How to optimize performance using instancing in a scene full of skinned-mesh?
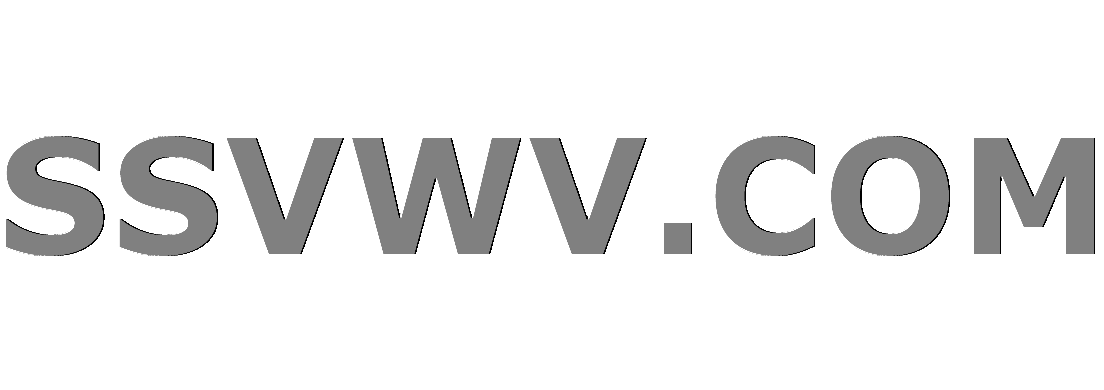
Multi tool use
I’m working on a web tower defense game based on three.js. Now I’m stuck at optimizing the performance.
My game loads two GLTF models as enemy and tower, both have skinned-mesh. When the player creates a tower or the game spawns an enemy, I use THREE.AnimationUtils.clone
to clone the loaded model. Then I add this cloned model to the scene. For animation, I use THREE.AnimationObjectGroup
to animate all the enemies.
This results in an average of 370 draw-calls per frame in the performance test with the scene loaded with 45 towers and 70 enemies, which is a nightmare for the game.
I think maybe using instancing can optimize the performance because every tower and enemy share the same model and state in each frame, but only rotation and position are different. But after I studied some examples using instancing, there is no example using instancing with skinned-mesh. (There is a discussion here, but the result here doesn't mention any method with instancing.)
Is there any chance that this can be done with three.js, or some other solution for this situation?
Update
After researched more I found some concepts maybe can help me to implement instancing with skinned-mesh.
Concept
The original post here implement skinned-mesh with instancing in Unity. (It's written in Chinese, I translated the main concept in the following.)
After loaded a skinned-mesh, it has an initial state with all vertices (for clarity, each initial vertex denote as PLT
in the following). In any frame of the animation, the final position of PLT
(denote as PI
) equals to a series of matrix multiplication PI = (M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_1 * PLT) + (M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_2 * PLT) + (...)
M_bind_1
is the bone-binding matrix of bone 1.
M_m_n
means the transformation of bonem
relative to it's initial state under the coordinate system of bonen
.
For simplify, use M_f_i = M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_i
to represent the transformation. M_f_i
means bone-binding matrix of bone i
after multiplication at frame f
, so PI = (M_f_1 * PLT) + (M_f_2 * PLT) + (...)
Once we know M_f_i
, we can calculate the position of every vertex in frame f
.
The process above can be done inside GPU by passing M_f_i
which wrap as a texture. (Under the premise that the skinned-mesh needs to animate around 10 animations and less amount of bones, the require memory is about 0.75Mb.). Finally, we can pass different frame number f
to each instance to render skinned-mesh with animation in one draw-call.
Implement with three.js
I haven't build an example code yet because I don't know the concept can work on WebGL or not (also I'm not familiar with GLSL), but I think the way to implement it with three.js can done as the following.
- Follow here to get
M_f_i
. - Use
THREE.InstancedBufferGeometry
andTHREE.RawShaderMaterial
.
- In
uniforms
pass initial geometry,M_f_i
and texture. - In
vertexShader
processPI = (M_f_1 * PLT) + (M_f_2 * PLT) + (...)
. - In
fragmentShader
process texture (I have no idea how to do it).
- In
- Pass
f
and other instance attribute usingTHREE.InstancedBufferAttribute
.
Problem
- Where is
M_f
and how to get it byTHREE.AnimationClip
in step 1? - How to index each
PLT
(vertex in geometry)? - How to deal with texture?
- How to deal with hierarchy
Object3D
(Object3D.children
haveTHREE.Mesh
andTHREE.SkinnedMesh
at the same time)?
I need someone to tell me this idea works in three.js or not, and how to solve the problem above.
javascript three.js
New contributor
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I’m working on a web tower defense game based on three.js. Now I’m stuck at optimizing the performance.
My game loads two GLTF models as enemy and tower, both have skinned-mesh. When the player creates a tower or the game spawns an enemy, I use THREE.AnimationUtils.clone
to clone the loaded model. Then I add this cloned model to the scene. For animation, I use THREE.AnimationObjectGroup
to animate all the enemies.
This results in an average of 370 draw-calls per frame in the performance test with the scene loaded with 45 towers and 70 enemies, which is a nightmare for the game.
I think maybe using instancing can optimize the performance because every tower and enemy share the same model and state in each frame, but only rotation and position are different. But after I studied some examples using instancing, there is no example using instancing with skinned-mesh. (There is a discussion here, but the result here doesn't mention any method with instancing.)
Is there any chance that this can be done with three.js, or some other solution for this situation?
Update
After researched more I found some concepts maybe can help me to implement instancing with skinned-mesh.
Concept
The original post here implement skinned-mesh with instancing in Unity. (It's written in Chinese, I translated the main concept in the following.)
After loaded a skinned-mesh, it has an initial state with all vertices (for clarity, each initial vertex denote as PLT
in the following). In any frame of the animation, the final position of PLT
(denote as PI
) equals to a series of matrix multiplication PI = (M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_1 * PLT) + (M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_2 * PLT) + (...)
M_bind_1
is the bone-binding matrix of bone 1.
M_m_n
means the transformation of bonem
relative to it's initial state under the coordinate system of bonen
.
For simplify, use M_f_i = M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_i
to represent the transformation. M_f_i
means bone-binding matrix of bone i
after multiplication at frame f
, so PI = (M_f_1 * PLT) + (M_f_2 * PLT) + (...)
Once we know M_f_i
, we can calculate the position of every vertex in frame f
.
The process above can be done inside GPU by passing M_f_i
which wrap as a texture. (Under the premise that the skinned-mesh needs to animate around 10 animations and less amount of bones, the require memory is about 0.75Mb.). Finally, we can pass different frame number f
to each instance to render skinned-mesh with animation in one draw-call.
Implement with three.js
I haven't build an example code yet because I don't know the concept can work on WebGL or not (also I'm not familiar with GLSL), but I think the way to implement it with three.js can done as the following.
- Follow here to get
M_f_i
. - Use
THREE.InstancedBufferGeometry
andTHREE.RawShaderMaterial
.
- In
uniforms
pass initial geometry,M_f_i
and texture. - In
vertexShader
processPI = (M_f_1 * PLT) + (M_f_2 * PLT) + (...)
. - In
fragmentShader
process texture (I have no idea how to do it).
- In
- Pass
f
and other instance attribute usingTHREE.InstancedBufferAttribute
.
Problem
- Where is
M_f
and how to get it byTHREE.AnimationClip
in step 1? - How to index each
PLT
(vertex in geometry)? - How to deal with texture?
- How to deal with hierarchy
Object3D
(Object3D.children
haveTHREE.Mesh
andTHREE.SkinnedMesh
at the same time)?
I need someone to tell me this idea works in three.js or not, and how to solve the problem above.
javascript three.js
New contributor
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I’m working on a web tower defense game based on three.js. Now I’m stuck at optimizing the performance.
My game loads two GLTF models as enemy and tower, both have skinned-mesh. When the player creates a tower or the game spawns an enemy, I use THREE.AnimationUtils.clone
to clone the loaded model. Then I add this cloned model to the scene. For animation, I use THREE.AnimationObjectGroup
to animate all the enemies.
This results in an average of 370 draw-calls per frame in the performance test with the scene loaded with 45 towers and 70 enemies, which is a nightmare for the game.
I think maybe using instancing can optimize the performance because every tower and enemy share the same model and state in each frame, but only rotation and position are different. But after I studied some examples using instancing, there is no example using instancing with skinned-mesh. (There is a discussion here, but the result here doesn't mention any method with instancing.)
Is there any chance that this can be done with three.js, or some other solution for this situation?
Update
After researched more I found some concepts maybe can help me to implement instancing with skinned-mesh.
Concept
The original post here implement skinned-mesh with instancing in Unity. (It's written in Chinese, I translated the main concept in the following.)
After loaded a skinned-mesh, it has an initial state with all vertices (for clarity, each initial vertex denote as PLT
in the following). In any frame of the animation, the final position of PLT
(denote as PI
) equals to a series of matrix multiplication PI = (M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_1 * PLT) + (M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_2 * PLT) + (...)
M_bind_1
is the bone-binding matrix of bone 1.
M_m_n
means the transformation of bonem
relative to it's initial state under the coordinate system of bonen
.
For simplify, use M_f_i = M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_i
to represent the transformation. M_f_i
means bone-binding matrix of bone i
after multiplication at frame f
, so PI = (M_f_1 * PLT) + (M_f_2 * PLT) + (...)
Once we know M_f_i
, we can calculate the position of every vertex in frame f
.
The process above can be done inside GPU by passing M_f_i
which wrap as a texture. (Under the premise that the skinned-mesh needs to animate around 10 animations and less amount of bones, the require memory is about 0.75Mb.). Finally, we can pass different frame number f
to each instance to render skinned-mesh with animation in one draw-call.
Implement with three.js
I haven't build an example code yet because I don't know the concept can work on WebGL or not (also I'm not familiar with GLSL), but I think the way to implement it with three.js can done as the following.
- Follow here to get
M_f_i
. - Use
THREE.InstancedBufferGeometry
andTHREE.RawShaderMaterial
.
- In
uniforms
pass initial geometry,M_f_i
and texture. - In
vertexShader
processPI = (M_f_1 * PLT) + (M_f_2 * PLT) + (...)
. - In
fragmentShader
process texture (I have no idea how to do it).
- In
- Pass
f
and other instance attribute usingTHREE.InstancedBufferAttribute
.
Problem
- Where is
M_f
and how to get it byTHREE.AnimationClip
in step 1? - How to index each
PLT
(vertex in geometry)? - How to deal with texture?
- How to deal with hierarchy
Object3D
(Object3D.children
haveTHREE.Mesh
andTHREE.SkinnedMesh
at the same time)?
I need someone to tell me this idea works in three.js or not, and how to solve the problem above.
javascript three.js
New contributor
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I’m working on a web tower defense game based on three.js. Now I’m stuck at optimizing the performance.
My game loads two GLTF models as enemy and tower, both have skinned-mesh. When the player creates a tower or the game spawns an enemy, I use THREE.AnimationUtils.clone
to clone the loaded model. Then I add this cloned model to the scene. For animation, I use THREE.AnimationObjectGroup
to animate all the enemies.
This results in an average of 370 draw-calls per frame in the performance test with the scene loaded with 45 towers and 70 enemies, which is a nightmare for the game.
I think maybe using instancing can optimize the performance because every tower and enemy share the same model and state in each frame, but only rotation and position are different. But after I studied some examples using instancing, there is no example using instancing with skinned-mesh. (There is a discussion here, but the result here doesn't mention any method with instancing.)
Is there any chance that this can be done with three.js, or some other solution for this situation?
Update
After researched more I found some concepts maybe can help me to implement instancing with skinned-mesh.
Concept
The original post here implement skinned-mesh with instancing in Unity. (It's written in Chinese, I translated the main concept in the following.)
After loaded a skinned-mesh, it has an initial state with all vertices (for clarity, each initial vertex denote as PLT
in the following). In any frame of the animation, the final position of PLT
(denote as PI
) equals to a series of matrix multiplication PI = (M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_1 * PLT) + (M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_2 * PLT) + (...)
M_bind_1
is the bone-binding matrix of bone 1.
M_m_n
means the transformation of bonem
relative to it's initial state under the coordinate system of bonen
.
For simplify, use M_f_i = M_rootlocal * ... * M_2_3 * M_1_2 * M_bind_i
to represent the transformation. M_f_i
means bone-binding matrix of bone i
after multiplication at frame f
, so PI = (M_f_1 * PLT) + (M_f_2 * PLT) + (...)
Once we know M_f_i
, we can calculate the position of every vertex in frame f
.
The process above can be done inside GPU by passing M_f_i
which wrap as a texture. (Under the premise that the skinned-mesh needs to animate around 10 animations and less amount of bones, the require memory is about 0.75Mb.). Finally, we can pass different frame number f
to each instance to render skinned-mesh with animation in one draw-call.
Implement with three.js
I haven't build an example code yet because I don't know the concept can work on WebGL or not (also I'm not familiar with GLSL), but I think the way to implement it with three.js can done as the following.
- Follow here to get
M_f_i
. - Use
THREE.InstancedBufferGeometry
andTHREE.RawShaderMaterial
.
- In
uniforms
pass initial geometry,M_f_i
and texture. - In
vertexShader
processPI = (M_f_1 * PLT) + (M_f_2 * PLT) + (...)
. - In
fragmentShader
process texture (I have no idea how to do it).
- In
- Pass
f
and other instance attribute usingTHREE.InstancedBufferAttribute
.
Problem
- Where is
M_f
and how to get it byTHREE.AnimationClip
in step 1? - How to index each
PLT
(vertex in geometry)? - How to deal with texture?
- How to deal with hierarchy
Object3D
(Object3D.children
haveTHREE.Mesh
andTHREE.SkinnedMesh
at the same time)?
I need someone to tell me this idea works in three.js or not, and how to solve the problem above.
javascript three.js
javascript three.js
New contributor
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Dec 28 '18 at 8:38
New contributor
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Dec 27 '18 at 15:48
YanJen Chen
163
163
New contributor
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
YanJen Chen is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I remember there was a Geometry or Mesh Merge function that was really helping me in the past with such a cases. I recommend you search in that direction.
There are many counterparts to its usage such as loosing the individuality of each 3d object you use but when possible you should use it for static elements like environment objects, in other cases it may be also useful if your individual objects/towers are based on many single 3d objects in the way that they become just one...
From my experience (could vary a lot depending on each computer and size of 3d viewport) at the end you should never have more than 50 (simple) 3d objects in front of your visible camera area and reuse all materials, geometries, mesh... otherwise you'll end up having a very poor performance as soon as something fun is happening in your game.
Hope it helps!
Appreciate your reply! I thinkmerge
is a good way for environment objects, but it will hard to implement to the dynamic objects such as enemy and tower because their behavior is depends on the user. I have simply the model before using it. The enemy model contains 3THREE.Bone
and 1THREE.SkinnedMesh
. The tower model contains 2THREE.Mesh
, 1THREE.Bone
and 1THREE.SkinnedMesh
. All models have simplified its geometry.
– YanJen Chen
Dec 27 '18 at 16:49
Why do you need aBone
and aSkinnedMesh
in your tower? Shouldn't it simply need 2Mesh
es for the base and rotating turret?
– Marquizzo
Dec 27 '18 at 22:00
There are some animations for the barrel part, which useBone
to control the behaviors. @Marquizzo
– YanJen Chen
Dec 27 '18 at 23:08
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
YanJen Chen is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53947554%2fhow-to-optimize-performance-using-instancing-in-a-scene-full-of-skinned-mesh%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I remember there was a Geometry or Mesh Merge function that was really helping me in the past with such a cases. I recommend you search in that direction.
There are many counterparts to its usage such as loosing the individuality of each 3d object you use but when possible you should use it for static elements like environment objects, in other cases it may be also useful if your individual objects/towers are based on many single 3d objects in the way that they become just one...
From my experience (could vary a lot depending on each computer and size of 3d viewport) at the end you should never have more than 50 (simple) 3d objects in front of your visible camera area and reuse all materials, geometries, mesh... otherwise you'll end up having a very poor performance as soon as something fun is happening in your game.
Hope it helps!
Appreciate your reply! I thinkmerge
is a good way for environment objects, but it will hard to implement to the dynamic objects such as enemy and tower because their behavior is depends on the user. I have simply the model before using it. The enemy model contains 3THREE.Bone
and 1THREE.SkinnedMesh
. The tower model contains 2THREE.Mesh
, 1THREE.Bone
and 1THREE.SkinnedMesh
. All models have simplified its geometry.
– YanJen Chen
Dec 27 '18 at 16:49
Why do you need aBone
and aSkinnedMesh
in your tower? Shouldn't it simply need 2Mesh
es for the base and rotating turret?
– Marquizzo
Dec 27 '18 at 22:00
There are some animations for the barrel part, which useBone
to control the behaviors. @Marquizzo
– YanJen Chen
Dec 27 '18 at 23:08
add a comment |
I remember there was a Geometry or Mesh Merge function that was really helping me in the past with such a cases. I recommend you search in that direction.
There are many counterparts to its usage such as loosing the individuality of each 3d object you use but when possible you should use it for static elements like environment objects, in other cases it may be also useful if your individual objects/towers are based on many single 3d objects in the way that they become just one...
From my experience (could vary a lot depending on each computer and size of 3d viewport) at the end you should never have more than 50 (simple) 3d objects in front of your visible camera area and reuse all materials, geometries, mesh... otherwise you'll end up having a very poor performance as soon as something fun is happening in your game.
Hope it helps!
Appreciate your reply! I thinkmerge
is a good way for environment objects, but it will hard to implement to the dynamic objects such as enemy and tower because their behavior is depends on the user. I have simply the model before using it. The enemy model contains 3THREE.Bone
and 1THREE.SkinnedMesh
. The tower model contains 2THREE.Mesh
, 1THREE.Bone
and 1THREE.SkinnedMesh
. All models have simplified its geometry.
– YanJen Chen
Dec 27 '18 at 16:49
Why do you need aBone
and aSkinnedMesh
in your tower? Shouldn't it simply need 2Mesh
es for the base and rotating turret?
– Marquizzo
Dec 27 '18 at 22:00
There are some animations for the barrel part, which useBone
to control the behaviors. @Marquizzo
– YanJen Chen
Dec 27 '18 at 23:08
add a comment |
I remember there was a Geometry or Mesh Merge function that was really helping me in the past with such a cases. I recommend you search in that direction.
There are many counterparts to its usage such as loosing the individuality of each 3d object you use but when possible you should use it for static elements like environment objects, in other cases it may be also useful if your individual objects/towers are based on many single 3d objects in the way that they become just one...
From my experience (could vary a lot depending on each computer and size of 3d viewport) at the end you should never have more than 50 (simple) 3d objects in front of your visible camera area and reuse all materials, geometries, mesh... otherwise you'll end up having a very poor performance as soon as something fun is happening in your game.
Hope it helps!
I remember there was a Geometry or Mesh Merge function that was really helping me in the past with such a cases. I recommend you search in that direction.
There are many counterparts to its usage such as loosing the individuality of each 3d object you use but when possible you should use it for static elements like environment objects, in other cases it may be also useful if your individual objects/towers are based on many single 3d objects in the way that they become just one...
From my experience (could vary a lot depending on each computer and size of 3d viewport) at the end you should never have more than 50 (simple) 3d objects in front of your visible camera area and reuse all materials, geometries, mesh... otherwise you'll end up having a very poor performance as soon as something fun is happening in your game.
Hope it helps!
answered Dec 27 '18 at 16:28
juagicre
4391023
4391023
Appreciate your reply! I thinkmerge
is a good way for environment objects, but it will hard to implement to the dynamic objects such as enemy and tower because their behavior is depends on the user. I have simply the model before using it. The enemy model contains 3THREE.Bone
and 1THREE.SkinnedMesh
. The tower model contains 2THREE.Mesh
, 1THREE.Bone
and 1THREE.SkinnedMesh
. All models have simplified its geometry.
– YanJen Chen
Dec 27 '18 at 16:49
Why do you need aBone
and aSkinnedMesh
in your tower? Shouldn't it simply need 2Mesh
es for the base and rotating turret?
– Marquizzo
Dec 27 '18 at 22:00
There are some animations for the barrel part, which useBone
to control the behaviors. @Marquizzo
– YanJen Chen
Dec 27 '18 at 23:08
add a comment |
Appreciate your reply! I thinkmerge
is a good way for environment objects, but it will hard to implement to the dynamic objects such as enemy and tower because their behavior is depends on the user. I have simply the model before using it. The enemy model contains 3THREE.Bone
and 1THREE.SkinnedMesh
. The tower model contains 2THREE.Mesh
, 1THREE.Bone
and 1THREE.SkinnedMesh
. All models have simplified its geometry.
– YanJen Chen
Dec 27 '18 at 16:49
Why do you need aBone
and aSkinnedMesh
in your tower? Shouldn't it simply need 2Mesh
es for the base and rotating turret?
– Marquizzo
Dec 27 '18 at 22:00
There are some animations for the barrel part, which useBone
to control the behaviors. @Marquizzo
– YanJen Chen
Dec 27 '18 at 23:08
Appreciate your reply! I think
merge
is a good way for environment objects, but it will hard to implement to the dynamic objects such as enemy and tower because their behavior is depends on the user. I have simply the model before using it. The enemy model contains 3 THREE.Bone
and 1 THREE.SkinnedMesh
. The tower model contains 2 THREE.Mesh
, 1 THREE.Bone
and 1 THREE.SkinnedMesh
. All models have simplified its geometry.– YanJen Chen
Dec 27 '18 at 16:49
Appreciate your reply! I think
merge
is a good way for environment objects, but it will hard to implement to the dynamic objects such as enemy and tower because their behavior is depends on the user. I have simply the model before using it. The enemy model contains 3 THREE.Bone
and 1 THREE.SkinnedMesh
. The tower model contains 2 THREE.Mesh
, 1 THREE.Bone
and 1 THREE.SkinnedMesh
. All models have simplified its geometry.– YanJen Chen
Dec 27 '18 at 16:49
Why do you need a
Bone
and a SkinnedMesh
in your tower? Shouldn't it simply need 2 Mesh
es for the base and rotating turret?– Marquizzo
Dec 27 '18 at 22:00
Why do you need a
Bone
and a SkinnedMesh
in your tower? Shouldn't it simply need 2 Mesh
es for the base and rotating turret?– Marquizzo
Dec 27 '18 at 22:00
There are some animations for the barrel part, which use
Bone
to control the behaviors. @Marquizzo– YanJen Chen
Dec 27 '18 at 23:08
There are some animations for the barrel part, which use
Bone
to control the behaviors. @Marquizzo– YanJen Chen
Dec 27 '18 at 23:08
add a comment |
YanJen Chen is a new contributor. Be nice, and check out our Code of Conduct.
YanJen Chen is a new contributor. Be nice, and check out our Code of Conduct.
YanJen Chen is a new contributor. Be nice, and check out our Code of Conduct.
YanJen Chen is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53947554%2fhow-to-optimize-performance-using-instancing-in-a-scene-full-of-skinned-mesh%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2NGfLD