Concurrent in queue of Linkedlist
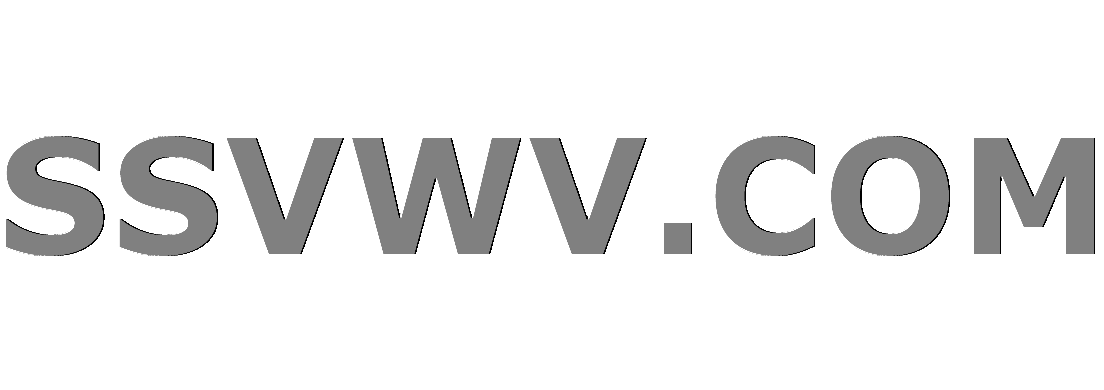
Multi tool use
multiple threads add elements to the same queue in parallel and other threads consume elements in it.
When I use LinkedList
in the example below, the queue will end up with serveral null value in it, and the size is a constant value. No matter how many times the /consumer
is requested, the queue still has null value and the size is non-zero.
So I change Linkedlist
to ConcurrentLinkedQueue
and the problem disappear.It seems a thread-safe problem.
How can queue
has null element? why queue.poll()
can't poll the null
element in queue?
Example:
@RestController
@RequestMapping("/v1")
public class SendController {
public static Queue<String> queue = new ConcurrentLinkedQueue<>();//ok
public static Queue<String> queue = new LinkedList<>();//will have null value in it and can't poll from it
@GetMapping(value = "/produce")
public String pro() {
String s = UUID.randomUUID().toString();
queue.add(s);
return "true";
}
@GetMapping(value = "/consume")
public String con() {
if(queue.size() == 0) {
return null;
}
return queue.poll();
}
java linked-list queue thread-safety
add a comment |
multiple threads add elements to the same queue in parallel and other threads consume elements in it.
When I use LinkedList
in the example below, the queue will end up with serveral null value in it, and the size is a constant value. No matter how many times the /consumer
is requested, the queue still has null value and the size is non-zero.
So I change Linkedlist
to ConcurrentLinkedQueue
and the problem disappear.It seems a thread-safe problem.
How can queue
has null element? why queue.poll()
can't poll the null
element in queue?
Example:
@RestController
@RequestMapping("/v1")
public class SendController {
public static Queue<String> queue = new ConcurrentLinkedQueue<>();//ok
public static Queue<String> queue = new LinkedList<>();//will have null value in it and can't poll from it
@GetMapping(value = "/produce")
public String pro() {
String s = UUID.randomUUID().toString();
queue.add(s);
return "true";
}
@GetMapping(value = "/consume")
public String con() {
if(queue.size() == 0) {
return null;
}
return queue.poll();
}
java linked-list queue thread-safety
3
Please read How to Ask and provide a Minimal, Complete, and Verifiable example.
– Rafael
Jan 3 at 4:16
add a comment |
multiple threads add elements to the same queue in parallel and other threads consume elements in it.
When I use LinkedList
in the example below, the queue will end up with serveral null value in it, and the size is a constant value. No matter how many times the /consumer
is requested, the queue still has null value and the size is non-zero.
So I change Linkedlist
to ConcurrentLinkedQueue
and the problem disappear.It seems a thread-safe problem.
How can queue
has null element? why queue.poll()
can't poll the null
element in queue?
Example:
@RestController
@RequestMapping("/v1")
public class SendController {
public static Queue<String> queue = new ConcurrentLinkedQueue<>();//ok
public static Queue<String> queue = new LinkedList<>();//will have null value in it and can't poll from it
@GetMapping(value = "/produce")
public String pro() {
String s = UUID.randomUUID().toString();
queue.add(s);
return "true";
}
@GetMapping(value = "/consume")
public String con() {
if(queue.size() == 0) {
return null;
}
return queue.poll();
}
java linked-list queue thread-safety
multiple threads add elements to the same queue in parallel and other threads consume elements in it.
When I use LinkedList
in the example below, the queue will end up with serveral null value in it, and the size is a constant value. No matter how many times the /consumer
is requested, the queue still has null value and the size is non-zero.
So I change Linkedlist
to ConcurrentLinkedQueue
and the problem disappear.It seems a thread-safe problem.
How can queue
has null element? why queue.poll()
can't poll the null
element in queue?
Example:
@RestController
@RequestMapping("/v1")
public class SendController {
public static Queue<String> queue = new ConcurrentLinkedQueue<>();//ok
public static Queue<String> queue = new LinkedList<>();//will have null value in it and can't poll from it
@GetMapping(value = "/produce")
public String pro() {
String s = UUID.randomUUID().toString();
queue.add(s);
return "true";
}
@GetMapping(value = "/consume")
public String con() {
if(queue.size() == 0) {
return null;
}
return queue.poll();
}
java linked-list queue thread-safety
java linked-list queue thread-safety
edited Jan 3 at 8:07
teek
asked Jan 3 at 4:12
teekteek
307215
307215
3
Please read How to Ask and provide a Minimal, Complete, and Verifiable example.
– Rafael
Jan 3 at 4:16
add a comment |
3
Please read How to Ask and provide a Minimal, Complete, and Verifiable example.
– Rafael
Jan 3 at 4:16
3
3
Please read How to Ask and provide a Minimal, Complete, and Verifiable example.
– Rafael
Jan 3 at 4:16
Please read How to Ask and provide a Minimal, Complete, and Verifiable example.
– Rafael
Jan 3 at 4:16
add a comment |
1 Answer
1
active
oldest
votes
Poll method return null if queue is empty and it can poll null element also.Why null elements are coming within the queue it is strange
package test;
import java.util.LinkedList;
public class TestLL {
public static void main(String args) {
// TODO Auto-generated method stub
LinkedList<String> ll = new LinkedList<>();
System.out.println(ll.poll());
System.out.println(ll.size());
String s = "abc";
ll.add(s);
s = null;
ll.add("cde");
ll.add("efg");
System.out.println(ll.size());
String pollStr = ll.poll();
System.out.println("pollStr=" + pollStr);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println();
ll.add(null);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.size());
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54016258%2fconcurrent-in-queue-of-linkedlist%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Poll method return null if queue is empty and it can poll null element also.Why null elements are coming within the queue it is strange
package test;
import java.util.LinkedList;
public class TestLL {
public static void main(String args) {
// TODO Auto-generated method stub
LinkedList<String> ll = new LinkedList<>();
System.out.println(ll.poll());
System.out.println(ll.size());
String s = "abc";
ll.add(s);
s = null;
ll.add("cde");
ll.add("efg");
System.out.println(ll.size());
String pollStr = ll.poll();
System.out.println("pollStr=" + pollStr);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println();
ll.add(null);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.size());
}
}
add a comment |
Poll method return null if queue is empty and it can poll null element also.Why null elements are coming within the queue it is strange
package test;
import java.util.LinkedList;
public class TestLL {
public static void main(String args) {
// TODO Auto-generated method stub
LinkedList<String> ll = new LinkedList<>();
System.out.println(ll.poll());
System.out.println(ll.size());
String s = "abc";
ll.add(s);
s = null;
ll.add("cde");
ll.add("efg");
System.out.println(ll.size());
String pollStr = ll.poll();
System.out.println("pollStr=" + pollStr);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println();
ll.add(null);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.size());
}
}
add a comment |
Poll method return null if queue is empty and it can poll null element also.Why null elements are coming within the queue it is strange
package test;
import java.util.LinkedList;
public class TestLL {
public static void main(String args) {
// TODO Auto-generated method stub
LinkedList<String> ll = new LinkedList<>();
System.out.println(ll.poll());
System.out.println(ll.size());
String s = "abc";
ll.add(s);
s = null;
ll.add("cde");
ll.add("efg");
System.out.println(ll.size());
String pollStr = ll.poll();
System.out.println("pollStr=" + pollStr);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println();
ll.add(null);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.size());
}
}
Poll method return null if queue is empty and it can poll null element also.Why null elements are coming within the queue it is strange
package test;
import java.util.LinkedList;
public class TestLL {
public static void main(String args) {
// TODO Auto-generated method stub
LinkedList<String> ll = new LinkedList<>();
System.out.println(ll.poll());
System.out.println(ll.size());
String s = "abc";
ll.add(s);
s = null;
ll.add("cde");
ll.add("efg");
System.out.println(ll.size());
String pollStr = ll.poll();
System.out.println("pollStr=" + pollStr);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println(ll.poll());
System.out.println();
ll.add(null);
System.out.println(ll.size());
System.out.println(ll.poll());
System.out.println(ll.size());
}
}
edited Jan 3 at 6:24


Farhana
2,67752335
2,67752335
answered Jan 3 at 5:53
vivekdubeyvivekdubey
1516
1516
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54016258%2fconcurrent-in-queue-of-linkedlist%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
t 8,NHB9T7bDNLRGJiL4s apX8s7 hU1fDmyX,yj9Fg,kb9g
3
Please read How to Ask and provide a Minimal, Complete, and Verifiable example.
– Rafael
Jan 3 at 4:16