Three.js: Handling per-instance texture offsets with instanced geometry
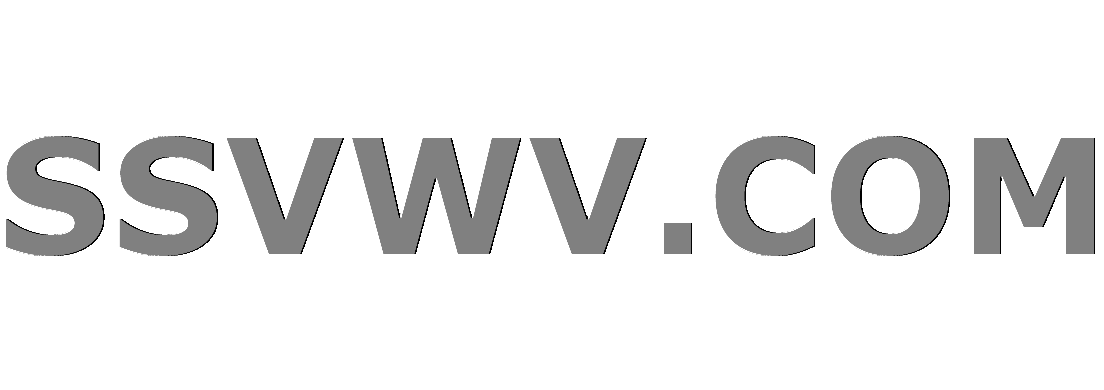
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
My question in brief: Are there any examples of how one can render textures with custom (non grid-like) offsets using indexed, instanced quads in Three.js?
My question with context: I am working on a Three.js scene with lots of quads, each of which is meant to display an image from an image atlas. Each image in the atlas is 32px on each side. The quads are rendered using an indexed, instanced geometry; so there are 4 vertices that define the "prototype" for all quad instances, and all instance-specific information is supplied to a RawShaderMaterial
as attributes.
The challenge is that the image to be represented on a given quad is contained in a 32px square in the atlas, but those little images can have margins. So while the images form a grid of sorts in the atlas, each has a known margin of unused pixels at the top and sides (sample atlas region):
The relevant attributes that handle these margins are:
var geometry = new THREE.InstancedBufferGeometry();
// uv identifies the blueprint uv values
geometry.addAttribute('uv', new THREE.BufferAttribute(new Float32Array([ 1, 1, 0, 1, 0, 0, 1, 0, ]), 2));
// texOffset = the offset of an instance's 32px cell in the atlas {x,y}
geometry.addAttribute('texOffset', new IBA(... 2, true, 1));
// uvOffset = the left/right and top/bottom margins of an instance's image in its 32px cell
geometry.addAttribute('uvOffset', new IBA(..., 2, true, 1));
Then inside the fragment shader I can render the full 32px cell for the instance with:
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
My question is: How can I render just the subset of a 32px square that contains the image, assuming I can create a vec2 uvOffset
that includes the left and top offsets for the image in its 32px cell container? Any help others can offer with this question would be greatly appreciated.
three.js
add a comment |
My question in brief: Are there any examples of how one can render textures with custom (non grid-like) offsets using indexed, instanced quads in Three.js?
My question with context: I am working on a Three.js scene with lots of quads, each of which is meant to display an image from an image atlas. Each image in the atlas is 32px on each side. The quads are rendered using an indexed, instanced geometry; so there are 4 vertices that define the "prototype" for all quad instances, and all instance-specific information is supplied to a RawShaderMaterial
as attributes.
The challenge is that the image to be represented on a given quad is contained in a 32px square in the atlas, but those little images can have margins. So while the images form a grid of sorts in the atlas, each has a known margin of unused pixels at the top and sides (sample atlas region):
The relevant attributes that handle these margins are:
var geometry = new THREE.InstancedBufferGeometry();
// uv identifies the blueprint uv values
geometry.addAttribute('uv', new THREE.BufferAttribute(new Float32Array([ 1, 1, 0, 1, 0, 0, 1, 0, ]), 2));
// texOffset = the offset of an instance's 32px cell in the atlas {x,y}
geometry.addAttribute('texOffset', new IBA(... 2, true, 1));
// uvOffset = the left/right and top/bottom margins of an instance's image in its 32px cell
geometry.addAttribute('uvOffset', new IBA(..., 2, true, 1));
Then inside the fragment shader I can render the full 32px cell for the instance with:
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
My question is: How can I render just the subset of a 32px square that contains the image, assuming I can create a vec2 uvOffset
that includes the left and top offsets for the image in its 32px cell container? Any help others can offer with this question would be greatly appreciated.
three.js
1
What do you mean "How can I render just the subset of a 32px square that contains the image"? Do you want anything in these black margins to simply be transparent instead of black?
– Marquizzo
Jan 3 at 23:06
Well right now my quads have black margins on the top/bottom or left/right because they're sampling from the full 32px by 32px cell that corresponds to a given instance. But I want the quad to only show the non-margin portion of the 32px cell. I don't want to discard the black pixels (that led to troubles with GPU picking); I want to just paint the full quad with a subset of the given 32px by 32px cell. Does that make sense?
– duhaime
Jan 4 at 14:23
add a comment |
My question in brief: Are there any examples of how one can render textures with custom (non grid-like) offsets using indexed, instanced quads in Three.js?
My question with context: I am working on a Three.js scene with lots of quads, each of which is meant to display an image from an image atlas. Each image in the atlas is 32px on each side. The quads are rendered using an indexed, instanced geometry; so there are 4 vertices that define the "prototype" for all quad instances, and all instance-specific information is supplied to a RawShaderMaterial
as attributes.
The challenge is that the image to be represented on a given quad is contained in a 32px square in the atlas, but those little images can have margins. So while the images form a grid of sorts in the atlas, each has a known margin of unused pixels at the top and sides (sample atlas region):
The relevant attributes that handle these margins are:
var geometry = new THREE.InstancedBufferGeometry();
// uv identifies the blueprint uv values
geometry.addAttribute('uv', new THREE.BufferAttribute(new Float32Array([ 1, 1, 0, 1, 0, 0, 1, 0, ]), 2));
// texOffset = the offset of an instance's 32px cell in the atlas {x,y}
geometry.addAttribute('texOffset', new IBA(... 2, true, 1));
// uvOffset = the left/right and top/bottom margins of an instance's image in its 32px cell
geometry.addAttribute('uvOffset', new IBA(..., 2, true, 1));
Then inside the fragment shader I can render the full 32px cell for the instance with:
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
My question is: How can I render just the subset of a 32px square that contains the image, assuming I can create a vec2 uvOffset
that includes the left and top offsets for the image in its 32px cell container? Any help others can offer with this question would be greatly appreciated.
three.js
My question in brief: Are there any examples of how one can render textures with custom (non grid-like) offsets using indexed, instanced quads in Three.js?
My question with context: I am working on a Three.js scene with lots of quads, each of which is meant to display an image from an image atlas. Each image in the atlas is 32px on each side. The quads are rendered using an indexed, instanced geometry; so there are 4 vertices that define the "prototype" for all quad instances, and all instance-specific information is supplied to a RawShaderMaterial
as attributes.
The challenge is that the image to be represented on a given quad is contained in a 32px square in the atlas, but those little images can have margins. So while the images form a grid of sorts in the atlas, each has a known margin of unused pixels at the top and sides (sample atlas region):
The relevant attributes that handle these margins are:
var geometry = new THREE.InstancedBufferGeometry();
// uv identifies the blueprint uv values
geometry.addAttribute('uv', new THREE.BufferAttribute(new Float32Array([ 1, 1, 0, 1, 0, 0, 1, 0, ]), 2));
// texOffset = the offset of an instance's 32px cell in the atlas {x,y}
geometry.addAttribute('texOffset', new IBA(... 2, true, 1));
// uvOffset = the left/right and top/bottom margins of an instance's image in its 32px cell
geometry.addAttribute('uvOffset', new IBA(..., 2, true, 1));
Then inside the fragment shader I can render the full 32px cell for the instance with:
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
My question is: How can I render just the subset of a 32px square that contains the image, assuming I can create a vec2 uvOffset
that includes the left and top offsets for the image in its 32px cell container? Any help others can offer with this question would be greatly appreciated.
three.js
three.js
asked Jan 3 at 20:20


duhaimeduhaime
9,16456087
9,16456087
1
What do you mean "How can I render just the subset of a 32px square that contains the image"? Do you want anything in these black margins to simply be transparent instead of black?
– Marquizzo
Jan 3 at 23:06
Well right now my quads have black margins on the top/bottom or left/right because they're sampling from the full 32px by 32px cell that corresponds to a given instance. But I want the quad to only show the non-margin portion of the 32px cell. I don't want to discard the black pixels (that led to troubles with GPU picking); I want to just paint the full quad with a subset of the given 32px by 32px cell. Does that make sense?
– duhaime
Jan 4 at 14:23
add a comment |
1
What do you mean "How can I render just the subset of a 32px square that contains the image"? Do you want anything in these black margins to simply be transparent instead of black?
– Marquizzo
Jan 3 at 23:06
Well right now my quads have black margins on the top/bottom or left/right because they're sampling from the full 32px by 32px cell that corresponds to a given instance. But I want the quad to only show the non-margin portion of the 32px cell. I don't want to discard the black pixels (that led to troubles with GPU picking); I want to just paint the full quad with a subset of the given 32px by 32px cell. Does that make sense?
– duhaime
Jan 4 at 14:23
1
1
What do you mean "How can I render just the subset of a 32px square that contains the image"? Do you want anything in these black margins to simply be transparent instead of black?
– Marquizzo
Jan 3 at 23:06
What do you mean "How can I render just the subset of a 32px square that contains the image"? Do you want anything in these black margins to simply be transparent instead of black?
– Marquizzo
Jan 3 at 23:06
Well right now my quads have black margins on the top/bottom or left/right because they're sampling from the full 32px by 32px cell that corresponds to a given instance. But I want the quad to only show the non-margin portion of the 32px cell. I don't want to discard the black pixels (that led to troubles with GPU picking); I want to just paint the full quad with a subset of the given 32px by 32px cell. Does that make sense?
– duhaime
Jan 4 at 14:23
Well right now my quads have black margins on the top/bottom or left/right because they're sampling from the full 32px by 32px cell that corresponds to a given instance. But I want the quad to only show the non-margin portion of the 32px cell. I don't want to discard the black pixels (that led to troubles with GPU picking); I want to just paint the full quad with a subset of the given 32px by 32px cell. Does that make sense?
– duhaime
Jan 4 at 14:23
add a comment |
1 Answer
1
active
oldest
votes
The range of UVs is [0.0, 1.0], so let's say you want a margin of 0.1 on left/right sides. This leaves you with a visible range of [0.1, 0.9]
. To make the margins invisible, you can use the step()
function to create an opacity curve such as this one:
Anything below 0.1 and anything above 0.9 will have an opacity of 0.
// You first declare the range of the margin (this has x and y)
vec2 uvMargin = vec2(0.1, 0.2);
// Anything outside the margin will have 0 opacity,
// anything inside the margin will have 1 opacity
// First, you apply a step() function to the left X value
float opacity = step(uvMargin.x, vUV.x);
// then to the right X value (1.0 - margin)
opacity *= step(vUV.x, 1.0 - uvMargin.x);
// then the top Y value
opacity *= step(uvMargin.y, vUV.y)
// then the bottom Y value
opacity *= step(vUV.y, 1.0 - uvMargin.y);
// Sample the texture
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
// Apply opacity to alpha
gl_FragColor.a = opacity;
This can be optimized, but I took it step-by-step for clarity. When you set transparent: true
to your material, you'll see that anything outside the margin is invisible.
- If you find problems with GPU picking, maybe you could add an indistinguishable amount to opacity, like
gl_FragColor.a = opacity + 0.01;
- If you find aliasing or jagged edges that you don't like, you could try
smoothstep()
instead. - You'll have to declare the uvMargin as an
InstancedBufferAttribute
instead of doing it in the shader like I did.
Thanks for this @Marquizzo. I'm only anxious about GPU picking behavior. If I add "an indistinguishable amount" to opacity, will that not influence GPU picking? If that were the case, do you have any sense why I might have had troubles GPU picking when using textured GL_POINTS (which discarded altogether the margins)?
– duhaime
Jan 4 at 23:37
@duhaime I've never done GPU picking on transparent objects before, so I'm not sure what kind of problem you could be running into, sorry. But if the transparency is the issue, I recommended not going fully transparent, so instead ofalpha = 0
, it would bealpha = 0.01
and possibly that would fix it? I'm not sure.
– Marquizzo
Jan 8 at 18:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54029252%2fthree-js-handling-per-instance-texture-offsets-with-instanced-geometry%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The range of UVs is [0.0, 1.0], so let's say you want a margin of 0.1 on left/right sides. This leaves you with a visible range of [0.1, 0.9]
. To make the margins invisible, you can use the step()
function to create an opacity curve such as this one:
Anything below 0.1 and anything above 0.9 will have an opacity of 0.
// You first declare the range of the margin (this has x and y)
vec2 uvMargin = vec2(0.1, 0.2);
// Anything outside the margin will have 0 opacity,
// anything inside the margin will have 1 opacity
// First, you apply a step() function to the left X value
float opacity = step(uvMargin.x, vUV.x);
// then to the right X value (1.0 - margin)
opacity *= step(vUV.x, 1.0 - uvMargin.x);
// then the top Y value
opacity *= step(uvMargin.y, vUV.y)
// then the bottom Y value
opacity *= step(vUV.y, 1.0 - uvMargin.y);
// Sample the texture
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
// Apply opacity to alpha
gl_FragColor.a = opacity;
This can be optimized, but I took it step-by-step for clarity. When you set transparent: true
to your material, you'll see that anything outside the margin is invisible.
- If you find problems with GPU picking, maybe you could add an indistinguishable amount to opacity, like
gl_FragColor.a = opacity + 0.01;
- If you find aliasing or jagged edges that you don't like, you could try
smoothstep()
instead. - You'll have to declare the uvMargin as an
InstancedBufferAttribute
instead of doing it in the shader like I did.
Thanks for this @Marquizzo. I'm only anxious about GPU picking behavior. If I add "an indistinguishable amount" to opacity, will that not influence GPU picking? If that were the case, do you have any sense why I might have had troubles GPU picking when using textured GL_POINTS (which discarded altogether the margins)?
– duhaime
Jan 4 at 23:37
@duhaime I've never done GPU picking on transparent objects before, so I'm not sure what kind of problem you could be running into, sorry. But if the transparency is the issue, I recommended not going fully transparent, so instead ofalpha = 0
, it would bealpha = 0.01
and possibly that would fix it? I'm not sure.
– Marquizzo
Jan 8 at 18:00
add a comment |
The range of UVs is [0.0, 1.0], so let's say you want a margin of 0.1 on left/right sides. This leaves you with a visible range of [0.1, 0.9]
. To make the margins invisible, you can use the step()
function to create an opacity curve such as this one:
Anything below 0.1 and anything above 0.9 will have an opacity of 0.
// You first declare the range of the margin (this has x and y)
vec2 uvMargin = vec2(0.1, 0.2);
// Anything outside the margin will have 0 opacity,
// anything inside the margin will have 1 opacity
// First, you apply a step() function to the left X value
float opacity = step(uvMargin.x, vUV.x);
// then to the right X value (1.0 - margin)
opacity *= step(vUV.x, 1.0 - uvMargin.x);
// then the top Y value
opacity *= step(uvMargin.y, vUV.y)
// then the bottom Y value
opacity *= step(vUV.y, 1.0 - uvMargin.y);
// Sample the texture
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
// Apply opacity to alpha
gl_FragColor.a = opacity;
This can be optimized, but I took it step-by-step for clarity. When you set transparent: true
to your material, you'll see that anything outside the margin is invisible.
- If you find problems with GPU picking, maybe you could add an indistinguishable amount to opacity, like
gl_FragColor.a = opacity + 0.01;
- If you find aliasing or jagged edges that you don't like, you could try
smoothstep()
instead. - You'll have to declare the uvMargin as an
InstancedBufferAttribute
instead of doing it in the shader like I did.
Thanks for this @Marquizzo. I'm only anxious about GPU picking behavior. If I add "an indistinguishable amount" to opacity, will that not influence GPU picking? If that were the case, do you have any sense why I might have had troubles GPU picking when using textured GL_POINTS (which discarded altogether the margins)?
– duhaime
Jan 4 at 23:37
@duhaime I've never done GPU picking on transparent objects before, so I'm not sure what kind of problem you could be running into, sorry. But if the transparency is the issue, I recommended not going fully transparent, so instead ofalpha = 0
, it would bealpha = 0.01
and possibly that would fix it? I'm not sure.
– Marquizzo
Jan 8 at 18:00
add a comment |
The range of UVs is [0.0, 1.0], so let's say you want a margin of 0.1 on left/right sides. This leaves you with a visible range of [0.1, 0.9]
. To make the margins invisible, you can use the step()
function to create an opacity curve such as this one:
Anything below 0.1 and anything above 0.9 will have an opacity of 0.
// You first declare the range of the margin (this has x and y)
vec2 uvMargin = vec2(0.1, 0.2);
// Anything outside the margin will have 0 opacity,
// anything inside the margin will have 1 opacity
// First, you apply a step() function to the left X value
float opacity = step(uvMargin.x, vUV.x);
// then to the right X value (1.0 - margin)
opacity *= step(vUV.x, 1.0 - uvMargin.x);
// then the top Y value
opacity *= step(uvMargin.y, vUV.y)
// then the bottom Y value
opacity *= step(vUV.y, 1.0 - uvMargin.y);
// Sample the texture
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
// Apply opacity to alpha
gl_FragColor.a = opacity;
This can be optimized, but I took it step-by-step for clarity. When you set transparent: true
to your material, you'll see that anything outside the margin is invisible.
- If you find problems with GPU picking, maybe you could add an indistinguishable amount to opacity, like
gl_FragColor.a = opacity + 0.01;
- If you find aliasing or jagged edges that you don't like, you could try
smoothstep()
instead. - You'll have to declare the uvMargin as an
InstancedBufferAttribute
instead of doing it in the shader like I did.
The range of UVs is [0.0, 1.0], so let's say you want a margin of 0.1 on left/right sides. This leaves you with a visible range of [0.1, 0.9]
. To make the margins invisible, you can use the step()
function to create an opacity curve such as this one:
Anything below 0.1 and anything above 0.9 will have an opacity of 0.
// You first declare the range of the margin (this has x and y)
vec2 uvMargin = vec2(0.1, 0.2);
// Anything outside the margin will have 0 opacity,
// anything inside the margin will have 1 opacity
// First, you apply a step() function to the left X value
float opacity = step(uvMargin.x, vUV.x);
// then to the right X value (1.0 - margin)
opacity *= step(vUV.x, 1.0 - uvMargin.x);
// then the top Y value
opacity *= step(uvMargin.y, vUV.y)
// then the bottom Y value
opacity *= step(vUV.y, 1.0 - uvMargin.y);
// Sample the texture
vec2 scaledUv = vUv + vTextureOffset;
gl_FragColor = texture2D(texIdx, scaledUv);
// Apply opacity to alpha
gl_FragColor.a = opacity;
This can be optimized, but I took it step-by-step for clarity. When you set transparent: true
to your material, you'll see that anything outside the margin is invisible.
- If you find problems with GPU picking, maybe you could add an indistinguishable amount to opacity, like
gl_FragColor.a = opacity + 0.01;
- If you find aliasing or jagged edges that you don't like, you could try
smoothstep()
instead. - You'll have to declare the uvMargin as an
InstancedBufferAttribute
instead of doing it in the shader like I did.
edited Jan 4 at 18:12
answered Jan 4 at 18:04


MarquizzoMarquizzo
6,61152045
6,61152045
Thanks for this @Marquizzo. I'm only anxious about GPU picking behavior. If I add "an indistinguishable amount" to opacity, will that not influence GPU picking? If that were the case, do you have any sense why I might have had troubles GPU picking when using textured GL_POINTS (which discarded altogether the margins)?
– duhaime
Jan 4 at 23:37
@duhaime I've never done GPU picking on transparent objects before, so I'm not sure what kind of problem you could be running into, sorry. But if the transparency is the issue, I recommended not going fully transparent, so instead ofalpha = 0
, it would bealpha = 0.01
and possibly that would fix it? I'm not sure.
– Marquizzo
Jan 8 at 18:00
add a comment |
Thanks for this @Marquizzo. I'm only anxious about GPU picking behavior. If I add "an indistinguishable amount" to opacity, will that not influence GPU picking? If that were the case, do you have any sense why I might have had troubles GPU picking when using textured GL_POINTS (which discarded altogether the margins)?
– duhaime
Jan 4 at 23:37
@duhaime I've never done GPU picking on transparent objects before, so I'm not sure what kind of problem you could be running into, sorry. But if the transparency is the issue, I recommended not going fully transparent, so instead ofalpha = 0
, it would bealpha = 0.01
and possibly that would fix it? I'm not sure.
– Marquizzo
Jan 8 at 18:00
Thanks for this @Marquizzo. I'm only anxious about GPU picking behavior. If I add "an indistinguishable amount" to opacity, will that not influence GPU picking? If that were the case, do you have any sense why I might have had troubles GPU picking when using textured GL_POINTS (which discarded altogether the margins)?
– duhaime
Jan 4 at 23:37
Thanks for this @Marquizzo. I'm only anxious about GPU picking behavior. If I add "an indistinguishable amount" to opacity, will that not influence GPU picking? If that were the case, do you have any sense why I might have had troubles GPU picking when using textured GL_POINTS (which discarded altogether the margins)?
– duhaime
Jan 4 at 23:37
@duhaime I've never done GPU picking on transparent objects before, so I'm not sure what kind of problem you could be running into, sorry. But if the transparency is the issue, I recommended not going fully transparent, so instead of
alpha = 0
, it would be alpha = 0.01
and possibly that would fix it? I'm not sure.– Marquizzo
Jan 8 at 18:00
@duhaime I've never done GPU picking on transparent objects before, so I'm not sure what kind of problem you could be running into, sorry. But if the transparency is the issue, I recommended not going fully transparent, so instead of
alpha = 0
, it would be alpha = 0.01
and possibly that would fix it? I'm not sure.– Marquizzo
Jan 8 at 18:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54029252%2fthree-js-handling-per-instance-texture-offsets-with-instanced-geometry%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qimUm1,tJgVoiu,E
1
What do you mean "How can I render just the subset of a 32px square that contains the image"? Do you want anything in these black margins to simply be transparent instead of black?
– Marquizzo
Jan 3 at 23:06
Well right now my quads have black margins on the top/bottom or left/right because they're sampling from the full 32px by 32px cell that corresponds to a given instance. But I want the quad to only show the non-margin portion of the 32px cell. I don't want to discard the black pixels (that led to troubles with GPU picking); I want to just paint the full quad with a subset of the given 32px by 32px cell. Does that make sense?
– duhaime
Jan 4 at 14:23