Distinguish between single and Multi touch Android
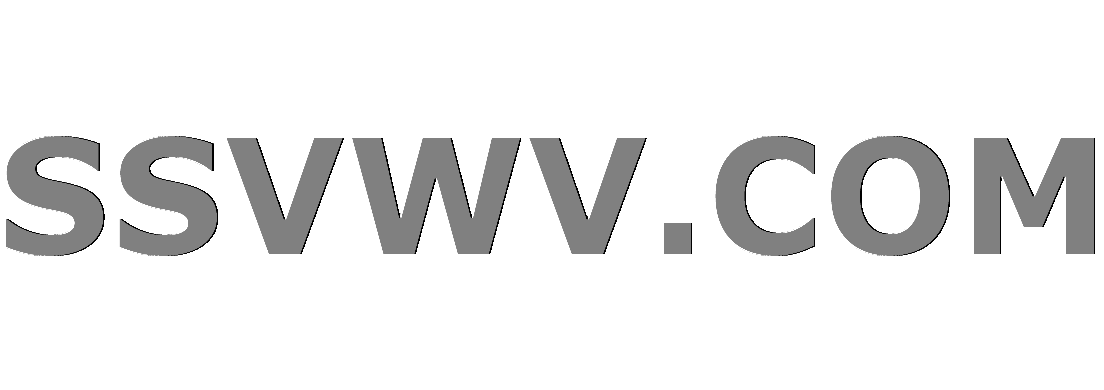
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I don't know if my question is valid or not for this forum. I do apologize in advance if that's not the case.
How to distinguish between single and multiple touch(with two fingers)?
What I want determine exactly is one button will be in corner of the screen.
Case A:
If user touches single touch and hold on this view, drag event should perform
Case B:
If user performs multiple touch that is holding the screen with two fingers with one finger on the button and the other on corner of the opposite side should launch different screen.
The below code I used to for holding on the screen for Case B.
Using the below code how to achieve Case A as well.
// touch listener which handles onLongPress(two points hold) and drag events
private View.OnTouchListener mOnTouchListener = new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent event) {
final int displayWidth = ((WindowManager) mContext.getSystemService(Context.WINDOW_SERVICE))
.getDefaultDisplay().getWidth();
final int displayHeight = ((WindowManager) mContext.getSystemService(Context.WINDOW_SERVICE))
.getDefaultDisplay().getHeight();
// coordinates for drag listener
float dX = 0;
float dY = 0;
// coordinates of first
float firx = 0;
float firy = 0;
// coordinates of second
float secx = 0;
float secy = 0;
Log.d(TAG, "onTouch->(Event : " + event.toString() + ")");
// captures first touch in hold
if (event.getActionMasked() == MotionEvent.ACTION_DOWN) {
firx = event.getX();
firy = event.getY();
dX = view.getX() - event.getRawX();
dY = view.getY() - event.getRawY();
Log.d(TAG, "1st cor:" + firx + ":" + firy);
}
// captures drag event
if (event.getActionMasked() ==MotionEvent.ACTION_MOVE){
//handleAppSwap( Math.round(dX),Math.round(event.getRawX() + dX));
Log.d(TAG, "Drag event happens" );
}
// captures second touch event in hold
if (event.getActionMasked() == MotionEvent.ACTION_POINTER_DOWN && event.getActionIndex() == 0) {
Log.d(TAG, "captures second ");
secx = event.getX();
secy = event.getY();
Log.d(TAG, "2nd cor:" + secx + ":" + secy);
mSecondFingerTimeDown = System.currentTimeMillis();
}
// release event on screen corners
if (event.getActionMasked() == MotionEvent.ACTION_POINTER_UP && event.getActionIndex() == 0) {
if (((firx < X_OFFSET && firy < Y_OFFSET + 100) && (secx > displayWidth - X_OFFSET && secy > displayHeight - Y_OFFSET)) || ((secx < X_OFFSET && secy < Y_OFFSET) && (firx > displayWidth - X_OFFSET && firy > displayHeight - Y_OFFSET))) {
if ((System.currentTimeMillis() - mSecondFingerTimeDown) >= 5000) {
Log.d(TAG, "HOLD AND RELEASE........");
mEngHandler.removeCallbacksAndMessages(null);
Message msg = mEngHandler.obtainMessage(ACTION_BOTTOM_RIGHT_RELEASE);
mEngHandler.sendMessage(msg);
}
}
}
return false;
}
};
Thanks in Advance. Any link or code will be helpful


add a comment |
I don't know if my question is valid or not for this forum. I do apologize in advance if that's not the case.
How to distinguish between single and multiple touch(with two fingers)?
What I want determine exactly is one button will be in corner of the screen.
Case A:
If user touches single touch and hold on this view, drag event should perform
Case B:
If user performs multiple touch that is holding the screen with two fingers with one finger on the button and the other on corner of the opposite side should launch different screen.
The below code I used to for holding on the screen for Case B.
Using the below code how to achieve Case A as well.
// touch listener which handles onLongPress(two points hold) and drag events
private View.OnTouchListener mOnTouchListener = new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent event) {
final int displayWidth = ((WindowManager) mContext.getSystemService(Context.WINDOW_SERVICE))
.getDefaultDisplay().getWidth();
final int displayHeight = ((WindowManager) mContext.getSystemService(Context.WINDOW_SERVICE))
.getDefaultDisplay().getHeight();
// coordinates for drag listener
float dX = 0;
float dY = 0;
// coordinates of first
float firx = 0;
float firy = 0;
// coordinates of second
float secx = 0;
float secy = 0;
Log.d(TAG, "onTouch->(Event : " + event.toString() + ")");
// captures first touch in hold
if (event.getActionMasked() == MotionEvent.ACTION_DOWN) {
firx = event.getX();
firy = event.getY();
dX = view.getX() - event.getRawX();
dY = view.getY() - event.getRawY();
Log.d(TAG, "1st cor:" + firx + ":" + firy);
}
// captures drag event
if (event.getActionMasked() ==MotionEvent.ACTION_MOVE){
//handleAppSwap( Math.round(dX),Math.round(event.getRawX() + dX));
Log.d(TAG, "Drag event happens" );
}
// captures second touch event in hold
if (event.getActionMasked() == MotionEvent.ACTION_POINTER_DOWN && event.getActionIndex() == 0) {
Log.d(TAG, "captures second ");
secx = event.getX();
secy = event.getY();
Log.d(TAG, "2nd cor:" + secx + ":" + secy);
mSecondFingerTimeDown = System.currentTimeMillis();
}
// release event on screen corners
if (event.getActionMasked() == MotionEvent.ACTION_POINTER_UP && event.getActionIndex() == 0) {
if (((firx < X_OFFSET && firy < Y_OFFSET + 100) && (secx > displayWidth - X_OFFSET && secy > displayHeight - Y_OFFSET)) || ((secx < X_OFFSET && secy < Y_OFFSET) && (firx > displayWidth - X_OFFSET && firy > displayHeight - Y_OFFSET))) {
if ((System.currentTimeMillis() - mSecondFingerTimeDown) >= 5000) {
Log.d(TAG, "HOLD AND RELEASE........");
mEngHandler.removeCallbacksAndMessages(null);
Message msg = mEngHandler.obtainMessage(ACTION_BOTTOM_RIGHT_RELEASE);
mEngHandler.sendMessage(msg);
}
}
}
return false;
}
};
Thanks in Advance. Any link or code will be helpful


Why not just read Google's Android Doc? developer.android.com/training/gestures/multi
– emandt
Jan 4 at 8:19
Thanks for your reply . I have gone through this before putting up the question. docs mentioned event.getPointerCount() > 1 should be used.for multi touch events I tried with sample app even for multitouches for first time and last time it will go to single touch block in between multitouches code will get executed .
– Manju
Jan 4 at 8:58
add a comment |
I don't know if my question is valid or not for this forum. I do apologize in advance if that's not the case.
How to distinguish between single and multiple touch(with two fingers)?
What I want determine exactly is one button will be in corner of the screen.
Case A:
If user touches single touch and hold on this view, drag event should perform
Case B:
If user performs multiple touch that is holding the screen with two fingers with one finger on the button and the other on corner of the opposite side should launch different screen.
The below code I used to for holding on the screen for Case B.
Using the below code how to achieve Case A as well.
// touch listener which handles onLongPress(two points hold) and drag events
private View.OnTouchListener mOnTouchListener = new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent event) {
final int displayWidth = ((WindowManager) mContext.getSystemService(Context.WINDOW_SERVICE))
.getDefaultDisplay().getWidth();
final int displayHeight = ((WindowManager) mContext.getSystemService(Context.WINDOW_SERVICE))
.getDefaultDisplay().getHeight();
// coordinates for drag listener
float dX = 0;
float dY = 0;
// coordinates of first
float firx = 0;
float firy = 0;
// coordinates of second
float secx = 0;
float secy = 0;
Log.d(TAG, "onTouch->(Event : " + event.toString() + ")");
// captures first touch in hold
if (event.getActionMasked() == MotionEvent.ACTION_DOWN) {
firx = event.getX();
firy = event.getY();
dX = view.getX() - event.getRawX();
dY = view.getY() - event.getRawY();
Log.d(TAG, "1st cor:" + firx + ":" + firy);
}
// captures drag event
if (event.getActionMasked() ==MotionEvent.ACTION_MOVE){
//handleAppSwap( Math.round(dX),Math.round(event.getRawX() + dX));
Log.d(TAG, "Drag event happens" );
}
// captures second touch event in hold
if (event.getActionMasked() == MotionEvent.ACTION_POINTER_DOWN && event.getActionIndex() == 0) {
Log.d(TAG, "captures second ");
secx = event.getX();
secy = event.getY();
Log.d(TAG, "2nd cor:" + secx + ":" + secy);
mSecondFingerTimeDown = System.currentTimeMillis();
}
// release event on screen corners
if (event.getActionMasked() == MotionEvent.ACTION_POINTER_UP && event.getActionIndex() == 0) {
if (((firx < X_OFFSET && firy < Y_OFFSET + 100) && (secx > displayWidth - X_OFFSET && secy > displayHeight - Y_OFFSET)) || ((secx < X_OFFSET && secy < Y_OFFSET) && (firx > displayWidth - X_OFFSET && firy > displayHeight - Y_OFFSET))) {
if ((System.currentTimeMillis() - mSecondFingerTimeDown) >= 5000) {
Log.d(TAG, "HOLD AND RELEASE........");
mEngHandler.removeCallbacksAndMessages(null);
Message msg = mEngHandler.obtainMessage(ACTION_BOTTOM_RIGHT_RELEASE);
mEngHandler.sendMessage(msg);
}
}
}
return false;
}
};
Thanks in Advance. Any link or code will be helpful


I don't know if my question is valid or not for this forum. I do apologize in advance if that's not the case.
How to distinguish between single and multiple touch(with two fingers)?
What I want determine exactly is one button will be in corner of the screen.
Case A:
If user touches single touch and hold on this view, drag event should perform
Case B:
If user performs multiple touch that is holding the screen with two fingers with one finger on the button and the other on corner of the opposite side should launch different screen.
The below code I used to for holding on the screen for Case B.
Using the below code how to achieve Case A as well.
// touch listener which handles onLongPress(two points hold) and drag events
private View.OnTouchListener mOnTouchListener = new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent event) {
final int displayWidth = ((WindowManager) mContext.getSystemService(Context.WINDOW_SERVICE))
.getDefaultDisplay().getWidth();
final int displayHeight = ((WindowManager) mContext.getSystemService(Context.WINDOW_SERVICE))
.getDefaultDisplay().getHeight();
// coordinates for drag listener
float dX = 0;
float dY = 0;
// coordinates of first
float firx = 0;
float firy = 0;
// coordinates of second
float secx = 0;
float secy = 0;
Log.d(TAG, "onTouch->(Event : " + event.toString() + ")");
// captures first touch in hold
if (event.getActionMasked() == MotionEvent.ACTION_DOWN) {
firx = event.getX();
firy = event.getY();
dX = view.getX() - event.getRawX();
dY = view.getY() - event.getRawY();
Log.d(TAG, "1st cor:" + firx + ":" + firy);
}
// captures drag event
if (event.getActionMasked() ==MotionEvent.ACTION_MOVE){
//handleAppSwap( Math.round(dX),Math.round(event.getRawX() + dX));
Log.d(TAG, "Drag event happens" );
}
// captures second touch event in hold
if (event.getActionMasked() == MotionEvent.ACTION_POINTER_DOWN && event.getActionIndex() == 0) {
Log.d(TAG, "captures second ");
secx = event.getX();
secy = event.getY();
Log.d(TAG, "2nd cor:" + secx + ":" + secy);
mSecondFingerTimeDown = System.currentTimeMillis();
}
// release event on screen corners
if (event.getActionMasked() == MotionEvent.ACTION_POINTER_UP && event.getActionIndex() == 0) {
if (((firx < X_OFFSET && firy < Y_OFFSET + 100) && (secx > displayWidth - X_OFFSET && secy > displayHeight - Y_OFFSET)) || ((secx < X_OFFSET && secy < Y_OFFSET) && (firx > displayWidth - X_OFFSET && firy > displayHeight - Y_OFFSET))) {
if ((System.currentTimeMillis() - mSecondFingerTimeDown) >= 5000) {
Log.d(TAG, "HOLD AND RELEASE........");
mEngHandler.removeCallbacksAndMessages(null);
Message msg = mEngHandler.obtainMessage(ACTION_BOTTOM_RIGHT_RELEASE);
mEngHandler.sendMessage(msg);
}
}
}
return false;
}
};
Thanks in Advance. Any link or code will be helpful




edited Jan 4 at 7:55


Nero
7091520
7091520
asked Jan 4 at 7:48


ManjuManju
525417
525417
Why not just read Google's Android Doc? developer.android.com/training/gestures/multi
– emandt
Jan 4 at 8:19
Thanks for your reply . I have gone through this before putting up the question. docs mentioned event.getPointerCount() > 1 should be used.for multi touch events I tried with sample app even for multitouches for first time and last time it will go to single touch block in between multitouches code will get executed .
– Manju
Jan 4 at 8:58
add a comment |
Why not just read Google's Android Doc? developer.android.com/training/gestures/multi
– emandt
Jan 4 at 8:19
Thanks for your reply . I have gone through this before putting up the question. docs mentioned event.getPointerCount() > 1 should be used.for multi touch events I tried with sample app even for multitouches for first time and last time it will go to single touch block in between multitouches code will get executed .
– Manju
Jan 4 at 8:58
Why not just read Google's Android Doc? developer.android.com/training/gestures/multi
– emandt
Jan 4 at 8:19
Why not just read Google's Android Doc? developer.android.com/training/gestures/multi
– emandt
Jan 4 at 8:19
Thanks for your reply . I have gone through this before putting up the question. docs mentioned event.getPointerCount() > 1 should be used.for multi touch events I tried with sample app even for multitouches for first time and last time it will go to single touch block in between multitouches code will get executed .
– Manju
Jan 4 at 8:58
Thanks for your reply . I have gone through this before putting up the question. docs mentioned event.getPointerCount() > 1 should be used.for multi touch events I tried with sample app even for multitouches for first time and last time it will go to single touch block in between multitouches code will get executed .
– Manju
Jan 4 at 8:58
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54034942%2fdistinguish-between-single-and-multi-touch-android%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54034942%2fdistinguish-between-single-and-multi-touch-android%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jG0t6,pI,eywpxmzGn7vH 4vaHiL,ho9J,z,3vxuImJRUZU8dlGGdmJxMc1y55 PhqwXU8032rcolT 4faKB,Q
Why not just read Google's Android Doc? developer.android.com/training/gestures/multi
– emandt
Jan 4 at 8:19
Thanks for your reply . I have gone through this before putting up the question. docs mentioned event.getPointerCount() > 1 should be used.for multi touch events I tried with sample app even for multitouches for first time and last time it will go to single touch block in between multitouches code will get executed .
– Manju
Jan 4 at 8:58