Check if all checkboxes of form are checked
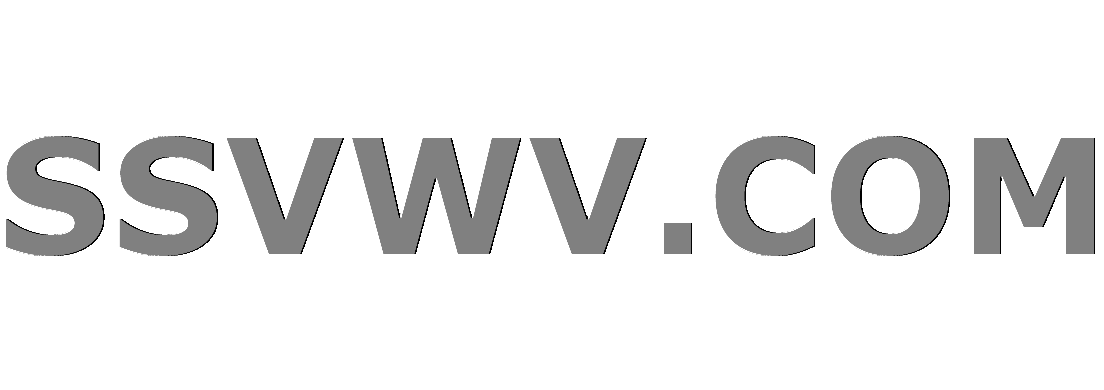
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have multiple checkboxes that I created dinamically from code, So I know I can get checkboxes using:
foreach (var checkBox in this.Controls.OfType<CheckBox>())
{
}
But that I want to know or my desire result is to know if all checkboxes of my form are checked something like a bool returning true if all checkboxes are checked or false if one is missing... How can I achieve that? Regards
bool allChecked = ...
c# winforms
add a comment |
I have multiple checkboxes that I created dinamically from code, So I know I can get checkboxes using:
foreach (var checkBox in this.Controls.OfType<CheckBox>())
{
}
But that I want to know or my desire result is to know if all checkboxes of my form are checked something like a bool returning true if all checkboxes are checked or false if one is missing... How can I achieve that? Regards
bool allChecked = ...
c# winforms
Can't you just get all the unchecked controls by reversing the logic of your where clause and checking that the count is equal to zero?
– Lithium
Jan 3 at 22:08
1
Are any of the CheckBoxes inside panels or other containers?
– LarsTech
Jan 3 at 22:13
Using your code above, you could start out withbool allChecked = true;
and inside yourforeach
add the lines:if (!checkbox.Checked) { allChecked = false; break; }
– Rufus L
Jan 3 at 22:15
add a comment |
I have multiple checkboxes that I created dinamically from code, So I know I can get checkboxes using:
foreach (var checkBox in this.Controls.OfType<CheckBox>())
{
}
But that I want to know or my desire result is to know if all checkboxes of my form are checked something like a bool returning true if all checkboxes are checked or false if one is missing... How can I achieve that? Regards
bool allChecked = ...
c# winforms
I have multiple checkboxes that I created dinamically from code, So I know I can get checkboxes using:
foreach (var checkBox in this.Controls.OfType<CheckBox>())
{
}
But that I want to know or my desire result is to know if all checkboxes of my form are checked something like a bool returning true if all checkboxes are checked or false if one is missing... How can I achieve that? Regards
bool allChecked = ...
c# winforms
c# winforms
edited Jan 3 at 22:08
Jonathan
asked Jan 3 at 22:05
JonathanJonathan
28412
28412
Can't you just get all the unchecked controls by reversing the logic of your where clause and checking that the count is equal to zero?
– Lithium
Jan 3 at 22:08
1
Are any of the CheckBoxes inside panels or other containers?
– LarsTech
Jan 3 at 22:13
Using your code above, you could start out withbool allChecked = true;
and inside yourforeach
add the lines:if (!checkbox.Checked) { allChecked = false; break; }
– Rufus L
Jan 3 at 22:15
add a comment |
Can't you just get all the unchecked controls by reversing the logic of your where clause and checking that the count is equal to zero?
– Lithium
Jan 3 at 22:08
1
Are any of the CheckBoxes inside panels or other containers?
– LarsTech
Jan 3 at 22:13
Using your code above, you could start out withbool allChecked = true;
and inside yourforeach
add the lines:if (!checkbox.Checked) { allChecked = false; break; }
– Rufus L
Jan 3 at 22:15
Can't you just get all the unchecked controls by reversing the logic of your where clause and checking that the count is equal to zero?
– Lithium
Jan 3 at 22:08
Can't you just get all the unchecked controls by reversing the logic of your where clause and checking that the count is equal to zero?
– Lithium
Jan 3 at 22:08
1
1
Are any of the CheckBoxes inside panels or other containers?
– LarsTech
Jan 3 at 22:13
Are any of the CheckBoxes inside panels or other containers?
– LarsTech
Jan 3 at 22:13
Using your code above, you could start out with
bool allChecked = true;
and inside your foreach
add the lines: if (!checkbox.Checked) { allChecked = false; break; }
– Rufus L
Jan 3 at 22:15
Using your code above, you could start out with
bool allChecked = true;
and inside your foreach
add the lines: if (!checkbox.Checked) { allChecked = false; break; }
– Rufus L
Jan 3 at 22:15
add a comment |
3 Answers
3
active
oldest
votes
If you want to verify that all checkboxes on the form are checked, even those that belong to other container controls, you will have to iterate over each control's Controls
collection (not just those belonging to the Form
).
One way to do this is to write a recursive method that takes in a container control (like the Form
) and examines all the controls in it's collection. If any contained control is a Checkbox
and it isn't checked, then return false
. Otherwise, perform a recursive check on that control's Controls
collection. If neither of these checks is false, then return true.
For example:
private static bool ContainedCheckboxesAreChecked(Control containerControl)
{
// For each control in the container
foreach (Control control in containerControl.Controls)
{
// Return false if the control is a checkbox and it's not checked
if (!(control as CheckBox)?.Checked ?? false) return false;
// Do a recursive check on this control's Controls collection
if (!ContainedCheckboxesAreChecked(control)) return false;
}
// If we got this far, return true
return true;
}
Now, if you call this and pass in the main form as the container control, you will examine all controls on the form, including in all containers (even nested containers):
bool allCheckBoxesAreChecked = ContainedCheckboxesAreChecked(this);
add a comment |
Simply as
bool allChecked = this.Controls.OfType<CheckBox>().All(c => c.Checked);
I am not sure what AllControls
(in original unedited post) is. Because this property doesn't seem to be a standard one. This example uses the standard Controls collection present in any control container like the top level Form
The problem is more complex if you have your CheckBoxes distributed inside different control containers. In that case you could use a recursive function that iteratively explore your controls and count how many of them are checked
int result = RecursiveCheck(f.Controls);
if(result > 0)
Console.WriteLine("Something is not checked");
int RecursiveCheck(Control.ControlCollection col)
{
int count = 0;
foreach(Control c in col)
{
if (c.Controls.Count > 0)
{
count += c.Controls.OfType<CheckBox>().Count(x => !x.Checked);
count += RecursiveCheck(c.Controls);
}
}
return count;
}
I try it but it always return True value
– Jonathan
Jan 3 at 22:23
Did you have your checkboxes inside some kind of container? (A panel or a groupbox) In this case you need to use the controls collection of the container
– Steve
Jan 3 at 22:26
Yes, they are inside a FlowLayoutPanel
– Jonathan
Jan 3 at 22:28
Then change this with the name of your FlowLayoutPanel
– Steve
Jan 3 at 22:29
Problem is they are inside FlowLayoutPanel, but inside it I have GroupBoxes, so each GroupBox have different checkboxes, I have list of groupBox so I iterate as:foreach (var g in groupBoxes) { }
, but now, how can I know if all checkboxes are checked if they are in different Groupboxes?
– Jonathan
Jan 3 at 22:42
|
show 1 more comment
if you are sure that Checkboxes available, you can use
var allChecked = this.Controls.OfType<CheckBox>().All(x => x.Checked);
but if there is possibility that the Enumerable
yields no result then you need to use the code below. because All operator returns true
for empty Enumerable
bool allChecked = false;
var checkBoxes = this.Controls.OfType<CheckBox>();
if (checkBoxes.Any())
allChecked = checkboxes.All(x => x.Checked);
I try but it always returning True value
– Jonathan
Jan 3 at 22:22
It returns true but this may be undesirable since no checkboxes is found in your this.Controls
– Derviş Kayımbaşıoğlu
Jan 3 at 22:52
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030437%2fcheck-if-all-checkboxes-of-form-are-checked%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want to verify that all checkboxes on the form are checked, even those that belong to other container controls, you will have to iterate over each control's Controls
collection (not just those belonging to the Form
).
One way to do this is to write a recursive method that takes in a container control (like the Form
) and examines all the controls in it's collection. If any contained control is a Checkbox
and it isn't checked, then return false
. Otherwise, perform a recursive check on that control's Controls
collection. If neither of these checks is false, then return true.
For example:
private static bool ContainedCheckboxesAreChecked(Control containerControl)
{
// For each control in the container
foreach (Control control in containerControl.Controls)
{
// Return false if the control is a checkbox and it's not checked
if (!(control as CheckBox)?.Checked ?? false) return false;
// Do a recursive check on this control's Controls collection
if (!ContainedCheckboxesAreChecked(control)) return false;
}
// If we got this far, return true
return true;
}
Now, if you call this and pass in the main form as the container control, you will examine all controls on the form, including in all containers (even nested containers):
bool allCheckBoxesAreChecked = ContainedCheckboxesAreChecked(this);
add a comment |
If you want to verify that all checkboxes on the form are checked, even those that belong to other container controls, you will have to iterate over each control's Controls
collection (not just those belonging to the Form
).
One way to do this is to write a recursive method that takes in a container control (like the Form
) and examines all the controls in it's collection. If any contained control is a Checkbox
and it isn't checked, then return false
. Otherwise, perform a recursive check on that control's Controls
collection. If neither of these checks is false, then return true.
For example:
private static bool ContainedCheckboxesAreChecked(Control containerControl)
{
// For each control in the container
foreach (Control control in containerControl.Controls)
{
// Return false if the control is a checkbox and it's not checked
if (!(control as CheckBox)?.Checked ?? false) return false;
// Do a recursive check on this control's Controls collection
if (!ContainedCheckboxesAreChecked(control)) return false;
}
// If we got this far, return true
return true;
}
Now, if you call this and pass in the main form as the container control, you will examine all controls on the form, including in all containers (even nested containers):
bool allCheckBoxesAreChecked = ContainedCheckboxesAreChecked(this);
add a comment |
If you want to verify that all checkboxes on the form are checked, even those that belong to other container controls, you will have to iterate over each control's Controls
collection (not just those belonging to the Form
).
One way to do this is to write a recursive method that takes in a container control (like the Form
) and examines all the controls in it's collection. If any contained control is a Checkbox
and it isn't checked, then return false
. Otherwise, perform a recursive check on that control's Controls
collection. If neither of these checks is false, then return true.
For example:
private static bool ContainedCheckboxesAreChecked(Control containerControl)
{
// For each control in the container
foreach (Control control in containerControl.Controls)
{
// Return false if the control is a checkbox and it's not checked
if (!(control as CheckBox)?.Checked ?? false) return false;
// Do a recursive check on this control's Controls collection
if (!ContainedCheckboxesAreChecked(control)) return false;
}
// If we got this far, return true
return true;
}
Now, if you call this and pass in the main form as the container control, you will examine all controls on the form, including in all containers (even nested containers):
bool allCheckBoxesAreChecked = ContainedCheckboxesAreChecked(this);
If you want to verify that all checkboxes on the form are checked, even those that belong to other container controls, you will have to iterate over each control's Controls
collection (not just those belonging to the Form
).
One way to do this is to write a recursive method that takes in a container control (like the Form
) and examines all the controls in it's collection. If any contained control is a Checkbox
and it isn't checked, then return false
. Otherwise, perform a recursive check on that control's Controls
collection. If neither of these checks is false, then return true.
For example:
private static bool ContainedCheckboxesAreChecked(Control containerControl)
{
// For each control in the container
foreach (Control control in containerControl.Controls)
{
// Return false if the control is a checkbox and it's not checked
if (!(control as CheckBox)?.Checked ?? false) return false;
// Do a recursive check on this control's Controls collection
if (!ContainedCheckboxesAreChecked(control)) return false;
}
// If we got this far, return true
return true;
}
Now, if you call this and pass in the main form as the container control, you will examine all controls on the form, including in all containers (even nested containers):
bool allCheckBoxesAreChecked = ContainedCheckboxesAreChecked(this);
answered Jan 3 at 22:59


Rufus LRufus L
19.3k31732
19.3k31732
add a comment |
add a comment |
Simply as
bool allChecked = this.Controls.OfType<CheckBox>().All(c => c.Checked);
I am not sure what AllControls
(in original unedited post) is. Because this property doesn't seem to be a standard one. This example uses the standard Controls collection present in any control container like the top level Form
The problem is more complex if you have your CheckBoxes distributed inside different control containers. In that case you could use a recursive function that iteratively explore your controls and count how many of them are checked
int result = RecursiveCheck(f.Controls);
if(result > 0)
Console.WriteLine("Something is not checked");
int RecursiveCheck(Control.ControlCollection col)
{
int count = 0;
foreach(Control c in col)
{
if (c.Controls.Count > 0)
{
count += c.Controls.OfType<CheckBox>().Count(x => !x.Checked);
count += RecursiveCheck(c.Controls);
}
}
return count;
}
I try it but it always return True value
– Jonathan
Jan 3 at 22:23
Did you have your checkboxes inside some kind of container? (A panel or a groupbox) In this case you need to use the controls collection of the container
– Steve
Jan 3 at 22:26
Yes, they are inside a FlowLayoutPanel
– Jonathan
Jan 3 at 22:28
Then change this with the name of your FlowLayoutPanel
– Steve
Jan 3 at 22:29
Problem is they are inside FlowLayoutPanel, but inside it I have GroupBoxes, so each GroupBox have different checkboxes, I have list of groupBox so I iterate as:foreach (var g in groupBoxes) { }
, but now, how can I know if all checkboxes are checked if they are in different Groupboxes?
– Jonathan
Jan 3 at 22:42
|
show 1 more comment
Simply as
bool allChecked = this.Controls.OfType<CheckBox>().All(c => c.Checked);
I am not sure what AllControls
(in original unedited post) is. Because this property doesn't seem to be a standard one. This example uses the standard Controls collection present in any control container like the top level Form
The problem is more complex if you have your CheckBoxes distributed inside different control containers. In that case you could use a recursive function that iteratively explore your controls and count how many of them are checked
int result = RecursiveCheck(f.Controls);
if(result > 0)
Console.WriteLine("Something is not checked");
int RecursiveCheck(Control.ControlCollection col)
{
int count = 0;
foreach(Control c in col)
{
if (c.Controls.Count > 0)
{
count += c.Controls.OfType<CheckBox>().Count(x => !x.Checked);
count += RecursiveCheck(c.Controls);
}
}
return count;
}
I try it but it always return True value
– Jonathan
Jan 3 at 22:23
Did you have your checkboxes inside some kind of container? (A panel or a groupbox) In this case you need to use the controls collection of the container
– Steve
Jan 3 at 22:26
Yes, they are inside a FlowLayoutPanel
– Jonathan
Jan 3 at 22:28
Then change this with the name of your FlowLayoutPanel
– Steve
Jan 3 at 22:29
Problem is they are inside FlowLayoutPanel, but inside it I have GroupBoxes, so each GroupBox have different checkboxes, I have list of groupBox so I iterate as:foreach (var g in groupBoxes) { }
, but now, how can I know if all checkboxes are checked if they are in different Groupboxes?
– Jonathan
Jan 3 at 22:42
|
show 1 more comment
Simply as
bool allChecked = this.Controls.OfType<CheckBox>().All(c => c.Checked);
I am not sure what AllControls
(in original unedited post) is. Because this property doesn't seem to be a standard one. This example uses the standard Controls collection present in any control container like the top level Form
The problem is more complex if you have your CheckBoxes distributed inside different control containers. In that case you could use a recursive function that iteratively explore your controls and count how many of them are checked
int result = RecursiveCheck(f.Controls);
if(result > 0)
Console.WriteLine("Something is not checked");
int RecursiveCheck(Control.ControlCollection col)
{
int count = 0;
foreach(Control c in col)
{
if (c.Controls.Count > 0)
{
count += c.Controls.OfType<CheckBox>().Count(x => !x.Checked);
count += RecursiveCheck(c.Controls);
}
}
return count;
}
Simply as
bool allChecked = this.Controls.OfType<CheckBox>().All(c => c.Checked);
I am not sure what AllControls
(in original unedited post) is. Because this property doesn't seem to be a standard one. This example uses the standard Controls collection present in any control container like the top level Form
The problem is more complex if you have your CheckBoxes distributed inside different control containers. In that case you could use a recursive function that iteratively explore your controls and count how many of them are checked
int result = RecursiveCheck(f.Controls);
if(result > 0)
Console.WriteLine("Something is not checked");
int RecursiveCheck(Control.ControlCollection col)
{
int count = 0;
foreach(Control c in col)
{
if (c.Controls.Count > 0)
{
count += c.Controls.OfType<CheckBox>().Count(x => !x.Checked);
count += RecursiveCheck(c.Controls);
}
}
return count;
}
edited Jan 3 at 22:53
answered Jan 3 at 22:08


SteveSteve
183k16160222
183k16160222
I try it but it always return True value
– Jonathan
Jan 3 at 22:23
Did you have your checkboxes inside some kind of container? (A panel or a groupbox) In this case you need to use the controls collection of the container
– Steve
Jan 3 at 22:26
Yes, they are inside a FlowLayoutPanel
– Jonathan
Jan 3 at 22:28
Then change this with the name of your FlowLayoutPanel
– Steve
Jan 3 at 22:29
Problem is they are inside FlowLayoutPanel, but inside it I have GroupBoxes, so each GroupBox have different checkboxes, I have list of groupBox so I iterate as:foreach (var g in groupBoxes) { }
, but now, how can I know if all checkboxes are checked if they are in different Groupboxes?
– Jonathan
Jan 3 at 22:42
|
show 1 more comment
I try it but it always return True value
– Jonathan
Jan 3 at 22:23
Did you have your checkboxes inside some kind of container? (A panel or a groupbox) In this case you need to use the controls collection of the container
– Steve
Jan 3 at 22:26
Yes, they are inside a FlowLayoutPanel
– Jonathan
Jan 3 at 22:28
Then change this with the name of your FlowLayoutPanel
– Steve
Jan 3 at 22:29
Problem is they are inside FlowLayoutPanel, but inside it I have GroupBoxes, so each GroupBox have different checkboxes, I have list of groupBox so I iterate as:foreach (var g in groupBoxes) { }
, but now, how can I know if all checkboxes are checked if they are in different Groupboxes?
– Jonathan
Jan 3 at 22:42
I try it but it always return True value
– Jonathan
Jan 3 at 22:23
I try it but it always return True value
– Jonathan
Jan 3 at 22:23
Did you have your checkboxes inside some kind of container? (A panel or a groupbox) In this case you need to use the controls collection of the container
– Steve
Jan 3 at 22:26
Did you have your checkboxes inside some kind of container? (A panel or a groupbox) In this case you need to use the controls collection of the container
– Steve
Jan 3 at 22:26
Yes, they are inside a FlowLayoutPanel
– Jonathan
Jan 3 at 22:28
Yes, they are inside a FlowLayoutPanel
– Jonathan
Jan 3 at 22:28
Then change this with the name of your FlowLayoutPanel
– Steve
Jan 3 at 22:29
Then change this with the name of your FlowLayoutPanel
– Steve
Jan 3 at 22:29
Problem is they are inside FlowLayoutPanel, but inside it I have GroupBoxes, so each GroupBox have different checkboxes, I have list of groupBox so I iterate as:
foreach (var g in groupBoxes) { }
, but now, how can I know if all checkboxes are checked if they are in different Groupboxes?– Jonathan
Jan 3 at 22:42
Problem is they are inside FlowLayoutPanel, but inside it I have GroupBoxes, so each GroupBox have different checkboxes, I have list of groupBox so I iterate as:
foreach (var g in groupBoxes) { }
, but now, how can I know if all checkboxes are checked if they are in different Groupboxes?– Jonathan
Jan 3 at 22:42
|
show 1 more comment
if you are sure that Checkboxes available, you can use
var allChecked = this.Controls.OfType<CheckBox>().All(x => x.Checked);
but if there is possibility that the Enumerable
yields no result then you need to use the code below. because All operator returns true
for empty Enumerable
bool allChecked = false;
var checkBoxes = this.Controls.OfType<CheckBox>();
if (checkBoxes.Any())
allChecked = checkboxes.All(x => x.Checked);
I try but it always returning True value
– Jonathan
Jan 3 at 22:22
It returns true but this may be undesirable since no checkboxes is found in your this.Controls
– Derviş Kayımbaşıoğlu
Jan 3 at 22:52
add a comment |
if you are sure that Checkboxes available, you can use
var allChecked = this.Controls.OfType<CheckBox>().All(x => x.Checked);
but if there is possibility that the Enumerable
yields no result then you need to use the code below. because All operator returns true
for empty Enumerable
bool allChecked = false;
var checkBoxes = this.Controls.OfType<CheckBox>();
if (checkBoxes.Any())
allChecked = checkboxes.All(x => x.Checked);
I try but it always returning True value
– Jonathan
Jan 3 at 22:22
It returns true but this may be undesirable since no checkboxes is found in your this.Controls
– Derviş Kayımbaşıoğlu
Jan 3 at 22:52
add a comment |
if you are sure that Checkboxes available, you can use
var allChecked = this.Controls.OfType<CheckBox>().All(x => x.Checked);
but if there is possibility that the Enumerable
yields no result then you need to use the code below. because All operator returns true
for empty Enumerable
bool allChecked = false;
var checkBoxes = this.Controls.OfType<CheckBox>();
if (checkBoxes.Any())
allChecked = checkboxes.All(x => x.Checked);
if you are sure that Checkboxes available, you can use
var allChecked = this.Controls.OfType<CheckBox>().All(x => x.Checked);
but if there is possibility that the Enumerable
yields no result then you need to use the code below. because All operator returns true
for empty Enumerable
bool allChecked = false;
var checkBoxes = this.Controls.OfType<CheckBox>();
if (checkBoxes.Any())
allChecked = checkboxes.All(x => x.Checked);
edited Jan 3 at 22:23
answered Jan 3 at 22:17


Derviş KayımbaşıoğluDerviş Kayımbaşıoğlu
15.7k22042
15.7k22042
I try but it always returning True value
– Jonathan
Jan 3 at 22:22
It returns true but this may be undesirable since no checkboxes is found in your this.Controls
– Derviş Kayımbaşıoğlu
Jan 3 at 22:52
add a comment |
I try but it always returning True value
– Jonathan
Jan 3 at 22:22
It returns true but this may be undesirable since no checkboxes is found in your this.Controls
– Derviş Kayımbaşıoğlu
Jan 3 at 22:52
I try but it always returning True value
– Jonathan
Jan 3 at 22:22
I try but it always returning True value
– Jonathan
Jan 3 at 22:22
It returns true but this may be undesirable since no checkboxes is found in your this.Controls
– Derviş Kayımbaşıoğlu
Jan 3 at 22:52
It returns true but this may be undesirable since no checkboxes is found in your this.Controls
– Derviş Kayımbaşıoğlu
Jan 3 at 22:52
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030437%2fcheck-if-all-checkboxes-of-form-are-checked%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KOX2TPnQTqc0maY,wyPEDLX6SWhs1BT,U Fjwe
Can't you just get all the unchecked controls by reversing the logic of your where clause and checking that the count is equal to zero?
– Lithium
Jan 3 at 22:08
1
Are any of the CheckBoxes inside panels or other containers?
– LarsTech
Jan 3 at 22:13
Using your code above, you could start out with
bool allChecked = true;
and inside yourforeach
add the lines:if (!checkbox.Checked) { allChecked = false; break; }
– Rufus L
Jan 3 at 22:15