Trying to figure out how to make GET request on Vue.js using Axios but failing to do so
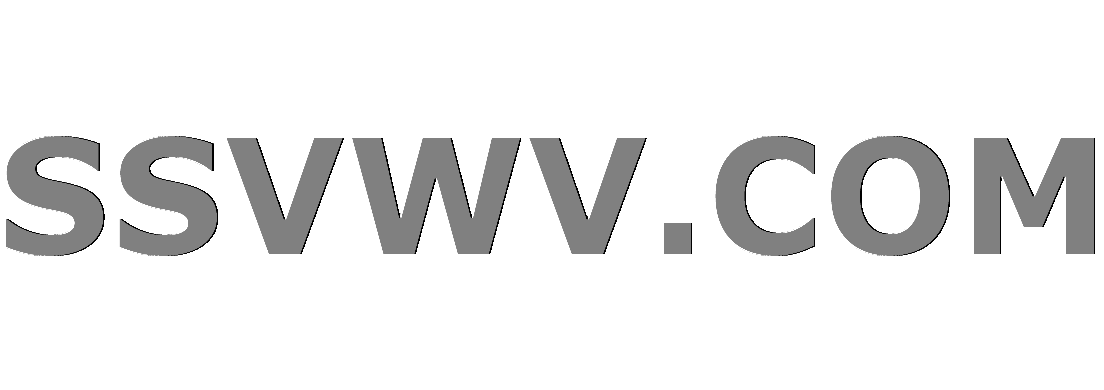
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
My Vue project was created using webpack-simple template so I only have main.js and App.vue in my src folder right now. Main.js looks like this:
import Vue from 'vue'
import App from './App.vue'
new Vue({
el: '#app',
render: h => h(App)
})
and App.vue looks like this:
<template>
<div id="app">
</div>
</template>
<script>
import axios from 'axios';
axios.defaults.baseURL = 'https://www.bungie.net/Platform';
axios.defaults.headers.common = {
'X-API-Key': 'ecab73fd6c714d02b64f0c75503671d1'
};
export default {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
}
</script>
<style lang="scss">
</style>
I am following Axios's Github page instructions on how to do the GET request but I seem to be doing something wrong. My goal is to make a GET request to:
https://www.bungie.net/Platform/User/GetBungieNetUserById/1/
which can be done only if I have a X-API-Key header.
For this reason I've set
axios.defaults.baseURL = 'https://www.bungie.net/Platform';
axios.defaults.headers.common = {
'X-API-Key': 'ecab73fd6c714d02b64f0c75503671d1'
};
And then I'm making the GET request. Sadly I'm getting a failed to compile error:
./node_modules/babel-loader/lib!./node_modules/vue-loader/lib/selector.js?type=script&index=0!./src/App.vue
Module build failed: SyntaxError: C:/MAMP/htdocs/Destiny/src/App.vue: Unexpected token, expected , (14:7)
I'm quite new to Vue so the mistake I am making might be really silly. I'd appreciate any help in finding it.
vue.js vuejs2 axios
add a comment |
My Vue project was created using webpack-simple template so I only have main.js and App.vue in my src folder right now. Main.js looks like this:
import Vue from 'vue'
import App from './App.vue'
new Vue({
el: '#app',
render: h => h(App)
})
and App.vue looks like this:
<template>
<div id="app">
</div>
</template>
<script>
import axios from 'axios';
axios.defaults.baseURL = 'https://www.bungie.net/Platform';
axios.defaults.headers.common = {
'X-API-Key': 'ecab73fd6c714d02b64f0c75503671d1'
};
export default {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
}
</script>
<style lang="scss">
</style>
I am following Axios's Github page instructions on how to do the GET request but I seem to be doing something wrong. My goal is to make a GET request to:
https://www.bungie.net/Platform/User/GetBungieNetUserById/1/
which can be done only if I have a X-API-Key header.
For this reason I've set
axios.defaults.baseURL = 'https://www.bungie.net/Platform';
axios.defaults.headers.common = {
'X-API-Key': 'ecab73fd6c714d02b64f0c75503671d1'
};
And then I'm making the GET request. Sadly I'm getting a failed to compile error:
./node_modules/babel-loader/lib!./node_modules/vue-loader/lib/selector.js?type=script&index=0!./src/App.vue
Module build failed: SyntaxError: C:/MAMP/htdocs/Destiny/src/App.vue: Unexpected token, expected , (14:7)
I'm quite new to Vue so the mistake I am making might be really silly. I'd appreciate any help in finding it.
vue.js vuejs2 axios
add a comment |
My Vue project was created using webpack-simple template so I only have main.js and App.vue in my src folder right now. Main.js looks like this:
import Vue from 'vue'
import App from './App.vue'
new Vue({
el: '#app',
render: h => h(App)
})
and App.vue looks like this:
<template>
<div id="app">
</div>
</template>
<script>
import axios from 'axios';
axios.defaults.baseURL = 'https://www.bungie.net/Platform';
axios.defaults.headers.common = {
'X-API-Key': 'ecab73fd6c714d02b64f0c75503671d1'
};
export default {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
}
</script>
<style lang="scss">
</style>
I am following Axios's Github page instructions on how to do the GET request but I seem to be doing something wrong. My goal is to make a GET request to:
https://www.bungie.net/Platform/User/GetBungieNetUserById/1/
which can be done only if I have a X-API-Key header.
For this reason I've set
axios.defaults.baseURL = 'https://www.bungie.net/Platform';
axios.defaults.headers.common = {
'X-API-Key': 'ecab73fd6c714d02b64f0c75503671d1'
};
And then I'm making the GET request. Sadly I'm getting a failed to compile error:
./node_modules/babel-loader/lib!./node_modules/vue-loader/lib/selector.js?type=script&index=0!./src/App.vue
Module build failed: SyntaxError: C:/MAMP/htdocs/Destiny/src/App.vue: Unexpected token, expected , (14:7)
I'm quite new to Vue so the mistake I am making might be really silly. I'd appreciate any help in finding it.
vue.js vuejs2 axios
My Vue project was created using webpack-simple template so I only have main.js and App.vue in my src folder right now. Main.js looks like this:
import Vue from 'vue'
import App from './App.vue'
new Vue({
el: '#app',
render: h => h(App)
})
and App.vue looks like this:
<template>
<div id="app">
</div>
</template>
<script>
import axios from 'axios';
axios.defaults.baseURL = 'https://www.bungie.net/Platform';
axios.defaults.headers.common = {
'X-API-Key': 'ecab73fd6c714d02b64f0c75503671d1'
};
export default {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
}
</script>
<style lang="scss">
</style>
I am following Axios's Github page instructions on how to do the GET request but I seem to be doing something wrong. My goal is to make a GET request to:
https://www.bungie.net/Platform/User/GetBungieNetUserById/1/
which can be done only if I have a X-API-Key header.
For this reason I've set
axios.defaults.baseURL = 'https://www.bungie.net/Platform';
axios.defaults.headers.common = {
'X-API-Key': 'ecab73fd6c714d02b64f0c75503671d1'
};
And then I'm making the GET request. Sadly I'm getting a failed to compile error:
./node_modules/babel-loader/lib!./node_modules/vue-loader/lib/selector.js?type=script&index=0!./src/App.vue
Module build failed: SyntaxError: C:/MAMP/htdocs/Destiny/src/App.vue: Unexpected token, expected , (14:7)
I'm quite new to Vue so the mistake I am making might be really silly. I'd appreciate any help in finding it.
vue.js vuejs2 axios
vue.js vuejs2 axios
asked Jan 3 at 20:21
BobimaruBobimaru
9761515
9761515
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Assuming you want to run this code when the Vue component is mounted:
export default {
mounted() {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
},
}
You can't just slap raw JS in the export default
block - Vue expects a component definition, with various properties, some of which may be/contain functions.
Oh yes, this is indeed the case.
– Bobimaru
Jan 3 at 20:46
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54029268%2ftrying-to-figure-out-how-to-make-get-request-on-vue-js-using-axios-but-failing-t%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Assuming you want to run this code when the Vue component is mounted:
export default {
mounted() {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
},
}
You can't just slap raw JS in the export default
block - Vue expects a component definition, with various properties, some of which may be/contain functions.
Oh yes, this is indeed the case.
– Bobimaru
Jan 3 at 20:46
add a comment |
Assuming you want to run this code when the Vue component is mounted:
export default {
mounted() {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
},
}
You can't just slap raw JS in the export default
block - Vue expects a component definition, with various properties, some of which may be/contain functions.
Oh yes, this is indeed the case.
– Bobimaru
Jan 3 at 20:46
add a comment |
Assuming you want to run this code when the Vue component is mounted:
export default {
mounted() {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
},
}
You can't just slap raw JS in the export default
block - Vue expects a component definition, with various properties, some of which may be/contain functions.
Assuming you want to run this code when the Vue component is mounted:
export default {
mounted() {
axios.get('/User/GetBungieNetUserById/1/')
.then(function(response) {
console.log(response.data);
console.log(response.status);
});
},
}
You can't just slap raw JS in the export default
block - Vue expects a component definition, with various properties, some of which may be/contain functions.
answered Jan 3 at 20:42
ceejayozceejayoz
144k34225311
144k34225311
Oh yes, this is indeed the case.
– Bobimaru
Jan 3 at 20:46
add a comment |
Oh yes, this is indeed the case.
– Bobimaru
Jan 3 at 20:46
Oh yes, this is indeed the case.
– Bobimaru
Jan 3 at 20:46
Oh yes, this is indeed the case.
– Bobimaru
Jan 3 at 20:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54029268%2ftrying-to-figure-out-how-to-make-get-request-on-vue-js-using-axios-but-failing-t%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vcm jGstgSYE,Rs4MXcKbh wgfS,Nb AP BSh879 k8DGFsQgmQdY0Os,Imj rqT