How do I pass vertex color through the shader pipeline?
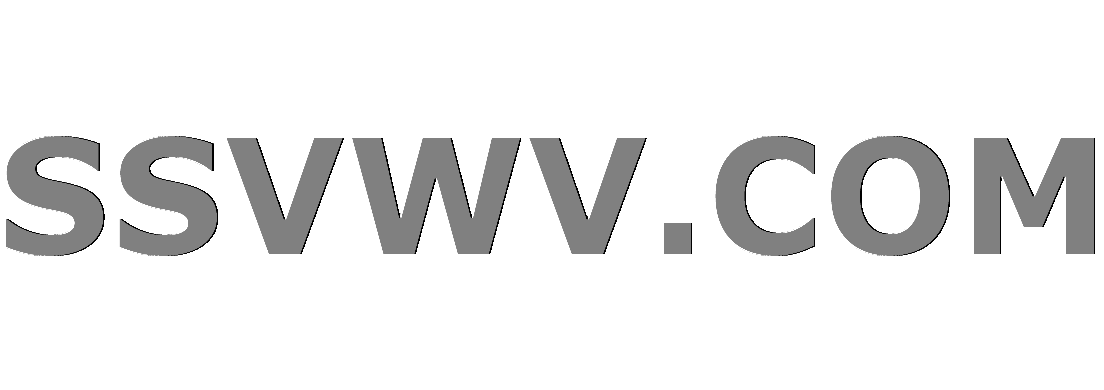
Multi tool use
I am trying to pass vertex color through the vertex, geometry and fragment shader:
glBegin(GL_POINTS);
glVertex3f(-2.0f, 0.0f, 0.0); glColor3f(0.0,1.0,0.0);
glVertex3f(+2.0f, 0.0f, 0.0); glColor3f(0.0,0.0,1.0);
glEnd();
vertex shader:
# version 130
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
geometry shader:
#version 150
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
varying vec4 colorv;
varying vec4 color;
void main (void)
{
for(int i = 0; i < gl_in.length(); ++i)
{
color = colorv;
gl_Position = gl_in[i].gl_Position;
EmitVertex();
EndPrimitive();
}
}
fragment shader:
# version 130
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
However it is not working :-(. Both points get a grey color.
How do I do this?
opengl glsl geometry-shader
add a comment |
I am trying to pass vertex color through the vertex, geometry and fragment shader:
glBegin(GL_POINTS);
glVertex3f(-2.0f, 0.0f, 0.0); glColor3f(0.0,1.0,0.0);
glVertex3f(+2.0f, 0.0f, 0.0); glColor3f(0.0,0.0,1.0);
glEnd();
vertex shader:
# version 130
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
geometry shader:
#version 150
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
varying vec4 colorv;
varying vec4 color;
void main (void)
{
for(int i = 0; i < gl_in.length(); ++i)
{
color = colorv;
gl_Position = gl_in[i].gl_Position;
EmitVertex();
EndPrimitive();
}
}
fragment shader:
# version 130
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
However it is not working :-(. Both points get a grey color.
How do I do this?
opengl glsl geometry-shader
2
CallglColor()
before theglVertex()
that you want it applied to.
– genpfault
Jan 22 '13 at 22:09
2
Why aren't you using using#version 150
in all your shaders?
– genpfault
Jan 22 '13 at 22:12
1
@genpfault: 1. colorv is written to by the vertex shader 2. thanks! However the problem persist. 3. When it comes to the fragment shader I found it much easier to use gl_FragColor (have not understood how to do this in version 150 yet).
– Andy
Jan 22 '13 at 22:14
Yep, misunderstanding on my part, disregard. Somehow I thought it was geom->vert->frag, not vert->geom->frag.
– genpfault
Jan 22 '13 at 22:15
1
@genpfault: I also thought so first :-). That would be more intuitive I think.
– Andy
Jan 22 '13 at 22:41
add a comment |
I am trying to pass vertex color through the vertex, geometry and fragment shader:
glBegin(GL_POINTS);
glVertex3f(-2.0f, 0.0f, 0.0); glColor3f(0.0,1.0,0.0);
glVertex3f(+2.0f, 0.0f, 0.0); glColor3f(0.0,0.0,1.0);
glEnd();
vertex shader:
# version 130
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
geometry shader:
#version 150
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
varying vec4 colorv;
varying vec4 color;
void main (void)
{
for(int i = 0; i < gl_in.length(); ++i)
{
color = colorv;
gl_Position = gl_in[i].gl_Position;
EmitVertex();
EndPrimitive();
}
}
fragment shader:
# version 130
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
However it is not working :-(. Both points get a grey color.
How do I do this?
opengl glsl geometry-shader
I am trying to pass vertex color through the vertex, geometry and fragment shader:
glBegin(GL_POINTS);
glVertex3f(-2.0f, 0.0f, 0.0); glColor3f(0.0,1.0,0.0);
glVertex3f(+2.0f, 0.0f, 0.0); glColor3f(0.0,0.0,1.0);
glEnd();
vertex shader:
# version 130
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
geometry shader:
#version 150
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
varying vec4 colorv;
varying vec4 color;
void main (void)
{
for(int i = 0; i < gl_in.length(); ++i)
{
color = colorv;
gl_Position = gl_in[i].gl_Position;
EmitVertex();
EndPrimitive();
}
}
fragment shader:
# version 130
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
However it is not working :-(. Both points get a grey color.
How do I do this?
opengl glsl geometry-shader
opengl glsl geometry-shader
edited Dec 30 '18 at 12:10


jpw
40.5k64463
40.5k64463
asked Jan 22 '13 at 21:54
AndyAndy
69711031
69711031
2
CallglColor()
before theglVertex()
that you want it applied to.
– genpfault
Jan 22 '13 at 22:09
2
Why aren't you using using#version 150
in all your shaders?
– genpfault
Jan 22 '13 at 22:12
1
@genpfault: 1. colorv is written to by the vertex shader 2. thanks! However the problem persist. 3. When it comes to the fragment shader I found it much easier to use gl_FragColor (have not understood how to do this in version 150 yet).
– Andy
Jan 22 '13 at 22:14
Yep, misunderstanding on my part, disregard. Somehow I thought it was geom->vert->frag, not vert->geom->frag.
– genpfault
Jan 22 '13 at 22:15
1
@genpfault: I also thought so first :-). That would be more intuitive I think.
– Andy
Jan 22 '13 at 22:41
add a comment |
2
CallglColor()
before theglVertex()
that you want it applied to.
– genpfault
Jan 22 '13 at 22:09
2
Why aren't you using using#version 150
in all your shaders?
– genpfault
Jan 22 '13 at 22:12
1
@genpfault: 1. colorv is written to by the vertex shader 2. thanks! However the problem persist. 3. When it comes to the fragment shader I found it much easier to use gl_FragColor (have not understood how to do this in version 150 yet).
– Andy
Jan 22 '13 at 22:14
Yep, misunderstanding on my part, disregard. Somehow I thought it was geom->vert->frag, not vert->geom->frag.
– genpfault
Jan 22 '13 at 22:15
1
@genpfault: I also thought so first :-). That would be more intuitive I think.
– Andy
Jan 22 '13 at 22:41
2
2
Call
glColor()
before the glVertex()
that you want it applied to.– genpfault
Jan 22 '13 at 22:09
Call
glColor()
before the glVertex()
that you want it applied to.– genpfault
Jan 22 '13 at 22:09
2
2
Why aren't you using using
#version 150
in all your shaders?– genpfault
Jan 22 '13 at 22:12
Why aren't you using using
#version 150
in all your shaders?– genpfault
Jan 22 '13 at 22:12
1
1
@genpfault: 1. colorv is written to by the vertex shader 2. thanks! However the problem persist. 3. When it comes to the fragment shader I found it much easier to use gl_FragColor (have not understood how to do this in version 150 yet).
– Andy
Jan 22 '13 at 22:14
@genpfault: 1. colorv is written to by the vertex shader 2. thanks! However the problem persist. 3. When it comes to the fragment shader I found it much easier to use gl_FragColor (have not understood how to do this in version 150 yet).
– Andy
Jan 22 '13 at 22:14
Yep, misunderstanding on my part, disregard. Somehow I thought it was geom->vert->frag, not vert->geom->frag.
– genpfault
Jan 22 '13 at 22:15
Yep, misunderstanding on my part, disregard. Somehow I thought it was geom->vert->frag, not vert->geom->frag.
– genpfault
Jan 22 '13 at 22:15
1
1
@genpfault: I also thought so first :-). That would be more intuitive I think.
– Andy
Jan 22 '13 at 22:41
@genpfault: I also thought so first :-). That would be more intuitive I think.
– Andy
Jan 22 '13 at 22:41
add a comment |
1 Answer
1
active
oldest
votes
Try changing the input/ouput declaration in your geometry shader from this:
varying vec4 colorv;
varying vec4 color;
to this:
in vec4 colorv;
out vec4 color;
Like so:
#include <GL/glew.h>
#include <GL/glut.h>
#include <iostream>
#include <vector>
using namespace std;
#define GLSL( version, shader ) "#version " #version "n" #shader
const GLchar* vert = GLSL
(
130,
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
);
const GLchar* geom = GLSL
(
150,
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
in vec4 colorv;
out vec4 color;
void main (void)
{
for( int i = 0; i < gl_in.length(); ++i )
{
color = colorv[i];
gl_Position = gl_in[i].gl_Position;
EmitVertex();
}
EndPrimitive();
}
);
const GLchar* frag = GLSL
(
130,
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
);
GLuint LoadShader( GLenum type, const string& aShaderSrc )
{
GLuint shader = glCreateShader( type );
const char* srcPtr = aShaderSrc.c_str();
glShaderSource( shader, 1, &srcPtr, NULL );
glCompileShader( shader );
return shader;
}
GLuint LoadProgram( const string& aVertSrc, const string& aGeomSrc, const string& aFragSrc )
{
GLuint vert = LoadShader( GL_VERTEX_SHADER, aVertSrc );
GLuint geom = LoadShader( GL_GEOMETRY_SHADER, aGeomSrc );
GLuint frag = LoadShader( GL_FRAGMENT_SHADER, aFragSrc );
GLuint program = glCreateProgram();
glAttachShader( program, vert );
glAttachShader( program, geom );
glAttachShader( program, frag );
glLinkProgram( program );
return program;
}
GLuint prog = 0;
void display()
{
glClear( GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT );
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
double w = glutGet( GLUT_WINDOW_WIDTH );
double h = glutGet( GLUT_WINDOW_HEIGHT );
double ar = w / h;
glOrtho( -2 * ar, 2 * ar, -2, 2, -1, 1);
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
glUseProgram( prog );
glBegin(GL_POINTS);
glColor3ub( 0, 255, 0 );
glVertex3f(-1.0f, 0.0f, 0.0);
glColor3ub( 0, 0, 255 );
glVertex3f(+1.0f, 0.0f, 0.0);
glEnd();
glutSwapBuffers();
}
int main( int argc, char **argv )
{
glutInit( &argc, argv );
glutInitDisplayMode( GLUT_RGBA | GLUT_DEPTH | GLUT_DOUBLE );
glutInitWindowSize( 640, 480 );
glutCreateWindow( "Geometry Shaders" );
glewInit();
prog = LoadProgram( vert, geom, frag );
glutDisplayFunc( display );
glutMainLoop();
return 0;
}
1
That worked! Thanks genpfault! Such a small change but with a big effect :-).
– Andy
Jan 23 '13 at 7:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f14468724%2fhow-do-i-pass-vertex-color-through-the-shader-pipeline%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try changing the input/ouput declaration in your geometry shader from this:
varying vec4 colorv;
varying vec4 color;
to this:
in vec4 colorv;
out vec4 color;
Like so:
#include <GL/glew.h>
#include <GL/glut.h>
#include <iostream>
#include <vector>
using namespace std;
#define GLSL( version, shader ) "#version " #version "n" #shader
const GLchar* vert = GLSL
(
130,
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
);
const GLchar* geom = GLSL
(
150,
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
in vec4 colorv;
out vec4 color;
void main (void)
{
for( int i = 0; i < gl_in.length(); ++i )
{
color = colorv[i];
gl_Position = gl_in[i].gl_Position;
EmitVertex();
}
EndPrimitive();
}
);
const GLchar* frag = GLSL
(
130,
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
);
GLuint LoadShader( GLenum type, const string& aShaderSrc )
{
GLuint shader = glCreateShader( type );
const char* srcPtr = aShaderSrc.c_str();
glShaderSource( shader, 1, &srcPtr, NULL );
glCompileShader( shader );
return shader;
}
GLuint LoadProgram( const string& aVertSrc, const string& aGeomSrc, const string& aFragSrc )
{
GLuint vert = LoadShader( GL_VERTEX_SHADER, aVertSrc );
GLuint geom = LoadShader( GL_GEOMETRY_SHADER, aGeomSrc );
GLuint frag = LoadShader( GL_FRAGMENT_SHADER, aFragSrc );
GLuint program = glCreateProgram();
glAttachShader( program, vert );
glAttachShader( program, geom );
glAttachShader( program, frag );
glLinkProgram( program );
return program;
}
GLuint prog = 0;
void display()
{
glClear( GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT );
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
double w = glutGet( GLUT_WINDOW_WIDTH );
double h = glutGet( GLUT_WINDOW_HEIGHT );
double ar = w / h;
glOrtho( -2 * ar, 2 * ar, -2, 2, -1, 1);
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
glUseProgram( prog );
glBegin(GL_POINTS);
glColor3ub( 0, 255, 0 );
glVertex3f(-1.0f, 0.0f, 0.0);
glColor3ub( 0, 0, 255 );
glVertex3f(+1.0f, 0.0f, 0.0);
glEnd();
glutSwapBuffers();
}
int main( int argc, char **argv )
{
glutInit( &argc, argv );
glutInitDisplayMode( GLUT_RGBA | GLUT_DEPTH | GLUT_DOUBLE );
glutInitWindowSize( 640, 480 );
glutCreateWindow( "Geometry Shaders" );
glewInit();
prog = LoadProgram( vert, geom, frag );
glutDisplayFunc( display );
glutMainLoop();
return 0;
}
1
That worked! Thanks genpfault! Such a small change but with a big effect :-).
– Andy
Jan 23 '13 at 7:16
add a comment |
Try changing the input/ouput declaration in your geometry shader from this:
varying vec4 colorv;
varying vec4 color;
to this:
in vec4 colorv;
out vec4 color;
Like so:
#include <GL/glew.h>
#include <GL/glut.h>
#include <iostream>
#include <vector>
using namespace std;
#define GLSL( version, shader ) "#version " #version "n" #shader
const GLchar* vert = GLSL
(
130,
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
);
const GLchar* geom = GLSL
(
150,
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
in vec4 colorv;
out vec4 color;
void main (void)
{
for( int i = 0; i < gl_in.length(); ++i )
{
color = colorv[i];
gl_Position = gl_in[i].gl_Position;
EmitVertex();
}
EndPrimitive();
}
);
const GLchar* frag = GLSL
(
130,
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
);
GLuint LoadShader( GLenum type, const string& aShaderSrc )
{
GLuint shader = glCreateShader( type );
const char* srcPtr = aShaderSrc.c_str();
glShaderSource( shader, 1, &srcPtr, NULL );
glCompileShader( shader );
return shader;
}
GLuint LoadProgram( const string& aVertSrc, const string& aGeomSrc, const string& aFragSrc )
{
GLuint vert = LoadShader( GL_VERTEX_SHADER, aVertSrc );
GLuint geom = LoadShader( GL_GEOMETRY_SHADER, aGeomSrc );
GLuint frag = LoadShader( GL_FRAGMENT_SHADER, aFragSrc );
GLuint program = glCreateProgram();
glAttachShader( program, vert );
glAttachShader( program, geom );
glAttachShader( program, frag );
glLinkProgram( program );
return program;
}
GLuint prog = 0;
void display()
{
glClear( GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT );
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
double w = glutGet( GLUT_WINDOW_WIDTH );
double h = glutGet( GLUT_WINDOW_HEIGHT );
double ar = w / h;
glOrtho( -2 * ar, 2 * ar, -2, 2, -1, 1);
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
glUseProgram( prog );
glBegin(GL_POINTS);
glColor3ub( 0, 255, 0 );
glVertex3f(-1.0f, 0.0f, 0.0);
glColor3ub( 0, 0, 255 );
glVertex3f(+1.0f, 0.0f, 0.0);
glEnd();
glutSwapBuffers();
}
int main( int argc, char **argv )
{
glutInit( &argc, argv );
glutInitDisplayMode( GLUT_RGBA | GLUT_DEPTH | GLUT_DOUBLE );
glutInitWindowSize( 640, 480 );
glutCreateWindow( "Geometry Shaders" );
glewInit();
prog = LoadProgram( vert, geom, frag );
glutDisplayFunc( display );
glutMainLoop();
return 0;
}
1
That worked! Thanks genpfault! Such a small change but with a big effect :-).
– Andy
Jan 23 '13 at 7:16
add a comment |
Try changing the input/ouput declaration in your geometry shader from this:
varying vec4 colorv;
varying vec4 color;
to this:
in vec4 colorv;
out vec4 color;
Like so:
#include <GL/glew.h>
#include <GL/glut.h>
#include <iostream>
#include <vector>
using namespace std;
#define GLSL( version, shader ) "#version " #version "n" #shader
const GLchar* vert = GLSL
(
130,
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
);
const GLchar* geom = GLSL
(
150,
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
in vec4 colorv;
out vec4 color;
void main (void)
{
for( int i = 0; i < gl_in.length(); ++i )
{
color = colorv[i];
gl_Position = gl_in[i].gl_Position;
EmitVertex();
}
EndPrimitive();
}
);
const GLchar* frag = GLSL
(
130,
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
);
GLuint LoadShader( GLenum type, const string& aShaderSrc )
{
GLuint shader = glCreateShader( type );
const char* srcPtr = aShaderSrc.c_str();
glShaderSource( shader, 1, &srcPtr, NULL );
glCompileShader( shader );
return shader;
}
GLuint LoadProgram( const string& aVertSrc, const string& aGeomSrc, const string& aFragSrc )
{
GLuint vert = LoadShader( GL_VERTEX_SHADER, aVertSrc );
GLuint geom = LoadShader( GL_GEOMETRY_SHADER, aGeomSrc );
GLuint frag = LoadShader( GL_FRAGMENT_SHADER, aFragSrc );
GLuint program = glCreateProgram();
glAttachShader( program, vert );
glAttachShader( program, geom );
glAttachShader( program, frag );
glLinkProgram( program );
return program;
}
GLuint prog = 0;
void display()
{
glClear( GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT );
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
double w = glutGet( GLUT_WINDOW_WIDTH );
double h = glutGet( GLUT_WINDOW_HEIGHT );
double ar = w / h;
glOrtho( -2 * ar, 2 * ar, -2, 2, -1, 1);
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
glUseProgram( prog );
glBegin(GL_POINTS);
glColor3ub( 0, 255, 0 );
glVertex3f(-1.0f, 0.0f, 0.0);
glColor3ub( 0, 0, 255 );
glVertex3f(+1.0f, 0.0f, 0.0);
glEnd();
glutSwapBuffers();
}
int main( int argc, char **argv )
{
glutInit( &argc, argv );
glutInitDisplayMode( GLUT_RGBA | GLUT_DEPTH | GLUT_DOUBLE );
glutInitWindowSize( 640, 480 );
glutCreateWindow( "Geometry Shaders" );
glewInit();
prog = LoadProgram( vert, geom, frag );
glutDisplayFunc( display );
glutMainLoop();
return 0;
}
Try changing the input/ouput declaration in your geometry shader from this:
varying vec4 colorv;
varying vec4 color;
to this:
in vec4 colorv;
out vec4 color;
Like so:
#include <GL/glew.h>
#include <GL/glut.h>
#include <iostream>
#include <vector>
using namespace std;
#define GLSL( version, shader ) "#version " #version "n" #shader
const GLchar* vert = GLSL
(
130,
varying vec4 colorv;
void main() {
// pass trough:
gl_Position = gl_ProjectionMatrix * gl_ModelViewMatrix * gl_Vertex;
colorv = gl_Color;
}
);
const GLchar* geom = GLSL
(
150,
layout(points) in; // origo of cell
layout(points, max_vertices = 1) out;
in vec4 colorv;
out vec4 color;
void main (void)
{
for( int i = 0; i < gl_in.length(); ++i )
{
color = colorv[i];
gl_Position = gl_in[i].gl_Position;
EmitVertex();
}
EndPrimitive();
}
);
const GLchar* frag = GLSL
(
130,
varying vec4 color;
void main (void)
{
// pass-trough:
gl_FragColor = color;
}
);
GLuint LoadShader( GLenum type, const string& aShaderSrc )
{
GLuint shader = glCreateShader( type );
const char* srcPtr = aShaderSrc.c_str();
glShaderSource( shader, 1, &srcPtr, NULL );
glCompileShader( shader );
return shader;
}
GLuint LoadProgram( const string& aVertSrc, const string& aGeomSrc, const string& aFragSrc )
{
GLuint vert = LoadShader( GL_VERTEX_SHADER, aVertSrc );
GLuint geom = LoadShader( GL_GEOMETRY_SHADER, aGeomSrc );
GLuint frag = LoadShader( GL_FRAGMENT_SHADER, aFragSrc );
GLuint program = glCreateProgram();
glAttachShader( program, vert );
glAttachShader( program, geom );
glAttachShader( program, frag );
glLinkProgram( program );
return program;
}
GLuint prog = 0;
void display()
{
glClear( GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT );
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
double w = glutGet( GLUT_WINDOW_WIDTH );
double h = glutGet( GLUT_WINDOW_HEIGHT );
double ar = w / h;
glOrtho( -2 * ar, 2 * ar, -2, 2, -1, 1);
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
glUseProgram( prog );
glBegin(GL_POINTS);
glColor3ub( 0, 255, 0 );
glVertex3f(-1.0f, 0.0f, 0.0);
glColor3ub( 0, 0, 255 );
glVertex3f(+1.0f, 0.0f, 0.0);
glEnd();
glutSwapBuffers();
}
int main( int argc, char **argv )
{
glutInit( &argc, argv );
glutInitDisplayMode( GLUT_RGBA | GLUT_DEPTH | GLUT_DOUBLE );
glutInitWindowSize( 640, 480 );
glutCreateWindow( "Geometry Shaders" );
glewInit();
prog = LoadProgram( vert, geom, frag );
glutDisplayFunc( display );
glutMainLoop();
return 0;
}
edited Jan 22 '13 at 23:06
answered Jan 22 '13 at 22:46


genpfaultgenpfault
41.9k95398
41.9k95398
1
That worked! Thanks genpfault! Such a small change but with a big effect :-).
– Andy
Jan 23 '13 at 7:16
add a comment |
1
That worked! Thanks genpfault! Such a small change but with a big effect :-).
– Andy
Jan 23 '13 at 7:16
1
1
That worked! Thanks genpfault! Such a small change but with a big effect :-).
– Andy
Jan 23 '13 at 7:16
That worked! Thanks genpfault! Such a small change but with a big effect :-).
– Andy
Jan 23 '13 at 7:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f14468724%2fhow-do-i-pass-vertex-color-through-the-shader-pipeline%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gE O Y pepq9,N qXapZnb8BWY 51,fmZq84uyxrzQ,yR5f53Sn,MbwZ0t F umdH xvicVuQ,16eo,fH7wOJiLYIB4wAz Qh E,TNNmlC3
2
Call
glColor()
before theglVertex()
that you want it applied to.– genpfault
Jan 22 '13 at 22:09
2
Why aren't you using using
#version 150
in all your shaders?– genpfault
Jan 22 '13 at 22:12
1
@genpfault: 1. colorv is written to by the vertex shader 2. thanks! However the problem persist. 3. When it comes to the fragment shader I found it much easier to use gl_FragColor (have not understood how to do this in version 150 yet).
– Andy
Jan 22 '13 at 22:14
Yep, misunderstanding on my part, disregard. Somehow I thought it was geom->vert->frag, not vert->geom->frag.
– genpfault
Jan 22 '13 at 22:15
1
@genpfault: I also thought so first :-). That would be more intuitive I think.
– Andy
Jan 22 '13 at 22:41