Swift - check if a timestamp is yesterday, today, tomorrow, or X days ago
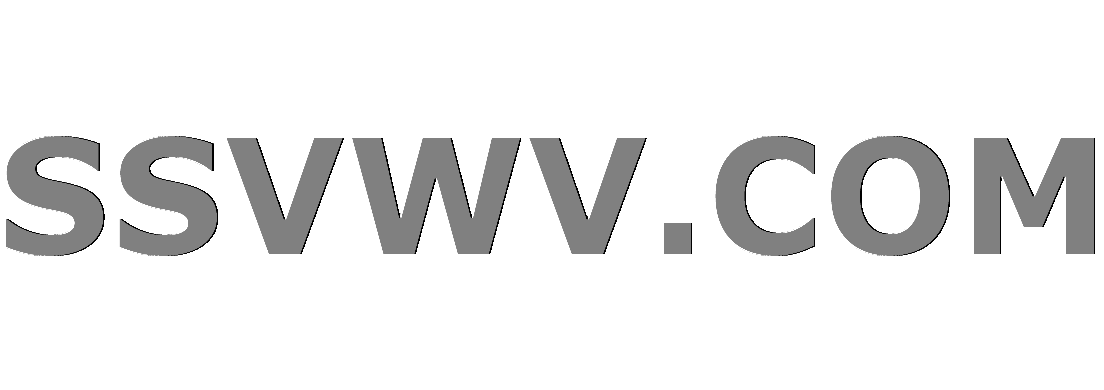
Multi tool use
I'm trying to work out how to decide if a given timestamp occurs today, or +1 / -1 days. Essentially, I'd like to do something like this (Pseudocode)
IF days_from_today(timestamp) == -1 RETURN 'Yesterday'
ELSE IF days_from_today(timestamp) == 0 RETURN 'Today'
ELSE IF days_from_today(timestamp) == 1 RETURN 'Tomorrow'
ELSE IF days_from_today(timestamp) < 1 RETURN days_from_today(timestamp) + ' days ago'
ELSE RETURN 'In ' + days_from_today(timestamp) + ' ago'
Crucially though, it needs to be in Swift and I'm struggling with the NSDate / NSCalendar objects. I started with working out the time difference like this:
let calendar = NSCalendar.currentCalendar()
let date = NSDate(timeIntervalSince1970: Double(timestamp))
let timeDifference = calendar.components([.Second,.Minute,.Day,.Hour],
fromDate: date, toDate: NSDate(), options: NSCalendarOptions())
However comparing in this way isn't easy, because the .Day
is different depending on the time of day and the timestamp. In PHP I'd just use mktime to create a new date, based on the start of the day (i.e. mktime(0,0,0)
), but I'm not sure of the easiest way to do that in Swift.
Does anybody have a good idea on how to approach this? Perhaps an extension to NSDate or something similar would be best?
ios swift nsdate nscalendar
add a comment |
I'm trying to work out how to decide if a given timestamp occurs today, or +1 / -1 days. Essentially, I'd like to do something like this (Pseudocode)
IF days_from_today(timestamp) == -1 RETURN 'Yesterday'
ELSE IF days_from_today(timestamp) == 0 RETURN 'Today'
ELSE IF days_from_today(timestamp) == 1 RETURN 'Tomorrow'
ELSE IF days_from_today(timestamp) < 1 RETURN days_from_today(timestamp) + ' days ago'
ELSE RETURN 'In ' + days_from_today(timestamp) + ' ago'
Crucially though, it needs to be in Swift and I'm struggling with the NSDate / NSCalendar objects. I started with working out the time difference like this:
let calendar = NSCalendar.currentCalendar()
let date = NSDate(timeIntervalSince1970: Double(timestamp))
let timeDifference = calendar.components([.Second,.Minute,.Day,.Hour],
fromDate: date, toDate: NSDate(), options: NSCalendarOptions())
However comparing in this way isn't easy, because the .Day
is different depending on the time of day and the timestamp. In PHP I'd just use mktime to create a new date, based on the start of the day (i.e. mktime(0,0,0)
), but I'm not sure of the easiest way to do that in Swift.
Does anybody have a good idea on how to approach this? Perhaps an extension to NSDate or something similar would be best?
ios swift nsdate nscalendar
add a comment |
I'm trying to work out how to decide if a given timestamp occurs today, or +1 / -1 days. Essentially, I'd like to do something like this (Pseudocode)
IF days_from_today(timestamp) == -1 RETURN 'Yesterday'
ELSE IF days_from_today(timestamp) == 0 RETURN 'Today'
ELSE IF days_from_today(timestamp) == 1 RETURN 'Tomorrow'
ELSE IF days_from_today(timestamp) < 1 RETURN days_from_today(timestamp) + ' days ago'
ELSE RETURN 'In ' + days_from_today(timestamp) + ' ago'
Crucially though, it needs to be in Swift and I'm struggling with the NSDate / NSCalendar objects. I started with working out the time difference like this:
let calendar = NSCalendar.currentCalendar()
let date = NSDate(timeIntervalSince1970: Double(timestamp))
let timeDifference = calendar.components([.Second,.Minute,.Day,.Hour],
fromDate: date, toDate: NSDate(), options: NSCalendarOptions())
However comparing in this way isn't easy, because the .Day
is different depending on the time of day and the timestamp. In PHP I'd just use mktime to create a new date, based on the start of the day (i.e. mktime(0,0,0)
), but I'm not sure of the easiest way to do that in Swift.
Does anybody have a good idea on how to approach this? Perhaps an extension to NSDate or something similar would be best?
ios swift nsdate nscalendar
I'm trying to work out how to decide if a given timestamp occurs today, or +1 / -1 days. Essentially, I'd like to do something like this (Pseudocode)
IF days_from_today(timestamp) == -1 RETURN 'Yesterday'
ELSE IF days_from_today(timestamp) == 0 RETURN 'Today'
ELSE IF days_from_today(timestamp) == 1 RETURN 'Tomorrow'
ELSE IF days_from_today(timestamp) < 1 RETURN days_from_today(timestamp) + ' days ago'
ELSE RETURN 'In ' + days_from_today(timestamp) + ' ago'
Crucially though, it needs to be in Swift and I'm struggling with the NSDate / NSCalendar objects. I started with working out the time difference like this:
let calendar = NSCalendar.currentCalendar()
let date = NSDate(timeIntervalSince1970: Double(timestamp))
let timeDifference = calendar.components([.Second,.Minute,.Day,.Hour],
fromDate: date, toDate: NSDate(), options: NSCalendarOptions())
However comparing in this way isn't easy, because the .Day
is different depending on the time of day and the timestamp. In PHP I'd just use mktime to create a new date, based on the start of the day (i.e. mktime(0,0,0)
), but I'm not sure of the easiest way to do that in Swift.
Does anybody have a good idea on how to approach this? Perhaps an extension to NSDate or something similar would be best?
ios swift nsdate nscalendar
ios swift nsdate nscalendar
edited Sep 6 '16 at 9:21
Ben
asked Sep 6 '16 at 9:13


BenBen
1,82222140
1,82222140
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
Calendar
has methods for all three cases
func isDateInYesterday(_ date: Date) -> Bool
func isDateInToday(_ date: Date) -> Bool
func isDateInTomorrow(_ date: Date) -> Bool
To calculate the days earlier than yesterday use
func dateComponents(_ components: Set<Calendar.Component>,
from start: Date,
to end: Date) -> DateComponents
pass [.day]
to components
and get the day
property from the result.
This is a function which considers also is in
for earlier and later dates by stripping the time part (Swift 3+).
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
if calendar.isDateInYesterday(date) { return "Yesterday" }
else if calendar.isDateInToday(date) { return "Today" }
else if calendar.isDateInTomorrow(date) { return "Tomorrow" }
else {
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if day < 1 { return "(-day) days ago" }
else { return "In (day) days" }
}
}
Alternatively you could use DateFormatter
for Yesterday, Today and Tomorrow to get localized strings for free
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if abs(day) < 2 {
let formatter = DateFormatter()
formatter.dateStyle = .short
formatter.timeStyle = .none
formatter.doesRelativeDateFormatting = true
return formatter.string(from: date)
} else if day > 1 {
return "In (day) days"
} else {
return "(-day) days ago"
}
}
Thank you! The top part looks perfect. With the bottom part, it could be 10am here, but the timestamp might be 9am tomorrow, which would give a different result to if it was 11am tomorrow. Or have I mis-understood how that works?
– Ben
Sep 6 '16 at 9:33
Great stuff, thanks again. The only change I made was the days ago line, by adding anabs
to remove the negation, i.e.return "(abs(day)) days ago"
– Ben
Sep 6 '16 at 9:52
Of course, thanks :-)
– vadian
Sep 6 '16 at 9:54
could u help me with this question :- stackoverflow.com/questions/48320572/… @vadian
– Dilip Tiwari
Jan 18 '18 at 12:02
Your "Best" answer requires manual calculations and checks for each case while some work could be done by iOS by default
– Vyachaslav Gerchicov
Jan 3 at 9:08
|
show 6 more comments
Swift 3/4:
Calendar.current.isDateInToday(yourDate)
Calendar.current.isDateInYesterday(yourDate)
Calendar.current.isDateInTomorrow(yourDate)
Additionally:
Calendar.current.isDateInWeekend(yourDate)
Note that for some countries weekend may be different than Saturday-Sunday, it depends on the calendar.
You can also use autoupdatingCurrent
instead of current
calendar which will track user updates. You use it the same way:
Calendar.autoupdatingCurrent.isDateInToday(yourDate)
Calendar
is a type alias for the NSCalendar
.
1
Omg yes! Just what I needed.
– Khoury
Apr 4 '18 at 5:55
add a comment |
Swift 4 update:
let calendar = Calendar.current
let date = Date()
calendar.isDateInYesterday(date)
calendar.isDateInToday(date)
calendar.isDateInTomorrow(date)
add a comment |
NSCalender has new methods that you can use directly.
NSCalendar.currentCalendar().isDateInTomorrow(NSDate())//Replace NSDate() with your date
NSCalendar.currentCalendar().isDateInYesterday()
NSCalendar.currentCalendar().isDateInTomorrow()
Hope this helps
add a comment |
1)According to your example you want to receive labels "Yesterday", "Today" and etc. iOS can do this by default:
https://developer.apple.com/documentation/foundation/nsdateformatter/1415848-doesrelativedateformatting?language=objc
2)If you want to compute your custom label when iOS don't add these labels by itself then alternatively you can use 2 DateFormatter
objects with both doesRelativeDateFormatting == true
and doesRelativeDateFormatting == false
and compare if their result date strings are the same or different
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f39345101%2fswift-check-if-a-timestamp-is-yesterday-today-tomorrow-or-x-days-ago%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Calendar
has methods for all three cases
func isDateInYesterday(_ date: Date) -> Bool
func isDateInToday(_ date: Date) -> Bool
func isDateInTomorrow(_ date: Date) -> Bool
To calculate the days earlier than yesterday use
func dateComponents(_ components: Set<Calendar.Component>,
from start: Date,
to end: Date) -> DateComponents
pass [.day]
to components
and get the day
property from the result.
This is a function which considers also is in
for earlier and later dates by stripping the time part (Swift 3+).
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
if calendar.isDateInYesterday(date) { return "Yesterday" }
else if calendar.isDateInToday(date) { return "Today" }
else if calendar.isDateInTomorrow(date) { return "Tomorrow" }
else {
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if day < 1 { return "(-day) days ago" }
else { return "In (day) days" }
}
}
Alternatively you could use DateFormatter
for Yesterday, Today and Tomorrow to get localized strings for free
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if abs(day) < 2 {
let formatter = DateFormatter()
formatter.dateStyle = .short
formatter.timeStyle = .none
formatter.doesRelativeDateFormatting = true
return formatter.string(from: date)
} else if day > 1 {
return "In (day) days"
} else {
return "(-day) days ago"
}
}
Thank you! The top part looks perfect. With the bottom part, it could be 10am here, but the timestamp might be 9am tomorrow, which would give a different result to if it was 11am tomorrow. Or have I mis-understood how that works?
– Ben
Sep 6 '16 at 9:33
Great stuff, thanks again. The only change I made was the days ago line, by adding anabs
to remove the negation, i.e.return "(abs(day)) days ago"
– Ben
Sep 6 '16 at 9:52
Of course, thanks :-)
– vadian
Sep 6 '16 at 9:54
could u help me with this question :- stackoverflow.com/questions/48320572/… @vadian
– Dilip Tiwari
Jan 18 '18 at 12:02
Your "Best" answer requires manual calculations and checks for each case while some work could be done by iOS by default
– Vyachaslav Gerchicov
Jan 3 at 9:08
|
show 6 more comments
Calendar
has methods for all three cases
func isDateInYesterday(_ date: Date) -> Bool
func isDateInToday(_ date: Date) -> Bool
func isDateInTomorrow(_ date: Date) -> Bool
To calculate the days earlier than yesterday use
func dateComponents(_ components: Set<Calendar.Component>,
from start: Date,
to end: Date) -> DateComponents
pass [.day]
to components
and get the day
property from the result.
This is a function which considers also is in
for earlier and later dates by stripping the time part (Swift 3+).
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
if calendar.isDateInYesterday(date) { return "Yesterday" }
else if calendar.isDateInToday(date) { return "Today" }
else if calendar.isDateInTomorrow(date) { return "Tomorrow" }
else {
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if day < 1 { return "(-day) days ago" }
else { return "In (day) days" }
}
}
Alternatively you could use DateFormatter
for Yesterday, Today and Tomorrow to get localized strings for free
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if abs(day) < 2 {
let formatter = DateFormatter()
formatter.dateStyle = .short
formatter.timeStyle = .none
formatter.doesRelativeDateFormatting = true
return formatter.string(from: date)
} else if day > 1 {
return "In (day) days"
} else {
return "(-day) days ago"
}
}
Thank you! The top part looks perfect. With the bottom part, it could be 10am here, but the timestamp might be 9am tomorrow, which would give a different result to if it was 11am tomorrow. Or have I mis-understood how that works?
– Ben
Sep 6 '16 at 9:33
Great stuff, thanks again. The only change I made was the days ago line, by adding anabs
to remove the negation, i.e.return "(abs(day)) days ago"
– Ben
Sep 6 '16 at 9:52
Of course, thanks :-)
– vadian
Sep 6 '16 at 9:54
could u help me with this question :- stackoverflow.com/questions/48320572/… @vadian
– Dilip Tiwari
Jan 18 '18 at 12:02
Your "Best" answer requires manual calculations and checks for each case while some work could be done by iOS by default
– Vyachaslav Gerchicov
Jan 3 at 9:08
|
show 6 more comments
Calendar
has methods for all three cases
func isDateInYesterday(_ date: Date) -> Bool
func isDateInToday(_ date: Date) -> Bool
func isDateInTomorrow(_ date: Date) -> Bool
To calculate the days earlier than yesterday use
func dateComponents(_ components: Set<Calendar.Component>,
from start: Date,
to end: Date) -> DateComponents
pass [.day]
to components
and get the day
property from the result.
This is a function which considers also is in
for earlier and later dates by stripping the time part (Swift 3+).
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
if calendar.isDateInYesterday(date) { return "Yesterday" }
else if calendar.isDateInToday(date) { return "Today" }
else if calendar.isDateInTomorrow(date) { return "Tomorrow" }
else {
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if day < 1 { return "(-day) days ago" }
else { return "In (day) days" }
}
}
Alternatively you could use DateFormatter
for Yesterday, Today and Tomorrow to get localized strings for free
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if abs(day) < 2 {
let formatter = DateFormatter()
formatter.dateStyle = .short
formatter.timeStyle = .none
formatter.doesRelativeDateFormatting = true
return formatter.string(from: date)
} else if day > 1 {
return "In (day) days"
} else {
return "(-day) days ago"
}
}
Calendar
has methods for all three cases
func isDateInYesterday(_ date: Date) -> Bool
func isDateInToday(_ date: Date) -> Bool
func isDateInTomorrow(_ date: Date) -> Bool
To calculate the days earlier than yesterday use
func dateComponents(_ components: Set<Calendar.Component>,
from start: Date,
to end: Date) -> DateComponents
pass [.day]
to components
and get the day
property from the result.
This is a function which considers also is in
for earlier and later dates by stripping the time part (Swift 3+).
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
if calendar.isDateInYesterday(date) { return "Yesterday" }
else if calendar.isDateInToday(date) { return "Today" }
else if calendar.isDateInTomorrow(date) { return "Tomorrow" }
else {
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if day < 1 { return "(-day) days ago" }
else { return "In (day) days" }
}
}
Alternatively you could use DateFormatter
for Yesterday, Today and Tomorrow to get localized strings for free
func dayDifference(from interval : TimeInterval) -> String
{
let calendar = Calendar.current
let date = Date(timeIntervalSince1970: interval)
let startOfNow = calendar.startOfDay(for: Date())
let startOfTimeStamp = calendar.startOfDay(for: date)
let components = calendar.dateComponents([.day], from: startOfNow, to: startOfTimeStamp)
let day = components.day!
if abs(day) < 2 {
let formatter = DateFormatter()
formatter.dateStyle = .short
formatter.timeStyle = .none
formatter.doesRelativeDateFormatting = true
return formatter.string(from: date)
} else if day > 1 {
return "In (day) days"
} else {
return "(-day) days ago"
}
}
edited Jan 3 at 15:11
answered Sep 6 '16 at 9:17


vadianvadian
153k17165190
153k17165190
Thank you! The top part looks perfect. With the bottom part, it could be 10am here, but the timestamp might be 9am tomorrow, which would give a different result to if it was 11am tomorrow. Or have I mis-understood how that works?
– Ben
Sep 6 '16 at 9:33
Great stuff, thanks again. The only change I made was the days ago line, by adding anabs
to remove the negation, i.e.return "(abs(day)) days ago"
– Ben
Sep 6 '16 at 9:52
Of course, thanks :-)
– vadian
Sep 6 '16 at 9:54
could u help me with this question :- stackoverflow.com/questions/48320572/… @vadian
– Dilip Tiwari
Jan 18 '18 at 12:02
Your "Best" answer requires manual calculations and checks for each case while some work could be done by iOS by default
– Vyachaslav Gerchicov
Jan 3 at 9:08
|
show 6 more comments
Thank you! The top part looks perfect. With the bottom part, it could be 10am here, but the timestamp might be 9am tomorrow, which would give a different result to if it was 11am tomorrow. Or have I mis-understood how that works?
– Ben
Sep 6 '16 at 9:33
Great stuff, thanks again. The only change I made was the days ago line, by adding anabs
to remove the negation, i.e.return "(abs(day)) days ago"
– Ben
Sep 6 '16 at 9:52
Of course, thanks :-)
– vadian
Sep 6 '16 at 9:54
could u help me with this question :- stackoverflow.com/questions/48320572/… @vadian
– Dilip Tiwari
Jan 18 '18 at 12:02
Your "Best" answer requires manual calculations and checks for each case while some work could be done by iOS by default
– Vyachaslav Gerchicov
Jan 3 at 9:08
Thank you! The top part looks perfect. With the bottom part, it could be 10am here, but the timestamp might be 9am tomorrow, which would give a different result to if it was 11am tomorrow. Or have I mis-understood how that works?
– Ben
Sep 6 '16 at 9:33
Thank you! The top part looks perfect. With the bottom part, it could be 10am here, but the timestamp might be 9am tomorrow, which would give a different result to if it was 11am tomorrow. Or have I mis-understood how that works?
– Ben
Sep 6 '16 at 9:33
Great stuff, thanks again. The only change I made was the days ago line, by adding an
abs
to remove the negation, i.e. return "(abs(day)) days ago"
– Ben
Sep 6 '16 at 9:52
Great stuff, thanks again. The only change I made was the days ago line, by adding an
abs
to remove the negation, i.e. return "(abs(day)) days ago"
– Ben
Sep 6 '16 at 9:52
Of course, thanks :-)
– vadian
Sep 6 '16 at 9:54
Of course, thanks :-)
– vadian
Sep 6 '16 at 9:54
could u help me with this question :- stackoverflow.com/questions/48320572/… @vadian
– Dilip Tiwari
Jan 18 '18 at 12:02
could u help me with this question :- stackoverflow.com/questions/48320572/… @vadian
– Dilip Tiwari
Jan 18 '18 at 12:02
Your "Best" answer requires manual calculations and checks for each case while some work could be done by iOS by default
– Vyachaslav Gerchicov
Jan 3 at 9:08
Your "Best" answer requires manual calculations and checks for each case while some work could be done by iOS by default
– Vyachaslav Gerchicov
Jan 3 at 9:08
|
show 6 more comments
Swift 3/4:
Calendar.current.isDateInToday(yourDate)
Calendar.current.isDateInYesterday(yourDate)
Calendar.current.isDateInTomorrow(yourDate)
Additionally:
Calendar.current.isDateInWeekend(yourDate)
Note that for some countries weekend may be different than Saturday-Sunday, it depends on the calendar.
You can also use autoupdatingCurrent
instead of current
calendar which will track user updates. You use it the same way:
Calendar.autoupdatingCurrent.isDateInToday(yourDate)
Calendar
is a type alias for the NSCalendar
.
1
Omg yes! Just what I needed.
– Khoury
Apr 4 '18 at 5:55
add a comment |
Swift 3/4:
Calendar.current.isDateInToday(yourDate)
Calendar.current.isDateInYesterday(yourDate)
Calendar.current.isDateInTomorrow(yourDate)
Additionally:
Calendar.current.isDateInWeekend(yourDate)
Note that for some countries weekend may be different than Saturday-Sunday, it depends on the calendar.
You can also use autoupdatingCurrent
instead of current
calendar which will track user updates. You use it the same way:
Calendar.autoupdatingCurrent.isDateInToday(yourDate)
Calendar
is a type alias for the NSCalendar
.
1
Omg yes! Just what I needed.
– Khoury
Apr 4 '18 at 5:55
add a comment |
Swift 3/4:
Calendar.current.isDateInToday(yourDate)
Calendar.current.isDateInYesterday(yourDate)
Calendar.current.isDateInTomorrow(yourDate)
Additionally:
Calendar.current.isDateInWeekend(yourDate)
Note that for some countries weekend may be different than Saturday-Sunday, it depends on the calendar.
You can also use autoupdatingCurrent
instead of current
calendar which will track user updates. You use it the same way:
Calendar.autoupdatingCurrent.isDateInToday(yourDate)
Calendar
is a type alias for the NSCalendar
.
Swift 3/4:
Calendar.current.isDateInToday(yourDate)
Calendar.current.isDateInYesterday(yourDate)
Calendar.current.isDateInTomorrow(yourDate)
Additionally:
Calendar.current.isDateInWeekend(yourDate)
Note that for some countries weekend may be different than Saturday-Sunday, it depends on the calendar.
You can also use autoupdatingCurrent
instead of current
calendar which will track user updates. You use it the same way:
Calendar.autoupdatingCurrent.isDateInToday(yourDate)
Calendar
is a type alias for the NSCalendar
.
edited Feb 12 at 11:40
answered Mar 21 '17 at 13:06


KlimczakMKlimczakM
6,69864674
6,69864674
1
Omg yes! Just what I needed.
– Khoury
Apr 4 '18 at 5:55
add a comment |
1
Omg yes! Just what I needed.
– Khoury
Apr 4 '18 at 5:55
1
1
Omg yes! Just what I needed.
– Khoury
Apr 4 '18 at 5:55
Omg yes! Just what I needed.
– Khoury
Apr 4 '18 at 5:55
add a comment |
Swift 4 update:
let calendar = Calendar.current
let date = Date()
calendar.isDateInYesterday(date)
calendar.isDateInToday(date)
calendar.isDateInTomorrow(date)
add a comment |
Swift 4 update:
let calendar = Calendar.current
let date = Date()
calendar.isDateInYesterday(date)
calendar.isDateInToday(date)
calendar.isDateInTomorrow(date)
add a comment |
Swift 4 update:
let calendar = Calendar.current
let date = Date()
calendar.isDateInYesterday(date)
calendar.isDateInToday(date)
calendar.isDateInTomorrow(date)
Swift 4 update:
let calendar = Calendar.current
let date = Date()
calendar.isDateInYesterday(date)
calendar.isDateInToday(date)
calendar.isDateInTomorrow(date)
answered Jan 25 '18 at 15:19
EdwardEdward
838723
838723
add a comment |
add a comment |
NSCalender has new methods that you can use directly.
NSCalendar.currentCalendar().isDateInTomorrow(NSDate())//Replace NSDate() with your date
NSCalendar.currentCalendar().isDateInYesterday()
NSCalendar.currentCalendar().isDateInTomorrow()
Hope this helps
add a comment |
NSCalender has new methods that you can use directly.
NSCalendar.currentCalendar().isDateInTomorrow(NSDate())//Replace NSDate() with your date
NSCalendar.currentCalendar().isDateInYesterday()
NSCalendar.currentCalendar().isDateInTomorrow()
Hope this helps
add a comment |
NSCalender has new methods that you can use directly.
NSCalendar.currentCalendar().isDateInTomorrow(NSDate())//Replace NSDate() with your date
NSCalendar.currentCalendar().isDateInYesterday()
NSCalendar.currentCalendar().isDateInTomorrow()
Hope this helps
NSCalender has new methods that you can use directly.
NSCalendar.currentCalendar().isDateInTomorrow(NSDate())//Replace NSDate() with your date
NSCalendar.currentCalendar().isDateInYesterday()
NSCalendar.currentCalendar().isDateInTomorrow()
Hope this helps
edited Oct 5 '18 at 7:33
answered Sep 6 '16 at 9:17
nishith Singhnishith Singh
1,594818
1,594818
add a comment |
add a comment |
1)According to your example you want to receive labels "Yesterday", "Today" and etc. iOS can do this by default:
https://developer.apple.com/documentation/foundation/nsdateformatter/1415848-doesrelativedateformatting?language=objc
2)If you want to compute your custom label when iOS don't add these labels by itself then alternatively you can use 2 DateFormatter
objects with both doesRelativeDateFormatting == true
and doesRelativeDateFormatting == false
and compare if their result date strings are the same or different
add a comment |
1)According to your example you want to receive labels "Yesterday", "Today" and etc. iOS can do this by default:
https://developer.apple.com/documentation/foundation/nsdateformatter/1415848-doesrelativedateformatting?language=objc
2)If you want to compute your custom label when iOS don't add these labels by itself then alternatively you can use 2 DateFormatter
objects with both doesRelativeDateFormatting == true
and doesRelativeDateFormatting == false
and compare if their result date strings are the same or different
add a comment |
1)According to your example you want to receive labels "Yesterday", "Today" and etc. iOS can do this by default:
https://developer.apple.com/documentation/foundation/nsdateformatter/1415848-doesrelativedateformatting?language=objc
2)If you want to compute your custom label when iOS don't add these labels by itself then alternatively you can use 2 DateFormatter
objects with both doesRelativeDateFormatting == true
and doesRelativeDateFormatting == false
and compare if their result date strings are the same or different
1)According to your example you want to receive labels "Yesterday", "Today" and etc. iOS can do this by default:
https://developer.apple.com/documentation/foundation/nsdateformatter/1415848-doesrelativedateformatting?language=objc
2)If you want to compute your custom label when iOS don't add these labels by itself then alternatively you can use 2 DateFormatter
objects with both doesRelativeDateFormatting == true
and doesRelativeDateFormatting == false
and compare if their result date strings are the same or different
answered Jan 3 at 9:06
Vyachaslav GerchicovVyachaslav Gerchicov
7181825
7181825
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f39345101%2fswift-check-if-a-timestamp-is-yesterday-today-tomorrow-or-x-days-ago%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hojXt,KQjGH,Gb2IYT1y1 eeO hGglmvXKxtDXf5MEMV