How do I make my modal close in x seconds?
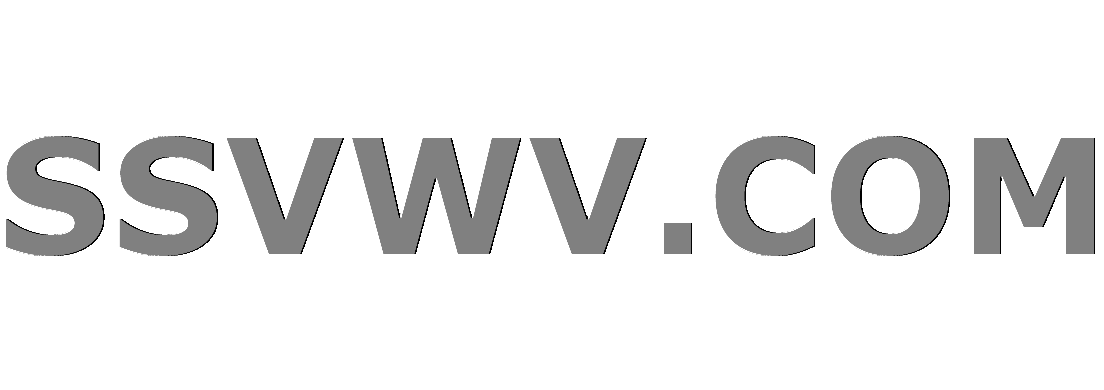
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am using ReactJS and a Bootstrap modal. I can open the modal just fine, but I would like it to close after 3 seconds.
I tried setTimeout as you can see below, but it doesn't close. I gave setTimeout a callback of handleClose, but after console logging, I can see that handleClose is not being called.
Here is the ItemDetailView Component:
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { Card, CardImg, CardText, CardBody,
CardTitle, CardSubtitle } from 'reactstrap';
import { addToCart } from '../actions/addToCartAction';
import './ItemDetailView.css';
import ItemAddedModal from './ItemAddedModal';
class ItemDetailView extends Component {
constructor(props) {
super(props);
this.state = {
modalOpen: false
}
// this.toggle = this.toggle.bind(this);
};
// toggle() {
// this.setState({
// modalOpen: !this.state.modalOpen
// });
// };
handleOpen = () => {
console.log("Cart Open", this.state.modalOpen);
this.setState({
modalOpen: true
},() => {setTimeout(this.handleClose(), 3000)});
// setTimeout(this.handleClose(), 3000);
};
handleClose = () => {
this.setState({
modalOpen: false
});
console.log('handleClose fired!')
};
addToCartHandler = () => {
this.props.addToCart(this.props.card);
console.log('addToCart++', this.props.quantity);
this.handleOpen()
// this.setState({
// modalOpen: true
// });
};
render() {
if (!this.props.title) {
return null;
}
return (
<div className="detail-view-wrapper">
<Card className="text-center detail-view-card">
{/* <CardImg top width="100%" src={"/" + this.props.img} alt={this.props.title} /> */}
<CardImg className="detail-view-img" top width="100%" src={"/" + this.props.img} alt={this.props.title} />
<CardBody>
<CardTitle className={"card-title"}>{this.props.title}</CardTitle>
<CardSubtitle>${this.props.price}</CardSubtitle>
<CardText>{this.props.description}</CardText>
{/* <SvgIcon className="cart-icon" onClick={() => this.addToCartHandler()} >
<AddShoppingCart />
</SvgIcon> */}
<button className= "add-to-cart-button" onClick={() => this.addToCartHandler()}>Add To Cart</button>
</CardBody>
</Card>
<ItemAddedModal open={this.state.modalOpen} toggle={this.toggle} />
</div>
);
}
}
const mapStateToProps = state => {
if (!state.data.cardData) {
return {
title: null,
img: null,
description: null,
price: null
}
}
const card = state.data.cardData[state.card.id]
return {
card: card,
title: card.title,
id: card.id,
img: card.img,
description: card.description,
price: card.price,
quantity: 0
};
}
export default connect(mapStateToProps, { addToCart })(ItemDetailView);
Here is the ItemAddedModal:
import React from 'react';
import { Modal, ModalHeader } from 'reactstrap';
import './ItemAddedModal.css';
class ItemAddedModal extends React.Component {
render () {
return (
<div>
<Modal className="item-added-modal" isOpen={this.props.open} toggle={this.props.toggle} className={this.props.className}>
<ModalHeader className="item-added-modal-header">
<p className="item-added-modal-p">Item Added To Cart</p>
</ModalHeader>
</Modal>
</div>
)
};
}
export default ItemAddedModal;
javascript reactjs twitter-bootstrap
|
show 1 more comment
I am using ReactJS and a Bootstrap modal. I can open the modal just fine, but I would like it to close after 3 seconds.
I tried setTimeout as you can see below, but it doesn't close. I gave setTimeout a callback of handleClose, but after console logging, I can see that handleClose is not being called.
Here is the ItemDetailView Component:
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { Card, CardImg, CardText, CardBody,
CardTitle, CardSubtitle } from 'reactstrap';
import { addToCart } from '../actions/addToCartAction';
import './ItemDetailView.css';
import ItemAddedModal from './ItemAddedModal';
class ItemDetailView extends Component {
constructor(props) {
super(props);
this.state = {
modalOpen: false
}
// this.toggle = this.toggle.bind(this);
};
// toggle() {
// this.setState({
// modalOpen: !this.state.modalOpen
// });
// };
handleOpen = () => {
console.log("Cart Open", this.state.modalOpen);
this.setState({
modalOpen: true
},() => {setTimeout(this.handleClose(), 3000)});
// setTimeout(this.handleClose(), 3000);
};
handleClose = () => {
this.setState({
modalOpen: false
});
console.log('handleClose fired!')
};
addToCartHandler = () => {
this.props.addToCart(this.props.card);
console.log('addToCart++', this.props.quantity);
this.handleOpen()
// this.setState({
// modalOpen: true
// });
};
render() {
if (!this.props.title) {
return null;
}
return (
<div className="detail-view-wrapper">
<Card className="text-center detail-view-card">
{/* <CardImg top width="100%" src={"/" + this.props.img} alt={this.props.title} /> */}
<CardImg className="detail-view-img" top width="100%" src={"/" + this.props.img} alt={this.props.title} />
<CardBody>
<CardTitle className={"card-title"}>{this.props.title}</CardTitle>
<CardSubtitle>${this.props.price}</CardSubtitle>
<CardText>{this.props.description}</CardText>
{/* <SvgIcon className="cart-icon" onClick={() => this.addToCartHandler()} >
<AddShoppingCart />
</SvgIcon> */}
<button className= "add-to-cart-button" onClick={() => this.addToCartHandler()}>Add To Cart</button>
</CardBody>
</Card>
<ItemAddedModal open={this.state.modalOpen} toggle={this.toggle} />
</div>
);
}
}
const mapStateToProps = state => {
if (!state.data.cardData) {
return {
title: null,
img: null,
description: null,
price: null
}
}
const card = state.data.cardData[state.card.id]
return {
card: card,
title: card.title,
id: card.id,
img: card.img,
description: card.description,
price: card.price,
quantity: 0
};
}
export default connect(mapStateToProps, { addToCart })(ItemDetailView);
Here is the ItemAddedModal:
import React from 'react';
import { Modal, ModalHeader } from 'reactstrap';
import './ItemAddedModal.css';
class ItemAddedModal extends React.Component {
render () {
return (
<div>
<Modal className="item-added-modal" isOpen={this.props.open} toggle={this.props.toggle} className={this.props.className}>
<ModalHeader className="item-added-modal-header">
<p className="item-added-modal-p">Item Added To Cart</p>
</ModalHeader>
</Modal>
</div>
)
};
}
export default ItemAddedModal;
javascript reactjs twitter-bootstrap
1
Are you suretoggle
is being called buthandleClose
is not? I would expect that iftoggle
is called,handleClose
would also be called, but then getTypeError: this.setState is not a function
.
– Amadan
Jan 4 at 5:12
Did you make sure to call toggle function you pass inside of theItemAddedModal
? Still no logs?
– Uma
Jan 4 at 5:16
Are you suretoggle
is invoked? It looks like you're opening the modal withaddToCartHandler
which doesn't set the timeout
– ic3b3rg
Jan 4 at 5:21
can you show theItemAddedModal
code?
– Thinker
Jan 4 at 5:26
Ok I edited the original post to include both components. You guys were right, toggle was not even being called so I commented it. As you can see I tried a few of the suggestions. The setState solution below is in effect now and I am getting the console log "handleClose fired" but the modal no longer shows. I guess that means the sequence is firing but there is another problem.
– frootloops
Jan 4 at 11:57
|
show 1 more comment
I am using ReactJS and a Bootstrap modal. I can open the modal just fine, but I would like it to close after 3 seconds.
I tried setTimeout as you can see below, but it doesn't close. I gave setTimeout a callback of handleClose, but after console logging, I can see that handleClose is not being called.
Here is the ItemDetailView Component:
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { Card, CardImg, CardText, CardBody,
CardTitle, CardSubtitle } from 'reactstrap';
import { addToCart } from '../actions/addToCartAction';
import './ItemDetailView.css';
import ItemAddedModal from './ItemAddedModal';
class ItemDetailView extends Component {
constructor(props) {
super(props);
this.state = {
modalOpen: false
}
// this.toggle = this.toggle.bind(this);
};
// toggle() {
// this.setState({
// modalOpen: !this.state.modalOpen
// });
// };
handleOpen = () => {
console.log("Cart Open", this.state.modalOpen);
this.setState({
modalOpen: true
},() => {setTimeout(this.handleClose(), 3000)});
// setTimeout(this.handleClose(), 3000);
};
handleClose = () => {
this.setState({
modalOpen: false
});
console.log('handleClose fired!')
};
addToCartHandler = () => {
this.props.addToCart(this.props.card);
console.log('addToCart++', this.props.quantity);
this.handleOpen()
// this.setState({
// modalOpen: true
// });
};
render() {
if (!this.props.title) {
return null;
}
return (
<div className="detail-view-wrapper">
<Card className="text-center detail-view-card">
{/* <CardImg top width="100%" src={"/" + this.props.img} alt={this.props.title} /> */}
<CardImg className="detail-view-img" top width="100%" src={"/" + this.props.img} alt={this.props.title} />
<CardBody>
<CardTitle className={"card-title"}>{this.props.title}</CardTitle>
<CardSubtitle>${this.props.price}</CardSubtitle>
<CardText>{this.props.description}</CardText>
{/* <SvgIcon className="cart-icon" onClick={() => this.addToCartHandler()} >
<AddShoppingCart />
</SvgIcon> */}
<button className= "add-to-cart-button" onClick={() => this.addToCartHandler()}>Add To Cart</button>
</CardBody>
</Card>
<ItemAddedModal open={this.state.modalOpen} toggle={this.toggle} />
</div>
);
}
}
const mapStateToProps = state => {
if (!state.data.cardData) {
return {
title: null,
img: null,
description: null,
price: null
}
}
const card = state.data.cardData[state.card.id]
return {
card: card,
title: card.title,
id: card.id,
img: card.img,
description: card.description,
price: card.price,
quantity: 0
};
}
export default connect(mapStateToProps, { addToCart })(ItemDetailView);
Here is the ItemAddedModal:
import React from 'react';
import { Modal, ModalHeader } from 'reactstrap';
import './ItemAddedModal.css';
class ItemAddedModal extends React.Component {
render () {
return (
<div>
<Modal className="item-added-modal" isOpen={this.props.open} toggle={this.props.toggle} className={this.props.className}>
<ModalHeader className="item-added-modal-header">
<p className="item-added-modal-p">Item Added To Cart</p>
</ModalHeader>
</Modal>
</div>
)
};
}
export default ItemAddedModal;
javascript reactjs twitter-bootstrap
I am using ReactJS and a Bootstrap modal. I can open the modal just fine, but I would like it to close after 3 seconds.
I tried setTimeout as you can see below, but it doesn't close. I gave setTimeout a callback of handleClose, but after console logging, I can see that handleClose is not being called.
Here is the ItemDetailView Component:
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { Card, CardImg, CardText, CardBody,
CardTitle, CardSubtitle } from 'reactstrap';
import { addToCart } from '../actions/addToCartAction';
import './ItemDetailView.css';
import ItemAddedModal from './ItemAddedModal';
class ItemDetailView extends Component {
constructor(props) {
super(props);
this.state = {
modalOpen: false
}
// this.toggle = this.toggle.bind(this);
};
// toggle() {
// this.setState({
// modalOpen: !this.state.modalOpen
// });
// };
handleOpen = () => {
console.log("Cart Open", this.state.modalOpen);
this.setState({
modalOpen: true
},() => {setTimeout(this.handleClose(), 3000)});
// setTimeout(this.handleClose(), 3000);
};
handleClose = () => {
this.setState({
modalOpen: false
});
console.log('handleClose fired!')
};
addToCartHandler = () => {
this.props.addToCart(this.props.card);
console.log('addToCart++', this.props.quantity);
this.handleOpen()
// this.setState({
// modalOpen: true
// });
};
render() {
if (!this.props.title) {
return null;
}
return (
<div className="detail-view-wrapper">
<Card className="text-center detail-view-card">
{/* <CardImg top width="100%" src={"/" + this.props.img} alt={this.props.title} /> */}
<CardImg className="detail-view-img" top width="100%" src={"/" + this.props.img} alt={this.props.title} />
<CardBody>
<CardTitle className={"card-title"}>{this.props.title}</CardTitle>
<CardSubtitle>${this.props.price}</CardSubtitle>
<CardText>{this.props.description}</CardText>
{/* <SvgIcon className="cart-icon" onClick={() => this.addToCartHandler()} >
<AddShoppingCart />
</SvgIcon> */}
<button className= "add-to-cart-button" onClick={() => this.addToCartHandler()}>Add To Cart</button>
</CardBody>
</Card>
<ItemAddedModal open={this.state.modalOpen} toggle={this.toggle} />
</div>
);
}
}
const mapStateToProps = state => {
if (!state.data.cardData) {
return {
title: null,
img: null,
description: null,
price: null
}
}
const card = state.data.cardData[state.card.id]
return {
card: card,
title: card.title,
id: card.id,
img: card.img,
description: card.description,
price: card.price,
quantity: 0
};
}
export default connect(mapStateToProps, { addToCart })(ItemDetailView);
Here is the ItemAddedModal:
import React from 'react';
import { Modal, ModalHeader } from 'reactstrap';
import './ItemAddedModal.css';
class ItemAddedModal extends React.Component {
render () {
return (
<div>
<Modal className="item-added-modal" isOpen={this.props.open} toggle={this.props.toggle} className={this.props.className}>
<ModalHeader className="item-added-modal-header">
<p className="item-added-modal-p">Item Added To Cart</p>
</ModalHeader>
</Modal>
</div>
)
};
}
export default ItemAddedModal;
javascript reactjs twitter-bootstrap
javascript reactjs twitter-bootstrap
edited Feb 26 at 21:40


halfer
14.8k759117
14.8k759117
asked Jan 4 at 5:04


frootloopsfrootloops
598
598
1
Are you suretoggle
is being called buthandleClose
is not? I would expect that iftoggle
is called,handleClose
would also be called, but then getTypeError: this.setState is not a function
.
– Amadan
Jan 4 at 5:12
Did you make sure to call toggle function you pass inside of theItemAddedModal
? Still no logs?
– Uma
Jan 4 at 5:16
Are you suretoggle
is invoked? It looks like you're opening the modal withaddToCartHandler
which doesn't set the timeout
– ic3b3rg
Jan 4 at 5:21
can you show theItemAddedModal
code?
– Thinker
Jan 4 at 5:26
Ok I edited the original post to include both components. You guys were right, toggle was not even being called so I commented it. As you can see I tried a few of the suggestions. The setState solution below is in effect now and I am getting the console log "handleClose fired" but the modal no longer shows. I guess that means the sequence is firing but there is another problem.
– frootloops
Jan 4 at 11:57
|
show 1 more comment
1
Are you suretoggle
is being called buthandleClose
is not? I would expect that iftoggle
is called,handleClose
would also be called, but then getTypeError: this.setState is not a function
.
– Amadan
Jan 4 at 5:12
Did you make sure to call toggle function you pass inside of theItemAddedModal
? Still no logs?
– Uma
Jan 4 at 5:16
Are you suretoggle
is invoked? It looks like you're opening the modal withaddToCartHandler
which doesn't set the timeout
– ic3b3rg
Jan 4 at 5:21
can you show theItemAddedModal
code?
– Thinker
Jan 4 at 5:26
Ok I edited the original post to include both components. You guys were right, toggle was not even being called so I commented it. As you can see I tried a few of the suggestions. The setState solution below is in effect now and I am getting the console log "handleClose fired" but the modal no longer shows. I guess that means the sequence is firing but there is another problem.
– frootloops
Jan 4 at 11:57
1
1
Are you sure
toggle
is being called but handleClose
is not? I would expect that if toggle
is called, handleClose
would also be called, but then get TypeError: this.setState is not a function
.– Amadan
Jan 4 at 5:12
Are you sure
toggle
is being called but handleClose
is not? I would expect that if toggle
is called, handleClose
would also be called, but then get TypeError: this.setState is not a function
.– Amadan
Jan 4 at 5:12
Did you make sure to call toggle function you pass inside of the
ItemAddedModal
? Still no logs?– Uma
Jan 4 at 5:16
Did you make sure to call toggle function you pass inside of the
ItemAddedModal
? Still no logs?– Uma
Jan 4 at 5:16
Are you sure
toggle
is invoked? It looks like you're opening the modal with addToCartHandler
which doesn't set the timeout– ic3b3rg
Jan 4 at 5:21
Are you sure
toggle
is invoked? It looks like you're opening the modal with addToCartHandler
which doesn't set the timeout– ic3b3rg
Jan 4 at 5:21
can you show the
ItemAddedModal
code?– Thinker
Jan 4 at 5:26
can you show the
ItemAddedModal
code?– Thinker
Jan 4 at 5:26
Ok I edited the original post to include both components. You guys were right, toggle was not even being called so I commented it. As you can see I tried a few of the suggestions. The setState solution below is in effect now and I am getting the console log "handleClose fired" but the modal no longer shows. I guess that means the sequence is firing but there is another problem.
– frootloops
Jan 4 at 11:57
Ok I edited the original post to include both components. You guys were right, toggle was not even being called so I commented it. As you can see I tried a few of the suggestions. The setState solution below is in effect now and I am getting the console log "handleClose fired" but the modal no longer shows. I guess that means the sequence is firing but there is another problem.
– frootloops
Jan 4 at 11:57
|
show 1 more comment
1 Answer
1
active
oldest
votes
To perform an action after a state is set, we need to pass a callback to setState.
this.setState({
modalOpen: true
},()=>{
console.log(this.state.modalOpen);});
Thanks for the help. Tried your callback and it seems to be connecting according to the console logs it is getting all the way to handleClose, but the modal no longer shows at all.
– frootloops
Jan 4 at 11:59
Nevermind, I got it! I was adding parentheses after handleClose:setTimeout(this.handleClose(), 3000);
but should besetTimeout(this.handleClose, 3000);
. Thanks a lot for all of your help guys!
– frootloops
Jan 4 at 12:13
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54033350%2fhow-do-i-make-my-modal-close-in-x-seconds%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
To perform an action after a state is set, we need to pass a callback to setState.
this.setState({
modalOpen: true
},()=>{
console.log(this.state.modalOpen);});
Thanks for the help. Tried your callback and it seems to be connecting according to the console logs it is getting all the way to handleClose, but the modal no longer shows at all.
– frootloops
Jan 4 at 11:59
Nevermind, I got it! I was adding parentheses after handleClose:setTimeout(this.handleClose(), 3000);
but should besetTimeout(this.handleClose, 3000);
. Thanks a lot for all of your help guys!
– frootloops
Jan 4 at 12:13
add a comment |
To perform an action after a state is set, we need to pass a callback to setState.
this.setState({
modalOpen: true
},()=>{
console.log(this.state.modalOpen);});
Thanks for the help. Tried your callback and it seems to be connecting according to the console logs it is getting all the way to handleClose, but the modal no longer shows at all.
– frootloops
Jan 4 at 11:59
Nevermind, I got it! I was adding parentheses after handleClose:setTimeout(this.handleClose(), 3000);
but should besetTimeout(this.handleClose, 3000);
. Thanks a lot for all of your help guys!
– frootloops
Jan 4 at 12:13
add a comment |
To perform an action after a state is set, we need to pass a callback to setState.
this.setState({
modalOpen: true
},()=>{
console.log(this.state.modalOpen);});
To perform an action after a state is set, we need to pass a callback to setState.
this.setState({
modalOpen: true
},()=>{
console.log(this.state.modalOpen);});
edited Jan 4 at 7:42


Bhargav Rao♦
31.1k2093114
31.1k2093114
answered Jan 4 at 6:11
Sathya PSathya P
725
725
Thanks for the help. Tried your callback and it seems to be connecting according to the console logs it is getting all the way to handleClose, but the modal no longer shows at all.
– frootloops
Jan 4 at 11:59
Nevermind, I got it! I was adding parentheses after handleClose:setTimeout(this.handleClose(), 3000);
but should besetTimeout(this.handleClose, 3000);
. Thanks a lot for all of your help guys!
– frootloops
Jan 4 at 12:13
add a comment |
Thanks for the help. Tried your callback and it seems to be connecting according to the console logs it is getting all the way to handleClose, but the modal no longer shows at all.
– frootloops
Jan 4 at 11:59
Nevermind, I got it! I was adding parentheses after handleClose:setTimeout(this.handleClose(), 3000);
but should besetTimeout(this.handleClose, 3000);
. Thanks a lot for all of your help guys!
– frootloops
Jan 4 at 12:13
Thanks for the help. Tried your callback and it seems to be connecting according to the console logs it is getting all the way to handleClose, but the modal no longer shows at all.
– frootloops
Jan 4 at 11:59
Thanks for the help. Tried your callback and it seems to be connecting according to the console logs it is getting all the way to handleClose, but the modal no longer shows at all.
– frootloops
Jan 4 at 11:59
Nevermind, I got it! I was adding parentheses after handleClose:
setTimeout(this.handleClose(), 3000);
but should be setTimeout(this.handleClose, 3000);
. Thanks a lot for all of your help guys!– frootloops
Jan 4 at 12:13
Nevermind, I got it! I was adding parentheses after handleClose:
setTimeout(this.handleClose(), 3000);
but should be setTimeout(this.handleClose, 3000);
. Thanks a lot for all of your help guys!– frootloops
Jan 4 at 12:13
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54033350%2fhow-do-i-make-my-modal-close-in-x-seconds%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RCl8Ol,kfn7XCJ2F ghI4 c5D1vi5q GYR1mVA,1W,e0otTHMkV,MV,qnu,IMVo7,QBjQsI
1
Are you sure
toggle
is being called buthandleClose
is not? I would expect that iftoggle
is called,handleClose
would also be called, but then getTypeError: this.setState is not a function
.– Amadan
Jan 4 at 5:12
Did you make sure to call toggle function you pass inside of the
ItemAddedModal
? Still no logs?– Uma
Jan 4 at 5:16
Are you sure
toggle
is invoked? It looks like you're opening the modal withaddToCartHandler
which doesn't set the timeout– ic3b3rg
Jan 4 at 5:21
can you show the
ItemAddedModal
code?– Thinker
Jan 4 at 5:26
Ok I edited the original post to include both components. You guys were right, toggle was not even being called so I commented it. As you can see I tried a few of the suggestions. The setState solution below is in effect now and I am getting the console log "handleClose fired" but the modal no longer shows. I guess that means the sequence is firing but there is another problem.
– frootloops
Jan 4 at 11:57