How to convert the extracted text from PDF to JSON or XML format in Python?
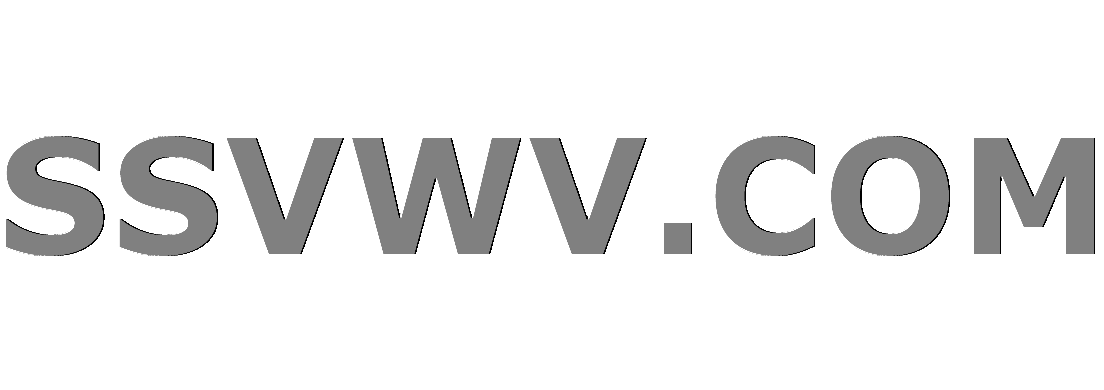
Multi tool use
I am using PyPDF2 to extract the data from PDF file and then converting into Text format?
PDF format for the file is like this:
Name : John
Address: 123street , USA
Phone No: 123456
Gender: Male
Name : Jim
Address: 456street , USA
Phone No: 456899
Gender: Male
In Python I am using this code:
import PyPDF2
pdf_file = open('C:\Users\Desktop\Sampletest.pdf', 'rb')
read_pdf = PyPDF2.PdfFileReader(pdf_file)
number_of_pages = read_pdf.getNumPages()
page = read_pdf.getPage(0)
page_content = page.extractText()
page_content
This is the outcome which I get from page_content:
'Name : John n nAddress: 123street , USA n nPhone No: 123456n nGender: Male n n nName : Jim n nAddress: 456street , USA n nPhone No: 456899n nGender: Male n n n'
How do I format it in a JSON or XML format so that I could use extracted data in SQL server database.
I tried using this approach as well
import json
data = json.dumps(page_content)
formatj = json.loads(data)
print (formatj)
Output:
Name : John
Address: 123street , USA
Phone No: 123456
Gender: Male
Name : Jim
Address: 456street , USA
Phone No: 456899
Gender: Male
This is the same output which I have in my word file, but I don't think that this is in JSON format.
python json xml pypdf2
add a comment |
I am using PyPDF2 to extract the data from PDF file and then converting into Text format?
PDF format for the file is like this:
Name : John
Address: 123street , USA
Phone No: 123456
Gender: Male
Name : Jim
Address: 456street , USA
Phone No: 456899
Gender: Male
In Python I am using this code:
import PyPDF2
pdf_file = open('C:\Users\Desktop\Sampletest.pdf', 'rb')
read_pdf = PyPDF2.PdfFileReader(pdf_file)
number_of_pages = read_pdf.getNumPages()
page = read_pdf.getPage(0)
page_content = page.extractText()
page_content
This is the outcome which I get from page_content:
'Name : John n nAddress: 123street , USA n nPhone No: 123456n nGender: Male n n nName : Jim n nAddress: 456street , USA n nPhone No: 456899n nGender: Male n n n'
How do I format it in a JSON or XML format so that I could use extracted data in SQL server database.
I tried using this approach as well
import json
data = json.dumps(page_content)
formatj = json.loads(data)
print (formatj)
Output:
Name : John
Address: 123street , USA
Phone No: 123456
Gender: Male
Name : Jim
Address: 456street , USA
Phone No: 456899
Gender: Male
This is the same output which I have in my word file, but I don't think that this is in JSON format.
python json xml pypdf2
Where are you running this code? In the REPL? Have you triedprint(page_content)
instead? Having one variable on a line by itself doesn't produce any output in a script
– cricket_007
Oct 6 '18 at 3:57
What have you tried so far?
– Klaus D.
Oct 6 '18 at 3:59
add a comment |
I am using PyPDF2 to extract the data from PDF file and then converting into Text format?
PDF format for the file is like this:
Name : John
Address: 123street , USA
Phone No: 123456
Gender: Male
Name : Jim
Address: 456street , USA
Phone No: 456899
Gender: Male
In Python I am using this code:
import PyPDF2
pdf_file = open('C:\Users\Desktop\Sampletest.pdf', 'rb')
read_pdf = PyPDF2.PdfFileReader(pdf_file)
number_of_pages = read_pdf.getNumPages()
page = read_pdf.getPage(0)
page_content = page.extractText()
page_content
This is the outcome which I get from page_content:
'Name : John n nAddress: 123street , USA n nPhone No: 123456n nGender: Male n n nName : Jim n nAddress: 456street , USA n nPhone No: 456899n nGender: Male n n n'
How do I format it in a JSON or XML format so that I could use extracted data in SQL server database.
I tried using this approach as well
import json
data = json.dumps(page_content)
formatj = json.loads(data)
print (formatj)
Output:
Name : John
Address: 123street , USA
Phone No: 123456
Gender: Male
Name : Jim
Address: 456street , USA
Phone No: 456899
Gender: Male
This is the same output which I have in my word file, but I don't think that this is in JSON format.
python json xml pypdf2
I am using PyPDF2 to extract the data from PDF file and then converting into Text format?
PDF format for the file is like this:
Name : John
Address: 123street , USA
Phone No: 123456
Gender: Male
Name : Jim
Address: 456street , USA
Phone No: 456899
Gender: Male
In Python I am using this code:
import PyPDF2
pdf_file = open('C:\Users\Desktop\Sampletest.pdf', 'rb')
read_pdf = PyPDF2.PdfFileReader(pdf_file)
number_of_pages = read_pdf.getNumPages()
page = read_pdf.getPage(0)
page_content = page.extractText()
page_content
This is the outcome which I get from page_content:
'Name : John n nAddress: 123street , USA n nPhone No: 123456n nGender: Male n n nName : Jim n nAddress: 456street , USA n nPhone No: 456899n nGender: Male n n n'
How do I format it in a JSON or XML format so that I could use extracted data in SQL server database.
I tried using this approach as well
import json
data = json.dumps(page_content)
formatj = json.loads(data)
print (formatj)
Output:
Name : John
Address: 123street , USA
Phone No: 123456
Gender: Male
Name : Jim
Address: 456street , USA
Phone No: 456899
Gender: Male
This is the same output which I have in my word file, but I don't think that this is in JSON format.
python json xml pypdf2
python json xml pypdf2
edited Oct 6 '18 at 5:29
Avi
asked Oct 6 '18 at 3:52
AviAvi
924314
924314
Where are you running this code? In the REPL? Have you triedprint(page_content)
instead? Having one variable on a line by itself doesn't produce any output in a script
– cricket_007
Oct 6 '18 at 3:57
What have you tried so far?
– Klaus D.
Oct 6 '18 at 3:59
add a comment |
Where are you running this code? In the REPL? Have you triedprint(page_content)
instead? Having one variable on a line by itself doesn't produce any output in a script
– cricket_007
Oct 6 '18 at 3:57
What have you tried so far?
– Klaus D.
Oct 6 '18 at 3:59
Where are you running this code? In the REPL? Have you tried
print(page_content)
instead? Having one variable on a line by itself doesn't produce any output in a script– cricket_007
Oct 6 '18 at 3:57
Where are you running this code? In the REPL? Have you tried
print(page_content)
instead? Having one variable on a line by itself doesn't produce any output in a script– cricket_007
Oct 6 '18 at 3:57
What have you tried so far?
– Klaus D.
Oct 6 '18 at 3:59
What have you tried so far?
– Klaus D.
Oct 6 '18 at 3:59
add a comment |
1 Answer
1
active
oldest
votes
Not so pretty, but this would get the job done, I think. You would get a dictionary which then gets printed by the json parser in a nice, pretty format.
import json
def get_data(page_content):
_dict = {}
page_content_list = page_content.splitlines()
for line in page_content_list:
if ':' not in line:
continue
key, value = line.split(':')
_dict[key.strip()] = value.strip()
return _dict
page_data = get_data(page_content)
json_data = json.dumps(page_data, indent=4)
print(json_data)
or, instead of those last 3 lines, just do this:
print(json.dumps(get_data(page_content), indent=4))
Thanks @UtahJarHead for the answer but it seems like I can only extract only one of the people information. In my case, I am getting this output. { "Name": "Jim", "Address": "456street , USA", "Phone No": "456899", "Gender": "Male" }
– Avi
Oct 6 '18 at 14:58
Right. The function I created, you are going to have to pick out each individual section. For instance, each time you see"Name:"
, you grab what's been gathered and shove it to theget_data()
function, process it, and head over to get the next person's data.
– UtahJarhead
Oct 6 '18 at 18:21
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52675556%2fhow-to-convert-the-extracted-text-from-pdf-to-json-or-xml-format-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Not so pretty, but this would get the job done, I think. You would get a dictionary which then gets printed by the json parser in a nice, pretty format.
import json
def get_data(page_content):
_dict = {}
page_content_list = page_content.splitlines()
for line in page_content_list:
if ':' not in line:
continue
key, value = line.split(':')
_dict[key.strip()] = value.strip()
return _dict
page_data = get_data(page_content)
json_data = json.dumps(page_data, indent=4)
print(json_data)
or, instead of those last 3 lines, just do this:
print(json.dumps(get_data(page_content), indent=4))
Thanks @UtahJarHead for the answer but it seems like I can only extract only one of the people information. In my case, I am getting this output. { "Name": "Jim", "Address": "456street , USA", "Phone No": "456899", "Gender": "Male" }
– Avi
Oct 6 '18 at 14:58
Right. The function I created, you are going to have to pick out each individual section. For instance, each time you see"Name:"
, you grab what's been gathered and shove it to theget_data()
function, process it, and head over to get the next person's data.
– UtahJarhead
Oct 6 '18 at 18:21
add a comment |
Not so pretty, but this would get the job done, I think. You would get a dictionary which then gets printed by the json parser in a nice, pretty format.
import json
def get_data(page_content):
_dict = {}
page_content_list = page_content.splitlines()
for line in page_content_list:
if ':' not in line:
continue
key, value = line.split(':')
_dict[key.strip()] = value.strip()
return _dict
page_data = get_data(page_content)
json_data = json.dumps(page_data, indent=4)
print(json_data)
or, instead of those last 3 lines, just do this:
print(json.dumps(get_data(page_content), indent=4))
Thanks @UtahJarHead for the answer but it seems like I can only extract only one of the people information. In my case, I am getting this output. { "Name": "Jim", "Address": "456street , USA", "Phone No": "456899", "Gender": "Male" }
– Avi
Oct 6 '18 at 14:58
Right. The function I created, you are going to have to pick out each individual section. For instance, each time you see"Name:"
, you grab what's been gathered and shove it to theget_data()
function, process it, and head over to get the next person's data.
– UtahJarhead
Oct 6 '18 at 18:21
add a comment |
Not so pretty, but this would get the job done, I think. You would get a dictionary which then gets printed by the json parser in a nice, pretty format.
import json
def get_data(page_content):
_dict = {}
page_content_list = page_content.splitlines()
for line in page_content_list:
if ':' not in line:
continue
key, value = line.split(':')
_dict[key.strip()] = value.strip()
return _dict
page_data = get_data(page_content)
json_data = json.dumps(page_data, indent=4)
print(json_data)
or, instead of those last 3 lines, just do this:
print(json.dumps(get_data(page_content), indent=4))
Not so pretty, but this would get the job done, I think. You would get a dictionary which then gets printed by the json parser in a nice, pretty format.
import json
def get_data(page_content):
_dict = {}
page_content_list = page_content.splitlines()
for line in page_content_list:
if ':' not in line:
continue
key, value = line.split(':')
_dict[key.strip()] = value.strip()
return _dict
page_data = get_data(page_content)
json_data = json.dumps(page_data, indent=4)
print(json_data)
or, instead of those last 3 lines, just do this:
print(json.dumps(get_data(page_content), indent=4))
answered Oct 6 '18 at 6:48
UtahJarheadUtahJarhead
1,129717
1,129717
Thanks @UtahJarHead for the answer but it seems like I can only extract only one of the people information. In my case, I am getting this output. { "Name": "Jim", "Address": "456street , USA", "Phone No": "456899", "Gender": "Male" }
– Avi
Oct 6 '18 at 14:58
Right. The function I created, you are going to have to pick out each individual section. For instance, each time you see"Name:"
, you grab what's been gathered and shove it to theget_data()
function, process it, and head over to get the next person's data.
– UtahJarhead
Oct 6 '18 at 18:21
add a comment |
Thanks @UtahJarHead for the answer but it seems like I can only extract only one of the people information. In my case, I am getting this output. { "Name": "Jim", "Address": "456street , USA", "Phone No": "456899", "Gender": "Male" }
– Avi
Oct 6 '18 at 14:58
Right. The function I created, you are going to have to pick out each individual section. For instance, each time you see"Name:"
, you grab what's been gathered and shove it to theget_data()
function, process it, and head over to get the next person's data.
– UtahJarhead
Oct 6 '18 at 18:21
Thanks @UtahJarHead for the answer but it seems like I can only extract only one of the people information. In my case, I am getting this output. { "Name": "Jim", "Address": "456street , USA", "Phone No": "456899", "Gender": "Male" }
– Avi
Oct 6 '18 at 14:58
Thanks @UtahJarHead for the answer but it seems like I can only extract only one of the people information. In my case, I am getting this output. { "Name": "Jim", "Address": "456street , USA", "Phone No": "456899", "Gender": "Male" }
– Avi
Oct 6 '18 at 14:58
Right. The function I created, you are going to have to pick out each individual section. For instance, each time you see
"Name:"
, you grab what's been gathered and shove it to the get_data()
function, process it, and head over to get the next person's data.– UtahJarhead
Oct 6 '18 at 18:21
Right. The function I created, you are going to have to pick out each individual section. For instance, each time you see
"Name:"
, you grab what's been gathered and shove it to the get_data()
function, process it, and head over to get the next person's data.– UtahJarhead
Oct 6 '18 at 18:21
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52675556%2fhow-to-convert-the-extracted-text-from-pdf-to-json-or-xml-format-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ku9BtwbJspiAnYeqDRs6J,sI1tj 5quFyKSNk2,fxtM,hYWhh
Where are you running this code? In the REPL? Have you tried
print(page_content)
instead? Having one variable on a line by itself doesn't produce any output in a script– cricket_007
Oct 6 '18 at 3:57
What have you tried so far?
– Klaus D.
Oct 6 '18 at 3:59