Failed to read data using reinterpret_cast in C++
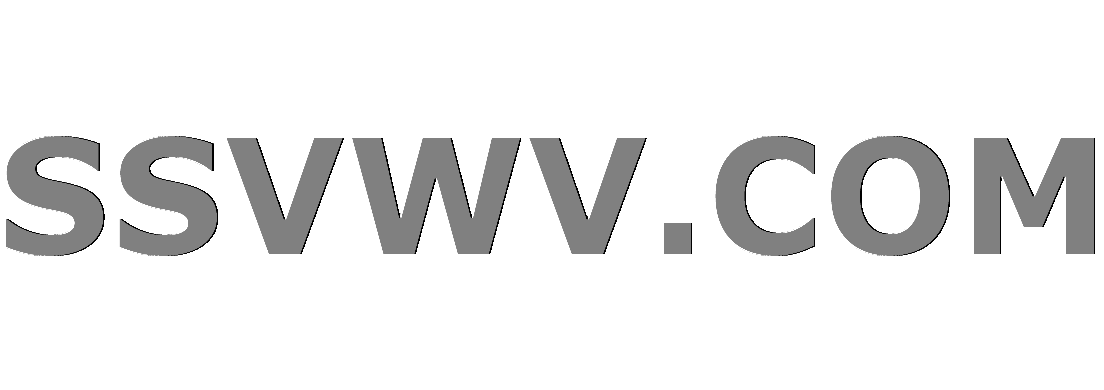
Multi tool use
#include <iostream>
#include <vector>
#include <fstream>
using namespace std;
int main(){
// write file
string file_name = "random_fstream.dat";
ofstream file(file_name, ios_base::out | ios_base::binary);
if (!file) {return EXIT_FAILURE;}
int r = 2;
int c = 3;
file.write(reinterpret_cast<char*>(&r),sizeof(r));
file.write(reinterpret_cast<char*>(&c),sizeof(c));
//------------------------------------------------
// read file
ifstream in(file_name, ios_base::in | ios_base::binary);
if (!in) {return EXIT_FAILURE;}
int x = 0;
int y = 0;
in.read(reinterpret_cast<char*>(&x),sizeof(x));
in.read(reinterpret_cast<char*>(&y),sizeof(y));
cout << x << y << endl;
return 0;
}
I was trying to practice write and read file in C++. First step was to write int value 2 and 3 into the file with binary form. Second step was to read the two number from that file.
Then I got x and y valued both equal to 0, but I expected to receive x=2
and y=3
. Where did I do wrong?
c++ file c++11 fstream reinterpret-cast
add a comment |
#include <iostream>
#include <vector>
#include <fstream>
using namespace std;
int main(){
// write file
string file_name = "random_fstream.dat";
ofstream file(file_name, ios_base::out | ios_base::binary);
if (!file) {return EXIT_FAILURE;}
int r = 2;
int c = 3;
file.write(reinterpret_cast<char*>(&r),sizeof(r));
file.write(reinterpret_cast<char*>(&c),sizeof(c));
//------------------------------------------------
// read file
ifstream in(file_name, ios_base::in | ios_base::binary);
if (!in) {return EXIT_FAILURE;}
int x = 0;
int y = 0;
in.read(reinterpret_cast<char*>(&x),sizeof(x));
in.read(reinterpret_cast<char*>(&y),sizeof(y));
cout << x << y << endl;
return 0;
}
I was trying to practice write and read file in C++. First step was to write int value 2 and 3 into the file with binary form. Second step was to read the two number from that file.
Then I got x and y valued both equal to 0, but I expected to receive x=2
and y=3
. Where did I do wrong?
c++ file c++11 fstream reinterpret-cast
1
Are you deliberately trying to read from the same file while it has not been closed after the write operations? Or is that an oversight?
– R Sahu
Jan 2 at 6:25
1
Also, you are reading intox
twice instead of reading intox
andy
.
– R Sahu
Jan 2 at 6:26
Yes, that was a typo.. Let me edit it now.
– ysgc
Jan 2 at 18:28
add a comment |
#include <iostream>
#include <vector>
#include <fstream>
using namespace std;
int main(){
// write file
string file_name = "random_fstream.dat";
ofstream file(file_name, ios_base::out | ios_base::binary);
if (!file) {return EXIT_FAILURE;}
int r = 2;
int c = 3;
file.write(reinterpret_cast<char*>(&r),sizeof(r));
file.write(reinterpret_cast<char*>(&c),sizeof(c));
//------------------------------------------------
// read file
ifstream in(file_name, ios_base::in | ios_base::binary);
if (!in) {return EXIT_FAILURE;}
int x = 0;
int y = 0;
in.read(reinterpret_cast<char*>(&x),sizeof(x));
in.read(reinterpret_cast<char*>(&y),sizeof(y));
cout << x << y << endl;
return 0;
}
I was trying to practice write and read file in C++. First step was to write int value 2 and 3 into the file with binary form. Second step was to read the two number from that file.
Then I got x and y valued both equal to 0, but I expected to receive x=2
and y=3
. Where did I do wrong?
c++ file c++11 fstream reinterpret-cast
#include <iostream>
#include <vector>
#include <fstream>
using namespace std;
int main(){
// write file
string file_name = "random_fstream.dat";
ofstream file(file_name, ios_base::out | ios_base::binary);
if (!file) {return EXIT_FAILURE;}
int r = 2;
int c = 3;
file.write(reinterpret_cast<char*>(&r),sizeof(r));
file.write(reinterpret_cast<char*>(&c),sizeof(c));
//------------------------------------------------
// read file
ifstream in(file_name, ios_base::in | ios_base::binary);
if (!in) {return EXIT_FAILURE;}
int x = 0;
int y = 0;
in.read(reinterpret_cast<char*>(&x),sizeof(x));
in.read(reinterpret_cast<char*>(&y),sizeof(y));
cout << x << y << endl;
return 0;
}
I was trying to practice write and read file in C++. First step was to write int value 2 and 3 into the file with binary form. Second step was to read the two number from that file.
Then I got x and y valued both equal to 0, but I expected to receive x=2
and y=3
. Where did I do wrong?
c++ file c++11 fstream reinterpret-cast
c++ file c++11 fstream reinterpret-cast
edited Jan 2 at 18:28
ysgc
asked Jan 2 at 6:23


ysgcysgc
32
32
1
Are you deliberately trying to read from the same file while it has not been closed after the write operations? Or is that an oversight?
– R Sahu
Jan 2 at 6:25
1
Also, you are reading intox
twice instead of reading intox
andy
.
– R Sahu
Jan 2 at 6:26
Yes, that was a typo.. Let me edit it now.
– ysgc
Jan 2 at 18:28
add a comment |
1
Are you deliberately trying to read from the same file while it has not been closed after the write operations? Or is that an oversight?
– R Sahu
Jan 2 at 6:25
1
Also, you are reading intox
twice instead of reading intox
andy
.
– R Sahu
Jan 2 at 6:26
Yes, that was a typo.. Let me edit it now.
– ysgc
Jan 2 at 18:28
1
1
Are you deliberately trying to read from the same file while it has not been closed after the write operations? Or is that an oversight?
– R Sahu
Jan 2 at 6:25
Are you deliberately trying to read from the same file while it has not been closed after the write operations? Or is that an oversight?
– R Sahu
Jan 2 at 6:25
1
1
Also, you are reading into
x
twice instead of reading into x
and y
.– R Sahu
Jan 2 at 6:26
Also, you are reading into
x
twice instead of reading into x
and y
.– R Sahu
Jan 2 at 6:26
Yes, that was a typo.. Let me edit it now.
– ysgc
Jan 2 at 18:28
Yes, that was a typo.. Let me edit it now.
– ysgc
Jan 2 at 18:28
add a comment |
1 Answer
1
active
oldest
votes
As have been pointed out in comments, you have at least two bugs:
The first problem is in your reads: both your reads use the address of x
and the size of y
, which I'm sure is just a typo.
Second, you have to close()
the file you opened for writing (or at least, flush your writes (sync()
) before reading that same data again, but that needs a whole lot of caution and special rules.) Anyways, you need to close the file, either by letting the handle (file
in this case) fall out of scope and getting its destructor called, or calling file.close()
yourself (after the writes.)
To prevent this problem, you generally have to check for errors every time you do any I/O. To do that, you can use the name of your file handle like a boolean variable, e.g.:
in.read(reinterpret_cast<char*>(&x),sizeof(x));
if (!in)
cerr << "First read failed." << endl;
in.read(reinterpret_cast<char*>(&y),sizeof(y));
if (!in)
cout << "Second read failed." << endl;
I you want to know what actually is going on, the class std::istream
has an explicit conversion to bool
that returns whether the last operation succeeded or not (or whether the stream is in a readable/good condition or not.) There is also a host of member functions defined for C++ streams (both input and output) that help you check for errors, the end of a stream, etc., e.g. bad()
, good()
, fail()
, eof()
, etc.
Again, just remember that any I/O operation can fail at any time for various reasons, so check its status!
"file.close()" solved my problem. Thanks a lot!
– ysgc
Jan 2 at 18:27
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54002083%2ffailed-to-read-data-using-reinterpret-castchar-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
As have been pointed out in comments, you have at least two bugs:
The first problem is in your reads: both your reads use the address of x
and the size of y
, which I'm sure is just a typo.
Second, you have to close()
the file you opened for writing (or at least, flush your writes (sync()
) before reading that same data again, but that needs a whole lot of caution and special rules.) Anyways, you need to close the file, either by letting the handle (file
in this case) fall out of scope and getting its destructor called, or calling file.close()
yourself (after the writes.)
To prevent this problem, you generally have to check for errors every time you do any I/O. To do that, you can use the name of your file handle like a boolean variable, e.g.:
in.read(reinterpret_cast<char*>(&x),sizeof(x));
if (!in)
cerr << "First read failed." << endl;
in.read(reinterpret_cast<char*>(&y),sizeof(y));
if (!in)
cout << "Second read failed." << endl;
I you want to know what actually is going on, the class std::istream
has an explicit conversion to bool
that returns whether the last operation succeeded or not (or whether the stream is in a readable/good condition or not.) There is also a host of member functions defined for C++ streams (both input and output) that help you check for errors, the end of a stream, etc., e.g. bad()
, good()
, fail()
, eof()
, etc.
Again, just remember that any I/O operation can fail at any time for various reasons, so check its status!
"file.close()" solved my problem. Thanks a lot!
– ysgc
Jan 2 at 18:27
add a comment |
As have been pointed out in comments, you have at least two bugs:
The first problem is in your reads: both your reads use the address of x
and the size of y
, which I'm sure is just a typo.
Second, you have to close()
the file you opened for writing (or at least, flush your writes (sync()
) before reading that same data again, but that needs a whole lot of caution and special rules.) Anyways, you need to close the file, either by letting the handle (file
in this case) fall out of scope and getting its destructor called, or calling file.close()
yourself (after the writes.)
To prevent this problem, you generally have to check for errors every time you do any I/O. To do that, you can use the name of your file handle like a boolean variable, e.g.:
in.read(reinterpret_cast<char*>(&x),sizeof(x));
if (!in)
cerr << "First read failed." << endl;
in.read(reinterpret_cast<char*>(&y),sizeof(y));
if (!in)
cout << "Second read failed." << endl;
I you want to know what actually is going on, the class std::istream
has an explicit conversion to bool
that returns whether the last operation succeeded or not (or whether the stream is in a readable/good condition or not.) There is also a host of member functions defined for C++ streams (both input and output) that help you check for errors, the end of a stream, etc., e.g. bad()
, good()
, fail()
, eof()
, etc.
Again, just remember that any I/O operation can fail at any time for various reasons, so check its status!
"file.close()" solved my problem. Thanks a lot!
– ysgc
Jan 2 at 18:27
add a comment |
As have been pointed out in comments, you have at least two bugs:
The first problem is in your reads: both your reads use the address of x
and the size of y
, which I'm sure is just a typo.
Second, you have to close()
the file you opened for writing (or at least, flush your writes (sync()
) before reading that same data again, but that needs a whole lot of caution and special rules.) Anyways, you need to close the file, either by letting the handle (file
in this case) fall out of scope and getting its destructor called, or calling file.close()
yourself (after the writes.)
To prevent this problem, you generally have to check for errors every time you do any I/O. To do that, you can use the name of your file handle like a boolean variable, e.g.:
in.read(reinterpret_cast<char*>(&x),sizeof(x));
if (!in)
cerr << "First read failed." << endl;
in.read(reinterpret_cast<char*>(&y),sizeof(y));
if (!in)
cout << "Second read failed." << endl;
I you want to know what actually is going on, the class std::istream
has an explicit conversion to bool
that returns whether the last operation succeeded or not (or whether the stream is in a readable/good condition or not.) There is also a host of member functions defined for C++ streams (both input and output) that help you check for errors, the end of a stream, etc., e.g. bad()
, good()
, fail()
, eof()
, etc.
Again, just remember that any I/O operation can fail at any time for various reasons, so check its status!
As have been pointed out in comments, you have at least two bugs:
The first problem is in your reads: both your reads use the address of x
and the size of y
, which I'm sure is just a typo.
Second, you have to close()
the file you opened for writing (or at least, flush your writes (sync()
) before reading that same data again, but that needs a whole lot of caution and special rules.) Anyways, you need to close the file, either by letting the handle (file
in this case) fall out of scope and getting its destructor called, or calling file.close()
yourself (after the writes.)
To prevent this problem, you generally have to check for errors every time you do any I/O. To do that, you can use the name of your file handle like a boolean variable, e.g.:
in.read(reinterpret_cast<char*>(&x),sizeof(x));
if (!in)
cerr << "First read failed." << endl;
in.read(reinterpret_cast<char*>(&y),sizeof(y));
if (!in)
cout << "Second read failed." << endl;
I you want to know what actually is going on, the class std::istream
has an explicit conversion to bool
that returns whether the last operation succeeded or not (or whether the stream is in a readable/good condition or not.) There is also a host of member functions defined for C++ streams (both input and output) that help you check for errors, the end of a stream, etc., e.g. bad()
, good()
, fail()
, eof()
, etc.
Again, just remember that any I/O operation can fail at any time for various reasons, so check its status!
edited Jan 2 at 7:13
answered Jan 2 at 6:58
yztyzt
6,36612436
6,36612436
"file.close()" solved my problem. Thanks a lot!
– ysgc
Jan 2 at 18:27
add a comment |
"file.close()" solved my problem. Thanks a lot!
– ysgc
Jan 2 at 18:27
"file.close()" solved my problem. Thanks a lot!
– ysgc
Jan 2 at 18:27
"file.close()" solved my problem. Thanks a lot!
– ysgc
Jan 2 at 18:27
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54002083%2ffailed-to-read-data-using-reinterpret-castchar-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KAKzibyykvC7caQ1taLBrWrk LsF6,5EEfqVkalarLI,U49hDbDjomPv1tt,U,p0VP7 gVWfMMi9 XTr3BG2U6bU5KtuwyLbHgK,EFF
1
Are you deliberately trying to read from the same file while it has not been closed after the write operations? Or is that an oversight?
– R Sahu
Jan 2 at 6:25
1
Also, you are reading into
x
twice instead of reading intox
andy
.– R Sahu
Jan 2 at 6:26
Yes, that was a typo.. Let me edit it now.
– ysgc
Jan 2 at 18:28