How to open a new console from python script and be able to manage it
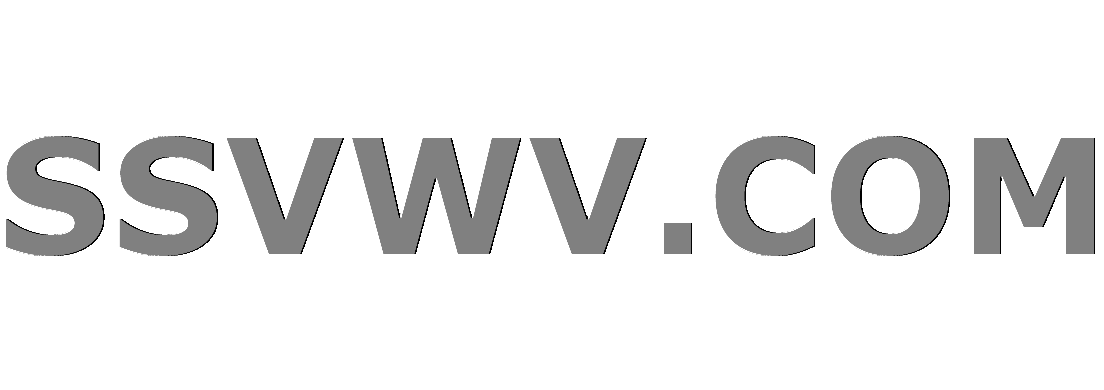
Multi tool use
I am writing a script that needs to be able to open a separate console window and read and write to it(on Windows). I want to use telnet and ssh from new consoles and the main console use as a manager
Right now i was trying thoes things:
main file
import subprocess as sp
from getpass import getpass
import telnetlib
import multiprocessing as mp
import os
def run(command):
cmd = sp.Popen(command.split(), sp.PIPE)
com, err = cmd.communicate()
print(com,err)
if __name__ == "__main__":
login = input("Podaj login: ")
password = getpass()
ip = "10.74.119.252"
command = f"python LogInConsol.py {ip} {login} {password}"
process = mp.Process(target=run, args= (command,))
process.start()
LogInConsol file
import telnetlib
import subprocess as sb
import sys
argv = sys.argv
ip = argv[1]
login = argv[2]
pw = argv[3]
# tn = telnetlib.Telnet(host=ip, port= 23)
# tn.read_until(b"login: ")
command = f"cmd.exe start /k telnet {ip}"
cmd = sb.run(command.split(),sb.PIPE)
com, err = cmd.communicate()
But the behavior is such that everything happens in main consol window(from which i start program)
UPDATE
This started to work as i wanted it to.
Main.py
from subprocess import Popen, PIPE, CREATE_NEW_CONSOLE
from getpass import getpass
import telnetlib
import multiprocessing as mp
import threading as th
def run(command):
cmd = Popen(command, PIPE, creationflags=CREATE_NEW_CONSOLE)
com, err = cmd.communicate()
print(com,err)
if __name__ == "__main__":
mp.freeze_support()
login = input("Podaj login: ")
password = getpass()
ip = "10.10.10.10"
command = f"python LogInConsol.py {ip} {login} {password}"
process = mp.Process(target=run, args= (command,))
process.start()
input("Wait ")
LogInConsol.py
import telnetlib
import subprocess as sp
import sys
argv = sys.argv
ip = argv[1]
login = argv[2]
pw = argv[3]
print(argv)
tn = telnetlib.Telnet(host=ip,port=23)
red = tn.read_until(b"username: ")
print(red)
python cmd
add a comment |
I am writing a script that needs to be able to open a separate console window and read and write to it(on Windows). I want to use telnet and ssh from new consoles and the main console use as a manager
Right now i was trying thoes things:
main file
import subprocess as sp
from getpass import getpass
import telnetlib
import multiprocessing as mp
import os
def run(command):
cmd = sp.Popen(command.split(), sp.PIPE)
com, err = cmd.communicate()
print(com,err)
if __name__ == "__main__":
login = input("Podaj login: ")
password = getpass()
ip = "10.74.119.252"
command = f"python LogInConsol.py {ip} {login} {password}"
process = mp.Process(target=run, args= (command,))
process.start()
LogInConsol file
import telnetlib
import subprocess as sb
import sys
argv = sys.argv
ip = argv[1]
login = argv[2]
pw = argv[3]
# tn = telnetlib.Telnet(host=ip, port= 23)
# tn.read_until(b"login: ")
command = f"cmd.exe start /k telnet {ip}"
cmd = sb.run(command.split(),sb.PIPE)
com, err = cmd.communicate()
But the behavior is such that everything happens in main consol window(from which i start program)
UPDATE
This started to work as i wanted it to.
Main.py
from subprocess import Popen, PIPE, CREATE_NEW_CONSOLE
from getpass import getpass
import telnetlib
import multiprocessing as mp
import threading as th
def run(command):
cmd = Popen(command, PIPE, creationflags=CREATE_NEW_CONSOLE)
com, err = cmd.communicate()
print(com,err)
if __name__ == "__main__":
mp.freeze_support()
login = input("Podaj login: ")
password = getpass()
ip = "10.10.10.10"
command = f"python LogInConsol.py {ip} {login} {password}"
process = mp.Process(target=run, args= (command,))
process.start()
input("Wait ")
LogInConsol.py
import telnetlib
import subprocess as sp
import sys
argv = sys.argv
ip = argv[1]
login = argv[2]
pw = argv[3]
print(argv)
tn = telnetlib.Telnet(host=ip,port=23)
red = tn.read_until(b"username: ")
print(red)
python cmd
add a comment |
I am writing a script that needs to be able to open a separate console window and read and write to it(on Windows). I want to use telnet and ssh from new consoles and the main console use as a manager
Right now i was trying thoes things:
main file
import subprocess as sp
from getpass import getpass
import telnetlib
import multiprocessing as mp
import os
def run(command):
cmd = sp.Popen(command.split(), sp.PIPE)
com, err = cmd.communicate()
print(com,err)
if __name__ == "__main__":
login = input("Podaj login: ")
password = getpass()
ip = "10.74.119.252"
command = f"python LogInConsol.py {ip} {login} {password}"
process = mp.Process(target=run, args= (command,))
process.start()
LogInConsol file
import telnetlib
import subprocess as sb
import sys
argv = sys.argv
ip = argv[1]
login = argv[2]
pw = argv[3]
# tn = telnetlib.Telnet(host=ip, port= 23)
# tn.read_until(b"login: ")
command = f"cmd.exe start /k telnet {ip}"
cmd = sb.run(command.split(),sb.PIPE)
com, err = cmd.communicate()
But the behavior is such that everything happens in main consol window(from which i start program)
UPDATE
This started to work as i wanted it to.
Main.py
from subprocess import Popen, PIPE, CREATE_NEW_CONSOLE
from getpass import getpass
import telnetlib
import multiprocessing as mp
import threading as th
def run(command):
cmd = Popen(command, PIPE, creationflags=CREATE_NEW_CONSOLE)
com, err = cmd.communicate()
print(com,err)
if __name__ == "__main__":
mp.freeze_support()
login = input("Podaj login: ")
password = getpass()
ip = "10.10.10.10"
command = f"python LogInConsol.py {ip} {login} {password}"
process = mp.Process(target=run, args= (command,))
process.start()
input("Wait ")
LogInConsol.py
import telnetlib
import subprocess as sp
import sys
argv = sys.argv
ip = argv[1]
login = argv[2]
pw = argv[3]
print(argv)
tn = telnetlib.Telnet(host=ip,port=23)
red = tn.read_until(b"username: ")
print(red)
python cmd
I am writing a script that needs to be able to open a separate console window and read and write to it(on Windows). I want to use telnet and ssh from new consoles and the main console use as a manager
Right now i was trying thoes things:
main file
import subprocess as sp
from getpass import getpass
import telnetlib
import multiprocessing as mp
import os
def run(command):
cmd = sp.Popen(command.split(), sp.PIPE)
com, err = cmd.communicate()
print(com,err)
if __name__ == "__main__":
login = input("Podaj login: ")
password = getpass()
ip = "10.74.119.252"
command = f"python LogInConsol.py {ip} {login} {password}"
process = mp.Process(target=run, args= (command,))
process.start()
LogInConsol file
import telnetlib
import subprocess as sb
import sys
argv = sys.argv
ip = argv[1]
login = argv[2]
pw = argv[3]
# tn = telnetlib.Telnet(host=ip, port= 23)
# tn.read_until(b"login: ")
command = f"cmd.exe start /k telnet {ip}"
cmd = sb.run(command.split(),sb.PIPE)
com, err = cmd.communicate()
But the behavior is such that everything happens in main consol window(from which i start program)
UPDATE
This started to work as i wanted it to.
Main.py
from subprocess import Popen, PIPE, CREATE_NEW_CONSOLE
from getpass import getpass
import telnetlib
import multiprocessing as mp
import threading as th
def run(command):
cmd = Popen(command, PIPE, creationflags=CREATE_NEW_CONSOLE)
com, err = cmd.communicate()
print(com,err)
if __name__ == "__main__":
mp.freeze_support()
login = input("Podaj login: ")
password = getpass()
ip = "10.10.10.10"
command = f"python LogInConsol.py {ip} {login} {password}"
process = mp.Process(target=run, args= (command,))
process.start()
input("Wait ")
LogInConsol.py
import telnetlib
import subprocess as sp
import sys
argv = sys.argv
ip = argv[1]
login = argv[2]
pw = argv[3]
print(argv)
tn = telnetlib.Telnet(host=ip,port=23)
red = tn.read_until(b"username: ")
print(red)
python cmd
python cmd
edited Jan 3 at 10:43
Adrian Baczyński
asked Dec 31 '18 at 12:44
Adrian BaczyńskiAdrian Baczyński
36111
36111
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Could try wshshell:
1. pip install pywin32-221-cp36-cp36m-win_amd64.whl
2. python.exe pywin32_postinstall.py -install (DOS command line)
in script:
import win32com.client
WshShell = win32com.client.Dispatch("WScript.Shell")
WshShell.run("cmd")
I am testing it and i may work. Can you tell me how to get to input and output of new console ?
– Adrian Baczyński
Jan 2 at 9:31
1
take a look at the answer to this question : stackoverflow.com/questions/6732543/… im not too familiar with the library myself so the shell.echo seems to be the answer
– Brandon Bailey
Jan 2 at 11:12
It's hard for me to wrap my mind around this api, the example is not very accurate. Still problem is not solved.
– Adrian Baczyński
Jan 2 at 13:52
try using subprocess.communicate. more here: stackoverflow.com/questions/25815078/… also subprocess.check_output will give the output of the subprocess call. You must call it correctly, in your example script you are not. try this documentation : docs.python.org/2/library/subprocess.html
– Brandon Bailey
Jan 3 at 12:33
add a comment |
Could this be a case of the XY problem? You need to connect via telnet or ssh, but do you really need that console in between?
You can make telnet connections in python using telnetlib and ssh ones with paramiko.
What i want is to start connection from main console but manage the rest from the new one
– Adrian Baczyński
Jan 2 at 16:22
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987673%2fhow-to-open-a-new-console-from-python-script-and-be-able-to-manage-it%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Could try wshshell:
1. pip install pywin32-221-cp36-cp36m-win_amd64.whl
2. python.exe pywin32_postinstall.py -install (DOS command line)
in script:
import win32com.client
WshShell = win32com.client.Dispatch("WScript.Shell")
WshShell.run("cmd")
I am testing it and i may work. Can you tell me how to get to input and output of new console ?
– Adrian Baczyński
Jan 2 at 9:31
1
take a look at the answer to this question : stackoverflow.com/questions/6732543/… im not too familiar with the library myself so the shell.echo seems to be the answer
– Brandon Bailey
Jan 2 at 11:12
It's hard for me to wrap my mind around this api, the example is not very accurate. Still problem is not solved.
– Adrian Baczyński
Jan 2 at 13:52
try using subprocess.communicate. more here: stackoverflow.com/questions/25815078/… also subprocess.check_output will give the output of the subprocess call. You must call it correctly, in your example script you are not. try this documentation : docs.python.org/2/library/subprocess.html
– Brandon Bailey
Jan 3 at 12:33
add a comment |
Could try wshshell:
1. pip install pywin32-221-cp36-cp36m-win_amd64.whl
2. python.exe pywin32_postinstall.py -install (DOS command line)
in script:
import win32com.client
WshShell = win32com.client.Dispatch("WScript.Shell")
WshShell.run("cmd")
I am testing it and i may work. Can you tell me how to get to input and output of new console ?
– Adrian Baczyński
Jan 2 at 9:31
1
take a look at the answer to this question : stackoverflow.com/questions/6732543/… im not too familiar with the library myself so the shell.echo seems to be the answer
– Brandon Bailey
Jan 2 at 11:12
It's hard for me to wrap my mind around this api, the example is not very accurate. Still problem is not solved.
– Adrian Baczyński
Jan 2 at 13:52
try using subprocess.communicate. more here: stackoverflow.com/questions/25815078/… also subprocess.check_output will give the output of the subprocess call. You must call it correctly, in your example script you are not. try this documentation : docs.python.org/2/library/subprocess.html
– Brandon Bailey
Jan 3 at 12:33
add a comment |
Could try wshshell:
1. pip install pywin32-221-cp36-cp36m-win_amd64.whl
2. python.exe pywin32_postinstall.py -install (DOS command line)
in script:
import win32com.client
WshShell = win32com.client.Dispatch("WScript.Shell")
WshShell.run("cmd")
Could try wshshell:
1. pip install pywin32-221-cp36-cp36m-win_amd64.whl
2. python.exe pywin32_postinstall.py -install (DOS command line)
in script:
import win32com.client
WshShell = win32com.client.Dispatch("WScript.Shell")
WshShell.run("cmd")
answered Dec 31 '18 at 14:02


Brandon BaileyBrandon Bailey
1477
1477
I am testing it and i may work. Can you tell me how to get to input and output of new console ?
– Adrian Baczyński
Jan 2 at 9:31
1
take a look at the answer to this question : stackoverflow.com/questions/6732543/… im not too familiar with the library myself so the shell.echo seems to be the answer
– Brandon Bailey
Jan 2 at 11:12
It's hard for me to wrap my mind around this api, the example is not very accurate. Still problem is not solved.
– Adrian Baczyński
Jan 2 at 13:52
try using subprocess.communicate. more here: stackoverflow.com/questions/25815078/… also subprocess.check_output will give the output of the subprocess call. You must call it correctly, in your example script you are not. try this documentation : docs.python.org/2/library/subprocess.html
– Brandon Bailey
Jan 3 at 12:33
add a comment |
I am testing it and i may work. Can you tell me how to get to input and output of new console ?
– Adrian Baczyński
Jan 2 at 9:31
1
take a look at the answer to this question : stackoverflow.com/questions/6732543/… im not too familiar with the library myself so the shell.echo seems to be the answer
– Brandon Bailey
Jan 2 at 11:12
It's hard for me to wrap my mind around this api, the example is not very accurate. Still problem is not solved.
– Adrian Baczyński
Jan 2 at 13:52
try using subprocess.communicate. more here: stackoverflow.com/questions/25815078/… also subprocess.check_output will give the output of the subprocess call. You must call it correctly, in your example script you are not. try this documentation : docs.python.org/2/library/subprocess.html
– Brandon Bailey
Jan 3 at 12:33
I am testing it and i may work. Can you tell me how to get to input and output of new console ?
– Adrian Baczyński
Jan 2 at 9:31
I am testing it and i may work. Can you tell me how to get to input and output of new console ?
– Adrian Baczyński
Jan 2 at 9:31
1
1
take a look at the answer to this question : stackoverflow.com/questions/6732543/… im not too familiar with the library myself so the shell.echo seems to be the answer
– Brandon Bailey
Jan 2 at 11:12
take a look at the answer to this question : stackoverflow.com/questions/6732543/… im not too familiar with the library myself so the shell.echo seems to be the answer
– Brandon Bailey
Jan 2 at 11:12
It's hard for me to wrap my mind around this api, the example is not very accurate. Still problem is not solved.
– Adrian Baczyński
Jan 2 at 13:52
It's hard for me to wrap my mind around this api, the example is not very accurate. Still problem is not solved.
– Adrian Baczyński
Jan 2 at 13:52
try using subprocess.communicate. more here: stackoverflow.com/questions/25815078/… also subprocess.check_output will give the output of the subprocess call. You must call it correctly, in your example script you are not. try this documentation : docs.python.org/2/library/subprocess.html
– Brandon Bailey
Jan 3 at 12:33
try using subprocess.communicate. more here: stackoverflow.com/questions/25815078/… also subprocess.check_output will give the output of the subprocess call. You must call it correctly, in your example script you are not. try this documentation : docs.python.org/2/library/subprocess.html
– Brandon Bailey
Jan 3 at 12:33
add a comment |
Could this be a case of the XY problem? You need to connect via telnet or ssh, but do you really need that console in between?
You can make telnet connections in python using telnetlib and ssh ones with paramiko.
What i want is to start connection from main console but manage the rest from the new one
– Adrian Baczyński
Jan 2 at 16:22
add a comment |
Could this be a case of the XY problem? You need to connect via telnet or ssh, but do you really need that console in between?
You can make telnet connections in python using telnetlib and ssh ones with paramiko.
What i want is to start connection from main console but manage the rest from the new one
– Adrian Baczyński
Jan 2 at 16:22
add a comment |
Could this be a case of the XY problem? You need to connect via telnet or ssh, but do you really need that console in between?
You can make telnet connections in python using telnetlib and ssh ones with paramiko.
Could this be a case of the XY problem? You need to connect via telnet or ssh, but do you really need that console in between?
You can make telnet connections in python using telnetlib and ssh ones with paramiko.
answered Jan 2 at 16:20
GaloisGirlGaloisGirl
313
313
What i want is to start connection from main console but manage the rest from the new one
– Adrian Baczyński
Jan 2 at 16:22
add a comment |
What i want is to start connection from main console but manage the rest from the new one
– Adrian Baczyński
Jan 2 at 16:22
What i want is to start connection from main console but manage the rest from the new one
– Adrian Baczyński
Jan 2 at 16:22
What i want is to start connection from main console but manage the rest from the new one
– Adrian Baczyński
Jan 2 at 16:22
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987673%2fhow-to-open-a-new-console-from-python-script-and-be-able-to-manage-it%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Dk Ib dFI,9U74Hxgizycy7W7AhDgUhein28b