How to print a christmas tree in Java, by calling methods?
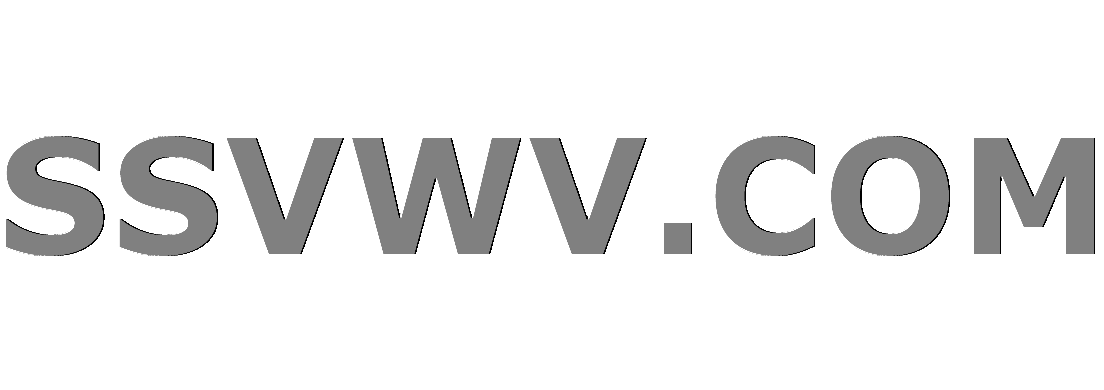
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I neeed help with the following problem.
This program has three outputs: one triangle, and two christmas trees. I have been successful with printing the triangle, but I can't figure out how to get my tree work. Here is the link to the exercise 40: https://materiaalit.github.io/2013-oo-programming/part1/week-2/
Please note, this is not my hoomework. I am learning how to code by myself, at home.
Here is the code:
public static void xmasTree(int height) {
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - 1);
printStars( i );
}
for( int j = 2; j <= height; j++ ){
printWhitespaces ( height - j );
printStars ( j - 1 );
}
}
This must be the final output:
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
***
***
But I get this:
*
**
***
****
*****
******
*******
********
*********
**********
*
**
***
****
*****
******
*******
********
*********
java
add a comment |
I neeed help with the following problem.
This program has three outputs: one triangle, and two christmas trees. I have been successful with printing the triangle, but I can't figure out how to get my tree work. Here is the link to the exercise 40: https://materiaalit.github.io/2013-oo-programming/part1/week-2/
Please note, this is not my hoomework. I am learning how to code by myself, at home.
Here is the code:
public static void xmasTree(int height) {
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - 1);
printStars( i );
}
for( int j = 2; j <= height; j++ ){
printWhitespaces ( height - j );
printStars ( j - 1 );
}
}
This must be the final output:
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
***
***
But I get this:
*
**
***
****
*****
******
*******
********
*********
**********
*
**
***
****
*****
******
*******
********
*********
java
add a comment |
I neeed help with the following problem.
This program has three outputs: one triangle, and two christmas trees. I have been successful with printing the triangle, but I can't figure out how to get my tree work. Here is the link to the exercise 40: https://materiaalit.github.io/2013-oo-programming/part1/week-2/
Please note, this is not my hoomework. I am learning how to code by myself, at home.
Here is the code:
public static void xmasTree(int height) {
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - 1);
printStars( i );
}
for( int j = 2; j <= height; j++ ){
printWhitespaces ( height - j );
printStars ( j - 1 );
}
}
This must be the final output:
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
***
***
But I get this:
*
**
***
****
*****
******
*******
********
*********
**********
*
**
***
****
*****
******
*******
********
*********
java
I neeed help with the following problem.
This program has three outputs: one triangle, and two christmas trees. I have been successful with printing the triangle, but I can't figure out how to get my tree work. Here is the link to the exercise 40: https://materiaalit.github.io/2013-oo-programming/part1/week-2/
Please note, this is not my hoomework. I am learning how to code by myself, at home.
Here is the code:
public static void xmasTree(int height) {
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - 1);
printStars( i );
}
for( int j = 2; j <= height; j++ ){
printWhitespaces ( height - j );
printStars ( j - 1 );
}
}
This must be the final output:
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
***
***
But I get this:
*
**
***
****
*****
******
*******
********
*********
**********
*
**
***
****
*****
******
*******
********
*********
java
java
edited Jan 4 at 4:59


Rajendra arora
1,51211018
1,51211018
asked Jan 4 at 4:52
MrdoMrdo
86
86
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Write down what you need to do.
Each line consist of spaces for indentation, and stars for the tree, so write down how many of each you need.
Since you'll be using a loop, e.g. counting from 0
to height - 1
, write down the iterator value too
height = 10
* 9 spaces, 1 stars, i = 0
*** 8 spaces, 3 stars, i = 1
***** 7 spaces, 5 stars, i = 2
******* 6 spaces, 7 stars, i = 3
********* 5 spaces, 9 stars, i = 4
*********** 4 spaces, 11 stars, i = 5
************* 3 spaces, 13 stars, i = 6
*************** 2 spaces, 15 stars, i = 7
***************** 1 spaces, 17 stars, i = 8
******************* 0 spaces, 19 stars, i = 9
*** 8 spaces, 3 stars
*** 8 spaces, 3 stars
Now see if you can discern the pattern:
spaces = height - 1 - i
stars = 2 * i + 1
For the trunk, you loop 2
times, use height - 2
spaces, and 3
stars, unless of course the trunk needs to somehow size with the tree height, but you didn't specify any rules for that.
thanks a lot!!! thank you for your detailed explaination.
– Mrdo
Jan 12 at 1:47
add a comment |
First of all, get used to using 0 as the first value in your loops, not 1. It takes some getting used to but it'll feel natural after 5 or 6 years :-)
You've certainly got a logic problem there. Each line for the top part of the tree should start at height - i -1
(not height - 1
) and contain i * 2 + 1
stars (not i
).
Similar issue with the trunk but I'll leave that with you as an exercise (since that's the point!).
Or, you could incrementi
by2
on every iteration.
– Elliott Frisch
Jan 4 at 5:01
add a comment |
public static void xmasTree(int height) {
// cone
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - i);
printStars( i );
printStars( i-1 );
System.out.println();
}
// trunk
for(int i=1 ; i<=4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
Explanation:
For printing the cone:
____h-1__*__h-1____
// h-i. Here, the number of stars = 2i-1. The star at position i becomes the midpoint
___h-1__***___h-1__
__h-2__*****__h-2__
and so on
For the trunk (assuming width changes):
__h/4__*****__h/4__
// width of the trunk = h/2
__h/4__*****__h/4__
and so on.
But as stated in the other answers, better to start with 0 in loops:
public static void xmasTree(int height) {
// cone
for (int i = 0; i < height; i++ ) {
printWhitespaces(height - i - 1);
printStars( i+1 );
printStars( i );
System.out.println();
}
// trunk
for(int i = 0 ; i<4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54033289%2fhow-to-print-a-christmas-tree-in-java-by-calling-methods%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Write down what you need to do.
Each line consist of spaces for indentation, and stars for the tree, so write down how many of each you need.
Since you'll be using a loop, e.g. counting from 0
to height - 1
, write down the iterator value too
height = 10
* 9 spaces, 1 stars, i = 0
*** 8 spaces, 3 stars, i = 1
***** 7 spaces, 5 stars, i = 2
******* 6 spaces, 7 stars, i = 3
********* 5 spaces, 9 stars, i = 4
*********** 4 spaces, 11 stars, i = 5
************* 3 spaces, 13 stars, i = 6
*************** 2 spaces, 15 stars, i = 7
***************** 1 spaces, 17 stars, i = 8
******************* 0 spaces, 19 stars, i = 9
*** 8 spaces, 3 stars
*** 8 spaces, 3 stars
Now see if you can discern the pattern:
spaces = height - 1 - i
stars = 2 * i + 1
For the trunk, you loop 2
times, use height - 2
spaces, and 3
stars, unless of course the trunk needs to somehow size with the tree height, but you didn't specify any rules for that.
thanks a lot!!! thank you for your detailed explaination.
– Mrdo
Jan 12 at 1:47
add a comment |
Write down what you need to do.
Each line consist of spaces for indentation, and stars for the tree, so write down how many of each you need.
Since you'll be using a loop, e.g. counting from 0
to height - 1
, write down the iterator value too
height = 10
* 9 spaces, 1 stars, i = 0
*** 8 spaces, 3 stars, i = 1
***** 7 spaces, 5 stars, i = 2
******* 6 spaces, 7 stars, i = 3
********* 5 spaces, 9 stars, i = 4
*********** 4 spaces, 11 stars, i = 5
************* 3 spaces, 13 stars, i = 6
*************** 2 spaces, 15 stars, i = 7
***************** 1 spaces, 17 stars, i = 8
******************* 0 spaces, 19 stars, i = 9
*** 8 spaces, 3 stars
*** 8 spaces, 3 stars
Now see if you can discern the pattern:
spaces = height - 1 - i
stars = 2 * i + 1
For the trunk, you loop 2
times, use height - 2
spaces, and 3
stars, unless of course the trunk needs to somehow size with the tree height, but you didn't specify any rules for that.
thanks a lot!!! thank you for your detailed explaination.
– Mrdo
Jan 12 at 1:47
add a comment |
Write down what you need to do.
Each line consist of spaces for indentation, and stars for the tree, so write down how many of each you need.
Since you'll be using a loop, e.g. counting from 0
to height - 1
, write down the iterator value too
height = 10
* 9 spaces, 1 stars, i = 0
*** 8 spaces, 3 stars, i = 1
***** 7 spaces, 5 stars, i = 2
******* 6 spaces, 7 stars, i = 3
********* 5 spaces, 9 stars, i = 4
*********** 4 spaces, 11 stars, i = 5
************* 3 spaces, 13 stars, i = 6
*************** 2 spaces, 15 stars, i = 7
***************** 1 spaces, 17 stars, i = 8
******************* 0 spaces, 19 stars, i = 9
*** 8 spaces, 3 stars
*** 8 spaces, 3 stars
Now see if you can discern the pattern:
spaces = height - 1 - i
stars = 2 * i + 1
For the trunk, you loop 2
times, use height - 2
spaces, and 3
stars, unless of course the trunk needs to somehow size with the tree height, but you didn't specify any rules for that.
Write down what you need to do.
Each line consist of spaces for indentation, and stars for the tree, so write down how many of each you need.
Since you'll be using a loop, e.g. counting from 0
to height - 1
, write down the iterator value too
height = 10
* 9 spaces, 1 stars, i = 0
*** 8 spaces, 3 stars, i = 1
***** 7 spaces, 5 stars, i = 2
******* 6 spaces, 7 stars, i = 3
********* 5 spaces, 9 stars, i = 4
*********** 4 spaces, 11 stars, i = 5
************* 3 spaces, 13 stars, i = 6
*************** 2 spaces, 15 stars, i = 7
***************** 1 spaces, 17 stars, i = 8
******************* 0 spaces, 19 stars, i = 9
*** 8 spaces, 3 stars
*** 8 spaces, 3 stars
Now see if you can discern the pattern:
spaces = height - 1 - i
stars = 2 * i + 1
For the trunk, you loop 2
times, use height - 2
spaces, and 3
stars, unless of course the trunk needs to somehow size with the tree height, but you didn't specify any rules for that.
answered Jan 4 at 5:11


AndreasAndreas
79.9k465129
79.9k465129
thanks a lot!!! thank you for your detailed explaination.
– Mrdo
Jan 12 at 1:47
add a comment |
thanks a lot!!! thank you for your detailed explaination.
– Mrdo
Jan 12 at 1:47
thanks a lot!!! thank you for your detailed explaination.
– Mrdo
Jan 12 at 1:47
thanks a lot!!! thank you for your detailed explaination.
– Mrdo
Jan 12 at 1:47
add a comment |
First of all, get used to using 0 as the first value in your loops, not 1. It takes some getting used to but it'll feel natural after 5 or 6 years :-)
You've certainly got a logic problem there. Each line for the top part of the tree should start at height - i -1
(not height - 1
) and contain i * 2 + 1
stars (not i
).
Similar issue with the trunk but I'll leave that with you as an exercise (since that's the point!).
Or, you could incrementi
by2
on every iteration.
– Elliott Frisch
Jan 4 at 5:01
add a comment |
First of all, get used to using 0 as the first value in your loops, not 1. It takes some getting used to but it'll feel natural after 5 or 6 years :-)
You've certainly got a logic problem there. Each line for the top part of the tree should start at height - i -1
(not height - 1
) and contain i * 2 + 1
stars (not i
).
Similar issue with the trunk but I'll leave that with you as an exercise (since that's the point!).
Or, you could incrementi
by2
on every iteration.
– Elliott Frisch
Jan 4 at 5:01
add a comment |
First of all, get used to using 0 as the first value in your loops, not 1. It takes some getting used to but it'll feel natural after 5 or 6 years :-)
You've certainly got a logic problem there. Each line for the top part of the tree should start at height - i -1
(not height - 1
) and contain i * 2 + 1
stars (not i
).
Similar issue with the trunk but I'll leave that with you as an exercise (since that's the point!).
First of all, get used to using 0 as the first value in your loops, not 1. It takes some getting used to but it'll feel natural after 5 or 6 years :-)
You've certainly got a logic problem there. Each line for the top part of the tree should start at height - i -1
(not height - 1
) and contain i * 2 + 1
stars (not i
).
Similar issue with the trunk but I'll leave that with you as an exercise (since that's the point!).
edited Jan 4 at 9:42
answered Jan 4 at 5:00
sprintersprinter
16.9k42961
16.9k42961
Or, you could incrementi
by2
on every iteration.
– Elliott Frisch
Jan 4 at 5:01
add a comment |
Or, you could incrementi
by2
on every iteration.
– Elliott Frisch
Jan 4 at 5:01
Or, you could increment
i
by 2
on every iteration.– Elliott Frisch
Jan 4 at 5:01
Or, you could increment
i
by 2
on every iteration.– Elliott Frisch
Jan 4 at 5:01
add a comment |
public static void xmasTree(int height) {
// cone
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - i);
printStars( i );
printStars( i-1 );
System.out.println();
}
// trunk
for(int i=1 ; i<=4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
Explanation:
For printing the cone:
____h-1__*__h-1____
// h-i. Here, the number of stars = 2i-1. The star at position i becomes the midpoint
___h-1__***___h-1__
__h-2__*****__h-2__
and so on
For the trunk (assuming width changes):
__h/4__*****__h/4__
// width of the trunk = h/2
__h/4__*****__h/4__
and so on.
But as stated in the other answers, better to start with 0 in loops:
public static void xmasTree(int height) {
// cone
for (int i = 0; i < height; i++ ) {
printWhitespaces(height - i - 1);
printStars( i+1 );
printStars( i );
System.out.println();
}
// trunk
for(int i = 0 ; i<4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
add a comment |
public static void xmasTree(int height) {
// cone
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - i);
printStars( i );
printStars( i-1 );
System.out.println();
}
// trunk
for(int i=1 ; i<=4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
Explanation:
For printing the cone:
____h-1__*__h-1____
// h-i. Here, the number of stars = 2i-1. The star at position i becomes the midpoint
___h-1__***___h-1__
__h-2__*****__h-2__
and so on
For the trunk (assuming width changes):
__h/4__*****__h/4__
// width of the trunk = h/2
__h/4__*****__h/4__
and so on.
But as stated in the other answers, better to start with 0 in loops:
public static void xmasTree(int height) {
// cone
for (int i = 0; i < height; i++ ) {
printWhitespaces(height - i - 1);
printStars( i+1 );
printStars( i );
System.out.println();
}
// trunk
for(int i = 0 ; i<4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
add a comment |
public static void xmasTree(int height) {
// cone
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - i);
printStars( i );
printStars( i-1 );
System.out.println();
}
// trunk
for(int i=1 ; i<=4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
Explanation:
For printing the cone:
____h-1__*__h-1____
// h-i. Here, the number of stars = 2i-1. The star at position i becomes the midpoint
___h-1__***___h-1__
__h-2__*****__h-2__
and so on
For the trunk (assuming width changes):
__h/4__*****__h/4__
// width of the trunk = h/2
__h/4__*****__h/4__
and so on.
But as stated in the other answers, better to start with 0 in loops:
public static void xmasTree(int height) {
// cone
for (int i = 0; i < height; i++ ) {
printWhitespaces(height - i - 1);
printStars( i+1 );
printStars( i );
System.out.println();
}
// trunk
for(int i = 0 ; i<4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
public static void xmasTree(int height) {
// cone
for (int i = 1; i <= height; i++ ) {
printWhitespaces(height - i);
printStars( i );
printStars( i-1 );
System.out.println();
}
// trunk
for(int i=1 ; i<=4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
Explanation:
For printing the cone:
____h-1__*__h-1____
// h-i. Here, the number of stars = 2i-1. The star at position i becomes the midpoint
___h-1__***___h-1__
__h-2__*****__h-2__
and so on
For the trunk (assuming width changes):
__h/4__*****__h/4__
// width of the trunk = h/2
__h/4__*****__h/4__
and so on.
But as stated in the other answers, better to start with 0 in loops:
public static void xmasTree(int height) {
// cone
for (int i = 0; i < height; i++ ) {
printWhitespaces(height - i - 1);
printStars( i+1 );
printStars( i );
System.out.println();
}
// trunk
for(int i = 0 ; i<4; i++){
printWhitespaces(height - height/4 -1);
printStars(height/2);
System.out.println();
}
}
edited Jan 4 at 5:27
answered Jan 4 at 5:19
Susmit AgrawalSusmit Agrawal
979514
979514
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54033289%2fhow-to-print-a-christmas-tree-in-java-by-calling-methods%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s5,ZAv69eGY1b9h,VUJ9I8s3Fd2m4b5eEvrjOEB9W emXDxjlEjyDu