Create a new json string from jq output elements
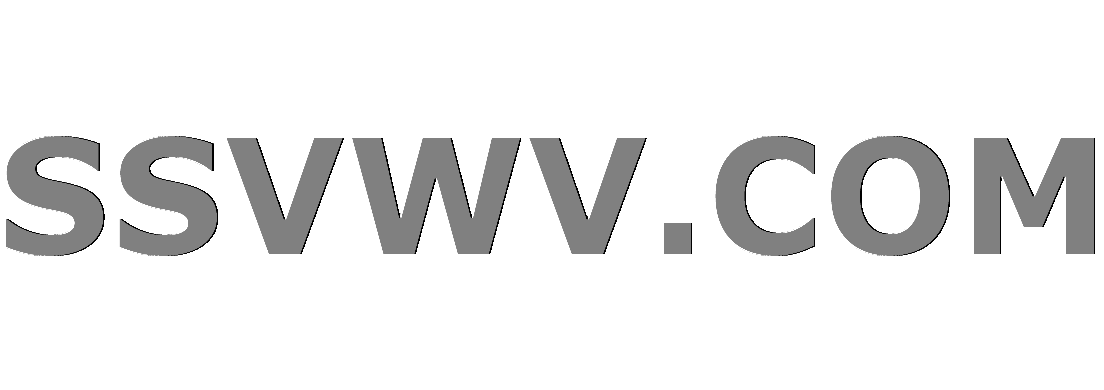
Multi tool use
My jq command returns objects in brackets but without comma separators. But I would like to create a new json string from it.
This call finds all elements of arr
that have a FooItem
in them and then returns texts
from the nested array at index 3
:
jq '.arr | select(index("FooItem")) | .[3].texts'
on this json (The original has more elements ):
{
"arr": [
[
"create",
"w199",
"FooItem",
{
"index": 0,
"texts": [
"aBarfoo",
"avalue"
]
}
],
[
"create",
"w200",
"NoItem",
{
"index": 1,
"val": 5,
"hearts": 5
}
],
[
"create",
"w200",
"FooItem",
{
"index": 1,
"texts": [
"mybarfoo",
"bValue"
]
}
]
]
}
returns this output:
[
"aBarfoo",
"avalue"
]
[
"mybarfoo",
"bValue"
]
But I'd like to create a new json from these objects that looks like this:
{
"arr": [
[
"aBarfoo",
"avalue"
],
[
"mybarfoo",
"bValue"
]
]
}
Can jq do this?
EDIT
One more addition: Considering that texts also has strings of zero length, how would you delete those/not have them in the result?
"texts": ["",
"mybarfoo",
"bValue",
""
]
json jq
add a comment |
My jq command returns objects in brackets but without comma separators. But I would like to create a new json string from it.
This call finds all elements of arr
that have a FooItem
in them and then returns texts
from the nested array at index 3
:
jq '.arr | select(index("FooItem")) | .[3].texts'
on this json (The original has more elements ):
{
"arr": [
[
"create",
"w199",
"FooItem",
{
"index": 0,
"texts": [
"aBarfoo",
"avalue"
]
}
],
[
"create",
"w200",
"NoItem",
{
"index": 1,
"val": 5,
"hearts": 5
}
],
[
"create",
"w200",
"FooItem",
{
"index": 1,
"texts": [
"mybarfoo",
"bValue"
]
}
]
]
}
returns this output:
[
"aBarfoo",
"avalue"
]
[
"mybarfoo",
"bValue"
]
But I'd like to create a new json from these objects that looks like this:
{
"arr": [
[
"aBarfoo",
"avalue"
],
[
"mybarfoo",
"bValue"
]
]
}
Can jq do this?
EDIT
One more addition: Considering that texts also has strings of zero length, how would you delete those/not have them in the result?
"texts": ["",
"mybarfoo",
"bValue",
""
]
json jq
add a comment |
My jq command returns objects in brackets but without comma separators. But I would like to create a new json string from it.
This call finds all elements of arr
that have a FooItem
in them and then returns texts
from the nested array at index 3
:
jq '.arr | select(index("FooItem")) | .[3].texts'
on this json (The original has more elements ):
{
"arr": [
[
"create",
"w199",
"FooItem",
{
"index": 0,
"texts": [
"aBarfoo",
"avalue"
]
}
],
[
"create",
"w200",
"NoItem",
{
"index": 1,
"val": 5,
"hearts": 5
}
],
[
"create",
"w200",
"FooItem",
{
"index": 1,
"texts": [
"mybarfoo",
"bValue"
]
}
]
]
}
returns this output:
[
"aBarfoo",
"avalue"
]
[
"mybarfoo",
"bValue"
]
But I'd like to create a new json from these objects that looks like this:
{
"arr": [
[
"aBarfoo",
"avalue"
],
[
"mybarfoo",
"bValue"
]
]
}
Can jq do this?
EDIT
One more addition: Considering that texts also has strings of zero length, how would you delete those/not have them in the result?
"texts": ["",
"mybarfoo",
"bValue",
""
]
json jq
My jq command returns objects in brackets but without comma separators. But I would like to create a new json string from it.
This call finds all elements of arr
that have a FooItem
in them and then returns texts
from the nested array at index 3
:
jq '.arr | select(index("FooItem")) | .[3].texts'
on this json (The original has more elements ):
{
"arr": [
[
"create",
"w199",
"FooItem",
{
"index": 0,
"texts": [
"aBarfoo",
"avalue"
]
}
],
[
"create",
"w200",
"NoItem",
{
"index": 1,
"val": 5,
"hearts": 5
}
],
[
"create",
"w200",
"FooItem",
{
"index": 1,
"texts": [
"mybarfoo",
"bValue"
]
}
]
]
}
returns this output:
[
"aBarfoo",
"avalue"
]
[
"mybarfoo",
"bValue"
]
But I'd like to create a new json from these objects that looks like this:
{
"arr": [
[
"aBarfoo",
"avalue"
],
[
"mybarfoo",
"bValue"
]
]
}
Can jq do this?
EDIT
One more addition: Considering that texts also has strings of zero length, how would you delete those/not have them in the result?
"texts": ["",
"mybarfoo",
"bValue",
""
]
json jq
json jq
edited Jan 1 at 11:09
tzippy
asked Dec 31 '18 at 12:48
tzippytzippy
2,6691656105
2,6691656105
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can always embed a stream of (zero or more) JSON entities within some other JSON structure by decorating the stream, that is, in the present case, by wrapping the STREAM as follows:
{ arr: [ STREAM ] }
In the present case, however, we can also take the view that we are simply editing the original document, and accordingly use a variation of the map(select(...))
idiom:
.arr |= map( select(index("FooItem")) | .[3].texts)
This latter approach ensures that the context of the "arr" key is preserved.
Addendum
To filter out the empty strings, simply add another map(select(...))
:
.arr |= map( select(index("FooItem"))
| .[3].texts | map(select(length>0)))
Thank you! it works! However I will definitely have to wrap my head around it to understand it.
– tzippy
Dec 31 '18 at 17:24
Is there a way to rename the arrayarr
in the output ?
– tzippy
Dec 31 '18 at 19:39
Of course. Using the first approach, you just specify the alternative name. Using the second approach, you could for example write:.newname = (.arr | map(...)) | del(.arr)
– peak
Dec 31 '18 at 20:09
Thank you @peak. I had one more final addition, but did not want to ask it in the comments, so I made an edit to the question. Maybe you can help me out one more time.
– tzippy
Jan 1 at 11:10
Thanks for the addendum!!
– tzippy
Jan 2 at 12:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987713%2fcreate-a-new-json-string-from-jq-output-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can always embed a stream of (zero or more) JSON entities within some other JSON structure by decorating the stream, that is, in the present case, by wrapping the STREAM as follows:
{ arr: [ STREAM ] }
In the present case, however, we can also take the view that we are simply editing the original document, and accordingly use a variation of the map(select(...))
idiom:
.arr |= map( select(index("FooItem")) | .[3].texts)
This latter approach ensures that the context of the "arr" key is preserved.
Addendum
To filter out the empty strings, simply add another map(select(...))
:
.arr |= map( select(index("FooItem"))
| .[3].texts | map(select(length>0)))
Thank you! it works! However I will definitely have to wrap my head around it to understand it.
– tzippy
Dec 31 '18 at 17:24
Is there a way to rename the arrayarr
in the output ?
– tzippy
Dec 31 '18 at 19:39
Of course. Using the first approach, you just specify the alternative name. Using the second approach, you could for example write:.newname = (.arr | map(...)) | del(.arr)
– peak
Dec 31 '18 at 20:09
Thank you @peak. I had one more final addition, but did not want to ask it in the comments, so I made an edit to the question. Maybe you can help me out one more time.
– tzippy
Jan 1 at 11:10
Thanks for the addendum!!
– tzippy
Jan 2 at 12:42
add a comment |
You can always embed a stream of (zero or more) JSON entities within some other JSON structure by decorating the stream, that is, in the present case, by wrapping the STREAM as follows:
{ arr: [ STREAM ] }
In the present case, however, we can also take the view that we are simply editing the original document, and accordingly use a variation of the map(select(...))
idiom:
.arr |= map( select(index("FooItem")) | .[3].texts)
This latter approach ensures that the context of the "arr" key is preserved.
Addendum
To filter out the empty strings, simply add another map(select(...))
:
.arr |= map( select(index("FooItem"))
| .[3].texts | map(select(length>0)))
Thank you! it works! However I will definitely have to wrap my head around it to understand it.
– tzippy
Dec 31 '18 at 17:24
Is there a way to rename the arrayarr
in the output ?
– tzippy
Dec 31 '18 at 19:39
Of course. Using the first approach, you just specify the alternative name. Using the second approach, you could for example write:.newname = (.arr | map(...)) | del(.arr)
– peak
Dec 31 '18 at 20:09
Thank you @peak. I had one more final addition, but did not want to ask it in the comments, so I made an edit to the question. Maybe you can help me out one more time.
– tzippy
Jan 1 at 11:10
Thanks for the addendum!!
– tzippy
Jan 2 at 12:42
add a comment |
You can always embed a stream of (zero or more) JSON entities within some other JSON structure by decorating the stream, that is, in the present case, by wrapping the STREAM as follows:
{ arr: [ STREAM ] }
In the present case, however, we can also take the view that we are simply editing the original document, and accordingly use a variation of the map(select(...))
idiom:
.arr |= map( select(index("FooItem")) | .[3].texts)
This latter approach ensures that the context of the "arr" key is preserved.
Addendum
To filter out the empty strings, simply add another map(select(...))
:
.arr |= map( select(index("FooItem"))
| .[3].texts | map(select(length>0)))
You can always embed a stream of (zero or more) JSON entities within some other JSON structure by decorating the stream, that is, in the present case, by wrapping the STREAM as follows:
{ arr: [ STREAM ] }
In the present case, however, we can also take the view that we are simply editing the original document, and accordingly use a variation of the map(select(...))
idiom:
.arr |= map( select(index("FooItem")) | .[3].texts)
This latter approach ensures that the context of the "arr" key is preserved.
Addendum
To filter out the empty strings, simply add another map(select(...))
:
.arr |= map( select(index("FooItem"))
| .[3].texts | map(select(length>0)))
edited Jan 1 at 14:23
answered Dec 31 '18 at 14:58
peakpeak
32.2k93959
32.2k93959
Thank you! it works! However I will definitely have to wrap my head around it to understand it.
– tzippy
Dec 31 '18 at 17:24
Is there a way to rename the arrayarr
in the output ?
– tzippy
Dec 31 '18 at 19:39
Of course. Using the first approach, you just specify the alternative name. Using the second approach, you could for example write:.newname = (.arr | map(...)) | del(.arr)
– peak
Dec 31 '18 at 20:09
Thank you @peak. I had one more final addition, but did not want to ask it in the comments, so I made an edit to the question. Maybe you can help me out one more time.
– tzippy
Jan 1 at 11:10
Thanks for the addendum!!
– tzippy
Jan 2 at 12:42
add a comment |
Thank you! it works! However I will definitely have to wrap my head around it to understand it.
– tzippy
Dec 31 '18 at 17:24
Is there a way to rename the arrayarr
in the output ?
– tzippy
Dec 31 '18 at 19:39
Of course. Using the first approach, you just specify the alternative name. Using the second approach, you could for example write:.newname = (.arr | map(...)) | del(.arr)
– peak
Dec 31 '18 at 20:09
Thank you @peak. I had one more final addition, but did not want to ask it in the comments, so I made an edit to the question. Maybe you can help me out one more time.
– tzippy
Jan 1 at 11:10
Thanks for the addendum!!
– tzippy
Jan 2 at 12:42
Thank you! it works! However I will definitely have to wrap my head around it to understand it.
– tzippy
Dec 31 '18 at 17:24
Thank you! it works! However I will definitely have to wrap my head around it to understand it.
– tzippy
Dec 31 '18 at 17:24
Is there a way to rename the array
arr
in the output ?– tzippy
Dec 31 '18 at 19:39
Is there a way to rename the array
arr
in the output ?– tzippy
Dec 31 '18 at 19:39
Of course. Using the first approach, you just specify the alternative name. Using the second approach, you could for example write:
.newname = (.arr | map(...)) | del(.arr)
– peak
Dec 31 '18 at 20:09
Of course. Using the first approach, you just specify the alternative name. Using the second approach, you could for example write:
.newname = (.arr | map(...)) | del(.arr)
– peak
Dec 31 '18 at 20:09
Thank you @peak. I had one more final addition, but did not want to ask it in the comments, so I made an edit to the question. Maybe you can help me out one more time.
– tzippy
Jan 1 at 11:10
Thank you @peak. I had one more final addition, but did not want to ask it in the comments, so I made an edit to the question. Maybe you can help me out one more time.
– tzippy
Jan 1 at 11:10
Thanks for the addendum!!
– tzippy
Jan 2 at 12:42
Thanks for the addendum!!
– tzippy
Jan 2 at 12:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53987713%2fcreate-a-new-json-string-from-jq-output-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uGxREH yD,n Tl,BIDYhWIxGuyYmu,xthuWbNh9 294fkGpyG,NiY1SFmbSEuNcwRh