Singleton object context using Unity IoC in window forms whole application and database transactions problem
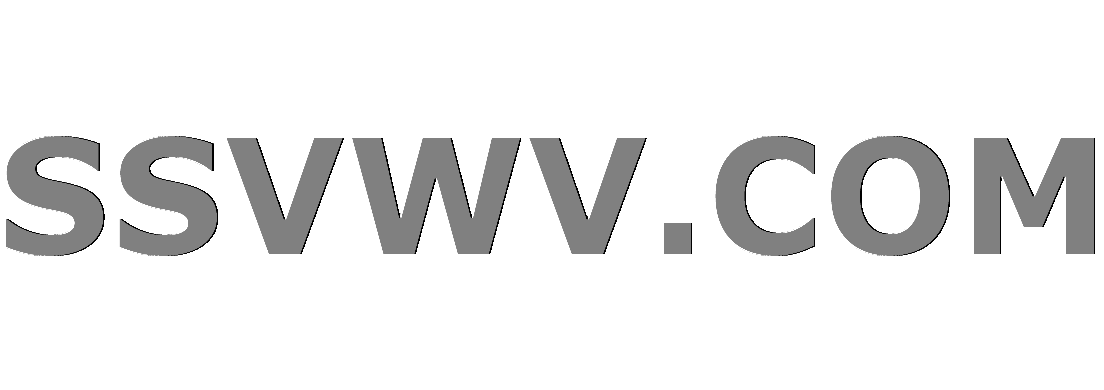
Multi tool use
I am using Unity IoC in C#, and DbContext with ContainerControlledLifetimeManager for application context with three layer architecture using entity framework, e.g.
This is a generic repository:
public interface IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
void Add(ModelClass entity);
void Delete(ModelClass entity);
void Update(ModelClass entity,int id);
bool Save();
void RefreshContext();
}
This is the Data Access layer interface:
public interface IShipmentAccess :
IRepository<ShipmentOrder, ShipmentOrderEntity>
{
List<ShipmentOrder> GetAllShipmentOrder();
List<ShipmentOrder> GetShipmentOrderWithId(int id);
bool UpdateFileExported(int id);
}
Implementation of DataAcess class
public class ShipmentOrderDataAccess : GenericRepository<ShipmentOrder,
ShipmentOrderEntity>, IShipmentAccess
{
ApplicationContext context;
public ShipmentOrderDataAccess(ApplicationContext context)
: base(context)
{
this.context = context;
}
}
Implementation of Service class
public class ShipmentOrderService : BaseService
{
ApplicationContext Context;
IShipmentAccess shipmentAccess;
PackingOrderService packingOrderService =
DependencyInjection.DependencyInjection.Retrieve<PackingOrderService>();
public ShipmentOrderService(ApplicationContext Context, IShipmentAccess
shipmentAccess)
{
this.Context = Context;
this.shipmentAccess = shipmentAccess;
}
}
And our dependency injection class looks like as follows:
Container.RegisterType<DbContext, LogisticsERP.DA.Entities.ApplicationContext>(
new ContainerControlledLifetimeManager());
Container.RegisterType<IShipmentAccess, ShipmentOrderDataAccess>();
But the problem here is that though we are using singleton life time manager (ContainerControlledLifetimeManager) of Unity and Entity Framework is is not loading updated data from database. I search on the Internet and found this solution to get updated data from database. Is it a correct solution?
public void RefreshContext()
{
foreach (var entity in context.ChangeTracker.Entries())
{
entity.Reload();
}
}
c# dependency-injection entity-framework-6 unity-container
add a comment |
I am using Unity IoC in C#, and DbContext with ContainerControlledLifetimeManager for application context with three layer architecture using entity framework, e.g.
This is a generic repository:
public interface IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
void Add(ModelClass entity);
void Delete(ModelClass entity);
void Update(ModelClass entity,int id);
bool Save();
void RefreshContext();
}
This is the Data Access layer interface:
public interface IShipmentAccess :
IRepository<ShipmentOrder, ShipmentOrderEntity>
{
List<ShipmentOrder> GetAllShipmentOrder();
List<ShipmentOrder> GetShipmentOrderWithId(int id);
bool UpdateFileExported(int id);
}
Implementation of DataAcess class
public class ShipmentOrderDataAccess : GenericRepository<ShipmentOrder,
ShipmentOrderEntity>, IShipmentAccess
{
ApplicationContext context;
public ShipmentOrderDataAccess(ApplicationContext context)
: base(context)
{
this.context = context;
}
}
Implementation of Service class
public class ShipmentOrderService : BaseService
{
ApplicationContext Context;
IShipmentAccess shipmentAccess;
PackingOrderService packingOrderService =
DependencyInjection.DependencyInjection.Retrieve<PackingOrderService>();
public ShipmentOrderService(ApplicationContext Context, IShipmentAccess
shipmentAccess)
{
this.Context = Context;
this.shipmentAccess = shipmentAccess;
}
}
And our dependency injection class looks like as follows:
Container.RegisterType<DbContext, LogisticsERP.DA.Entities.ApplicationContext>(
new ContainerControlledLifetimeManager());
Container.RegisterType<IShipmentAccess, ShipmentOrderDataAccess>();
But the problem here is that though we are using singleton life time manager (ContainerControlledLifetimeManager) of Unity and Entity Framework is is not loading updated data from database. I search on the Internet and found this solution to get updated data from database. Is it a correct solution?
public void RefreshContext()
{
foreach (var entity in context.ChangeTracker.Entries())
{
entity.Reload();
}
}
c# dependency-injection entity-framework-6 unity-container
1
You most likely misconfigured Unity by introducing a Captive Dependency. But unfortunately, there is no way for us to tell, as your code doesn't show the problem. Try creating a Minimal, Complete, and Verifiable Example.
– Steven
Jan 1 at 20:43
1
If you are using your DbContext as singleton and if other apllications or instances of your application also do updates on your database then this is an expected result. This is a known issue of EntityFramework. When you get an entity, it caches it and when you later read the entity from your DbContext, it provides the same object from cache. It has nothing to do with unity. Your solution works but I recommend you to google about "EntityFramework caching"
– NthDeveloper
Jan 1 at 21:18
Off-topic: why don't you injectpackingOrderService
?
– Gert Arnold
Jan 1 at 21:54
add a comment |
I am using Unity IoC in C#, and DbContext with ContainerControlledLifetimeManager for application context with three layer architecture using entity framework, e.g.
This is a generic repository:
public interface IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
void Add(ModelClass entity);
void Delete(ModelClass entity);
void Update(ModelClass entity,int id);
bool Save();
void RefreshContext();
}
This is the Data Access layer interface:
public interface IShipmentAccess :
IRepository<ShipmentOrder, ShipmentOrderEntity>
{
List<ShipmentOrder> GetAllShipmentOrder();
List<ShipmentOrder> GetShipmentOrderWithId(int id);
bool UpdateFileExported(int id);
}
Implementation of DataAcess class
public class ShipmentOrderDataAccess : GenericRepository<ShipmentOrder,
ShipmentOrderEntity>, IShipmentAccess
{
ApplicationContext context;
public ShipmentOrderDataAccess(ApplicationContext context)
: base(context)
{
this.context = context;
}
}
Implementation of Service class
public class ShipmentOrderService : BaseService
{
ApplicationContext Context;
IShipmentAccess shipmentAccess;
PackingOrderService packingOrderService =
DependencyInjection.DependencyInjection.Retrieve<PackingOrderService>();
public ShipmentOrderService(ApplicationContext Context, IShipmentAccess
shipmentAccess)
{
this.Context = Context;
this.shipmentAccess = shipmentAccess;
}
}
And our dependency injection class looks like as follows:
Container.RegisterType<DbContext, LogisticsERP.DA.Entities.ApplicationContext>(
new ContainerControlledLifetimeManager());
Container.RegisterType<IShipmentAccess, ShipmentOrderDataAccess>();
But the problem here is that though we are using singleton life time manager (ContainerControlledLifetimeManager) of Unity and Entity Framework is is not loading updated data from database. I search on the Internet and found this solution to get updated data from database. Is it a correct solution?
public void RefreshContext()
{
foreach (var entity in context.ChangeTracker.Entries())
{
entity.Reload();
}
}
c# dependency-injection entity-framework-6 unity-container
I am using Unity IoC in C#, and DbContext with ContainerControlledLifetimeManager for application context with three layer architecture using entity framework, e.g.
This is a generic repository:
public interface IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
void Add(ModelClass entity);
void Delete(ModelClass entity);
void Update(ModelClass entity,int id);
bool Save();
void RefreshContext();
}
This is the Data Access layer interface:
public interface IShipmentAccess :
IRepository<ShipmentOrder, ShipmentOrderEntity>
{
List<ShipmentOrder> GetAllShipmentOrder();
List<ShipmentOrder> GetShipmentOrderWithId(int id);
bool UpdateFileExported(int id);
}
Implementation of DataAcess class
public class ShipmentOrderDataAccess : GenericRepository<ShipmentOrder,
ShipmentOrderEntity>, IShipmentAccess
{
ApplicationContext context;
public ShipmentOrderDataAccess(ApplicationContext context)
: base(context)
{
this.context = context;
}
}
Implementation of Service class
public class ShipmentOrderService : BaseService
{
ApplicationContext Context;
IShipmentAccess shipmentAccess;
PackingOrderService packingOrderService =
DependencyInjection.DependencyInjection.Retrieve<PackingOrderService>();
public ShipmentOrderService(ApplicationContext Context, IShipmentAccess
shipmentAccess)
{
this.Context = Context;
this.shipmentAccess = shipmentAccess;
}
}
And our dependency injection class looks like as follows:
Container.RegisterType<DbContext, LogisticsERP.DA.Entities.ApplicationContext>(
new ContainerControlledLifetimeManager());
Container.RegisterType<IShipmentAccess, ShipmentOrderDataAccess>();
But the problem here is that though we are using singleton life time manager (ContainerControlledLifetimeManager) of Unity and Entity Framework is is not loading updated data from database. I search on the Internet and found this solution to get updated data from database. Is it a correct solution?
public void RefreshContext()
{
foreach (var entity in context.ChangeTracker.Entries())
{
entity.Reload();
}
}
c# dependency-injection entity-framework-6 unity-container
c# dependency-injection entity-framework-6 unity-container
edited Jan 3 at 6:58


Peter Mortensen
13.7k1986112
13.7k1986112
asked Jan 1 at 13:46


amir javaidamir javaid
11
11
1
You most likely misconfigured Unity by introducing a Captive Dependency. But unfortunately, there is no way for us to tell, as your code doesn't show the problem. Try creating a Minimal, Complete, and Verifiable Example.
– Steven
Jan 1 at 20:43
1
If you are using your DbContext as singleton and if other apllications or instances of your application also do updates on your database then this is an expected result. This is a known issue of EntityFramework. When you get an entity, it caches it and when you later read the entity from your DbContext, it provides the same object from cache. It has nothing to do with unity. Your solution works but I recommend you to google about "EntityFramework caching"
– NthDeveloper
Jan 1 at 21:18
Off-topic: why don't you injectpackingOrderService
?
– Gert Arnold
Jan 1 at 21:54
add a comment |
1
You most likely misconfigured Unity by introducing a Captive Dependency. But unfortunately, there is no way for us to tell, as your code doesn't show the problem. Try creating a Minimal, Complete, and Verifiable Example.
– Steven
Jan 1 at 20:43
1
If you are using your DbContext as singleton and if other apllications or instances of your application also do updates on your database then this is an expected result. This is a known issue of EntityFramework. When you get an entity, it caches it and when you later read the entity from your DbContext, it provides the same object from cache. It has nothing to do with unity. Your solution works but I recommend you to google about "EntityFramework caching"
– NthDeveloper
Jan 1 at 21:18
Off-topic: why don't you injectpackingOrderService
?
– Gert Arnold
Jan 1 at 21:54
1
1
You most likely misconfigured Unity by introducing a Captive Dependency. But unfortunately, there is no way for us to tell, as your code doesn't show the problem. Try creating a Minimal, Complete, and Verifiable Example.
– Steven
Jan 1 at 20:43
You most likely misconfigured Unity by introducing a Captive Dependency. But unfortunately, there is no way for us to tell, as your code doesn't show the problem. Try creating a Minimal, Complete, and Verifiable Example.
– Steven
Jan 1 at 20:43
1
1
If you are using your DbContext as singleton and if other apllications or instances of your application also do updates on your database then this is an expected result. This is a known issue of EntityFramework. When you get an entity, it caches it and when you later read the entity from your DbContext, it provides the same object from cache. It has nothing to do with unity. Your solution works but I recommend you to google about "EntityFramework caching"
– NthDeveloper
Jan 1 at 21:18
If you are using your DbContext as singleton and if other apllications or instances of your application also do updates on your database then this is an expected result. This is a known issue of EntityFramework. When you get an entity, it caches it and when you later read the entity from your DbContext, it provides the same object from cache. It has nothing to do with unity. Your solution works but I recommend you to google about "EntityFramework caching"
– NthDeveloper
Jan 1 at 21:18
Off-topic: why don't you inject
packingOrderService
?– Gert Arnold
Jan 1 at 21:54
Off-topic: why don't you inject
packingOrderService
?– Gert Arnold
Jan 1 at 21:54
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53995964%2fsingleton-object-context-using-unity-ioc-in-window-forms-whole-application-and-d%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53995964%2fsingleton-object-context-using-unity-ioc-in-window-forms-whole-application-and-d%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
z9Ds,wGoknr,TnVmlrLjehV,fPBGEB,Q5wiA,xL7uo2jH6XeYXq,eKP 130id1m,PXkoBP7V Ev6ZzWHTj NWP1oqSqR
1
You most likely misconfigured Unity by introducing a Captive Dependency. But unfortunately, there is no way for us to tell, as your code doesn't show the problem. Try creating a Minimal, Complete, and Verifiable Example.
– Steven
Jan 1 at 20:43
1
If you are using your DbContext as singleton and if other apllications or instances of your application also do updates on your database then this is an expected result. This is a known issue of EntityFramework. When you get an entity, it caches it and when you later read the entity from your DbContext, it provides the same object from cache. It has nothing to do with unity. Your solution works but I recommend you to google about "EntityFramework caching"
– NthDeveloper
Jan 1 at 21:18
Off-topic: why don't you inject
packingOrderService
?– Gert Arnold
Jan 1 at 21:54