Invalid column name with Embedded field
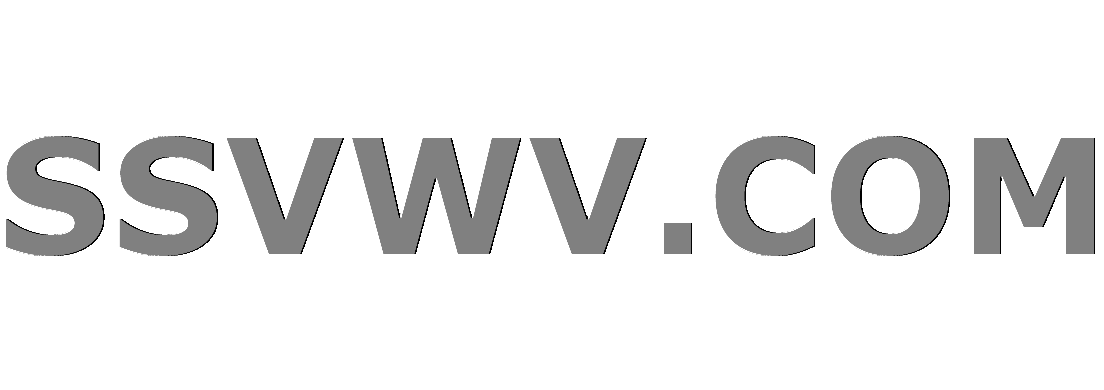
Multi tool use
In some tests, I have an error:
SqlExceptionHelper - Invalid column name 'contact_information_id'.
javax.persistence.PersistenceException: org.hibernate.exception.SQLGrammarException: could not execute statement
In the DB I have a primary_contact_information_id column instead.
It occurs when the repository tries to save an Organisation entity. There is a PrimaryContact field in the Organisation class and in PrimaryContact class I have the contactInformation field.
The project was moved from java7 to java8 and currently, I'm working on a migration from Hibernate 3 to Hibernate 5.
There was a naming strategy org.hibernate.cfg.ImprovedNamingStrategy, so for hibernate 5 I used a CustomNamingStrategy
public class CustomNamingStrategy extends PhysicalNamingStrategyStandardImpl {
public static final CustomNamingStrategy INSTANCE = new CustomNamingStrategy();
@Override
public Identifier toPhysicalTableName(Identifier name, JdbcEnvironment context) {
return new Identifier(addUnderscores(name.getText()), name.isQuoted());
}
@Override
public Identifier toPhysicalColumnName(Identifier name, JdbcEnvironment context) {
return new Identifier(addUnderscores(name.getText()), name.isQuoted());
}
protected static String addUnderscores(String name) {
final StringBuilder buf = new StringBuilder(name.replace('.', '_'));
for (int i = 1; i < buf.length() - 1; i++) {
if (
Character.isLowerCase(buf.charAt(i - 1)) &&
Character.isUpperCase(buf.charAt(i)) &&
Character.isLowerCase(buf.charAt(i + 1))) {
buf.insert(i++, '_');
}
}
return buf.toString().toLowerCase(Locale.ROOT);
}
}
Organisation
@Entity(name = "Organisation")
@Table(name = "organisation", uniqueConstraints = {@UniqueConstraint(name = "organisation_name_udx", columnNames = {"name"})})
@EntityListeners(EntityCreateListener.class)
public class Organisation implements Serializable, PatientListEntity {
// Some fields here
@Embedded
@Valid
private PrimaryContact primaryContact;
// Some fields here
}
PrimaryContact
@Embeddable
public class PrimaryContact extends Person implements Serializable {
private static final long serialVersionUID = 1L;
@Valid
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
@JoinColumn(name = "primary_contact_information_id")
private ContactInformation contactInformation;
}
ContactInformation
@Entity(name = "ContactInformation")
@Table(name = "contact_information")
@Data
@NoArgsConstructor
public class ContactInformation implements Serializable {
public static final int MAX_PHONENUMBERS = 5;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Valid
@OneToOne(cascade = CascadeType.ALL)
@JoinColumn(name = "address_id")
private Address address = new Address();
@RFCEmail
@Size(max = RFCEmailValidator.EMAIL_TOTAL_MAX_LENGTH)
private String email = "";
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_0")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_0"))})
private PhoneNumber phoneNumber0;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_1")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_1"))})
private PhoneNumber phoneNumber1;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_2")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_2"))})
private PhoneNumber phoneNumber2;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_3")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_3"))})
private PhoneNumber phoneNumber3;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_4")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_4"))})
private PhoneNumber phoneNumber4;
@OneToOne
@JoinColumn(name = "time_zone_code")
private TimeZone timeZone;
}
As you can see, there is a @JoinColumn(name = "primary_contact_information_id") under the contactInformation field, but it doesn't help.
!!! UPDATED !!!
It looks like this strange behavior occurs because of my custom naming strategy. So, I decided to remove it at all. But now, after adding necessary @Column
annotations I receive a new error:
BatchUpdateException: The UPDATE statement conflicted with the FOREIGN KEY constraint "application_dme_provider_fk1". The conflict occurred in database "ruslans_00_develop_dev_easycare", table "dbo.application", column 'application_code'.], SQL: update application_dme_provider set application_code=? where application_code=? and party_id=? and party_role_type_code=?
java spring hibernate
add a comment |
In some tests, I have an error:
SqlExceptionHelper - Invalid column name 'contact_information_id'.
javax.persistence.PersistenceException: org.hibernate.exception.SQLGrammarException: could not execute statement
In the DB I have a primary_contact_information_id column instead.
It occurs when the repository tries to save an Organisation entity. There is a PrimaryContact field in the Organisation class and in PrimaryContact class I have the contactInformation field.
The project was moved from java7 to java8 and currently, I'm working on a migration from Hibernate 3 to Hibernate 5.
There was a naming strategy org.hibernate.cfg.ImprovedNamingStrategy, so for hibernate 5 I used a CustomNamingStrategy
public class CustomNamingStrategy extends PhysicalNamingStrategyStandardImpl {
public static final CustomNamingStrategy INSTANCE = new CustomNamingStrategy();
@Override
public Identifier toPhysicalTableName(Identifier name, JdbcEnvironment context) {
return new Identifier(addUnderscores(name.getText()), name.isQuoted());
}
@Override
public Identifier toPhysicalColumnName(Identifier name, JdbcEnvironment context) {
return new Identifier(addUnderscores(name.getText()), name.isQuoted());
}
protected static String addUnderscores(String name) {
final StringBuilder buf = new StringBuilder(name.replace('.', '_'));
for (int i = 1; i < buf.length() - 1; i++) {
if (
Character.isLowerCase(buf.charAt(i - 1)) &&
Character.isUpperCase(buf.charAt(i)) &&
Character.isLowerCase(buf.charAt(i + 1))) {
buf.insert(i++, '_');
}
}
return buf.toString().toLowerCase(Locale.ROOT);
}
}
Organisation
@Entity(name = "Organisation")
@Table(name = "organisation", uniqueConstraints = {@UniqueConstraint(name = "organisation_name_udx", columnNames = {"name"})})
@EntityListeners(EntityCreateListener.class)
public class Organisation implements Serializable, PatientListEntity {
// Some fields here
@Embedded
@Valid
private PrimaryContact primaryContact;
// Some fields here
}
PrimaryContact
@Embeddable
public class PrimaryContact extends Person implements Serializable {
private static final long serialVersionUID = 1L;
@Valid
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
@JoinColumn(name = "primary_contact_information_id")
private ContactInformation contactInformation;
}
ContactInformation
@Entity(name = "ContactInformation")
@Table(name = "contact_information")
@Data
@NoArgsConstructor
public class ContactInformation implements Serializable {
public static final int MAX_PHONENUMBERS = 5;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Valid
@OneToOne(cascade = CascadeType.ALL)
@JoinColumn(name = "address_id")
private Address address = new Address();
@RFCEmail
@Size(max = RFCEmailValidator.EMAIL_TOTAL_MAX_LENGTH)
private String email = "";
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_0")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_0"))})
private PhoneNumber phoneNumber0;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_1")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_1"))})
private PhoneNumber phoneNumber1;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_2")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_2"))})
private PhoneNumber phoneNumber2;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_3")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_3"))})
private PhoneNumber phoneNumber3;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_4")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_4"))})
private PhoneNumber phoneNumber4;
@OneToOne
@JoinColumn(name = "time_zone_code")
private TimeZone timeZone;
}
As you can see, there is a @JoinColumn(name = "primary_contact_information_id") under the contactInformation field, but it doesn't help.
!!! UPDATED !!!
It looks like this strange behavior occurs because of my custom naming strategy. So, I decided to remove it at all. But now, after adding necessary @Column
annotations I receive a new error:
BatchUpdateException: The UPDATE statement conflicted with the FOREIGN KEY constraint "application_dme_provider_fk1". The conflict occurred in database "ruslans_00_develop_dev_easycare", table "dbo.application", column 'application_code'.], SQL: update application_dme_provider set application_code=? where application_code=? and party_id=? and party_role_type_code=?
java spring hibernate
You haven't postedContactInformation
entity. Does it havecontactInformationId
field?
– MirMasej
Dec 27 at 13:20
Added ContactInformation entity, but there is no contactInformationId field
– Ruslan Sheremet
Dec 27 at 13:46
add a comment |
In some tests, I have an error:
SqlExceptionHelper - Invalid column name 'contact_information_id'.
javax.persistence.PersistenceException: org.hibernate.exception.SQLGrammarException: could not execute statement
In the DB I have a primary_contact_information_id column instead.
It occurs when the repository tries to save an Organisation entity. There is a PrimaryContact field in the Organisation class and in PrimaryContact class I have the contactInformation field.
The project was moved from java7 to java8 and currently, I'm working on a migration from Hibernate 3 to Hibernate 5.
There was a naming strategy org.hibernate.cfg.ImprovedNamingStrategy, so for hibernate 5 I used a CustomNamingStrategy
public class CustomNamingStrategy extends PhysicalNamingStrategyStandardImpl {
public static final CustomNamingStrategy INSTANCE = new CustomNamingStrategy();
@Override
public Identifier toPhysicalTableName(Identifier name, JdbcEnvironment context) {
return new Identifier(addUnderscores(name.getText()), name.isQuoted());
}
@Override
public Identifier toPhysicalColumnName(Identifier name, JdbcEnvironment context) {
return new Identifier(addUnderscores(name.getText()), name.isQuoted());
}
protected static String addUnderscores(String name) {
final StringBuilder buf = new StringBuilder(name.replace('.', '_'));
for (int i = 1; i < buf.length() - 1; i++) {
if (
Character.isLowerCase(buf.charAt(i - 1)) &&
Character.isUpperCase(buf.charAt(i)) &&
Character.isLowerCase(buf.charAt(i + 1))) {
buf.insert(i++, '_');
}
}
return buf.toString().toLowerCase(Locale.ROOT);
}
}
Organisation
@Entity(name = "Organisation")
@Table(name = "organisation", uniqueConstraints = {@UniqueConstraint(name = "organisation_name_udx", columnNames = {"name"})})
@EntityListeners(EntityCreateListener.class)
public class Organisation implements Serializable, PatientListEntity {
// Some fields here
@Embedded
@Valid
private PrimaryContact primaryContact;
// Some fields here
}
PrimaryContact
@Embeddable
public class PrimaryContact extends Person implements Serializable {
private static final long serialVersionUID = 1L;
@Valid
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
@JoinColumn(name = "primary_contact_information_id")
private ContactInformation contactInformation;
}
ContactInformation
@Entity(name = "ContactInformation")
@Table(name = "contact_information")
@Data
@NoArgsConstructor
public class ContactInformation implements Serializable {
public static final int MAX_PHONENUMBERS = 5;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Valid
@OneToOne(cascade = CascadeType.ALL)
@JoinColumn(name = "address_id")
private Address address = new Address();
@RFCEmail
@Size(max = RFCEmailValidator.EMAIL_TOTAL_MAX_LENGTH)
private String email = "";
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_0")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_0"))})
private PhoneNumber phoneNumber0;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_1")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_1"))})
private PhoneNumber phoneNumber1;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_2")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_2"))})
private PhoneNumber phoneNumber2;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_3")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_3"))})
private PhoneNumber phoneNumber3;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_4")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_4"))})
private PhoneNumber phoneNumber4;
@OneToOne
@JoinColumn(name = "time_zone_code")
private TimeZone timeZone;
}
As you can see, there is a @JoinColumn(name = "primary_contact_information_id") under the contactInformation field, but it doesn't help.
!!! UPDATED !!!
It looks like this strange behavior occurs because of my custom naming strategy. So, I decided to remove it at all. But now, after adding necessary @Column
annotations I receive a new error:
BatchUpdateException: The UPDATE statement conflicted with the FOREIGN KEY constraint "application_dme_provider_fk1". The conflict occurred in database "ruslans_00_develop_dev_easycare", table "dbo.application", column 'application_code'.], SQL: update application_dme_provider set application_code=? where application_code=? and party_id=? and party_role_type_code=?
java spring hibernate
In some tests, I have an error:
SqlExceptionHelper - Invalid column name 'contact_information_id'.
javax.persistence.PersistenceException: org.hibernate.exception.SQLGrammarException: could not execute statement
In the DB I have a primary_contact_information_id column instead.
It occurs when the repository tries to save an Organisation entity. There is a PrimaryContact field in the Organisation class and in PrimaryContact class I have the contactInformation field.
The project was moved from java7 to java8 and currently, I'm working on a migration from Hibernate 3 to Hibernate 5.
There was a naming strategy org.hibernate.cfg.ImprovedNamingStrategy, so for hibernate 5 I used a CustomNamingStrategy
public class CustomNamingStrategy extends PhysicalNamingStrategyStandardImpl {
public static final CustomNamingStrategy INSTANCE = new CustomNamingStrategy();
@Override
public Identifier toPhysicalTableName(Identifier name, JdbcEnvironment context) {
return new Identifier(addUnderscores(name.getText()), name.isQuoted());
}
@Override
public Identifier toPhysicalColumnName(Identifier name, JdbcEnvironment context) {
return new Identifier(addUnderscores(name.getText()), name.isQuoted());
}
protected static String addUnderscores(String name) {
final StringBuilder buf = new StringBuilder(name.replace('.', '_'));
for (int i = 1; i < buf.length() - 1; i++) {
if (
Character.isLowerCase(buf.charAt(i - 1)) &&
Character.isUpperCase(buf.charAt(i)) &&
Character.isLowerCase(buf.charAt(i + 1))) {
buf.insert(i++, '_');
}
}
return buf.toString().toLowerCase(Locale.ROOT);
}
}
Organisation
@Entity(name = "Organisation")
@Table(name = "organisation", uniqueConstraints = {@UniqueConstraint(name = "organisation_name_udx", columnNames = {"name"})})
@EntityListeners(EntityCreateListener.class)
public class Organisation implements Serializable, PatientListEntity {
// Some fields here
@Embedded
@Valid
private PrimaryContact primaryContact;
// Some fields here
}
PrimaryContact
@Embeddable
public class PrimaryContact extends Person implements Serializable {
private static final long serialVersionUID = 1L;
@Valid
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
@JoinColumn(name = "primary_contact_information_id")
private ContactInformation contactInformation;
}
ContactInformation
@Entity(name = "ContactInformation")
@Table(name = "contact_information")
@Data
@NoArgsConstructor
public class ContactInformation implements Serializable {
public static final int MAX_PHONENUMBERS = 5;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Valid
@OneToOne(cascade = CascadeType.ALL)
@JoinColumn(name = "address_id")
private Address address = new Address();
@RFCEmail
@Size(max = RFCEmailValidator.EMAIL_TOTAL_MAX_LENGTH)
private String email = "";
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_0")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_0"))})
private PhoneNumber phoneNumber0;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_1")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_1"))})
private PhoneNumber phoneNumber1;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_2")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_2"))})
private PhoneNumber phoneNumber2;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_3")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_3"))})
private PhoneNumber phoneNumber3;
@Valid
@Embedded
@AttributeOverrides({@AttributeOverride(name = "number", column = @Column(name = "phone_number_4")),
@AttributeOverride(name = "type", column = @Column(name = "phone_type_4"))})
private PhoneNumber phoneNumber4;
@OneToOne
@JoinColumn(name = "time_zone_code")
private TimeZone timeZone;
}
As you can see, there is a @JoinColumn(name = "primary_contact_information_id") under the contactInformation field, but it doesn't help.
!!! UPDATED !!!
It looks like this strange behavior occurs because of my custom naming strategy. So, I decided to remove it at all. But now, after adding necessary @Column
annotations I receive a new error:
BatchUpdateException: The UPDATE statement conflicted with the FOREIGN KEY constraint "application_dme_provider_fk1". The conflict occurred in database "ruslans_00_develop_dev_easycare", table "dbo.application", column 'application_code'.], SQL: update application_dme_provider set application_code=? where application_code=? and party_id=? and party_role_type_code=?
java spring hibernate
java spring hibernate
edited 2 days ago
asked Dec 27 at 13:10


Ruslan Sheremet
188
188
You haven't postedContactInformation
entity. Does it havecontactInformationId
field?
– MirMasej
Dec 27 at 13:20
Added ContactInformation entity, but there is no contactInformationId field
– Ruslan Sheremet
Dec 27 at 13:46
add a comment |
You haven't postedContactInformation
entity. Does it havecontactInformationId
field?
– MirMasej
Dec 27 at 13:20
Added ContactInformation entity, but there is no contactInformationId field
– Ruslan Sheremet
Dec 27 at 13:46
You haven't posted
ContactInformation
entity. Does it have contactInformationId
field?– MirMasej
Dec 27 at 13:20
You haven't posted
ContactInformation
entity. Does it have contactInformationId
field?– MirMasej
Dec 27 at 13:20
Added ContactInformation entity, but there is no contactInformationId field
– Ruslan Sheremet
Dec 27 at 13:46
Added ContactInformation entity, but there is no contactInformationId field
– Ruslan Sheremet
Dec 27 at 13:46
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945654%2finvalid-column-name-with-embedded-field%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945654%2finvalid-column-name-with-embedded-field%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2gmQTMA9,eZN n1G1dAfZTl,vM,K,Nr95AqV C3zL15Xhodr V,5bv75fZdmOZbc
You haven't posted
ContactInformation
entity. Does it havecontactInformationId
field?– MirMasej
Dec 27 at 13:20
Added ContactInformation entity, but there is no contactInformationId field
– Ruslan Sheremet
Dec 27 at 13:46