How to print the student record data (a list of values) from a list of records nicely formatted?
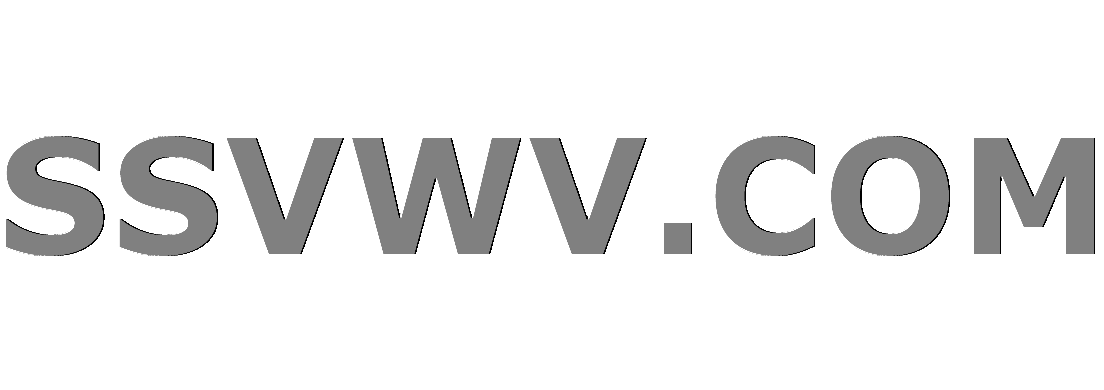
Multi tool use
I need help with my homework.
The requirements are:
The source code shall be written by Python3
The code shall contain the following:
if-else
statement
if-elif-else
statement
while
statement
for
statement
list
My code:
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number, Stud_Course, Year_Level, Stud_Name,
Address, Birthday, Father_Name, Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
for i in Stud_list:
print(i)
The output when displaying the list looks like:
[123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
[222222, 'Course', 2, 'Name2', 'Here2', Birth, 'HIM', 'HER']
But the output needs to look like this:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
And continues until it prints the entire student records.
How do I print it like that?
python python-3.x
add a comment |
I need help with my homework.
The requirements are:
The source code shall be written by Python3
The code shall contain the following:
if-else
statement
if-elif-else
statement
while
statement
for
statement
list
My code:
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number, Stud_Course, Year_Level, Stud_Name,
Address, Birthday, Father_Name, Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
for i in Stud_list:
print(i)
The output when displaying the list looks like:
[123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
[222222, 'Course', 2, 'Name2', 'Here2', Birth, 'HIM', 'HER']
But the output needs to look like this:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
And continues until it prints the entire student records.
How do I print it like that?
python python-3.x
2
good job getting to your output. Now, just manually add the string in front, at the end of the day, there is nothing special going on. For example:print("the number is " + str(1))
and so on.
– Paritosh Singh
Jan 1 at 13:48
The source code shall be written by Python3
Ouch.
– user5173426
Jan 1 at 14:10
add a comment |
I need help with my homework.
The requirements are:
The source code shall be written by Python3
The code shall contain the following:
if-else
statement
if-elif-else
statement
while
statement
for
statement
list
My code:
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number, Stud_Course, Year_Level, Stud_Name,
Address, Birthday, Father_Name, Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
for i in Stud_list:
print(i)
The output when displaying the list looks like:
[123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
[222222, 'Course', 2, 'Name2', 'Here2', Birth, 'HIM', 'HER']
But the output needs to look like this:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
And continues until it prints the entire student records.
How do I print it like that?
python python-3.x
I need help with my homework.
The requirements are:
The source code shall be written by Python3
The code shall contain the following:
if-else
statement
if-elif-else
statement
while
statement
for
statement
list
My code:
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number, Stud_Course, Year_Level, Stud_Name,
Address, Birthday, Father_Name, Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
for i in Stud_list:
print(i)
The output when displaying the list looks like:
[123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
[222222, 'Course', 2, 'Name2', 'Here2', Birth, 'HIM', 'HER']
But the output needs to look like this:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
And continues until it prints the entire student records.
How do I print it like that?
python python-3.x
python python-3.x
edited Jan 1 at 14:14


Patrick Artner
24.7k62443
24.7k62443
asked Jan 1 at 13:45
Wink101Wink101
182
182
2
good job getting to your output. Now, just manually add the string in front, at the end of the day, there is nothing special going on. For example:print("the number is " + str(1))
and so on.
– Paritosh Singh
Jan 1 at 13:48
The source code shall be written by Python3
Ouch.
– user5173426
Jan 1 at 14:10
add a comment |
2
good job getting to your output. Now, just manually add the string in front, at the end of the day, there is nothing special going on. For example:print("the number is " + str(1))
and so on.
– Paritosh Singh
Jan 1 at 13:48
The source code shall be written by Python3
Ouch.
– user5173426
Jan 1 at 14:10
2
2
good job getting to your output. Now, just manually add the string in front, at the end of the day, there is nothing special going on. For example:
print("the number is " + str(1))
and so on.– Paritosh Singh
Jan 1 at 13:48
good job getting to your output. Now, just manually add the string in front, at the end of the day, there is nothing special going on. For example:
print("the number is " + str(1))
and so on.– Paritosh Singh
Jan 1 at 13:48
The source code shall be written by Python3
Ouch.– user5173426
Jan 1 at 14:10
The source code shall be written by Python3
Ouch.– user5173426
Jan 1 at 14:10
add a comment |
5 Answers
5
active
oldest
votes
Read up on string formating - python3.6 onwards supports f-strings - before that you can use str.format():
var1 = "22"
var2 = "2*11"
print(f"{var1} = {var2}")
before ypthon 3.6 str.format()
is used to format strings, you can solve your output f.e. like this:
Stud_list = [[123456, 'Course', 1, 'Name', 'Here', "Birth", 'HIM', 'HER'],
[222222, 'Course', 2, 'Name2', 'Here2', "Birth", 'HIM', 'HER']]
for stud in Stud_list:
print(f"Student Number: {stud[0]}")
print(f"Student Course: {stud[1]}")
print(f"Year Level: {stud[2]}")
print(f"Student Name: {stud[3]}")
print(f"Address: {stud[4]}")
print(f"Birthdate: {stud[5]}")
print(f"Father's Name: {stud[6]}")
print(f"Mother's Name: {stud[7]}")
Output:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Doku:
https://docs.python.org/3/library/string.html#custom-string-formatting- str.format()
- f-strings
add a comment |
You can use f formatted string or .format
l = [123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
print("""Student Number: {}n
Student Course: {}n
Year Level: {}n
Student Name: {}n
Address: {}n
Birthdate: {}n
Father's Name: {}n
Mother's Name: {}n""".format(l[0], l[1], l[2], l[3], l[4], l[5], l[6], l[7]))
add a comment |
Your code needs to be modified like this:https://onlinegdb.com/BkLmYxFZN
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
k=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number,Stud_Course,Year_Level,Stud_Name,Address,Birthday,Father_Name,Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
if main_choice==2:
if not Stud_list:
print("List is empty")
else:
for i in Stud_list :
print("""
Student Number: {}
Student Course: {}
Year Level: {}
Student Name: {}
Address: {}
Birthdate: {}
Father's Name: {}
Mother's Name: {}""".format(i[0], i[1], i[2], i[3], i[4], i[5], i[6], i[7]))
add a comment |
Try this!
print('''Student Number: {}
nStudent Course: {}
nYear Level: {}
nStudent Name: {}
nAddress: {}
nBirthdate: {}
nFather's Name: {}
nMother's Name: {}'''.format(*i))
add a comment |
Stud_list you created is a list of list where you have stored all your data.
you can use multiple for loops or the index of the sublist.
You can use below code to print the desired output.
for i in Stud_list:
print("Student Number:",i[0])
print("Student Course:", i [1])
print("Year Level:",i[2])
print("Student Name:",i[3])
print("Address: ",i[4])
print("Birthdate: ",i[5])
print("Father's Name: ",i[6])
print("Mother's Name: ",i[7])
print()
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53995960%2fhow-to-print-the-student-record-data-a-list-of-values-from-a-list-of-records-n%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Read up on string formating - python3.6 onwards supports f-strings - before that you can use str.format():
var1 = "22"
var2 = "2*11"
print(f"{var1} = {var2}")
before ypthon 3.6 str.format()
is used to format strings, you can solve your output f.e. like this:
Stud_list = [[123456, 'Course', 1, 'Name', 'Here', "Birth", 'HIM', 'HER'],
[222222, 'Course', 2, 'Name2', 'Here2', "Birth", 'HIM', 'HER']]
for stud in Stud_list:
print(f"Student Number: {stud[0]}")
print(f"Student Course: {stud[1]}")
print(f"Year Level: {stud[2]}")
print(f"Student Name: {stud[3]}")
print(f"Address: {stud[4]}")
print(f"Birthdate: {stud[5]}")
print(f"Father's Name: {stud[6]}")
print(f"Mother's Name: {stud[7]}")
Output:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Doku:
https://docs.python.org/3/library/string.html#custom-string-formatting- str.format()
- f-strings
add a comment |
Read up on string formating - python3.6 onwards supports f-strings - before that you can use str.format():
var1 = "22"
var2 = "2*11"
print(f"{var1} = {var2}")
before ypthon 3.6 str.format()
is used to format strings, you can solve your output f.e. like this:
Stud_list = [[123456, 'Course', 1, 'Name', 'Here', "Birth", 'HIM', 'HER'],
[222222, 'Course', 2, 'Name2', 'Here2', "Birth", 'HIM', 'HER']]
for stud in Stud_list:
print(f"Student Number: {stud[0]}")
print(f"Student Course: {stud[1]}")
print(f"Year Level: {stud[2]}")
print(f"Student Name: {stud[3]}")
print(f"Address: {stud[4]}")
print(f"Birthdate: {stud[5]}")
print(f"Father's Name: {stud[6]}")
print(f"Mother's Name: {stud[7]}")
Output:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Doku:
https://docs.python.org/3/library/string.html#custom-string-formatting- str.format()
- f-strings
add a comment |
Read up on string formating - python3.6 onwards supports f-strings - before that you can use str.format():
var1 = "22"
var2 = "2*11"
print(f"{var1} = {var2}")
before ypthon 3.6 str.format()
is used to format strings, you can solve your output f.e. like this:
Stud_list = [[123456, 'Course', 1, 'Name', 'Here', "Birth", 'HIM', 'HER'],
[222222, 'Course', 2, 'Name2', 'Here2', "Birth", 'HIM', 'HER']]
for stud in Stud_list:
print(f"Student Number: {stud[0]}")
print(f"Student Course: {stud[1]}")
print(f"Year Level: {stud[2]}")
print(f"Student Name: {stud[3]}")
print(f"Address: {stud[4]}")
print(f"Birthdate: {stud[5]}")
print(f"Father's Name: {stud[6]}")
print(f"Mother's Name: {stud[7]}")
Output:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Doku:
https://docs.python.org/3/library/string.html#custom-string-formatting- str.format()
- f-strings
Read up on string formating - python3.6 onwards supports f-strings - before that you can use str.format():
var1 = "22"
var2 = "2*11"
print(f"{var1} = {var2}")
before ypthon 3.6 str.format()
is used to format strings, you can solve your output f.e. like this:
Stud_list = [[123456, 'Course', 1, 'Name', 'Here', "Birth", 'HIM', 'HER'],
[222222, 'Course', 2, 'Name2', 'Here2', "Birth", 'HIM', 'HER']]
for stud in Stud_list:
print(f"Student Number: {stud[0]}")
print(f"Student Course: {stud[1]}")
print(f"Year Level: {stud[2]}")
print(f"Student Name: {stud[3]}")
print(f"Address: {stud[4]}")
print(f"Birthdate: {stud[5]}")
print(f"Father's Name: {stud[6]}")
print(f"Mother's Name: {stud[7]}")
Output:
Student Number: 123456
Student Course: Course
Year Level: 1
Student Name: Name
Address: Here
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Student Number: 222222
Student Course: Course
Year Level: 2
Student Name: Name2
Address: Here2
Birthdate: Birth
Father's Name: HIM
Mother's Name: HER
Doku:
https://docs.python.org/3/library/string.html#custom-string-formatting- str.format()
- f-strings
edited Jan 1 at 14:14
answered Jan 1 at 13:56


Patrick ArtnerPatrick Artner
24.7k62443
24.7k62443
add a comment |
add a comment |
You can use f formatted string or .format
l = [123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
print("""Student Number: {}n
Student Course: {}n
Year Level: {}n
Student Name: {}n
Address: {}n
Birthdate: {}n
Father's Name: {}n
Mother's Name: {}n""".format(l[0], l[1], l[2], l[3], l[4], l[5], l[6], l[7]))
add a comment |
You can use f formatted string or .format
l = [123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
print("""Student Number: {}n
Student Course: {}n
Year Level: {}n
Student Name: {}n
Address: {}n
Birthdate: {}n
Father's Name: {}n
Mother's Name: {}n""".format(l[0], l[1], l[2], l[3], l[4], l[5], l[6], l[7]))
add a comment |
You can use f formatted string or .format
l = [123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
print("""Student Number: {}n
Student Course: {}n
Year Level: {}n
Student Name: {}n
Address: {}n
Birthdate: {}n
Father's Name: {}n
Mother's Name: {}n""".format(l[0], l[1], l[2], l[3], l[4], l[5], l[6], l[7]))
You can use f formatted string or .format
l = [123456, 'Course', 1, 'Name', 'Here', Birth, 'HIM', 'HER']
print("""Student Number: {}n
Student Course: {}n
Year Level: {}n
Student Name: {}n
Address: {}n
Birthdate: {}n
Father's Name: {}n
Mother's Name: {}n""".format(l[0], l[1], l[2], l[3], l[4], l[5], l[6], l[7]))
answered Jan 1 at 13:56
Aman RapariaAman Raparia
17518
17518
add a comment |
add a comment |
Your code needs to be modified like this:https://onlinegdb.com/BkLmYxFZN
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
k=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number,Stud_Course,Year_Level,Stud_Name,Address,Birthday,Father_Name,Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
if main_choice==2:
if not Stud_list:
print("List is empty")
else:
for i in Stud_list :
print("""
Student Number: {}
Student Course: {}
Year Level: {}
Student Name: {}
Address: {}
Birthdate: {}
Father's Name: {}
Mother's Name: {}""".format(i[0], i[1], i[2], i[3], i[4], i[5], i[6], i[7]))
add a comment |
Your code needs to be modified like this:https://onlinegdb.com/BkLmYxFZN
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
k=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number,Stud_Course,Year_Level,Stud_Name,Address,Birthday,Father_Name,Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
if main_choice==2:
if not Stud_list:
print("List is empty")
else:
for i in Stud_list :
print("""
Student Number: {}
Student Course: {}
Year Level: {}
Student Name: {}
Address: {}
Birthdate: {}
Father's Name: {}
Mother's Name: {}""".format(i[0], i[1], i[2], i[3], i[4], i[5], i[6], i[7]))
add a comment |
Your code needs to be modified like this:https://onlinegdb.com/BkLmYxFZN
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
k=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number,Stud_Course,Year_Level,Stud_Name,Address,Birthday,Father_Name,Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
if main_choice==2:
if not Stud_list:
print("List is empty")
else:
for i in Stud_list :
print("""
Student Number: {}
Student Course: {}
Year Level: {}
Student Name: {}
Address: {}
Birthdate: {}
Father's Name: {}
Mother's Name: {}""".format(i[0], i[1], i[2], i[3], i[4], i[5], i[6], i[7]))
Your code needs to be modified like this:https://onlinegdb.com/BkLmYxFZN
print(" Main Menu ")
print("[1] Input Student Records")
print("[2] Display Student Records")
main_choice=int(input("Choice: "))
Stud_list=
k=
choice1='y'
if main_choice==1:
while choice1=='y' or choice1=='Y':
Stud_number=int(input("Student Number: "))
Stud_Course=input("Student Course: ")
Year_Level=int(input("Year Level: "))
Stud_Name=input("Student Name:")
Address=input("Address: ")
Birthday=int(input("Birthdate: "))
Father_Name=input("Father's Name: ")
Mother_Name=input("Mother's Name: ")
Stud_list.append([Stud_number,Stud_Course,Year_Level,Stud_Name,Address,Birthday,Father_Name,Mother_Name])
choice1=input("Input another? [Y]/[N]: ")
if main_choice==2:
if not Stud_list:
print("List is empty")
else:
for i in Stud_list :
print("""
Student Number: {}
Student Course: {}
Year Level: {}
Student Name: {}
Address: {}
Birthdate: {}
Father's Name: {}
Mother's Name: {}""".format(i[0], i[1], i[2], i[3], i[4], i[5], i[6], i[7]))
edited Jan 1 at 14:29
answered Jan 1 at 14:16
i_thi_th
1,1601718
1,1601718
add a comment |
add a comment |
Try this!
print('''Student Number: {}
nStudent Course: {}
nYear Level: {}
nStudent Name: {}
nAddress: {}
nBirthdate: {}
nFather's Name: {}
nMother's Name: {}'''.format(*i))
add a comment |
Try this!
print('''Student Number: {}
nStudent Course: {}
nYear Level: {}
nStudent Name: {}
nAddress: {}
nBirthdate: {}
nFather's Name: {}
nMother's Name: {}'''.format(*i))
add a comment |
Try this!
print('''Student Number: {}
nStudent Course: {}
nYear Level: {}
nStudent Name: {}
nAddress: {}
nBirthdate: {}
nFather's Name: {}
nMother's Name: {}'''.format(*i))
Try this!
print('''Student Number: {}
nStudent Course: {}
nYear Level: {}
nStudent Name: {}
nAddress: {}
nBirthdate: {}
nFather's Name: {}
nMother's Name: {}'''.format(*i))
edited Jan 1 at 14:54
answered Jan 1 at 13:55


AI_LearningAI_Learning
3,57921033
3,57921033
add a comment |
add a comment |
Stud_list you created is a list of list where you have stored all your data.
you can use multiple for loops or the index of the sublist.
You can use below code to print the desired output.
for i in Stud_list:
print("Student Number:",i[0])
print("Student Course:", i [1])
print("Year Level:",i[2])
print("Student Name:",i[3])
print("Address: ",i[4])
print("Birthdate: ",i[5])
print("Father's Name: ",i[6])
print("Mother's Name: ",i[7])
print()
add a comment |
Stud_list you created is a list of list where you have stored all your data.
you can use multiple for loops or the index of the sublist.
You can use below code to print the desired output.
for i in Stud_list:
print("Student Number:",i[0])
print("Student Course:", i [1])
print("Year Level:",i[2])
print("Student Name:",i[3])
print("Address: ",i[4])
print("Birthdate: ",i[5])
print("Father's Name: ",i[6])
print("Mother's Name: ",i[7])
print()
add a comment |
Stud_list you created is a list of list where you have stored all your data.
you can use multiple for loops or the index of the sublist.
You can use below code to print the desired output.
for i in Stud_list:
print("Student Number:",i[0])
print("Student Course:", i [1])
print("Year Level:",i[2])
print("Student Name:",i[3])
print("Address: ",i[4])
print("Birthdate: ",i[5])
print("Father's Name: ",i[6])
print("Mother's Name: ",i[7])
print()
Stud_list you created is a list of list where you have stored all your data.
you can use multiple for loops or the index of the sublist.
You can use below code to print the desired output.
for i in Stud_list:
print("Student Number:",i[0])
print("Student Course:", i [1])
print("Year Level:",i[2])
print("Student Name:",i[3])
print("Address: ",i[4])
print("Birthdate: ",i[5])
print("Father's Name: ",i[6])
print("Mother's Name: ",i[7])
print()
answered Jan 1 at 14:19
Akshay SapraAkshay Sapra
196317
196317
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53995960%2fhow-to-print-the-student-record-data-a-list-of-values-from-a-list-of-records-n%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zxE8DM4YQt,pfuG
2
good job getting to your output. Now, just manually add the string in front, at the end of the day, there is nothing special going on. For example:
print("the number is " + str(1))
and so on.– Paritosh Singh
Jan 1 at 13:48
The source code shall be written by Python3
Ouch.– user5173426
Jan 1 at 14:10