How to correctly connect SQLite with textfields and labels in JavaFX?
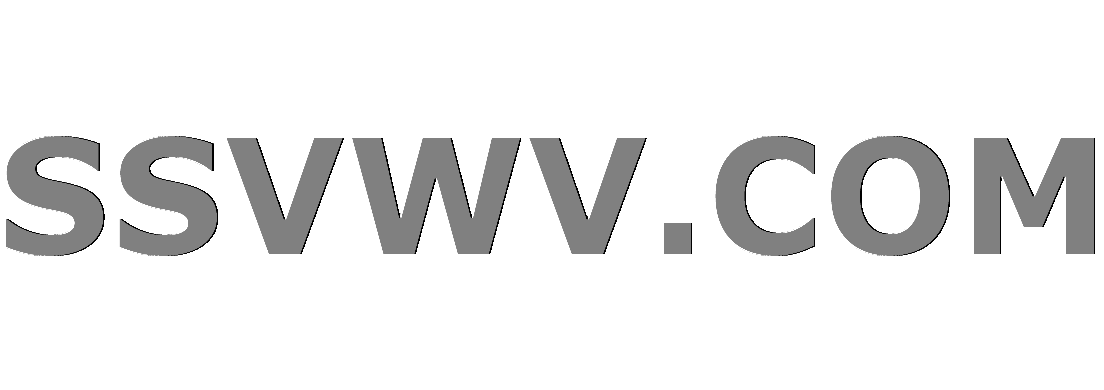
Multi tool use
I'm making an application with JavaFX, Scene Builder and SQLite. For managing SQLite database I'm using DB Browser
I have 3 fields in SQLite: ID, question, answer
When I press on Button "Add" a method is called and sends text from textaria with question to question tab in SQLite and text from textaria with answer do the same.
ID is a number and is autoincremented when I add these fields to SQLite
I successfully sent data from my window but I don't understand how to get data from SQlite and set it to label and combobox in my window
QuestController:
package card;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import java.net.URL;
import java.sql.*;
import java.util.ResourceBundle;
public class QuestController implements Initializable {
@FXML private TextArea ta_questText, ta_answerText;
@FXML private Label lb_numberQuest;
@FXML
private ComboBox<?> idQuest;
@Override
public void initialize(URL location, ResourceBundle resources) {
//register QuestController in Context Class
Context.getInstance().setQuestController(this);
}
@FXML
void addCard(ActionEvent event) {
PreparedStatement preparedStatement;
ResultSet resultSet;
String query = "select * from Cards where ID = ? and question = ? and
answer = ?";
Connection connection = DbConnection.getInstance().getConnection();
try {
String question = ta_questText.getText();
String answer = ta_answerText.getText();
Statement statement = connection.createStatement();
int status = statement.executeUpdate("insert into Cards (question,
answer) values ('"+question+"','"+answer+"')");
if (status > 0) {
System.out.println("question registered");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
DbConnection class:
package card;
import org.sqlite.SQLiteConnection;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DbConnection {
private DbConnection() {
}
public static DbConnection getInstance() {
return new DbConnection();
}
public Connection getConnection() {
String connect_string = "jdbc:sqlite:database.db";
Connection connection = null;
try {
Class.forName("org.sqlite.JDBC");
connection = DriverManager.getConnection("jdbc:sqlite:database.db");
} catch (SQLException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return connection;
}
}
How can I get all existing ID(numbers) from SQLite and put it to combobox?
How can choose a number(ID) and apply number to a label?
if I choose any number from combobox
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
java sqlite javafx scenebuilder
|
show 10 more comments
I'm making an application with JavaFX, Scene Builder and SQLite. For managing SQLite database I'm using DB Browser
I have 3 fields in SQLite: ID, question, answer
When I press on Button "Add" a method is called and sends text from textaria with question to question tab in SQLite and text from textaria with answer do the same.
ID is a number and is autoincremented when I add these fields to SQLite
I successfully sent data from my window but I don't understand how to get data from SQlite and set it to label and combobox in my window
QuestController:
package card;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import java.net.URL;
import java.sql.*;
import java.util.ResourceBundle;
public class QuestController implements Initializable {
@FXML private TextArea ta_questText, ta_answerText;
@FXML private Label lb_numberQuest;
@FXML
private ComboBox<?> idQuest;
@Override
public void initialize(URL location, ResourceBundle resources) {
//register QuestController in Context Class
Context.getInstance().setQuestController(this);
}
@FXML
void addCard(ActionEvent event) {
PreparedStatement preparedStatement;
ResultSet resultSet;
String query = "select * from Cards where ID = ? and question = ? and
answer = ?";
Connection connection = DbConnection.getInstance().getConnection();
try {
String question = ta_questText.getText();
String answer = ta_answerText.getText();
Statement statement = connection.createStatement();
int status = statement.executeUpdate("insert into Cards (question,
answer) values ('"+question+"','"+answer+"')");
if (status > 0) {
System.out.println("question registered");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
DbConnection class:
package card;
import org.sqlite.SQLiteConnection;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DbConnection {
private DbConnection() {
}
public static DbConnection getInstance() {
return new DbConnection();
}
public Connection getConnection() {
String connect_string = "jdbc:sqlite:database.db";
Connection connection = null;
try {
Class.forName("org.sqlite.JDBC");
connection = DriverManager.getConnection("jdbc:sqlite:database.db");
} catch (SQLException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return connection;
}
}
How can I get all existing ID(numbers) from SQLite and put it to combobox?
How can choose a number(ID) and apply number to a label?
if I choose any number from combobox
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
java sqlite javafx scenebuilder
I strongly recommend you look into the MCV pattern. You will definitely run into frustration in the future if you continue with your current model.
– Zephyr
Jan 2 at 6:39
You could start by creating aQuestion
class that contains your question'sid
,question
andanswer
. Then create a list ofQuestion
objects, fill the list from the database, and go from there.
– Zephyr
Jan 2 at 6:40
but user will add questions and answers by himself in application.Text goes to database from textarias.
– kentforth
Jan 2 at 6:47
Does that mean that having a Controller and it's FXML is bad practice?
– kentforth
Jan 2 at 6:52
You'll need to query your database by submitting query statements to SQLite via your JDBC connection. The documentation for your JDBC driver should document which classes you use to create and submit SQL statements. The results of your queries generally come back to your application as a ResultSet object. There's nothing wrong with SQLite, but it's not a Java database. You should consider HSQLCB (hsqldb.org) which is 100% Java and has a very mature JDBC driver with excellent documentation.
– scottb
Jan 2 at 6:56
|
show 10 more comments
I'm making an application with JavaFX, Scene Builder and SQLite. For managing SQLite database I'm using DB Browser
I have 3 fields in SQLite: ID, question, answer
When I press on Button "Add" a method is called and sends text from textaria with question to question tab in SQLite and text from textaria with answer do the same.
ID is a number and is autoincremented when I add these fields to SQLite
I successfully sent data from my window but I don't understand how to get data from SQlite and set it to label and combobox in my window
QuestController:
package card;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import java.net.URL;
import java.sql.*;
import java.util.ResourceBundle;
public class QuestController implements Initializable {
@FXML private TextArea ta_questText, ta_answerText;
@FXML private Label lb_numberQuest;
@FXML
private ComboBox<?> idQuest;
@Override
public void initialize(URL location, ResourceBundle resources) {
//register QuestController in Context Class
Context.getInstance().setQuestController(this);
}
@FXML
void addCard(ActionEvent event) {
PreparedStatement preparedStatement;
ResultSet resultSet;
String query = "select * from Cards where ID = ? and question = ? and
answer = ?";
Connection connection = DbConnection.getInstance().getConnection();
try {
String question = ta_questText.getText();
String answer = ta_answerText.getText();
Statement statement = connection.createStatement();
int status = statement.executeUpdate("insert into Cards (question,
answer) values ('"+question+"','"+answer+"')");
if (status > 0) {
System.out.println("question registered");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
DbConnection class:
package card;
import org.sqlite.SQLiteConnection;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DbConnection {
private DbConnection() {
}
public static DbConnection getInstance() {
return new DbConnection();
}
public Connection getConnection() {
String connect_string = "jdbc:sqlite:database.db";
Connection connection = null;
try {
Class.forName("org.sqlite.JDBC");
connection = DriverManager.getConnection("jdbc:sqlite:database.db");
} catch (SQLException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return connection;
}
}
How can I get all existing ID(numbers) from SQLite and put it to combobox?
How can choose a number(ID) and apply number to a label?
if I choose any number from combobox
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
java sqlite javafx scenebuilder
I'm making an application with JavaFX, Scene Builder and SQLite. For managing SQLite database I'm using DB Browser
I have 3 fields in SQLite: ID, question, answer
When I press on Button "Add" a method is called and sends text from textaria with question to question tab in SQLite and text from textaria with answer do the same.
ID is a number and is autoincremented when I add these fields to SQLite
I successfully sent data from my window but I don't understand how to get data from SQlite and set it to label and combobox in my window
QuestController:
package card;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import java.net.URL;
import java.sql.*;
import java.util.ResourceBundle;
public class QuestController implements Initializable {
@FXML private TextArea ta_questText, ta_answerText;
@FXML private Label lb_numberQuest;
@FXML
private ComboBox<?> idQuest;
@Override
public void initialize(URL location, ResourceBundle resources) {
//register QuestController in Context Class
Context.getInstance().setQuestController(this);
}
@FXML
void addCard(ActionEvent event) {
PreparedStatement preparedStatement;
ResultSet resultSet;
String query = "select * from Cards where ID = ? and question = ? and
answer = ?";
Connection connection = DbConnection.getInstance().getConnection();
try {
String question = ta_questText.getText();
String answer = ta_answerText.getText();
Statement statement = connection.createStatement();
int status = statement.executeUpdate("insert into Cards (question,
answer) values ('"+question+"','"+answer+"')");
if (status > 0) {
System.out.println("question registered");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
DbConnection class:
package card;
import org.sqlite.SQLiteConnection;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DbConnection {
private DbConnection() {
}
public static DbConnection getInstance() {
return new DbConnection();
}
public Connection getConnection() {
String connect_string = "jdbc:sqlite:database.db";
Connection connection = null;
try {
Class.forName("org.sqlite.JDBC");
connection = DriverManager.getConnection("jdbc:sqlite:database.db");
} catch (SQLException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return connection;
}
}
How can I get all existing ID(numbers) from SQLite and put it to combobox?
How can choose a number(ID) and apply number to a label?
if I choose any number from combobox
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
java sqlite javafx scenebuilder
java sqlite javafx scenebuilder
asked Jan 2 at 6:33
kentforthkentforth
6618
6618
I strongly recommend you look into the MCV pattern. You will definitely run into frustration in the future if you continue with your current model.
– Zephyr
Jan 2 at 6:39
You could start by creating aQuestion
class that contains your question'sid
,question
andanswer
. Then create a list ofQuestion
objects, fill the list from the database, and go from there.
– Zephyr
Jan 2 at 6:40
but user will add questions and answers by himself in application.Text goes to database from textarias.
– kentforth
Jan 2 at 6:47
Does that mean that having a Controller and it's FXML is bad practice?
– kentforth
Jan 2 at 6:52
You'll need to query your database by submitting query statements to SQLite via your JDBC connection. The documentation for your JDBC driver should document which classes you use to create and submit SQL statements. The results of your queries generally come back to your application as a ResultSet object. There's nothing wrong with SQLite, but it's not a Java database. You should consider HSQLCB (hsqldb.org) which is 100% Java and has a very mature JDBC driver with excellent documentation.
– scottb
Jan 2 at 6:56
|
show 10 more comments
I strongly recommend you look into the MCV pattern. You will definitely run into frustration in the future if you continue with your current model.
– Zephyr
Jan 2 at 6:39
You could start by creating aQuestion
class that contains your question'sid
,question
andanswer
. Then create a list ofQuestion
objects, fill the list from the database, and go from there.
– Zephyr
Jan 2 at 6:40
but user will add questions and answers by himself in application.Text goes to database from textarias.
– kentforth
Jan 2 at 6:47
Does that mean that having a Controller and it's FXML is bad practice?
– kentforth
Jan 2 at 6:52
You'll need to query your database by submitting query statements to SQLite via your JDBC connection. The documentation for your JDBC driver should document which classes you use to create and submit SQL statements. The results of your queries generally come back to your application as a ResultSet object. There's nothing wrong with SQLite, but it's not a Java database. You should consider HSQLCB (hsqldb.org) which is 100% Java and has a very mature JDBC driver with excellent documentation.
– scottb
Jan 2 at 6:56
I strongly recommend you look into the MCV pattern. You will definitely run into frustration in the future if you continue with your current model.
– Zephyr
Jan 2 at 6:39
I strongly recommend you look into the MCV pattern. You will definitely run into frustration in the future if you continue with your current model.
– Zephyr
Jan 2 at 6:39
You could start by creating a
Question
class that contains your question's id
, question
and answer
. Then create a list of Question
objects, fill the list from the database, and go from there.– Zephyr
Jan 2 at 6:40
You could start by creating a
Question
class that contains your question's id
, question
and answer
. Then create a list of Question
objects, fill the list from the database, and go from there.– Zephyr
Jan 2 at 6:40
but user will add questions and answers by himself in application.Text goes to database from textarias.
– kentforth
Jan 2 at 6:47
but user will add questions and answers by himself in application.Text goes to database from textarias.
– kentforth
Jan 2 at 6:47
Does that mean that having a Controller and it's FXML is bad practice?
– kentforth
Jan 2 at 6:52
Does that mean that having a Controller and it's FXML is bad practice?
– kentforth
Jan 2 at 6:52
You'll need to query your database by submitting query statements to SQLite via your JDBC connection. The documentation for your JDBC driver should document which classes you use to create and submit SQL statements. The results of your queries generally come back to your application as a ResultSet object. There's nothing wrong with SQLite, but it's not a Java database. You should consider HSQLCB (hsqldb.org) which is 100% Java and has a very mature JDBC driver with excellent documentation.
– scottb
Jan 2 at 6:56
You'll need to query your database by submitting query statements to SQLite via your JDBC connection. The documentation for your JDBC driver should document which classes you use to create and submit SQL statements. The results of your queries generally come back to your application as a ResultSet object. There's nothing wrong with SQLite, but it's not a Java database. You should consider HSQLCB (hsqldb.org) which is 100% Java and has a very mature JDBC driver with excellent documentation.
– scottb
Jan 2 at 6:56
|
show 10 more comments
1 Answer
1
active
oldest
votes
Just like the comments in your post, I highly suggest breaking down your codes into layers for better manageability. Good job on starting with your DbConnection
class. Additionally, JavaFx already setup some layer for you to start on.
These are the:
View: your FXML file that's created on scene builder
Controller: the JFx controller that FXML forces you to use
Now it is up to you to add more layers to manage your code. I will suggest starting with these:
Model: this will be the main data structure you will work on. For starters, maybe you can follow the structure of your database? Example:class Card
with fieldsid
,question
, andanswer
.
Persistence: this Java class will hold your SQL code. This is also responsible for converting theResultSet
object to your model object.
Then finally, keep in mind that your are working with layers. Make sure that their interactions don't leak.
View (FXML) <--> Controller + Model <--> Persistence + Model
To answer your questions:
How can I get all existing ID(numbers) from SQLite and put it to combobox?
Perform an SQL SELECT using your SQLite connection to fetch all the
id
s. (SELECT id FROM cards
perhaps?)On successful
SELECT
call, iterate through theResultSet
object. Each iteration should fetch the data fromid
column, convert it to string (or whatever type your combobox accepts), then add them all. (something like this:comboBox.getItems().add(id)
)
How can choose a number(ID) and apply number to a label?
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
Perform an SQL SELECT using your connection, this time add a WHERE clause in the statement to filter results. Since there is a dynamic part in your SQL now, using
PreparedStatement
will be good. Example:SELECT id, question, answer FROM card WHERE id=?
Using the results of the SQL calls, assign them to the proper JFx Components such as labels and text areas.
thanx for reply! I wll go and learn MVC pattern and try to apply all crap from SQLite to my labels and text)
– kentforth
Jan 2 at 11:12
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54002182%2fhow-to-correctly-connect-sqlite-with-textfields-and-labels-in-javafx%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Just like the comments in your post, I highly suggest breaking down your codes into layers for better manageability. Good job on starting with your DbConnection
class. Additionally, JavaFx already setup some layer for you to start on.
These are the:
View: your FXML file that's created on scene builder
Controller: the JFx controller that FXML forces you to use
Now it is up to you to add more layers to manage your code. I will suggest starting with these:
Model: this will be the main data structure you will work on. For starters, maybe you can follow the structure of your database? Example:class Card
with fieldsid
,question
, andanswer
.
Persistence: this Java class will hold your SQL code. This is also responsible for converting theResultSet
object to your model object.
Then finally, keep in mind that your are working with layers. Make sure that their interactions don't leak.
View (FXML) <--> Controller + Model <--> Persistence + Model
To answer your questions:
How can I get all existing ID(numbers) from SQLite and put it to combobox?
Perform an SQL SELECT using your SQLite connection to fetch all the
id
s. (SELECT id FROM cards
perhaps?)On successful
SELECT
call, iterate through theResultSet
object. Each iteration should fetch the data fromid
column, convert it to string (or whatever type your combobox accepts), then add them all. (something like this:comboBox.getItems().add(id)
)
How can choose a number(ID) and apply number to a label?
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
Perform an SQL SELECT using your connection, this time add a WHERE clause in the statement to filter results. Since there is a dynamic part in your SQL now, using
PreparedStatement
will be good. Example:SELECT id, question, answer FROM card WHERE id=?
Using the results of the SQL calls, assign them to the proper JFx Components such as labels and text areas.
thanx for reply! I wll go and learn MVC pattern and try to apply all crap from SQLite to my labels and text)
– kentforth
Jan 2 at 11:12
add a comment |
Just like the comments in your post, I highly suggest breaking down your codes into layers for better manageability. Good job on starting with your DbConnection
class. Additionally, JavaFx already setup some layer for you to start on.
These are the:
View: your FXML file that's created on scene builder
Controller: the JFx controller that FXML forces you to use
Now it is up to you to add more layers to manage your code. I will suggest starting with these:
Model: this will be the main data structure you will work on. For starters, maybe you can follow the structure of your database? Example:class Card
with fieldsid
,question
, andanswer
.
Persistence: this Java class will hold your SQL code. This is also responsible for converting theResultSet
object to your model object.
Then finally, keep in mind that your are working with layers. Make sure that their interactions don't leak.
View (FXML) <--> Controller + Model <--> Persistence + Model
To answer your questions:
How can I get all existing ID(numbers) from SQLite and put it to combobox?
Perform an SQL SELECT using your SQLite connection to fetch all the
id
s. (SELECT id FROM cards
perhaps?)On successful
SELECT
call, iterate through theResultSet
object. Each iteration should fetch the data fromid
column, convert it to string (or whatever type your combobox accepts), then add them all. (something like this:comboBox.getItems().add(id)
)
How can choose a number(ID) and apply number to a label?
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
Perform an SQL SELECT using your connection, this time add a WHERE clause in the statement to filter results. Since there is a dynamic part in your SQL now, using
PreparedStatement
will be good. Example:SELECT id, question, answer FROM card WHERE id=?
Using the results of the SQL calls, assign them to the proper JFx Components such as labels and text areas.
thanx for reply! I wll go and learn MVC pattern and try to apply all crap from SQLite to my labels and text)
– kentforth
Jan 2 at 11:12
add a comment |
Just like the comments in your post, I highly suggest breaking down your codes into layers for better manageability. Good job on starting with your DbConnection
class. Additionally, JavaFx already setup some layer for you to start on.
These are the:
View: your FXML file that's created on scene builder
Controller: the JFx controller that FXML forces you to use
Now it is up to you to add more layers to manage your code. I will suggest starting with these:
Model: this will be the main data structure you will work on. For starters, maybe you can follow the structure of your database? Example:class Card
with fieldsid
,question
, andanswer
.
Persistence: this Java class will hold your SQL code. This is also responsible for converting theResultSet
object to your model object.
Then finally, keep in mind that your are working with layers. Make sure that their interactions don't leak.
View (FXML) <--> Controller + Model <--> Persistence + Model
To answer your questions:
How can I get all existing ID(numbers) from SQLite and put it to combobox?
Perform an SQL SELECT using your SQLite connection to fetch all the
id
s. (SELECT id FROM cards
perhaps?)On successful
SELECT
call, iterate through theResultSet
object. Each iteration should fetch the data fromid
column, convert it to string (or whatever type your combobox accepts), then add them all. (something like this:comboBox.getItems().add(id)
)
How can choose a number(ID) and apply number to a label?
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
Perform an SQL SELECT using your connection, this time add a WHERE clause in the statement to filter results. Since there is a dynamic part in your SQL now, using
PreparedStatement
will be good. Example:SELECT id, question, answer FROM card WHERE id=?
Using the results of the SQL calls, assign them to the proper JFx Components such as labels and text areas.
Just like the comments in your post, I highly suggest breaking down your codes into layers for better manageability. Good job on starting with your DbConnection
class. Additionally, JavaFx already setup some layer for you to start on.
These are the:
View: your FXML file that's created on scene builder
Controller: the JFx controller that FXML forces you to use
Now it is up to you to add more layers to manage your code. I will suggest starting with these:
Model: this will be the main data structure you will work on. For starters, maybe you can follow the structure of your database? Example:class Card
with fieldsid
,question
, andanswer
.
Persistence: this Java class will hold your SQL code. This is also responsible for converting theResultSet
object to your model object.
Then finally, keep in mind that your are working with layers. Make sure that their interactions don't leak.
View (FXML) <--> Controller + Model <--> Persistence + Model
To answer your questions:
How can I get all existing ID(numbers) from SQLite and put it to combobox?
Perform an SQL SELECT using your SQLite connection to fetch all the
id
s. (SELECT id FROM cards
perhaps?)On successful
SELECT
call, iterate through theResultSet
object. Each iteration should fetch the data fromid
column, convert it to string (or whatever type your combobox accepts), then add them all. (something like this:comboBox.getItems().add(id)
)
How can choose a number(ID) and apply number to a label?
How can I apply text with question and answer from SQLite connected to that number to textarias in my window?
Perform an SQL SELECT using your connection, this time add a WHERE clause in the statement to filter results. Since there is a dynamic part in your SQL now, using
PreparedStatement
will be good. Example:SELECT id, question, answer FROM card WHERE id=?
Using the results of the SQL calls, assign them to the proper JFx Components such as labels and text areas.
edited Jan 2 at 11:18
answered Jan 2 at 10:44
KaNa0011KaNa0011
615614
615614
thanx for reply! I wll go and learn MVC pattern and try to apply all crap from SQLite to my labels and text)
– kentforth
Jan 2 at 11:12
add a comment |
thanx for reply! I wll go and learn MVC pattern and try to apply all crap from SQLite to my labels and text)
– kentforth
Jan 2 at 11:12
thanx for reply! I wll go and learn MVC pattern and try to apply all crap from SQLite to my labels and text)
– kentforth
Jan 2 at 11:12
thanx for reply! I wll go and learn MVC pattern and try to apply all crap from SQLite to my labels and text)
– kentforth
Jan 2 at 11:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54002182%2fhow-to-correctly-connect-sqlite-with-textfields-and-labels-in-javafx%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pbO E8KcQrk8StUkYf0grMSD0LTCFheHpXDTC18,MPBybrks7 Ga,G6QxFX0JevV4ow4w Zcbi Ean7kpXPuy
I strongly recommend you look into the MCV pattern. You will definitely run into frustration in the future if you continue with your current model.
– Zephyr
Jan 2 at 6:39
You could start by creating a
Question
class that contains your question'sid
,question
andanswer
. Then create a list ofQuestion
objects, fill the list from the database, and go from there.– Zephyr
Jan 2 at 6:40
but user will add questions and answers by himself in application.Text goes to database from textarias.
– kentforth
Jan 2 at 6:47
Does that mean that having a Controller and it's FXML is bad practice?
– kentforth
Jan 2 at 6:52
You'll need to query your database by submitting query statements to SQLite via your JDBC connection. The documentation for your JDBC driver should document which classes you use to create and submit SQL statements. The results of your queries generally come back to your application as a ResultSet object. There's nothing wrong with SQLite, but it's not a Java database. You should consider HSQLCB (hsqldb.org) which is 100% Java and has a very mature JDBC driver with excellent documentation.
– scottb
Jan 2 at 6:56