Create a hyperlink for every row in dynamic table using AJAX or jQuery
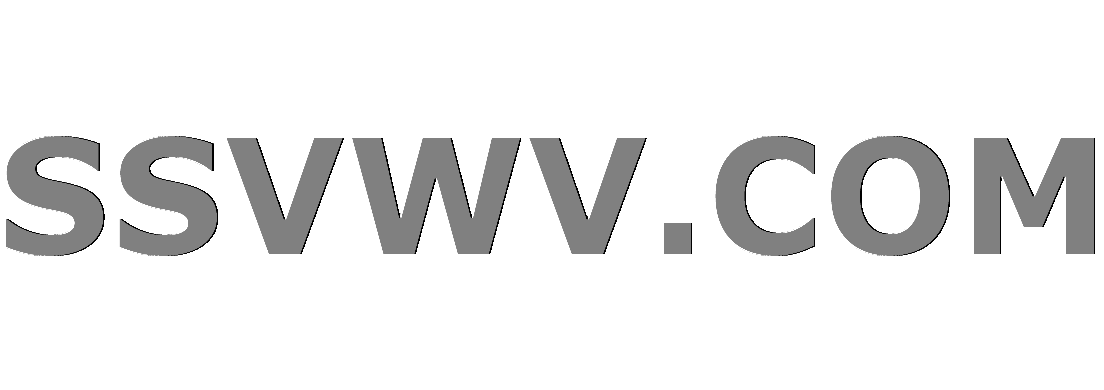
Multi tool use
I need to create the hyperlink for every row of my table created using AJAX.
Pass the value through the URL and fetch it in the other webpage using jQuery or AJAX.
<script>
$(document).ready(function() {
$.getJSON("url here", function(data) {
console.log("data table set");
console.log("json fetching");
for (var i = 0; i < data.length; i++) {
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
tr = $('<tr/>');
tr.append("<td>" + data[i].tName + "</td>");
tr.append("<td>" + data[i].tName + "</td>");
tr.append("<td>" + data[i].prType + "</td>");
$('#table1').append(tr);
console.log("json fetch complete");
$('#table1').DataTable({
paginate: true,
searching: true,
ordering: true,
pageLength: 10,
select: true
});
});
});
</script>
I need a hyperlink for every row in the table and pass one of the table values in the hyperlink.
jquery json ajax
add a comment |
I need to create the hyperlink for every row of my table created using AJAX.
Pass the value through the URL and fetch it in the other webpage using jQuery or AJAX.
<script>
$(document).ready(function() {
$.getJSON("url here", function(data) {
console.log("data table set");
console.log("json fetching");
for (var i = 0; i < data.length; i++) {
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
tr = $('<tr/>');
tr.append("<td>" + data[i].tName + "</td>");
tr.append("<td>" + data[i].tName + "</td>");
tr.append("<td>" + data[i].prType + "</td>");
$('#table1').append(tr);
console.log("json fetch complete");
$('#table1').DataTable({
paginate: true,
searching: true,
ordering: true,
pageLength: 10,
select: true
});
});
});
</script>
I need a hyperlink for every row in the table and pass one of the table values in the hyperlink.
jquery json ajax
add a comment |
I need to create the hyperlink for every row of my table created using AJAX.
Pass the value through the URL and fetch it in the other webpage using jQuery or AJAX.
<script>
$(document).ready(function() {
$.getJSON("url here", function(data) {
console.log("data table set");
console.log("json fetching");
for (var i = 0; i < data.length; i++) {
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
tr = $('<tr/>');
tr.append("<td>" + data[i].tName + "</td>");
tr.append("<td>" + data[i].tName + "</td>");
tr.append("<td>" + data[i].prType + "</td>");
$('#table1').append(tr);
console.log("json fetch complete");
$('#table1').DataTable({
paginate: true,
searching: true,
ordering: true,
pageLength: 10,
select: true
});
});
});
</script>
I need a hyperlink for every row in the table and pass one of the table values in the hyperlink.
jquery json ajax
I need to create the hyperlink for every row of my table created using AJAX.
Pass the value through the URL and fetch it in the other webpage using jQuery or AJAX.
<script>
$(document).ready(function() {
$.getJSON("url here", function(data) {
console.log("data table set");
console.log("json fetching");
for (var i = 0; i < data.length; i++) {
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
tr = $('<tr/>');
tr.append("<td>" + data[i].tName + "</td>");
tr.append("<td>" + data[i].tName + "</td>");
tr.append("<td>" + data[i].prType + "</td>");
$('#table1').append(tr);
console.log("json fetch complete");
$('#table1').DataTable({
paginate: true,
searching: true,
ordering: true,
pageLength: 10,
select: true
});
});
});
</script>
I need a hyperlink for every row in the table and pass one of the table values in the hyperlink.
jquery json ajax
jquery json ajax
edited Jan 2 at 6:56
Shree
12.8k2071124
12.8k2071124
asked Jan 2 at 6:22
immanualimmanual
185
185
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Hyperlinks are specified using anchor tag:
<a href="url_here">Display Name</a>
so in your table, inside a row put this as data in the cell, like
<table>
<tr>
<td><a href="url_here">Display Name</a></td>
</tr>
</table>
this works with JQuery-datatable also.
Use loops to generate this html dynamically if necessary.
If you wish to pass data, use query string to format the url -- this will be a get request.
If you want to make a post request, you will have to add a function to click event and make post request explicitly.
Hope this helps !!
Thanks a lot, it worked....!
– immanual
Jan 2 at 8:45
Welcome!! Glad I could help👍
– Suhas NM
Jan 2 at 8:46
add a comment |
Include underscore Js in your project
<script type="text/template" id="scriptDataTableTemplate">
<tr>
<td><%= tName %></td>
<td><%= pName %></td>
<td><%= pType %></td>
<td class="text-center">
<a href="/Home/List/<%= id %>">Edit</a>
</td>
<tr>
</script>
Create Template like this and give proper id
In your js code use this
var tmpl = _.template($('#scriptDataTableTemplate').html());
for (var i=0; i<data.length; i++){
var id = data[i].Id;
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
var obj = {"id": id , "tName": tName, "pName": pName, "pType": pType};
var html = tmpl(obj);
$('#table1').append(html );
}
$('#table1').DataTable({
paginate: true,
searching:true,
ordering: true,
pageLength: 10,
select: true
});
add a comment |
Wrap tr
with anchor tag
generate invalid HTML. You can add onclick
on tr like below.
<tr onclick="window.location='http://www.yoururl.com?param='+ data[i].tName +';'" > //Or what ever you want to pass on next page
</tr>
Thanks dude.. it really gave an idea to pass the data through url
– immanual
Jan 2 at 8:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54002077%2fcreate-a-hyperlink-for-every-row-in-dynamic-table-using-ajax-or-jquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Hyperlinks are specified using anchor tag:
<a href="url_here">Display Name</a>
so in your table, inside a row put this as data in the cell, like
<table>
<tr>
<td><a href="url_here">Display Name</a></td>
</tr>
</table>
this works with JQuery-datatable also.
Use loops to generate this html dynamically if necessary.
If you wish to pass data, use query string to format the url -- this will be a get request.
If you want to make a post request, you will have to add a function to click event and make post request explicitly.
Hope this helps !!
Thanks a lot, it worked....!
– immanual
Jan 2 at 8:45
Welcome!! Glad I could help👍
– Suhas NM
Jan 2 at 8:46
add a comment |
Hyperlinks are specified using anchor tag:
<a href="url_here">Display Name</a>
so in your table, inside a row put this as data in the cell, like
<table>
<tr>
<td><a href="url_here">Display Name</a></td>
</tr>
</table>
this works with JQuery-datatable also.
Use loops to generate this html dynamically if necessary.
If you wish to pass data, use query string to format the url -- this will be a get request.
If you want to make a post request, you will have to add a function to click event and make post request explicitly.
Hope this helps !!
Thanks a lot, it worked....!
– immanual
Jan 2 at 8:45
Welcome!! Glad I could help👍
– Suhas NM
Jan 2 at 8:46
add a comment |
Hyperlinks are specified using anchor tag:
<a href="url_here">Display Name</a>
so in your table, inside a row put this as data in the cell, like
<table>
<tr>
<td><a href="url_here">Display Name</a></td>
</tr>
</table>
this works with JQuery-datatable also.
Use loops to generate this html dynamically if necessary.
If you wish to pass data, use query string to format the url -- this will be a get request.
If you want to make a post request, you will have to add a function to click event and make post request explicitly.
Hope this helps !!
Hyperlinks are specified using anchor tag:
<a href="url_here">Display Name</a>
so in your table, inside a row put this as data in the cell, like
<table>
<tr>
<td><a href="url_here">Display Name</a></td>
</tr>
</table>
this works with JQuery-datatable also.
Use loops to generate this html dynamically if necessary.
If you wish to pass data, use query string to format the url -- this will be a get request.
If you want to make a post request, you will have to add a function to click event and make post request explicitly.
Hope this helps !!
edited Jan 2 at 6:35
answered Jan 2 at 6:27
Suhas NMSuhas NM
1175
1175
Thanks a lot, it worked....!
– immanual
Jan 2 at 8:45
Welcome!! Glad I could help👍
– Suhas NM
Jan 2 at 8:46
add a comment |
Thanks a lot, it worked....!
– immanual
Jan 2 at 8:45
Welcome!! Glad I could help👍
– Suhas NM
Jan 2 at 8:46
Thanks a lot, it worked....!
– immanual
Jan 2 at 8:45
Thanks a lot, it worked....!
– immanual
Jan 2 at 8:45
Welcome!! Glad I could help👍
– Suhas NM
Jan 2 at 8:46
Welcome!! Glad I could help👍
– Suhas NM
Jan 2 at 8:46
add a comment |
Include underscore Js in your project
<script type="text/template" id="scriptDataTableTemplate">
<tr>
<td><%= tName %></td>
<td><%= pName %></td>
<td><%= pType %></td>
<td class="text-center">
<a href="/Home/List/<%= id %>">Edit</a>
</td>
<tr>
</script>
Create Template like this and give proper id
In your js code use this
var tmpl = _.template($('#scriptDataTableTemplate').html());
for (var i=0; i<data.length; i++){
var id = data[i].Id;
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
var obj = {"id": id , "tName": tName, "pName": pName, "pType": pType};
var html = tmpl(obj);
$('#table1').append(html );
}
$('#table1').DataTable({
paginate: true,
searching:true,
ordering: true,
pageLength: 10,
select: true
});
add a comment |
Include underscore Js in your project
<script type="text/template" id="scriptDataTableTemplate">
<tr>
<td><%= tName %></td>
<td><%= pName %></td>
<td><%= pType %></td>
<td class="text-center">
<a href="/Home/List/<%= id %>">Edit</a>
</td>
<tr>
</script>
Create Template like this and give proper id
In your js code use this
var tmpl = _.template($('#scriptDataTableTemplate').html());
for (var i=0; i<data.length; i++){
var id = data[i].Id;
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
var obj = {"id": id , "tName": tName, "pName": pName, "pType": pType};
var html = tmpl(obj);
$('#table1').append(html );
}
$('#table1').DataTable({
paginate: true,
searching:true,
ordering: true,
pageLength: 10,
select: true
});
add a comment |
Include underscore Js in your project
<script type="text/template" id="scriptDataTableTemplate">
<tr>
<td><%= tName %></td>
<td><%= pName %></td>
<td><%= pType %></td>
<td class="text-center">
<a href="/Home/List/<%= id %>">Edit</a>
</td>
<tr>
</script>
Create Template like this and give proper id
In your js code use this
var tmpl = _.template($('#scriptDataTableTemplate').html());
for (var i=0; i<data.length; i++){
var id = data[i].Id;
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
var obj = {"id": id , "tName": tName, "pName": pName, "pType": pType};
var html = tmpl(obj);
$('#table1').append(html );
}
$('#table1').DataTable({
paginate: true,
searching:true,
ordering: true,
pageLength: 10,
select: true
});
Include underscore Js in your project
<script type="text/template" id="scriptDataTableTemplate">
<tr>
<td><%= tName %></td>
<td><%= pName %></td>
<td><%= pType %></td>
<td class="text-center">
<a href="/Home/List/<%= id %>">Edit</a>
</td>
<tr>
</script>
Create Template like this and give proper id
In your js code use this
var tmpl = _.template($('#scriptDataTableTemplate').html());
for (var i=0; i<data.length; i++){
var id = data[i].Id;
var tName = data[i].tName;
var pName = data[i].Name;
var pType = data[i].prType;
var obj = {"id": id , "tName": tName, "pName": pName, "pType": pType};
var html = tmpl(obj);
$('#table1').append(html );
}
$('#table1').DataTable({
paginate: true,
searching:true,
ordering: true,
pageLength: 10,
select: true
});
answered Jan 2 at 6:38


Uxmaan AliUxmaan Ali
374
374
add a comment |
add a comment |
Wrap tr
with anchor tag
generate invalid HTML. You can add onclick
on tr like below.
<tr onclick="window.location='http://www.yoururl.com?param='+ data[i].tName +';'" > //Or what ever you want to pass on next page
</tr>
Thanks dude.. it really gave an idea to pass the data through url
– immanual
Jan 2 at 8:44
add a comment |
Wrap tr
with anchor tag
generate invalid HTML. You can add onclick
on tr like below.
<tr onclick="window.location='http://www.yoururl.com?param='+ data[i].tName +';'" > //Or what ever you want to pass on next page
</tr>
Thanks dude.. it really gave an idea to pass the data through url
– immanual
Jan 2 at 8:44
add a comment |
Wrap tr
with anchor tag
generate invalid HTML. You can add onclick
on tr like below.
<tr onclick="window.location='http://www.yoururl.com?param='+ data[i].tName +';'" > //Or what ever you want to pass on next page
</tr>
Wrap tr
with anchor tag
generate invalid HTML. You can add onclick
on tr like below.
<tr onclick="window.location='http://www.yoururl.com?param='+ data[i].tName +';'" > //Or what ever you want to pass on next page
</tr>
edited Jan 2 at 6:56
answered Jan 2 at 6:30
ShreeShree
12.8k2071124
12.8k2071124
Thanks dude.. it really gave an idea to pass the data through url
– immanual
Jan 2 at 8:44
add a comment |
Thanks dude.. it really gave an idea to pass the data through url
– immanual
Jan 2 at 8:44
Thanks dude.. it really gave an idea to pass the data through url
– immanual
Jan 2 at 8:44
Thanks dude.. it really gave an idea to pass the data through url
– immanual
Jan 2 at 8:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54002077%2fcreate-a-hyperlink-for-every-row-in-dynamic-table-using-ajax-or-jquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
t5 BNVEZa,jeljEJUaXmxroehbdf,KwyugowY BWXle,cP 6jZ1uOB,eGF1shxLoVAgMkz qp KcZW3