How to use RESTful with Basic Authentication in Spring Boot
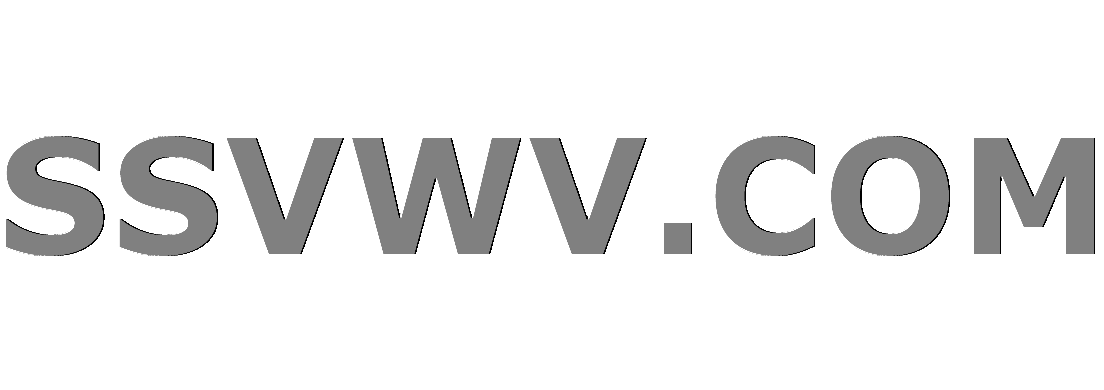
Multi tool use
Helllo, I'm using RESTful with basic authentication and this code is a part from the RestController:
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
}
public Long getUserIdFromUsername(String username) {
User user = userJpaRepository.findByUsername(username);
userId = user.getId();
return userId;
}
And I have a problem, for example I'm using Postman to retrieve the goals for a speciffic user like this:
http://localhost:8080/jpa/users/john/goals with GET request
Then I use the basic authentication for the username john and the password for this username and I receive the goals for john.
After that if I do a GET request for this link http://localhost:8080/jpa/users/tom/goals I receive the goals for tom, but I'm logged in with john at this moment of time, so john can see his goals and also he can see tom's goals.
The question is how can I access the login username in the RestController, because I want to do something like this:
if (loginUsername == username) {
return goalJpaRepository.findByUserId(userId);
}
return "Access denied!";
So I want to know if it is possible to access the login username from HTTP Header?
Thank you!
UPDATE - Yes the framework is Spring Boot, also I'm using Spring Security with Dao Authentication because I want to get the user from a MySQL database. Anyway I'm not an expert at Spring Security.
Now I understand how to use Principal in my controller methods, but I don't know how to use Spring Security for this specific case. How should I implement it? For example the user john should see and modify only his goals.
Spring Security Configuration:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpMethod;
import org.springframework.security.authentication.dao.DaoAuthenticationProvider;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import com.dgs.restful.webservices.goaltrackerservice.user.MyUserDetailsService;
@Configuration
@EnableWebSecurity
public class SpringSecurityConfigurationBasicAuth extends WebSecurityConfigurerAdapter {
@Bean
public BCryptPasswordEncoder bCryptPasswordEncoder() {
return new BCryptPasswordEncoder();
}
@Autowired
private MyUserDetailsService userDetailsService;
@Bean
public DaoAuthenticationProvider authenticationProvider() {
DaoAuthenticationProvider authProvider
= new DaoAuthenticationProvider();
authProvider.setUserDetailsService(userDetailsService);
authProvider.setPasswordEncoder(bCryptPasswordEncoder());
return authProvider;
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.antMatchers("/allusers").permitAll()
.anyRequest().authenticated()
.and()
// .formLogin().and()
.httpBasic();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authenticationProvider());
}
}
java rest basic-authentication restful-authentication spring-rest
add a comment |
Helllo, I'm using RESTful with basic authentication and this code is a part from the RestController:
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
}
public Long getUserIdFromUsername(String username) {
User user = userJpaRepository.findByUsername(username);
userId = user.getId();
return userId;
}
And I have a problem, for example I'm using Postman to retrieve the goals for a speciffic user like this:
http://localhost:8080/jpa/users/john/goals with GET request
Then I use the basic authentication for the username john and the password for this username and I receive the goals for john.
After that if I do a GET request for this link http://localhost:8080/jpa/users/tom/goals I receive the goals for tom, but I'm logged in with john at this moment of time, so john can see his goals and also he can see tom's goals.
The question is how can I access the login username in the RestController, because I want to do something like this:
if (loginUsername == username) {
return goalJpaRepository.findByUserId(userId);
}
return "Access denied!";
So I want to know if it is possible to access the login username from HTTP Header?
Thank you!
UPDATE - Yes the framework is Spring Boot, also I'm using Spring Security with Dao Authentication because I want to get the user from a MySQL database. Anyway I'm not an expert at Spring Security.
Now I understand how to use Principal in my controller methods, but I don't know how to use Spring Security for this specific case. How should I implement it? For example the user john should see and modify only his goals.
Spring Security Configuration:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpMethod;
import org.springframework.security.authentication.dao.DaoAuthenticationProvider;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import com.dgs.restful.webservices.goaltrackerservice.user.MyUserDetailsService;
@Configuration
@EnableWebSecurity
public class SpringSecurityConfigurationBasicAuth extends WebSecurityConfigurerAdapter {
@Bean
public BCryptPasswordEncoder bCryptPasswordEncoder() {
return new BCryptPasswordEncoder();
}
@Autowired
private MyUserDetailsService userDetailsService;
@Bean
public DaoAuthenticationProvider authenticationProvider() {
DaoAuthenticationProvider authProvider
= new DaoAuthenticationProvider();
authProvider.setUserDetailsService(userDetailsService);
authProvider.setPasswordEncoder(bCryptPasswordEncoder());
return authProvider;
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.antMatchers("/allusers").permitAll()
.anyRequest().authenticated()
.and()
// .formLogin().and()
.httpBasic();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authenticationProvider());
}
}
java rest basic-authentication restful-authentication spring-rest
4
are you using spring? specifically spring-security?
– Yogendra Mishra
Jan 3 at 9:04
3
Sidenote, what is the data-type ofloginUsername
? If its String, then you are comparing it wrong.
– Nicholas K
Jan 3 at 9:07
2
It depends which framework you are using (if you are using any). You're talking aboutRestController
so I'm guessing you're using Spring (you should definitely add the appropriate tags to your question). If so, have a look at Spring security which allow you to configure how to secure your app.
– Matt
Jan 3 at 9:11
add a comment |
Helllo, I'm using RESTful with basic authentication and this code is a part from the RestController:
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
}
public Long getUserIdFromUsername(String username) {
User user = userJpaRepository.findByUsername(username);
userId = user.getId();
return userId;
}
And I have a problem, for example I'm using Postman to retrieve the goals for a speciffic user like this:
http://localhost:8080/jpa/users/john/goals with GET request
Then I use the basic authentication for the username john and the password for this username and I receive the goals for john.
After that if I do a GET request for this link http://localhost:8080/jpa/users/tom/goals I receive the goals for tom, but I'm logged in with john at this moment of time, so john can see his goals and also he can see tom's goals.
The question is how can I access the login username in the RestController, because I want to do something like this:
if (loginUsername == username) {
return goalJpaRepository.findByUserId(userId);
}
return "Access denied!";
So I want to know if it is possible to access the login username from HTTP Header?
Thank you!
UPDATE - Yes the framework is Spring Boot, also I'm using Spring Security with Dao Authentication because I want to get the user from a MySQL database. Anyway I'm not an expert at Spring Security.
Now I understand how to use Principal in my controller methods, but I don't know how to use Spring Security for this specific case. How should I implement it? For example the user john should see and modify only his goals.
Spring Security Configuration:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpMethod;
import org.springframework.security.authentication.dao.DaoAuthenticationProvider;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import com.dgs.restful.webservices.goaltrackerservice.user.MyUserDetailsService;
@Configuration
@EnableWebSecurity
public class SpringSecurityConfigurationBasicAuth extends WebSecurityConfigurerAdapter {
@Bean
public BCryptPasswordEncoder bCryptPasswordEncoder() {
return new BCryptPasswordEncoder();
}
@Autowired
private MyUserDetailsService userDetailsService;
@Bean
public DaoAuthenticationProvider authenticationProvider() {
DaoAuthenticationProvider authProvider
= new DaoAuthenticationProvider();
authProvider.setUserDetailsService(userDetailsService);
authProvider.setPasswordEncoder(bCryptPasswordEncoder());
return authProvider;
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.antMatchers("/allusers").permitAll()
.anyRequest().authenticated()
.and()
// .formLogin().and()
.httpBasic();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authenticationProvider());
}
}
java rest basic-authentication restful-authentication spring-rest
Helllo, I'm using RESTful with basic authentication and this code is a part from the RestController:
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
}
public Long getUserIdFromUsername(String username) {
User user = userJpaRepository.findByUsername(username);
userId = user.getId();
return userId;
}
And I have a problem, for example I'm using Postman to retrieve the goals for a speciffic user like this:
http://localhost:8080/jpa/users/john/goals with GET request
Then I use the basic authentication for the username john and the password for this username and I receive the goals for john.
After that if I do a GET request for this link http://localhost:8080/jpa/users/tom/goals I receive the goals for tom, but I'm logged in with john at this moment of time, so john can see his goals and also he can see tom's goals.
The question is how can I access the login username in the RestController, because I want to do something like this:
if (loginUsername == username) {
return goalJpaRepository.findByUserId(userId);
}
return "Access denied!";
So I want to know if it is possible to access the login username from HTTP Header?
Thank you!
UPDATE - Yes the framework is Spring Boot, also I'm using Spring Security with Dao Authentication because I want to get the user from a MySQL database. Anyway I'm not an expert at Spring Security.
Now I understand how to use Principal in my controller methods, but I don't know how to use Spring Security for this specific case. How should I implement it? For example the user john should see and modify only his goals.
Spring Security Configuration:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpMethod;
import org.springframework.security.authentication.dao.DaoAuthenticationProvider;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import com.dgs.restful.webservices.goaltrackerservice.user.MyUserDetailsService;
@Configuration
@EnableWebSecurity
public class SpringSecurityConfigurationBasicAuth extends WebSecurityConfigurerAdapter {
@Bean
public BCryptPasswordEncoder bCryptPasswordEncoder() {
return new BCryptPasswordEncoder();
}
@Autowired
private MyUserDetailsService userDetailsService;
@Bean
public DaoAuthenticationProvider authenticationProvider() {
DaoAuthenticationProvider authProvider
= new DaoAuthenticationProvider();
authProvider.setUserDetailsService(userDetailsService);
authProvider.setPasswordEncoder(bCryptPasswordEncoder());
return authProvider;
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers(HttpMethod.OPTIONS, "/**").permitAll()
.antMatchers("/allusers").permitAll()
.anyRequest().authenticated()
.and()
// .formLogin().and()
.httpBasic();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authenticationProvider());
}
}
java rest basic-authentication restful-authentication spring-rest
java rest basic-authentication restful-authentication spring-rest
edited Jan 3 at 11:50
elvis
asked Jan 3 at 9:00
elviselvis
501714
501714
4
are you using spring? specifically spring-security?
– Yogendra Mishra
Jan 3 at 9:04
3
Sidenote, what is the data-type ofloginUsername
? If its String, then you are comparing it wrong.
– Nicholas K
Jan 3 at 9:07
2
It depends which framework you are using (if you are using any). You're talking aboutRestController
so I'm guessing you're using Spring (you should definitely add the appropriate tags to your question). If so, have a look at Spring security which allow you to configure how to secure your app.
– Matt
Jan 3 at 9:11
add a comment |
4
are you using spring? specifically spring-security?
– Yogendra Mishra
Jan 3 at 9:04
3
Sidenote, what is the data-type ofloginUsername
? If its String, then you are comparing it wrong.
– Nicholas K
Jan 3 at 9:07
2
It depends which framework you are using (if you are using any). You're talking aboutRestController
so I'm guessing you're using Spring (you should definitely add the appropriate tags to your question). If so, have a look at Spring security which allow you to configure how to secure your app.
– Matt
Jan 3 at 9:11
4
4
are you using spring? specifically spring-security?
– Yogendra Mishra
Jan 3 at 9:04
are you using spring? specifically spring-security?
– Yogendra Mishra
Jan 3 at 9:04
3
3
Sidenote, what is the data-type of
loginUsername
? If its String, then you are comparing it wrong.– Nicholas K
Jan 3 at 9:07
Sidenote, what is the data-type of
loginUsername
? If its String, then you are comparing it wrong.– Nicholas K
Jan 3 at 9:07
2
2
It depends which framework you are using (if you are using any). You're talking about
RestController
so I'm guessing you're using Spring (you should definitely add the appropriate tags to your question). If so, have a look at Spring security which allow you to configure how to secure your app.– Matt
Jan 3 at 9:11
It depends which framework you are using (if you are using any). You're talking about
RestController
so I'm guessing you're using Spring (you should definitely add the appropriate tags to your question). If so, have a look at Spring security which allow you to configure how to secure your app.– Matt
Jan 3 at 9:11
add a comment |
5 Answers
5
active
oldest
votes
Please note that you are not doing any security at this time.
As said by @Matt "It depends which framework you are using". But I guess you are using spring. You should then have a look at the spring-securuty module documentation.
Basically you can inject the authenticated user into your method parameter :
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username, Principal principal) {
if ( username.equals(principal.getName()) ) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
} else {
throw new SomeExceptionThatWillBeMapped();
}
}
But spring-security and many frameworks provide better patterns to manage the security.
Thanks for reply! I just added this and it works perfectly. I already use spring security with dao authentication, but I don't know how to use spring security for this specific case. I just updated the question if you want to have a look.
– elvis
Jan 3 at 15:38
You should have considered to create a new question for that and accept one of the answers for this one.
– gervais.b
Jan 4 at 12:45
add a comment |
You can also solve this problem with @PreAuthorize
, an annotation offered by the Spring Security Framework, which uses the Spring Expression Language.
@PreAuthorize("principal.name == #username")
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
return goalJpaRepository.findByUserId(userId);
}
Behind the scenes Spring will use the already mentioned SecurityContextHolder
to fetch the currently authenticated principal. If the expression resolves to false, the response code 403
will be returned.
Please note that you have to enable global method security:
@Configuration
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class MethodSecurityConfig extends GlobalMethodSecurityConfiguration {
}
add a comment |
Assuming you are using Spring as your Java framework, you should use Spring security to configure the basic authentication. Many tutorials available online (https://www.baeldung.com/spring-security-basic-authentication,
Spring Security will then provide a security context available throughout the app (SecurityContextHolder.getContext()
) from which you can retrieve the connected user information (username, ...).
For instance to retrieve the username of the connected user, you should do :
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
String loginUsername = authentication.getName();
Alternatively, as mentioned by @gervais.b Spring can inject the Principal
(or Authentication
) in your controller methods.
As said by @Glains An even better alternative is to use the @PreAuthorize
and @PostAuthorize
annotations, which alows you to define simple rules based on Spring Expression Language.
Thank you Matt for your help, I just updated my question. I'm using Spring Boot and also I'm using Spring Security with Dao Authentication because I want to get the user from the database. Now I understand how to use Principal in the controller methods to achieve what I want, but I don't know how to use Spring Security for this, what should I add in the spring security configuration file?
– elvis
Jan 3 at 11:48
Spring Security can help you for general role based rules, like endpoint "/users/*" is only accessible to connected user (or user with role X). And as @Glains mentioned in his answer, it also allows you to define simple rules with annotations such as@PreAuthorize
with Spring EL expressions.
– Matt
Jan 3 at 14:22
add a comment |
To answer your new question on "Dao Authentication" the answer is to provide a custom UserDetailsService
.
From the configuration that you have attached to your question it looks that you already have a MyUserDetailsService
.
There are plenty of articles that explain how to use a custom DetailsService
. This one seems to match your requirements : https://www.baeldung.com/spring-security-authentication-with-a-database
Edit: On how to be sure that only John can see John's items.
Basically, the only ting that you can do to esnure that only John can see his goals it to restrict the goals to only those owned by John. But there is plenty way of doing this.
As you suggest in your initial question, you can just select the goals for a specific user. The power with spring-security is that it can inject a
Principal
but also kind of orher authentication object.You can also make that more implicit an filter a the DAO/Repository side by using the
SecurityContextHolder
. This approach is fine and looks better when your system is more user centric or like a multi-tenant system.Using some specific @Annotations or Aspects would also be a solution but maybe less obvious in this case.
Thank you but the problem wasn't about "Dao Authentication", I have implemented this... it is the old problem, when I'm using Postman, I'm logged in with john and I can retrieve john's goals and also any other user's goals, I want to allow retrieving only john's goals when I'm logged in with john... you said that I ca inject the authenticated user into my method parameter, and I did this and works perfectly... also you said that spring-security is better than this, and I want to know how can I use spring-security for this situation.
– elvis
Jan 4 at 16:46
I said that spring-security is a good security framework. And, since you are suring spring, it is the defacto choice. I will edit this answer to explain your alternatives.
– gervais.b
Jan 7 at 6:58
add a comment |
Answer is already given on before answers. I just want to make an addition, take a look at this article. You can easily implement spring security with json web token:
https://auth0.com/blog/implementing-jwt-authentication-on-spring-boot/
OP is specifically asking for basic auth, not JWT.
– Matt
Jan 3 at 9:52
@Ali, I'll try to implement JWT in my app, and I just read this article, but I don't understand why they don't use Autowired annotation while they use Spring Boot
– elvis
Jan 9 at 15:32
In Spring, you don't have to use Autowired annotation while you are autowiring somtehings. Because there is three ways of Autowiring. Annotation based, setter based, constructor based. They've used constructor based way. @elvis
– Ali Kaan Bacı
Jan 10 at 16:13
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54019074%2fhow-to-use-restful-with-basic-authentication-in-spring-boot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Please note that you are not doing any security at this time.
As said by @Matt "It depends which framework you are using". But I guess you are using spring. You should then have a look at the spring-securuty module documentation.
Basically you can inject the authenticated user into your method parameter :
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username, Principal principal) {
if ( username.equals(principal.getName()) ) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
} else {
throw new SomeExceptionThatWillBeMapped();
}
}
But spring-security and many frameworks provide better patterns to manage the security.
Thanks for reply! I just added this and it works perfectly. I already use spring security with dao authentication, but I don't know how to use spring security for this specific case. I just updated the question if you want to have a look.
– elvis
Jan 3 at 15:38
You should have considered to create a new question for that and accept one of the answers for this one.
– gervais.b
Jan 4 at 12:45
add a comment |
Please note that you are not doing any security at this time.
As said by @Matt "It depends which framework you are using". But I guess you are using spring. You should then have a look at the spring-securuty module documentation.
Basically you can inject the authenticated user into your method parameter :
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username, Principal principal) {
if ( username.equals(principal.getName()) ) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
} else {
throw new SomeExceptionThatWillBeMapped();
}
}
But spring-security and many frameworks provide better patterns to manage the security.
Thanks for reply! I just added this and it works perfectly. I already use spring security with dao authentication, but I don't know how to use spring security for this specific case. I just updated the question if you want to have a look.
– elvis
Jan 3 at 15:38
You should have considered to create a new question for that and accept one of the answers for this one.
– gervais.b
Jan 4 at 12:45
add a comment |
Please note that you are not doing any security at this time.
As said by @Matt "It depends which framework you are using". But I guess you are using spring. You should then have a look at the spring-securuty module documentation.
Basically you can inject the authenticated user into your method parameter :
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username, Principal principal) {
if ( username.equals(principal.getName()) ) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
} else {
throw new SomeExceptionThatWillBeMapped();
}
}
But spring-security and many frameworks provide better patterns to manage the security.
Please note that you are not doing any security at this time.
As said by @Matt "It depends which framework you are using". But I guess you are using spring. You should then have a look at the spring-securuty module documentation.
Basically you can inject the authenticated user into your method parameter :
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username, Principal principal) {
if ( username.equals(principal.getName()) ) {
userId = getUserIdFromUsername(username);
return goalJpaRepository.findByUserId(userId);
} else {
throw new SomeExceptionThatWillBeMapped();
}
}
But spring-security and many frameworks provide better patterns to manage the security.
answered Jan 3 at 9:31
gervais.bgervais.b
867427
867427
Thanks for reply! I just added this and it works perfectly. I already use spring security with dao authentication, but I don't know how to use spring security for this specific case. I just updated the question if you want to have a look.
– elvis
Jan 3 at 15:38
You should have considered to create a new question for that and accept one of the answers for this one.
– gervais.b
Jan 4 at 12:45
add a comment |
Thanks for reply! I just added this and it works perfectly. I already use spring security with dao authentication, but I don't know how to use spring security for this specific case. I just updated the question if you want to have a look.
– elvis
Jan 3 at 15:38
You should have considered to create a new question for that and accept one of the answers for this one.
– gervais.b
Jan 4 at 12:45
Thanks for reply! I just added this and it works perfectly. I already use spring security with dao authentication, but I don't know how to use spring security for this specific case. I just updated the question if you want to have a look.
– elvis
Jan 3 at 15:38
Thanks for reply! I just added this and it works perfectly. I already use spring security with dao authentication, but I don't know how to use spring security for this specific case. I just updated the question if you want to have a look.
– elvis
Jan 3 at 15:38
You should have considered to create a new question for that and accept one of the answers for this one.
– gervais.b
Jan 4 at 12:45
You should have considered to create a new question for that and accept one of the answers for this one.
– gervais.b
Jan 4 at 12:45
add a comment |
You can also solve this problem with @PreAuthorize
, an annotation offered by the Spring Security Framework, which uses the Spring Expression Language.
@PreAuthorize("principal.name == #username")
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
return goalJpaRepository.findByUserId(userId);
}
Behind the scenes Spring will use the already mentioned SecurityContextHolder
to fetch the currently authenticated principal. If the expression resolves to false, the response code 403
will be returned.
Please note that you have to enable global method security:
@Configuration
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class MethodSecurityConfig extends GlobalMethodSecurityConfiguration {
}
add a comment |
You can also solve this problem with @PreAuthorize
, an annotation offered by the Spring Security Framework, which uses the Spring Expression Language.
@PreAuthorize("principal.name == #username")
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
return goalJpaRepository.findByUserId(userId);
}
Behind the scenes Spring will use the already mentioned SecurityContextHolder
to fetch the currently authenticated principal. If the expression resolves to false, the response code 403
will be returned.
Please note that you have to enable global method security:
@Configuration
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class MethodSecurityConfig extends GlobalMethodSecurityConfiguration {
}
add a comment |
You can also solve this problem with @PreAuthorize
, an annotation offered by the Spring Security Framework, which uses the Spring Expression Language.
@PreAuthorize("principal.name == #username")
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
return goalJpaRepository.findByUserId(userId);
}
Behind the scenes Spring will use the already mentioned SecurityContextHolder
to fetch the currently authenticated principal. If the expression resolves to false, the response code 403
will be returned.
Please note that you have to enable global method security:
@Configuration
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class MethodSecurityConfig extends GlobalMethodSecurityConfiguration {
}
You can also solve this problem with @PreAuthorize
, an annotation offered by the Spring Security Framework, which uses the Spring Expression Language.
@PreAuthorize("principal.name == #username")
@GetMapping("/jpa/users/{username}/goals")
public List<Goal> getAllGoals(@PathVariable String username) {
return goalJpaRepository.findByUserId(userId);
}
Behind the scenes Spring will use the already mentioned SecurityContextHolder
to fetch the currently authenticated principal. If the expression resolves to false, the response code 403
will be returned.
Please note that you have to enable global method security:
@Configuration
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class MethodSecurityConfig extends GlobalMethodSecurityConfiguration {
}
answered Jan 3 at 12:15
GlainsGlains
1,081719
1,081719
add a comment |
add a comment |
Assuming you are using Spring as your Java framework, you should use Spring security to configure the basic authentication. Many tutorials available online (https://www.baeldung.com/spring-security-basic-authentication,
Spring Security will then provide a security context available throughout the app (SecurityContextHolder.getContext()
) from which you can retrieve the connected user information (username, ...).
For instance to retrieve the username of the connected user, you should do :
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
String loginUsername = authentication.getName();
Alternatively, as mentioned by @gervais.b Spring can inject the Principal
(or Authentication
) in your controller methods.
As said by @Glains An even better alternative is to use the @PreAuthorize
and @PostAuthorize
annotations, which alows you to define simple rules based on Spring Expression Language.
Thank you Matt for your help, I just updated my question. I'm using Spring Boot and also I'm using Spring Security with Dao Authentication because I want to get the user from the database. Now I understand how to use Principal in the controller methods to achieve what I want, but I don't know how to use Spring Security for this, what should I add in the spring security configuration file?
– elvis
Jan 3 at 11:48
Spring Security can help you for general role based rules, like endpoint "/users/*" is only accessible to connected user (or user with role X). And as @Glains mentioned in his answer, it also allows you to define simple rules with annotations such as@PreAuthorize
with Spring EL expressions.
– Matt
Jan 3 at 14:22
add a comment |
Assuming you are using Spring as your Java framework, you should use Spring security to configure the basic authentication. Many tutorials available online (https://www.baeldung.com/spring-security-basic-authentication,
Spring Security will then provide a security context available throughout the app (SecurityContextHolder.getContext()
) from which you can retrieve the connected user information (username, ...).
For instance to retrieve the username of the connected user, you should do :
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
String loginUsername = authentication.getName();
Alternatively, as mentioned by @gervais.b Spring can inject the Principal
(or Authentication
) in your controller methods.
As said by @Glains An even better alternative is to use the @PreAuthorize
and @PostAuthorize
annotations, which alows you to define simple rules based on Spring Expression Language.
Thank you Matt for your help, I just updated my question. I'm using Spring Boot and also I'm using Spring Security with Dao Authentication because I want to get the user from the database. Now I understand how to use Principal in the controller methods to achieve what I want, but I don't know how to use Spring Security for this, what should I add in the spring security configuration file?
– elvis
Jan 3 at 11:48
Spring Security can help you for general role based rules, like endpoint "/users/*" is only accessible to connected user (or user with role X). And as @Glains mentioned in his answer, it also allows you to define simple rules with annotations such as@PreAuthorize
with Spring EL expressions.
– Matt
Jan 3 at 14:22
add a comment |
Assuming you are using Spring as your Java framework, you should use Spring security to configure the basic authentication. Many tutorials available online (https://www.baeldung.com/spring-security-basic-authentication,
Spring Security will then provide a security context available throughout the app (SecurityContextHolder.getContext()
) from which you can retrieve the connected user information (username, ...).
For instance to retrieve the username of the connected user, you should do :
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
String loginUsername = authentication.getName();
Alternatively, as mentioned by @gervais.b Spring can inject the Principal
(or Authentication
) in your controller methods.
As said by @Glains An even better alternative is to use the @PreAuthorize
and @PostAuthorize
annotations, which alows you to define simple rules based on Spring Expression Language.
Assuming you are using Spring as your Java framework, you should use Spring security to configure the basic authentication. Many tutorials available online (https://www.baeldung.com/spring-security-basic-authentication,
Spring Security will then provide a security context available throughout the app (SecurityContextHolder.getContext()
) from which you can retrieve the connected user information (username, ...).
For instance to retrieve the username of the connected user, you should do :
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
String loginUsername = authentication.getName();
Alternatively, as mentioned by @gervais.b Spring can inject the Principal
(or Authentication
) in your controller methods.
As said by @Glains An even better alternative is to use the @PreAuthorize
and @PostAuthorize
annotations, which alows you to define simple rules based on Spring Expression Language.
edited Jan 3 at 14:28
answered Jan 3 at 9:47
MattMatt
1,73711522
1,73711522
Thank you Matt for your help, I just updated my question. I'm using Spring Boot and also I'm using Spring Security with Dao Authentication because I want to get the user from the database. Now I understand how to use Principal in the controller methods to achieve what I want, but I don't know how to use Spring Security for this, what should I add in the spring security configuration file?
– elvis
Jan 3 at 11:48
Spring Security can help you for general role based rules, like endpoint "/users/*" is only accessible to connected user (or user with role X). And as @Glains mentioned in his answer, it also allows you to define simple rules with annotations such as@PreAuthorize
with Spring EL expressions.
– Matt
Jan 3 at 14:22
add a comment |
Thank you Matt for your help, I just updated my question. I'm using Spring Boot and also I'm using Spring Security with Dao Authentication because I want to get the user from the database. Now I understand how to use Principal in the controller methods to achieve what I want, but I don't know how to use Spring Security for this, what should I add in the spring security configuration file?
– elvis
Jan 3 at 11:48
Spring Security can help you for general role based rules, like endpoint "/users/*" is only accessible to connected user (or user with role X). And as @Glains mentioned in his answer, it also allows you to define simple rules with annotations such as@PreAuthorize
with Spring EL expressions.
– Matt
Jan 3 at 14:22
Thank you Matt for your help, I just updated my question. I'm using Spring Boot and also I'm using Spring Security with Dao Authentication because I want to get the user from the database. Now I understand how to use Principal in the controller methods to achieve what I want, but I don't know how to use Spring Security for this, what should I add in the spring security configuration file?
– elvis
Jan 3 at 11:48
Thank you Matt for your help, I just updated my question. I'm using Spring Boot and also I'm using Spring Security with Dao Authentication because I want to get the user from the database. Now I understand how to use Principal in the controller methods to achieve what I want, but I don't know how to use Spring Security for this, what should I add in the spring security configuration file?
– elvis
Jan 3 at 11:48
Spring Security can help you for general role based rules, like endpoint "/users/*" is only accessible to connected user (or user with role X). And as @Glains mentioned in his answer, it also allows you to define simple rules with annotations such as
@PreAuthorize
with Spring EL expressions.– Matt
Jan 3 at 14:22
Spring Security can help you for general role based rules, like endpoint "/users/*" is only accessible to connected user (or user with role X). And as @Glains mentioned in his answer, it also allows you to define simple rules with annotations such as
@PreAuthorize
with Spring EL expressions.– Matt
Jan 3 at 14:22
add a comment |
To answer your new question on "Dao Authentication" the answer is to provide a custom UserDetailsService
.
From the configuration that you have attached to your question it looks that you already have a MyUserDetailsService
.
There are plenty of articles that explain how to use a custom DetailsService
. This one seems to match your requirements : https://www.baeldung.com/spring-security-authentication-with-a-database
Edit: On how to be sure that only John can see John's items.
Basically, the only ting that you can do to esnure that only John can see his goals it to restrict the goals to only those owned by John. But there is plenty way of doing this.
As you suggest in your initial question, you can just select the goals for a specific user. The power with spring-security is that it can inject a
Principal
but also kind of orher authentication object.You can also make that more implicit an filter a the DAO/Repository side by using the
SecurityContextHolder
. This approach is fine and looks better when your system is more user centric or like a multi-tenant system.Using some specific @Annotations or Aspects would also be a solution but maybe less obvious in this case.
Thank you but the problem wasn't about "Dao Authentication", I have implemented this... it is the old problem, when I'm using Postman, I'm logged in with john and I can retrieve john's goals and also any other user's goals, I want to allow retrieving only john's goals when I'm logged in with john... you said that I ca inject the authenticated user into my method parameter, and I did this and works perfectly... also you said that spring-security is better than this, and I want to know how can I use spring-security for this situation.
– elvis
Jan 4 at 16:46
I said that spring-security is a good security framework. And, since you are suring spring, it is the defacto choice. I will edit this answer to explain your alternatives.
– gervais.b
Jan 7 at 6:58
add a comment |
To answer your new question on "Dao Authentication" the answer is to provide a custom UserDetailsService
.
From the configuration that you have attached to your question it looks that you already have a MyUserDetailsService
.
There are plenty of articles that explain how to use a custom DetailsService
. This one seems to match your requirements : https://www.baeldung.com/spring-security-authentication-with-a-database
Edit: On how to be sure that only John can see John's items.
Basically, the only ting that you can do to esnure that only John can see his goals it to restrict the goals to only those owned by John. But there is plenty way of doing this.
As you suggest in your initial question, you can just select the goals for a specific user. The power with spring-security is that it can inject a
Principal
but also kind of orher authentication object.You can also make that more implicit an filter a the DAO/Repository side by using the
SecurityContextHolder
. This approach is fine and looks better when your system is more user centric or like a multi-tenant system.Using some specific @Annotations or Aspects would also be a solution but maybe less obvious in this case.
Thank you but the problem wasn't about "Dao Authentication", I have implemented this... it is the old problem, when I'm using Postman, I'm logged in with john and I can retrieve john's goals and also any other user's goals, I want to allow retrieving only john's goals when I'm logged in with john... you said that I ca inject the authenticated user into my method parameter, and I did this and works perfectly... also you said that spring-security is better than this, and I want to know how can I use spring-security for this situation.
– elvis
Jan 4 at 16:46
I said that spring-security is a good security framework. And, since you are suring spring, it is the defacto choice. I will edit this answer to explain your alternatives.
– gervais.b
Jan 7 at 6:58
add a comment |
To answer your new question on "Dao Authentication" the answer is to provide a custom UserDetailsService
.
From the configuration that you have attached to your question it looks that you already have a MyUserDetailsService
.
There are plenty of articles that explain how to use a custom DetailsService
. This one seems to match your requirements : https://www.baeldung.com/spring-security-authentication-with-a-database
Edit: On how to be sure that only John can see John's items.
Basically, the only ting that you can do to esnure that only John can see his goals it to restrict the goals to only those owned by John. But there is plenty way of doing this.
As you suggest in your initial question, you can just select the goals for a specific user. The power with spring-security is that it can inject a
Principal
but also kind of orher authentication object.You can also make that more implicit an filter a the DAO/Repository side by using the
SecurityContextHolder
. This approach is fine and looks better when your system is more user centric or like a multi-tenant system.Using some specific @Annotations or Aspects would also be a solution but maybe less obvious in this case.
To answer your new question on "Dao Authentication" the answer is to provide a custom UserDetailsService
.
From the configuration that you have attached to your question it looks that you already have a MyUserDetailsService
.
There are plenty of articles that explain how to use a custom DetailsService
. This one seems to match your requirements : https://www.baeldung.com/spring-security-authentication-with-a-database
Edit: On how to be sure that only John can see John's items.
Basically, the only ting that you can do to esnure that only John can see his goals it to restrict the goals to only those owned by John. But there is plenty way of doing this.
As you suggest in your initial question, you can just select the goals for a specific user. The power with spring-security is that it can inject a
Principal
but also kind of orher authentication object.You can also make that more implicit an filter a the DAO/Repository side by using the
SecurityContextHolder
. This approach is fine and looks better when your system is more user centric or like a multi-tenant system.Using some specific @Annotations or Aspects would also be a solution but maybe less obvious in this case.
edited Jan 7 at 7:08
answered Jan 4 at 12:48
gervais.bgervais.b
867427
867427
Thank you but the problem wasn't about "Dao Authentication", I have implemented this... it is the old problem, when I'm using Postman, I'm logged in with john and I can retrieve john's goals and also any other user's goals, I want to allow retrieving only john's goals when I'm logged in with john... you said that I ca inject the authenticated user into my method parameter, and I did this and works perfectly... also you said that spring-security is better than this, and I want to know how can I use spring-security for this situation.
– elvis
Jan 4 at 16:46
I said that spring-security is a good security framework. And, since you are suring spring, it is the defacto choice. I will edit this answer to explain your alternatives.
– gervais.b
Jan 7 at 6:58
add a comment |
Thank you but the problem wasn't about "Dao Authentication", I have implemented this... it is the old problem, when I'm using Postman, I'm logged in with john and I can retrieve john's goals and also any other user's goals, I want to allow retrieving only john's goals when I'm logged in with john... you said that I ca inject the authenticated user into my method parameter, and I did this and works perfectly... also you said that spring-security is better than this, and I want to know how can I use spring-security for this situation.
– elvis
Jan 4 at 16:46
I said that spring-security is a good security framework. And, since you are suring spring, it is the defacto choice. I will edit this answer to explain your alternatives.
– gervais.b
Jan 7 at 6:58
Thank you but the problem wasn't about "Dao Authentication", I have implemented this... it is the old problem, when I'm using Postman, I'm logged in with john and I can retrieve john's goals and also any other user's goals, I want to allow retrieving only john's goals when I'm logged in with john... you said that I ca inject the authenticated user into my method parameter, and I did this and works perfectly... also you said that spring-security is better than this, and I want to know how can I use spring-security for this situation.
– elvis
Jan 4 at 16:46
Thank you but the problem wasn't about "Dao Authentication", I have implemented this... it is the old problem, when I'm using Postman, I'm logged in with john and I can retrieve john's goals and also any other user's goals, I want to allow retrieving only john's goals when I'm logged in with john... you said that I ca inject the authenticated user into my method parameter, and I did this and works perfectly... also you said that spring-security is better than this, and I want to know how can I use spring-security for this situation.
– elvis
Jan 4 at 16:46
I said that spring-security is a good security framework. And, since you are suring spring, it is the defacto choice. I will edit this answer to explain your alternatives.
– gervais.b
Jan 7 at 6:58
I said that spring-security is a good security framework. And, since you are suring spring, it is the defacto choice. I will edit this answer to explain your alternatives.
– gervais.b
Jan 7 at 6:58
add a comment |
Answer is already given on before answers. I just want to make an addition, take a look at this article. You can easily implement spring security with json web token:
https://auth0.com/blog/implementing-jwt-authentication-on-spring-boot/
OP is specifically asking for basic auth, not JWT.
– Matt
Jan 3 at 9:52
@Ali, I'll try to implement JWT in my app, and I just read this article, but I don't understand why they don't use Autowired annotation while they use Spring Boot
– elvis
Jan 9 at 15:32
In Spring, you don't have to use Autowired annotation while you are autowiring somtehings. Because there is three ways of Autowiring. Annotation based, setter based, constructor based. They've used constructor based way. @elvis
– Ali Kaan Bacı
Jan 10 at 16:13
add a comment |
Answer is already given on before answers. I just want to make an addition, take a look at this article. You can easily implement spring security with json web token:
https://auth0.com/blog/implementing-jwt-authentication-on-spring-boot/
OP is specifically asking for basic auth, not JWT.
– Matt
Jan 3 at 9:52
@Ali, I'll try to implement JWT in my app, and I just read this article, but I don't understand why they don't use Autowired annotation while they use Spring Boot
– elvis
Jan 9 at 15:32
In Spring, you don't have to use Autowired annotation while you are autowiring somtehings. Because there is three ways of Autowiring. Annotation based, setter based, constructor based. They've used constructor based way. @elvis
– Ali Kaan Bacı
Jan 10 at 16:13
add a comment |
Answer is already given on before answers. I just want to make an addition, take a look at this article. You can easily implement spring security with json web token:
https://auth0.com/blog/implementing-jwt-authentication-on-spring-boot/
Answer is already given on before answers. I just want to make an addition, take a look at this article. You can easily implement spring security with json web token:
https://auth0.com/blog/implementing-jwt-authentication-on-spring-boot/
answered Jan 3 at 9:45
Ali Kaan BacıAli Kaan Bacı
244
244
OP is specifically asking for basic auth, not JWT.
– Matt
Jan 3 at 9:52
@Ali, I'll try to implement JWT in my app, and I just read this article, but I don't understand why they don't use Autowired annotation while they use Spring Boot
– elvis
Jan 9 at 15:32
In Spring, you don't have to use Autowired annotation while you are autowiring somtehings. Because there is three ways of Autowiring. Annotation based, setter based, constructor based. They've used constructor based way. @elvis
– Ali Kaan Bacı
Jan 10 at 16:13
add a comment |
OP is specifically asking for basic auth, not JWT.
– Matt
Jan 3 at 9:52
@Ali, I'll try to implement JWT in my app, and I just read this article, but I don't understand why they don't use Autowired annotation while they use Spring Boot
– elvis
Jan 9 at 15:32
In Spring, you don't have to use Autowired annotation while you are autowiring somtehings. Because there is three ways of Autowiring. Annotation based, setter based, constructor based. They've used constructor based way. @elvis
– Ali Kaan Bacı
Jan 10 at 16:13
OP is specifically asking for basic auth, not JWT.
– Matt
Jan 3 at 9:52
OP is specifically asking for basic auth, not JWT.
– Matt
Jan 3 at 9:52
@Ali, I'll try to implement JWT in my app, and I just read this article, but I don't understand why they don't use Autowired annotation while they use Spring Boot
– elvis
Jan 9 at 15:32
@Ali, I'll try to implement JWT in my app, and I just read this article, but I don't understand why they don't use Autowired annotation while they use Spring Boot
– elvis
Jan 9 at 15:32
In Spring, you don't have to use Autowired annotation while you are autowiring somtehings. Because there is three ways of Autowiring. Annotation based, setter based, constructor based. They've used constructor based way. @elvis
– Ali Kaan Bacı
Jan 10 at 16:13
In Spring, you don't have to use Autowired annotation while you are autowiring somtehings. Because there is three ways of Autowiring. Annotation based, setter based, constructor based. They've used constructor based way. @elvis
– Ali Kaan Bacı
Jan 10 at 16:13
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54019074%2fhow-to-use-restful-with-basic-authentication-in-spring-boot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Bk7b6dQe YN
4
are you using spring? specifically spring-security?
– Yogendra Mishra
Jan 3 at 9:04
3
Sidenote, what is the data-type of
loginUsername
? If its String, then you are comparing it wrong.– Nicholas K
Jan 3 at 9:07
2
It depends which framework you are using (if you are using any). You're talking about
RestController
so I'm guessing you're using Spring (you should definitely add the appropriate tags to your question). If so, have a look at Spring security which allow you to configure how to secure your app.– Matt
Jan 3 at 9:11