few days ago) fixes the whole SecureMimeType.Unknown thing, so that work-around is not needed anymore. That said, what is the value of
decrypted.ContentType.MimeType
? Doesdecrypted.GetType()
returnMimeKit.Cryptography.ApplicationPkcs7Mime
? I would imagine that the decrypted part is anotherapplication/pkcs7-mime
part that you should callVerify()
on, but that's just a guess.– jstedfast
Jan 4 at 13:45