Why is cin.failbit always set even when input is valid?
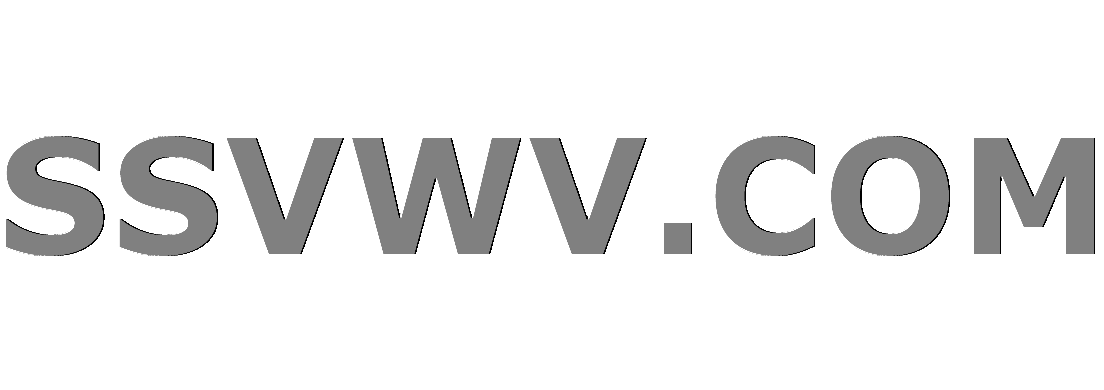
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I was trying to write a program that asks to input a char array using cin.getline()
and if given input is bigger than array length array gets extended.
I used cin.failbit
to see if user's input was too long. But nothing went right. So after debugging I figured out that the problem lies in failbit
.
So I wrote a simple program to see what was wrong about it and it turned out that somehow cin.failbit
always returns true when in if-statement even when input seems valid.
int main () {
char name[256];
std::cout << "Enter your name: ";
std::cin.getline (name,256, 'n');
std::cout << "characters read: " << std::cin.gcount() << std::endl;
if (std::cin.failbit){
std::cin.clear();
std::cout << "failedn";
}
}
For example, when input is "qwer" program outputs that 5 characters have been read (cin.gcount
), so it should be fine but it also outputs "fail" meaning that failbit flag is set. But I believe it shouldn't be in this case.
program output here
So can anyone explain why failbit
appears to be set permanently?
Any help will be highly appreciated
c++ arrays cin getline
|
show 3 more comments
I was trying to write a program that asks to input a char array using cin.getline()
and if given input is bigger than array length array gets extended.
I used cin.failbit
to see if user's input was too long. But nothing went right. So after debugging I figured out that the problem lies in failbit
.
So I wrote a simple program to see what was wrong about it and it turned out that somehow cin.failbit
always returns true when in if-statement even when input seems valid.
int main () {
char name[256];
std::cout << "Enter your name: ";
std::cin.getline (name,256, 'n');
std::cout << "characters read: " << std::cin.gcount() << std::endl;
if (std::cin.failbit){
std::cin.clear();
std::cout << "failedn";
}
}
For example, when input is "qwer" program outputs that 5 characters have been read (cin.gcount
), so it should be fine but it also outputs "fail" meaning that failbit flag is set. But I believe it shouldn't be in this case.
program output here
So can anyone explain why failbit
appears to be set permanently?
Any help will be highly appreciated
c++ arrays cin getline
@fran please answer in answer not comments.
– Yakk - Adam Nevraumont
Jan 3 at 20:33
FWIW you can useif (!std::cin)
instead to check if the stream is in a failure state.
– NathanOliver
Jan 3 at 20:33
3
I was mistaken, there is nofailbit
member function. It's checking that the value of the failbit bit mask isn't zero. I was thinking of thefail()
member function.std::cin.failbit
is a constant that indicates which bit represents a failed state, it's not checking the state of the stream.
– François Andrieux
Jan 3 at 20:33
thanks for replies. I considered using cin.fail() or !cin but they are not invoked when user's input is longer than the len of the array. User's input just gets cut and all characters past the size of array remain in the buffer (at least that is what I observed)
– keptt
Jan 3 at 20:42
1
@keptt No,failbit
is not a flag in the stateful sense. It indicates which bit is associated with that flag. It cannot be set or cleared, it's a constant. The phrase "thefailbit
flag" means "the bit indicated by the bitmaskfailbit
".
– François Andrieux
Jan 3 at 21:04
|
show 3 more comments
I was trying to write a program that asks to input a char array using cin.getline()
and if given input is bigger than array length array gets extended.
I used cin.failbit
to see if user's input was too long. But nothing went right. So after debugging I figured out that the problem lies in failbit
.
So I wrote a simple program to see what was wrong about it and it turned out that somehow cin.failbit
always returns true when in if-statement even when input seems valid.
int main () {
char name[256];
std::cout << "Enter your name: ";
std::cin.getline (name,256, 'n');
std::cout << "characters read: " << std::cin.gcount() << std::endl;
if (std::cin.failbit){
std::cin.clear();
std::cout << "failedn";
}
}
For example, when input is "qwer" program outputs that 5 characters have been read (cin.gcount
), so it should be fine but it also outputs "fail" meaning that failbit flag is set. But I believe it shouldn't be in this case.
program output here
So can anyone explain why failbit
appears to be set permanently?
Any help will be highly appreciated
c++ arrays cin getline
I was trying to write a program that asks to input a char array using cin.getline()
and if given input is bigger than array length array gets extended.
I used cin.failbit
to see if user's input was too long. But nothing went right. So after debugging I figured out that the problem lies in failbit
.
So I wrote a simple program to see what was wrong about it and it turned out that somehow cin.failbit
always returns true when in if-statement even when input seems valid.
int main () {
char name[256];
std::cout << "Enter your name: ";
std::cin.getline (name,256, 'n');
std::cout << "characters read: " << std::cin.gcount() << std::endl;
if (std::cin.failbit){
std::cin.clear();
std::cout << "failedn";
}
}
For example, when input is "qwer" program outputs that 5 characters have been read (cin.gcount
), so it should be fine but it also outputs "fail" meaning that failbit flag is set. But I believe it shouldn't be in this case.
program output here
So can anyone explain why failbit
appears to be set permanently?
Any help will be highly appreciated
c++ arrays cin getline
c++ arrays cin getline
edited Jan 3 at 20:33
scohe001
8,19212442
8,19212442
asked Jan 3 at 20:28


kepttkeptt
91
91
@fran please answer in answer not comments.
– Yakk - Adam Nevraumont
Jan 3 at 20:33
FWIW you can useif (!std::cin)
instead to check if the stream is in a failure state.
– NathanOliver
Jan 3 at 20:33
3
I was mistaken, there is nofailbit
member function. It's checking that the value of the failbit bit mask isn't zero. I was thinking of thefail()
member function.std::cin.failbit
is a constant that indicates which bit represents a failed state, it's not checking the state of the stream.
– François Andrieux
Jan 3 at 20:33
thanks for replies. I considered using cin.fail() or !cin but they are not invoked when user's input is longer than the len of the array. User's input just gets cut and all characters past the size of array remain in the buffer (at least that is what I observed)
– keptt
Jan 3 at 20:42
1
@keptt No,failbit
is not a flag in the stateful sense. It indicates which bit is associated with that flag. It cannot be set or cleared, it's a constant. The phrase "thefailbit
flag" means "the bit indicated by the bitmaskfailbit
".
– François Andrieux
Jan 3 at 21:04
|
show 3 more comments
@fran please answer in answer not comments.
– Yakk - Adam Nevraumont
Jan 3 at 20:33
FWIW you can useif (!std::cin)
instead to check if the stream is in a failure state.
– NathanOliver
Jan 3 at 20:33
3
I was mistaken, there is nofailbit
member function. It's checking that the value of the failbit bit mask isn't zero. I was thinking of thefail()
member function.std::cin.failbit
is a constant that indicates which bit represents a failed state, it's not checking the state of the stream.
– François Andrieux
Jan 3 at 20:33
thanks for replies. I considered using cin.fail() or !cin but they are not invoked when user's input is longer than the len of the array. User's input just gets cut and all characters past the size of array remain in the buffer (at least that is what I observed)
– keptt
Jan 3 at 20:42
1
@keptt No,failbit
is not a flag in the stateful sense. It indicates which bit is associated with that flag. It cannot be set or cleared, it's a constant. The phrase "thefailbit
flag" means "the bit indicated by the bitmaskfailbit
".
– François Andrieux
Jan 3 at 21:04
@fran please answer in answer not comments.
– Yakk - Adam Nevraumont
Jan 3 at 20:33
@fran please answer in answer not comments.
– Yakk - Adam Nevraumont
Jan 3 at 20:33
FWIW you can use
if (!std::cin)
instead to check if the stream is in a failure state.– NathanOliver
Jan 3 at 20:33
FWIW you can use
if (!std::cin)
instead to check if the stream is in a failure state.– NathanOliver
Jan 3 at 20:33
3
3
I was mistaken, there is no
failbit
member function. It's checking that the value of the failbit bit mask isn't zero. I was thinking of the fail()
member function. std::cin.failbit
is a constant that indicates which bit represents a failed state, it's not checking the state of the stream.– François Andrieux
Jan 3 at 20:33
I was mistaken, there is no
failbit
member function. It's checking that the value of the failbit bit mask isn't zero. I was thinking of the fail()
member function. std::cin.failbit
is a constant that indicates which bit represents a failed state, it's not checking the state of the stream.– François Andrieux
Jan 3 at 20:33
thanks for replies. I considered using cin.fail() or !cin but they are not invoked when user's input is longer than the len of the array. User's input just gets cut and all characters past the size of array remain in the buffer (at least that is what I observed)
– keptt
Jan 3 at 20:42
thanks for replies. I considered using cin.fail() or !cin but they are not invoked when user's input is longer than the len of the array. User's input just gets cut and all characters past the size of array remain in the buffer (at least that is what I observed)
– keptt
Jan 3 at 20:42
1
1
@keptt No,
failbit
is not a flag in the stateful sense. It indicates which bit is associated with that flag. It cannot be set or cleared, it's a constant. The phrase "the failbit
flag" means "the bit indicated by the bitmask failbit
".– François Andrieux
Jan 3 at 21:04
@keptt No,
failbit
is not a flag in the stateful sense. It indicates which bit is associated with that flag. It cannot be set or cleared, it's a constant. The phrase "the failbit
flag" means "the bit indicated by the bitmask failbit
".– François Andrieux
Jan 3 at 21:04
|
show 3 more comments
1 Answer
1
active
oldest
votes
std::cin.failbit
is a constant that indicates which of the error bits represents stream failure. It is not an indication of the stream's current state. To check if that bit is set, use the member function std::cin.fail()
instead.
However, if the stream failed to read due to reaching the end of the stream fail()
will return false
leading you to believe that it succeeded. Prefer std::cin.good()
to check if the last operation succeeded.
Documentation link
– user4581301
Jan 3 at 20:41
I appreciate the answer but for me the part about failbit just interferes with getline description link 4th block here states: "The failbit flag is set if the function extracts no characters, or if the delimiting character is not found once (n-1) characters have already been written to s". So for me it's confusing
– keptt
Jan 3 at 20:57
@keptt The error state is stored in a bitset.failbit
is a constant that indicates which of the bits represents failure. For example, it's value might be the constant8
indicating that the 4th bit indicates failure. In that instance, yourif
would be essentiallyif(8)
and doesn't account for the current state of your stream. An example of a use forfailbit
might bestd::cin.rdstate() & std::ios_base::failbit
to see if thefailbit
is1
in the bitset returned byrdstate()
.
– François Andrieux
Jan 3 at 20:59
thanks, I guess I get it now. I tried to act on your advise and used cin.good() - it worked just fine so far. Not to be too annoying but would you mind me asking about dealing with failbit? If it's a constant then it should have some kind of default value meaning if I type smth like - if(cin.failbit == default_value) it would mean that no errors that can trigger failbit were detected and everything is fine. Is it like that?
– keptt
Jan 3 at 21:17
@keptt No.failbit
has nothing to do withcin
's state. Imagine it's a global constant (because that's what it is). There is no useful information you can extract from it, it's a number and it's always that number for the lifetime of your program. Imagine everywhere you seefailbit
that it instead just says4
, or16
or whatever value it has. It cannot change during the lifetime of your program. It's only use is as a bitmask to help you interpret the value returned byrdstate()
. The fact thatstd::cin.failbit
compiles is misleading.failbit
is astatic constexpr
.
– François Andrieux
Jan 3 at 21:19
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54029350%2fwhy-is-cin-failbit-always-set-even-when-input-is-valid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
std::cin.failbit
is a constant that indicates which of the error bits represents stream failure. It is not an indication of the stream's current state. To check if that bit is set, use the member function std::cin.fail()
instead.
However, if the stream failed to read due to reaching the end of the stream fail()
will return false
leading you to believe that it succeeded. Prefer std::cin.good()
to check if the last operation succeeded.
Documentation link
– user4581301
Jan 3 at 20:41
I appreciate the answer but for me the part about failbit just interferes with getline description link 4th block here states: "The failbit flag is set if the function extracts no characters, or if the delimiting character is not found once (n-1) characters have already been written to s". So for me it's confusing
– keptt
Jan 3 at 20:57
@keptt The error state is stored in a bitset.failbit
is a constant that indicates which of the bits represents failure. For example, it's value might be the constant8
indicating that the 4th bit indicates failure. In that instance, yourif
would be essentiallyif(8)
and doesn't account for the current state of your stream. An example of a use forfailbit
might bestd::cin.rdstate() & std::ios_base::failbit
to see if thefailbit
is1
in the bitset returned byrdstate()
.
– François Andrieux
Jan 3 at 20:59
thanks, I guess I get it now. I tried to act on your advise and used cin.good() - it worked just fine so far. Not to be too annoying but would you mind me asking about dealing with failbit? If it's a constant then it should have some kind of default value meaning if I type smth like - if(cin.failbit == default_value) it would mean that no errors that can trigger failbit were detected and everything is fine. Is it like that?
– keptt
Jan 3 at 21:17
@keptt No.failbit
has nothing to do withcin
's state. Imagine it's a global constant (because that's what it is). There is no useful information you can extract from it, it's a number and it's always that number for the lifetime of your program. Imagine everywhere you seefailbit
that it instead just says4
, or16
or whatever value it has. It cannot change during the lifetime of your program. It's only use is as a bitmask to help you interpret the value returned byrdstate()
. The fact thatstd::cin.failbit
compiles is misleading.failbit
is astatic constexpr
.
– François Andrieux
Jan 3 at 21:19
|
show 1 more comment
std::cin.failbit
is a constant that indicates which of the error bits represents stream failure. It is not an indication of the stream's current state. To check if that bit is set, use the member function std::cin.fail()
instead.
However, if the stream failed to read due to reaching the end of the stream fail()
will return false
leading you to believe that it succeeded. Prefer std::cin.good()
to check if the last operation succeeded.
Documentation link
– user4581301
Jan 3 at 20:41
I appreciate the answer but for me the part about failbit just interferes with getline description link 4th block here states: "The failbit flag is set if the function extracts no characters, or if the delimiting character is not found once (n-1) characters have already been written to s". So for me it's confusing
– keptt
Jan 3 at 20:57
@keptt The error state is stored in a bitset.failbit
is a constant that indicates which of the bits represents failure. For example, it's value might be the constant8
indicating that the 4th bit indicates failure. In that instance, yourif
would be essentiallyif(8)
and doesn't account for the current state of your stream. An example of a use forfailbit
might bestd::cin.rdstate() & std::ios_base::failbit
to see if thefailbit
is1
in the bitset returned byrdstate()
.
– François Andrieux
Jan 3 at 20:59
thanks, I guess I get it now. I tried to act on your advise and used cin.good() - it worked just fine so far. Not to be too annoying but would you mind me asking about dealing with failbit? If it's a constant then it should have some kind of default value meaning if I type smth like - if(cin.failbit == default_value) it would mean that no errors that can trigger failbit were detected and everything is fine. Is it like that?
– keptt
Jan 3 at 21:17
@keptt No.failbit
has nothing to do withcin
's state. Imagine it's a global constant (because that's what it is). There is no useful information you can extract from it, it's a number and it's always that number for the lifetime of your program. Imagine everywhere you seefailbit
that it instead just says4
, or16
or whatever value it has. It cannot change during the lifetime of your program. It's only use is as a bitmask to help you interpret the value returned byrdstate()
. The fact thatstd::cin.failbit
compiles is misleading.failbit
is astatic constexpr
.
– François Andrieux
Jan 3 at 21:19
|
show 1 more comment
std::cin.failbit
is a constant that indicates which of the error bits represents stream failure. It is not an indication of the stream's current state. To check if that bit is set, use the member function std::cin.fail()
instead.
However, if the stream failed to read due to reaching the end of the stream fail()
will return false
leading you to believe that it succeeded. Prefer std::cin.good()
to check if the last operation succeeded.
std::cin.failbit
is a constant that indicates which of the error bits represents stream failure. It is not an indication of the stream's current state. To check if that bit is set, use the member function std::cin.fail()
instead.
However, if the stream failed to read due to reaching the end of the stream fail()
will return false
leading you to believe that it succeeded. Prefer std::cin.good()
to check if the last operation succeeded.
answered Jan 3 at 20:39


François AndrieuxFrançois Andrieux
16.4k32850
16.4k32850
Documentation link
– user4581301
Jan 3 at 20:41
I appreciate the answer but for me the part about failbit just interferes with getline description link 4th block here states: "The failbit flag is set if the function extracts no characters, or if the delimiting character is not found once (n-1) characters have already been written to s". So for me it's confusing
– keptt
Jan 3 at 20:57
@keptt The error state is stored in a bitset.failbit
is a constant that indicates which of the bits represents failure. For example, it's value might be the constant8
indicating that the 4th bit indicates failure. In that instance, yourif
would be essentiallyif(8)
and doesn't account for the current state of your stream. An example of a use forfailbit
might bestd::cin.rdstate() & std::ios_base::failbit
to see if thefailbit
is1
in the bitset returned byrdstate()
.
– François Andrieux
Jan 3 at 20:59
thanks, I guess I get it now. I tried to act on your advise and used cin.good() - it worked just fine so far. Not to be too annoying but would you mind me asking about dealing with failbit? If it's a constant then it should have some kind of default value meaning if I type smth like - if(cin.failbit == default_value) it would mean that no errors that can trigger failbit were detected and everything is fine. Is it like that?
– keptt
Jan 3 at 21:17
@keptt No.failbit
has nothing to do withcin
's state. Imagine it's a global constant (because that's what it is). There is no useful information you can extract from it, it's a number and it's always that number for the lifetime of your program. Imagine everywhere you seefailbit
that it instead just says4
, or16
or whatever value it has. It cannot change during the lifetime of your program. It's only use is as a bitmask to help you interpret the value returned byrdstate()
. The fact thatstd::cin.failbit
compiles is misleading.failbit
is astatic constexpr
.
– François Andrieux
Jan 3 at 21:19
|
show 1 more comment
Documentation link
– user4581301
Jan 3 at 20:41
I appreciate the answer but for me the part about failbit just interferes with getline description link 4th block here states: "The failbit flag is set if the function extracts no characters, or if the delimiting character is not found once (n-1) characters have already been written to s". So for me it's confusing
– keptt
Jan 3 at 20:57
@keptt The error state is stored in a bitset.failbit
is a constant that indicates which of the bits represents failure. For example, it's value might be the constant8
indicating that the 4th bit indicates failure. In that instance, yourif
would be essentiallyif(8)
and doesn't account for the current state of your stream. An example of a use forfailbit
might bestd::cin.rdstate() & std::ios_base::failbit
to see if thefailbit
is1
in the bitset returned byrdstate()
.
– François Andrieux
Jan 3 at 20:59
thanks, I guess I get it now. I tried to act on your advise and used cin.good() - it worked just fine so far. Not to be too annoying but would you mind me asking about dealing with failbit? If it's a constant then it should have some kind of default value meaning if I type smth like - if(cin.failbit == default_value) it would mean that no errors that can trigger failbit were detected and everything is fine. Is it like that?
– keptt
Jan 3 at 21:17
@keptt No.failbit
has nothing to do withcin
's state. Imagine it's a global constant (because that's what it is). There is no useful information you can extract from it, it's a number and it's always that number for the lifetime of your program. Imagine everywhere you seefailbit
that it instead just says4
, or16
or whatever value it has. It cannot change during the lifetime of your program. It's only use is as a bitmask to help you interpret the value returned byrdstate()
. The fact thatstd::cin.failbit
compiles is misleading.failbit
is astatic constexpr
.
– François Andrieux
Jan 3 at 21:19
Documentation link
– user4581301
Jan 3 at 20:41
Documentation link
– user4581301
Jan 3 at 20:41
I appreciate the answer but for me the part about failbit just interferes with getline description link 4th block here states: "The failbit flag is set if the function extracts no characters, or if the delimiting character is not found once (n-1) characters have already been written to s". So for me it's confusing
– keptt
Jan 3 at 20:57
I appreciate the answer but for me the part about failbit just interferes with getline description link 4th block here states: "The failbit flag is set if the function extracts no characters, or if the delimiting character is not found once (n-1) characters have already been written to s". So for me it's confusing
– keptt
Jan 3 at 20:57
@keptt The error state is stored in a bitset.
failbit
is a constant that indicates which of the bits represents failure. For example, it's value might be the constant 8
indicating that the 4th bit indicates failure. In that instance, your if
would be essentially if(8)
and doesn't account for the current state of your stream. An example of a use for failbit
might be std::cin.rdstate() & std::ios_base::failbit
to see if the failbit
is 1
in the bitset returned by rdstate()
.– François Andrieux
Jan 3 at 20:59
@keptt The error state is stored in a bitset.
failbit
is a constant that indicates which of the bits represents failure. For example, it's value might be the constant 8
indicating that the 4th bit indicates failure. In that instance, your if
would be essentially if(8)
and doesn't account for the current state of your stream. An example of a use for failbit
might be std::cin.rdstate() & std::ios_base::failbit
to see if the failbit
is 1
in the bitset returned by rdstate()
.– François Andrieux
Jan 3 at 20:59
thanks, I guess I get it now. I tried to act on your advise and used cin.good() - it worked just fine so far. Not to be too annoying but would you mind me asking about dealing with failbit? If it's a constant then it should have some kind of default value meaning if I type smth like - if(cin.failbit == default_value) it would mean that no errors that can trigger failbit were detected and everything is fine. Is it like that?
– keptt
Jan 3 at 21:17
thanks, I guess I get it now. I tried to act on your advise and used cin.good() - it worked just fine so far. Not to be too annoying but would you mind me asking about dealing with failbit? If it's a constant then it should have some kind of default value meaning if I type smth like - if(cin.failbit == default_value) it would mean that no errors that can trigger failbit were detected and everything is fine. Is it like that?
– keptt
Jan 3 at 21:17
@keptt No.
failbit
has nothing to do with cin
's state. Imagine it's a global constant (because that's what it is). There is no useful information you can extract from it, it's a number and it's always that number for the lifetime of your program. Imagine everywhere you see failbit
that it instead just says 4
, or 16
or whatever value it has. It cannot change during the lifetime of your program. It's only use is as a bitmask to help you interpret the value returned by rdstate()
. The fact that std::cin.failbit
compiles is misleading. failbit
is a static constexpr
.– François Andrieux
Jan 3 at 21:19
@keptt No.
failbit
has nothing to do with cin
's state. Imagine it's a global constant (because that's what it is). There is no useful information you can extract from it, it's a number and it's always that number for the lifetime of your program. Imagine everywhere you see failbit
that it instead just says 4
, or 16
or whatever value it has. It cannot change during the lifetime of your program. It's only use is as a bitmask to help you interpret the value returned by rdstate()
. The fact that std::cin.failbit
compiles is misleading. failbit
is a static constexpr
.– François Andrieux
Jan 3 at 21:19
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54029350%2fwhy-is-cin-failbit-always-set-even-when-input-is-valid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NLkP,k4llWSTVjWDyzcpxNgMzL8uSQ sbDVJSHqlEw42VYoGV3AvDs0M,CG0iy 8Q
@fran please answer in answer not comments.
– Yakk - Adam Nevraumont
Jan 3 at 20:33
FWIW you can use
if (!std::cin)
instead to check if the stream is in a failure state.– NathanOliver
Jan 3 at 20:33
3
I was mistaken, there is no
failbit
member function. It's checking that the value of the failbit bit mask isn't zero. I was thinking of thefail()
member function.std::cin.failbit
is a constant that indicates which bit represents a failed state, it's not checking the state of the stream.– François Andrieux
Jan 3 at 20:33
thanks for replies. I considered using cin.fail() or !cin but they are not invoked when user's input is longer than the len of the array. User's input just gets cut and all characters past the size of array remain in the buffer (at least that is what I observed)
– keptt
Jan 3 at 20:42
1
@keptt No,
failbit
is not a flag in the stateful sense. It indicates which bit is associated with that flag. It cannot be set or cleared, it's a constant. The phrase "thefailbit
flag" means "the bit indicated by the bitmaskfailbit
".– François Andrieux
Jan 3 at 21:04