Overriding explicit interface implementations?
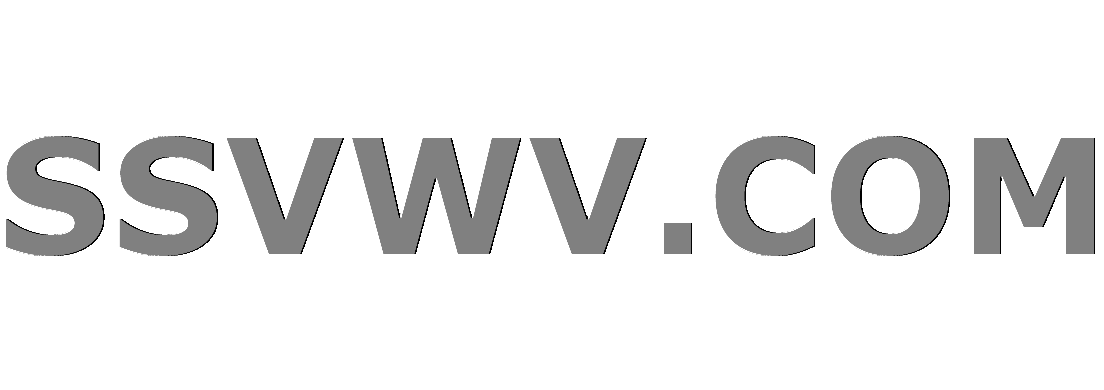
Multi tool use
What is the proper way to override explicit implementations of an interface in a child class?
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
// causes compile time errors
override string ITest.Speak()
{
// Note: I'd also like to be able to call the base implementation
return "Mooo" + base.Speak();
}
}
The above is a best guess for the syntax, but obviously it's wrong. It causes the following compile time errors:
error CS0621:
`ChildTest.ITest.Speak()': virtual or abstract members cannot be
private
error CS0540:
ChildTest.ITest.Speak()': containing type does not implement
ITest'
interface
error CS0106:
The modifier `override' is not valid for this item
I can actually get this to work without using explicit interfaces so it's not actually blocking me but I would really like know, for my own curiosity, what is the correct syntax if wanted to do this with explicit interfaces?
c# oop syntax interface
add a comment |
What is the proper way to override explicit implementations of an interface in a child class?
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
// causes compile time errors
override string ITest.Speak()
{
// Note: I'd also like to be able to call the base implementation
return "Mooo" + base.Speak();
}
}
The above is a best guess for the syntax, but obviously it's wrong. It causes the following compile time errors:
error CS0621:
`ChildTest.ITest.Speak()': virtual or abstract members cannot be
private
error CS0540:
ChildTest.ITest.Speak()': containing type does not implement
ITest'
interface
error CS0106:
The modifier `override' is not valid for this item
I can actually get this to work without using explicit interfaces so it's not actually blocking me but I would really like know, for my own curiosity, what is the correct syntax if wanted to do this with explicit interfaces?
c# oop syntax interface
2
What's wrong with implicit interface implementation? What are you trying to accomplish?
– chomba
May 18 '16 at 22:23
@chomba, The use of explicit interfaces has been a style choice in my codebase. I just think it's a lot cleaner to look at a class that implements 4 or 5 different interfaces and know what methods are associated with what interface. It's been fine for the majority of my use cases, this is the first time I've run into a limitation with them.
– James McMahon
May 18 '16 at 22:27
@JamesMcMahon As far as I know the main use case for explicit is multiple interfaces with members with identical signatures. I very rarely see them used and if I did I'd feel like whoever wrote the code didn't write C# very often.
– Casey
May 18 '16 at 22:28
Yeah that gets into programmer preference, there is a bunch of answers at stackoverflow.com/questions/143405 that go into the pros / cons of each approach.
– James McMahon
May 18 '16 at 22:32
@JamesMcMahon Yes, I agree, but I feel like this is one of those things that's mostly agreed upon by people who use the language often. It's the same way I'd feel weird about seeing C# code that saidvar some_object = new my_class()
; there's nothing wrong with snake case but it feels out of place in C#.
– Casey
May 18 '16 at 23:50
add a comment |
What is the proper way to override explicit implementations of an interface in a child class?
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
// causes compile time errors
override string ITest.Speak()
{
// Note: I'd also like to be able to call the base implementation
return "Mooo" + base.Speak();
}
}
The above is a best guess for the syntax, but obviously it's wrong. It causes the following compile time errors:
error CS0621:
`ChildTest.ITest.Speak()': virtual or abstract members cannot be
private
error CS0540:
ChildTest.ITest.Speak()': containing type does not implement
ITest'
interface
error CS0106:
The modifier `override' is not valid for this item
I can actually get this to work without using explicit interfaces so it's not actually blocking me but I would really like know, for my own curiosity, what is the correct syntax if wanted to do this with explicit interfaces?
c# oop syntax interface
What is the proper way to override explicit implementations of an interface in a child class?
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
// causes compile time errors
override string ITest.Speak()
{
// Note: I'd also like to be able to call the base implementation
return "Mooo" + base.Speak();
}
}
The above is a best guess for the syntax, but obviously it's wrong. It causes the following compile time errors:
error CS0621:
`ChildTest.ITest.Speak()': virtual or abstract members cannot be
private
error CS0540:
ChildTest.ITest.Speak()': containing type does not implement
ITest'
interface
error CS0106:
The modifier `override' is not valid for this item
I can actually get this to work without using explicit interfaces so it's not actually blocking me but I would really like know, for my own curiosity, what is the correct syntax if wanted to do this with explicit interfaces?
c# oop syntax interface
c# oop syntax interface
edited May 18 '16 at 22:23
asked May 18 '16 at 22:07
James McMahon
29.8k56174260
29.8k56174260
2
What's wrong with implicit interface implementation? What are you trying to accomplish?
– chomba
May 18 '16 at 22:23
@chomba, The use of explicit interfaces has been a style choice in my codebase. I just think it's a lot cleaner to look at a class that implements 4 or 5 different interfaces and know what methods are associated with what interface. It's been fine for the majority of my use cases, this is the first time I've run into a limitation with them.
– James McMahon
May 18 '16 at 22:27
@JamesMcMahon As far as I know the main use case for explicit is multiple interfaces with members with identical signatures. I very rarely see them used and if I did I'd feel like whoever wrote the code didn't write C# very often.
– Casey
May 18 '16 at 22:28
Yeah that gets into programmer preference, there is a bunch of answers at stackoverflow.com/questions/143405 that go into the pros / cons of each approach.
– James McMahon
May 18 '16 at 22:32
@JamesMcMahon Yes, I agree, but I feel like this is one of those things that's mostly agreed upon by people who use the language often. It's the same way I'd feel weird about seeing C# code that saidvar some_object = new my_class()
; there's nothing wrong with snake case but it feels out of place in C#.
– Casey
May 18 '16 at 23:50
add a comment |
2
What's wrong with implicit interface implementation? What are you trying to accomplish?
– chomba
May 18 '16 at 22:23
@chomba, The use of explicit interfaces has been a style choice in my codebase. I just think it's a lot cleaner to look at a class that implements 4 or 5 different interfaces and know what methods are associated with what interface. It's been fine for the majority of my use cases, this is the first time I've run into a limitation with them.
– James McMahon
May 18 '16 at 22:27
@JamesMcMahon As far as I know the main use case for explicit is multiple interfaces with members with identical signatures. I very rarely see them used and if I did I'd feel like whoever wrote the code didn't write C# very often.
– Casey
May 18 '16 at 22:28
Yeah that gets into programmer preference, there is a bunch of answers at stackoverflow.com/questions/143405 that go into the pros / cons of each approach.
– James McMahon
May 18 '16 at 22:32
@JamesMcMahon Yes, I agree, but I feel like this is one of those things that's mostly agreed upon by people who use the language often. It's the same way I'd feel weird about seeing C# code that saidvar some_object = new my_class()
; there's nothing wrong with snake case but it feels out of place in C#.
– Casey
May 18 '16 at 23:50
2
2
What's wrong with implicit interface implementation? What are you trying to accomplish?
– chomba
May 18 '16 at 22:23
What's wrong with implicit interface implementation? What are you trying to accomplish?
– chomba
May 18 '16 at 22:23
@chomba, The use of explicit interfaces has been a style choice in my codebase. I just think it's a lot cleaner to look at a class that implements 4 or 5 different interfaces and know what methods are associated with what interface. It's been fine for the majority of my use cases, this is the first time I've run into a limitation with them.
– James McMahon
May 18 '16 at 22:27
@chomba, The use of explicit interfaces has been a style choice in my codebase. I just think it's a lot cleaner to look at a class that implements 4 or 5 different interfaces and know what methods are associated with what interface. It's been fine for the majority of my use cases, this is the first time I've run into a limitation with them.
– James McMahon
May 18 '16 at 22:27
@JamesMcMahon As far as I know the main use case for explicit is multiple interfaces with members with identical signatures. I very rarely see them used and if I did I'd feel like whoever wrote the code didn't write C# very often.
– Casey
May 18 '16 at 22:28
@JamesMcMahon As far as I know the main use case for explicit is multiple interfaces with members with identical signatures. I very rarely see them used and if I did I'd feel like whoever wrote the code didn't write C# very often.
– Casey
May 18 '16 at 22:28
Yeah that gets into programmer preference, there is a bunch of answers at stackoverflow.com/questions/143405 that go into the pros / cons of each approach.
– James McMahon
May 18 '16 at 22:32
Yeah that gets into programmer preference, there is a bunch of answers at stackoverflow.com/questions/143405 that go into the pros / cons of each approach.
– James McMahon
May 18 '16 at 22:32
@JamesMcMahon Yes, I agree, but I feel like this is one of those things that's mostly agreed upon by people who use the language often. It's the same way I'd feel weird about seeing C# code that said
var some_object = new my_class()
; there's nothing wrong with snake case but it feels out of place in C#.– Casey
May 18 '16 at 23:50
@JamesMcMahon Yes, I agree, but I feel like this is one of those things that's mostly agreed upon by people who use the language often. It's the same way I'd feel weird about seeing C# code that said
var some_object = new my_class()
; there's nothing wrong with snake case but it feels out of place in C#.– Casey
May 18 '16 at 23:50
add a comment |
5 Answers
5
active
oldest
votes
An explicit interface implementation cannot be a virtual member. See section 13.4.1 of the C# language specification (it is outdated but this logic does not appear to have changed in C# 6.0). Specifically:
It is a compile-time error for an explicit interface member
implementation to include access modifiers, and it is a compile-time
error to include the modifiers abstract, virtual, override, or static.
This means, you will never be able to directly override this member.
What you can do as a workaround is to call another virtual method from your explicit implementation:
class Base : IBla
{
void IBla.DoSomething()
{
this.DoSomethingForIBla();
}
protected virtual void DoSomethingForIBla()
{
...
}
}
class Derived : Base
{
protected override void DoSomethingForIBla()
{
...
}
}
Great answer with the link to the docs. I've been using explicit interface as a stylistic choice without realize they had that limitation.
– James McMahon
May 18 '16 at 22:22
add a comment |
I also had a situation where I thought I wanted to override an explicit interface implementation and call the base class and found this question with its answers saying "can't be done".
The first thing to note is that in order to override the explicit interface implementation WITHOUT calling the base class is fairly simple. The derived class merely needs to implement the interface itself.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo";
}
// Note: any other interface functions will call ParentTest's implementation
}
However, there is now no "legitimate" way to call ParentTest
's implementation of ITest.Speak
on an object of type ChildTest
, as any attempt to use the interface will result in ChildTest
's implementation being called instead.
Thus, it is only the call to the base implementation that causes complication. To satisfy my curiosity, I proved that it CAN be done, but really it shouldn't...
Leaving the base class unchanged, the following does in fact allow the base class to be called using reflection.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo" + typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic).Invoke(this, new object[0]) as string;
}
}
NB if the sample code is enclosed in a namespace, the fully qualified interface name is required. e.g. "MyNamespace.ITest.Speak"
If the function will be called repeatedly and/or for many objects, performance can be improved by caching the method info, and/or creating a delegate for the base call, e.g.:
public class ChildTest : ParentTest, ITest
{
static ChildTest()
{
baseSpeakMethodInfo = typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic);
}
static private MethodInfo baseSpeakMethodInfo;
public ChildTest()
{
baseSpeak = baseSpeakMethodInfo.CreateDelegate(typeof(Func<string>), this) as Func<string>;
}
private Func<string> baseSpeak;
string ITest.Speak()
{
return "Mooo" + baseSpeak();
}
}
The only advantage this has over other answers is that it works if you cannot modify the base class. Otherwise, it's a horrible solution, and a mechanism should be created in the base class (as in other answers) to provide the derived class with a legitimate way to call the base implementation.
add a comment |
You can use a protected virtual
method, and keep the implementation non-public, so you still have explicit interface implementation which is just a wrapper around the implementation:
public class ParentTest : ITest
{
protected virtual string Speak_Impl()
{
return "Meow";
}
string ITest.Speak()
{
return Speak_Impl();
}
}
public class ChildTest : ParentTest
{
protected override string Speak_Impl()
{
return "Mooo";
}
}
Interesting, so there is no way to directly override an explicit interface implementation?
– James McMahon
May 18 '16 at 22:17
add a comment |
You can't override explicit interface implementations. They cannot be virtual so there's no way to directly override them. You can, however, make them indirectly virtual by having it call a protected virtual member:
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return Speak();
}
protected virtual string Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
protected override string Speak()
{
return "Mooo";
}
}
add a comment |
public virtual string Speak()
in the parent and public override string Speak()
in the child should work fine. You cannot use explicit interfaces for this use case. You can work around it by declaring a protected member and calling it in the explicit interface implementations if you need to use them.
1
That's no longer an explicit interface at that point. If you try and add the virtual keyword in front an explicit interface you geterror CS0106: The modifier
virtual' is not valid for this item`
– James McMahon
May 18 '16 at 22:12
@JamesMcMahon There's no reason your example needs to be an explicit interface; if that's a requirement you should change your example/question.
– Casey
May 18 '16 at 22:13
Updated the question to clarify things
– James McMahon
May 18 '16 at 22:14
@JamesMcMahon Explicit interface is only needed when you have to implement multiple interfaces with methods with the same signatures, and in this case the answer is to create methods that don't have those names and call them in the explicit interface implementations
– Casey
May 18 '16 at 22:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f37310896%2foverriding-explicit-interface-implementations%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
An explicit interface implementation cannot be a virtual member. See section 13.4.1 of the C# language specification (it is outdated but this logic does not appear to have changed in C# 6.0). Specifically:
It is a compile-time error for an explicit interface member
implementation to include access modifiers, and it is a compile-time
error to include the modifiers abstract, virtual, override, or static.
This means, you will never be able to directly override this member.
What you can do as a workaround is to call another virtual method from your explicit implementation:
class Base : IBla
{
void IBla.DoSomething()
{
this.DoSomethingForIBla();
}
protected virtual void DoSomethingForIBla()
{
...
}
}
class Derived : Base
{
protected override void DoSomethingForIBla()
{
...
}
}
Great answer with the link to the docs. I've been using explicit interface as a stylistic choice without realize they had that limitation.
– James McMahon
May 18 '16 at 22:22
add a comment |
An explicit interface implementation cannot be a virtual member. See section 13.4.1 of the C# language specification (it is outdated but this logic does not appear to have changed in C# 6.0). Specifically:
It is a compile-time error for an explicit interface member
implementation to include access modifiers, and it is a compile-time
error to include the modifiers abstract, virtual, override, or static.
This means, you will never be able to directly override this member.
What you can do as a workaround is to call another virtual method from your explicit implementation:
class Base : IBla
{
void IBla.DoSomething()
{
this.DoSomethingForIBla();
}
protected virtual void DoSomethingForIBla()
{
...
}
}
class Derived : Base
{
protected override void DoSomethingForIBla()
{
...
}
}
Great answer with the link to the docs. I've been using explicit interface as a stylistic choice without realize they had that limitation.
– James McMahon
May 18 '16 at 22:22
add a comment |
An explicit interface implementation cannot be a virtual member. See section 13.4.1 of the C# language specification (it is outdated but this logic does not appear to have changed in C# 6.0). Specifically:
It is a compile-time error for an explicit interface member
implementation to include access modifiers, and it is a compile-time
error to include the modifiers abstract, virtual, override, or static.
This means, you will never be able to directly override this member.
What you can do as a workaround is to call another virtual method from your explicit implementation:
class Base : IBla
{
void IBla.DoSomething()
{
this.DoSomethingForIBla();
}
protected virtual void DoSomethingForIBla()
{
...
}
}
class Derived : Base
{
protected override void DoSomethingForIBla()
{
...
}
}
An explicit interface implementation cannot be a virtual member. See section 13.4.1 of the C# language specification (it is outdated but this logic does not appear to have changed in C# 6.0). Specifically:
It is a compile-time error for an explicit interface member
implementation to include access modifiers, and it is a compile-time
error to include the modifiers abstract, virtual, override, or static.
This means, you will never be able to directly override this member.
What you can do as a workaround is to call another virtual method from your explicit implementation:
class Base : IBla
{
void IBla.DoSomething()
{
this.DoSomethingForIBla();
}
protected virtual void DoSomethingForIBla()
{
...
}
}
class Derived : Base
{
protected override void DoSomethingForIBla()
{
...
}
}
answered May 18 '16 at 22:17
Bas
22.4k43773
22.4k43773
Great answer with the link to the docs. I've been using explicit interface as a stylistic choice without realize they had that limitation.
– James McMahon
May 18 '16 at 22:22
add a comment |
Great answer with the link to the docs. I've been using explicit interface as a stylistic choice without realize they had that limitation.
– James McMahon
May 18 '16 at 22:22
Great answer with the link to the docs. I've been using explicit interface as a stylistic choice without realize they had that limitation.
– James McMahon
May 18 '16 at 22:22
Great answer with the link to the docs. I've been using explicit interface as a stylistic choice without realize they had that limitation.
– James McMahon
May 18 '16 at 22:22
add a comment |
I also had a situation where I thought I wanted to override an explicit interface implementation and call the base class and found this question with its answers saying "can't be done".
The first thing to note is that in order to override the explicit interface implementation WITHOUT calling the base class is fairly simple. The derived class merely needs to implement the interface itself.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo";
}
// Note: any other interface functions will call ParentTest's implementation
}
However, there is now no "legitimate" way to call ParentTest
's implementation of ITest.Speak
on an object of type ChildTest
, as any attempt to use the interface will result in ChildTest
's implementation being called instead.
Thus, it is only the call to the base implementation that causes complication. To satisfy my curiosity, I proved that it CAN be done, but really it shouldn't...
Leaving the base class unchanged, the following does in fact allow the base class to be called using reflection.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo" + typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic).Invoke(this, new object[0]) as string;
}
}
NB if the sample code is enclosed in a namespace, the fully qualified interface name is required. e.g. "MyNamespace.ITest.Speak"
If the function will be called repeatedly and/or for many objects, performance can be improved by caching the method info, and/or creating a delegate for the base call, e.g.:
public class ChildTest : ParentTest, ITest
{
static ChildTest()
{
baseSpeakMethodInfo = typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic);
}
static private MethodInfo baseSpeakMethodInfo;
public ChildTest()
{
baseSpeak = baseSpeakMethodInfo.CreateDelegate(typeof(Func<string>), this) as Func<string>;
}
private Func<string> baseSpeak;
string ITest.Speak()
{
return "Mooo" + baseSpeak();
}
}
The only advantage this has over other answers is that it works if you cannot modify the base class. Otherwise, it's a horrible solution, and a mechanism should be created in the base class (as in other answers) to provide the derived class with a legitimate way to call the base implementation.
add a comment |
I also had a situation where I thought I wanted to override an explicit interface implementation and call the base class and found this question with its answers saying "can't be done".
The first thing to note is that in order to override the explicit interface implementation WITHOUT calling the base class is fairly simple. The derived class merely needs to implement the interface itself.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo";
}
// Note: any other interface functions will call ParentTest's implementation
}
However, there is now no "legitimate" way to call ParentTest
's implementation of ITest.Speak
on an object of type ChildTest
, as any attempt to use the interface will result in ChildTest
's implementation being called instead.
Thus, it is only the call to the base implementation that causes complication. To satisfy my curiosity, I proved that it CAN be done, but really it shouldn't...
Leaving the base class unchanged, the following does in fact allow the base class to be called using reflection.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo" + typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic).Invoke(this, new object[0]) as string;
}
}
NB if the sample code is enclosed in a namespace, the fully qualified interface name is required. e.g. "MyNamespace.ITest.Speak"
If the function will be called repeatedly and/or for many objects, performance can be improved by caching the method info, and/or creating a delegate for the base call, e.g.:
public class ChildTest : ParentTest, ITest
{
static ChildTest()
{
baseSpeakMethodInfo = typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic);
}
static private MethodInfo baseSpeakMethodInfo;
public ChildTest()
{
baseSpeak = baseSpeakMethodInfo.CreateDelegate(typeof(Func<string>), this) as Func<string>;
}
private Func<string> baseSpeak;
string ITest.Speak()
{
return "Mooo" + baseSpeak();
}
}
The only advantage this has over other answers is that it works if you cannot modify the base class. Otherwise, it's a horrible solution, and a mechanism should be created in the base class (as in other answers) to provide the derived class with a legitimate way to call the base implementation.
add a comment |
I also had a situation where I thought I wanted to override an explicit interface implementation and call the base class and found this question with its answers saying "can't be done".
The first thing to note is that in order to override the explicit interface implementation WITHOUT calling the base class is fairly simple. The derived class merely needs to implement the interface itself.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo";
}
// Note: any other interface functions will call ParentTest's implementation
}
However, there is now no "legitimate" way to call ParentTest
's implementation of ITest.Speak
on an object of type ChildTest
, as any attempt to use the interface will result in ChildTest
's implementation being called instead.
Thus, it is only the call to the base implementation that causes complication. To satisfy my curiosity, I proved that it CAN be done, but really it shouldn't...
Leaving the base class unchanged, the following does in fact allow the base class to be called using reflection.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo" + typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic).Invoke(this, new object[0]) as string;
}
}
NB if the sample code is enclosed in a namespace, the fully qualified interface name is required. e.g. "MyNamespace.ITest.Speak"
If the function will be called repeatedly and/or for many objects, performance can be improved by caching the method info, and/or creating a delegate for the base call, e.g.:
public class ChildTest : ParentTest, ITest
{
static ChildTest()
{
baseSpeakMethodInfo = typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic);
}
static private MethodInfo baseSpeakMethodInfo;
public ChildTest()
{
baseSpeak = baseSpeakMethodInfo.CreateDelegate(typeof(Func<string>), this) as Func<string>;
}
private Func<string> baseSpeak;
string ITest.Speak()
{
return "Mooo" + baseSpeak();
}
}
The only advantage this has over other answers is that it works if you cannot modify the base class. Otherwise, it's a horrible solution, and a mechanism should be created in the base class (as in other answers) to provide the derived class with a legitimate way to call the base implementation.
I also had a situation where I thought I wanted to override an explicit interface implementation and call the base class and found this question with its answers saying "can't be done".
The first thing to note is that in order to override the explicit interface implementation WITHOUT calling the base class is fairly simple. The derived class merely needs to implement the interface itself.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo";
}
// Note: any other interface functions will call ParentTest's implementation
}
However, there is now no "legitimate" way to call ParentTest
's implementation of ITest.Speak
on an object of type ChildTest
, as any attempt to use the interface will result in ChildTest
's implementation being called instead.
Thus, it is only the call to the base implementation that causes complication. To satisfy my curiosity, I proved that it CAN be done, but really it shouldn't...
Leaving the base class unchanged, the following does in fact allow the base class to be called using reflection.
public class ChildTest : ParentTest, ITest
{
string ITest.Speak()
{
return "Mooo" + typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic).Invoke(this, new object[0]) as string;
}
}
NB if the sample code is enclosed in a namespace, the fully qualified interface name is required. e.g. "MyNamespace.ITest.Speak"
If the function will be called repeatedly and/or for many objects, performance can be improved by caching the method info, and/or creating a delegate for the base call, e.g.:
public class ChildTest : ParentTest, ITest
{
static ChildTest()
{
baseSpeakMethodInfo = typeof(ParentTest).GetMethod("ITest.Speak", BindingFlags.Instance | BindingFlags.NonPublic);
}
static private MethodInfo baseSpeakMethodInfo;
public ChildTest()
{
baseSpeak = baseSpeakMethodInfo.CreateDelegate(typeof(Func<string>), this) as Func<string>;
}
private Func<string> baseSpeak;
string ITest.Speak()
{
return "Mooo" + baseSpeak();
}
}
The only advantage this has over other answers is that it works if you cannot modify the base class. Otherwise, it's a horrible solution, and a mechanism should be created in the base class (as in other answers) to provide the derived class with a legitimate way to call the base implementation.
answered Feb 28 '18 at 9:45
Steve
24419
24419
add a comment |
add a comment |
You can use a protected virtual
method, and keep the implementation non-public, so you still have explicit interface implementation which is just a wrapper around the implementation:
public class ParentTest : ITest
{
protected virtual string Speak_Impl()
{
return "Meow";
}
string ITest.Speak()
{
return Speak_Impl();
}
}
public class ChildTest : ParentTest
{
protected override string Speak_Impl()
{
return "Mooo";
}
}
Interesting, so there is no way to directly override an explicit interface implementation?
– James McMahon
May 18 '16 at 22:17
add a comment |
You can use a protected virtual
method, and keep the implementation non-public, so you still have explicit interface implementation which is just a wrapper around the implementation:
public class ParentTest : ITest
{
protected virtual string Speak_Impl()
{
return "Meow";
}
string ITest.Speak()
{
return Speak_Impl();
}
}
public class ChildTest : ParentTest
{
protected override string Speak_Impl()
{
return "Mooo";
}
}
Interesting, so there is no way to directly override an explicit interface implementation?
– James McMahon
May 18 '16 at 22:17
add a comment |
You can use a protected virtual
method, and keep the implementation non-public, so you still have explicit interface implementation which is just a wrapper around the implementation:
public class ParentTest : ITest
{
protected virtual string Speak_Impl()
{
return "Meow";
}
string ITest.Speak()
{
return Speak_Impl();
}
}
public class ChildTest : ParentTest
{
protected override string Speak_Impl()
{
return "Mooo";
}
}
You can use a protected virtual
method, and keep the implementation non-public, so you still have explicit interface implementation which is just a wrapper around the implementation:
public class ParentTest : ITest
{
protected virtual string Speak_Impl()
{
return "Meow";
}
string ITest.Speak()
{
return Speak_Impl();
}
}
public class ChildTest : ParentTest
{
protected override string Speak_Impl()
{
return "Mooo";
}
}
answered May 18 '16 at 22:15
Joe Enos
30.5k1059122
30.5k1059122
Interesting, so there is no way to directly override an explicit interface implementation?
– James McMahon
May 18 '16 at 22:17
add a comment |
Interesting, so there is no way to directly override an explicit interface implementation?
– James McMahon
May 18 '16 at 22:17
Interesting, so there is no way to directly override an explicit interface implementation?
– James McMahon
May 18 '16 at 22:17
Interesting, so there is no way to directly override an explicit interface implementation?
– James McMahon
May 18 '16 at 22:17
add a comment |
You can't override explicit interface implementations. They cannot be virtual so there's no way to directly override them. You can, however, make them indirectly virtual by having it call a protected virtual member:
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return Speak();
}
protected virtual string Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
protected override string Speak()
{
return "Mooo";
}
}
add a comment |
You can't override explicit interface implementations. They cannot be virtual so there's no way to directly override them. You can, however, make them indirectly virtual by having it call a protected virtual member:
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return Speak();
}
protected virtual string Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
protected override string Speak()
{
return "Mooo";
}
}
add a comment |
You can't override explicit interface implementations. They cannot be virtual so there's no way to directly override them. You can, however, make them indirectly virtual by having it call a protected virtual member:
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return Speak();
}
protected virtual string Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
protected override string Speak()
{
return "Mooo";
}
}
You can't override explicit interface implementations. They cannot be virtual so there's no way to directly override them. You can, however, make them indirectly virtual by having it call a protected virtual member:
public interface ITest
{
string Speak();
}
public class ParentTest : ITest
{
string ITest.Speak()
{
return Speak();
}
protected virtual string Speak()
{
return "Meow";
}
}
public class ChildTest : ParentTest
{
protected override string Speak()
{
return "Mooo";
}
}
answered May 18 '16 at 22:17
D Stanley
122k9113174
122k9113174
add a comment |
add a comment |
public virtual string Speak()
in the parent and public override string Speak()
in the child should work fine. You cannot use explicit interfaces for this use case. You can work around it by declaring a protected member and calling it in the explicit interface implementations if you need to use them.
1
That's no longer an explicit interface at that point. If you try and add the virtual keyword in front an explicit interface you geterror CS0106: The modifier
virtual' is not valid for this item`
– James McMahon
May 18 '16 at 22:12
@JamesMcMahon There's no reason your example needs to be an explicit interface; if that's a requirement you should change your example/question.
– Casey
May 18 '16 at 22:13
Updated the question to clarify things
– James McMahon
May 18 '16 at 22:14
@JamesMcMahon Explicit interface is only needed when you have to implement multiple interfaces with methods with the same signatures, and in this case the answer is to create methods that don't have those names and call them in the explicit interface implementations
– Casey
May 18 '16 at 22:15
add a comment |
public virtual string Speak()
in the parent and public override string Speak()
in the child should work fine. You cannot use explicit interfaces for this use case. You can work around it by declaring a protected member and calling it in the explicit interface implementations if you need to use them.
1
That's no longer an explicit interface at that point. If you try and add the virtual keyword in front an explicit interface you geterror CS0106: The modifier
virtual' is not valid for this item`
– James McMahon
May 18 '16 at 22:12
@JamesMcMahon There's no reason your example needs to be an explicit interface; if that's a requirement you should change your example/question.
– Casey
May 18 '16 at 22:13
Updated the question to clarify things
– James McMahon
May 18 '16 at 22:14
@JamesMcMahon Explicit interface is only needed when you have to implement multiple interfaces with methods with the same signatures, and in this case the answer is to create methods that don't have those names and call them in the explicit interface implementations
– Casey
May 18 '16 at 22:15
add a comment |
public virtual string Speak()
in the parent and public override string Speak()
in the child should work fine. You cannot use explicit interfaces for this use case. You can work around it by declaring a protected member and calling it in the explicit interface implementations if you need to use them.
public virtual string Speak()
in the parent and public override string Speak()
in the child should work fine. You cannot use explicit interfaces for this use case. You can work around it by declaring a protected member and calling it in the explicit interface implementations if you need to use them.
edited May 18 '16 at 23:51
answered May 18 '16 at 22:10
Casey
2,4961734
2,4961734
1
That's no longer an explicit interface at that point. If you try and add the virtual keyword in front an explicit interface you geterror CS0106: The modifier
virtual' is not valid for this item`
– James McMahon
May 18 '16 at 22:12
@JamesMcMahon There's no reason your example needs to be an explicit interface; if that's a requirement you should change your example/question.
– Casey
May 18 '16 at 22:13
Updated the question to clarify things
– James McMahon
May 18 '16 at 22:14
@JamesMcMahon Explicit interface is only needed when you have to implement multiple interfaces with methods with the same signatures, and in this case the answer is to create methods that don't have those names and call them in the explicit interface implementations
– Casey
May 18 '16 at 22:15
add a comment |
1
That's no longer an explicit interface at that point. If you try and add the virtual keyword in front an explicit interface you geterror CS0106: The modifier
virtual' is not valid for this item`
– James McMahon
May 18 '16 at 22:12
@JamesMcMahon There's no reason your example needs to be an explicit interface; if that's a requirement you should change your example/question.
– Casey
May 18 '16 at 22:13
Updated the question to clarify things
– James McMahon
May 18 '16 at 22:14
@JamesMcMahon Explicit interface is only needed when you have to implement multiple interfaces with methods with the same signatures, and in this case the answer is to create methods that don't have those names and call them in the explicit interface implementations
– Casey
May 18 '16 at 22:15
1
1
That's no longer an explicit interface at that point. If you try and add the virtual keyword in front an explicit interface you get
error CS0106: The modifier
virtual' is not valid for this item`– James McMahon
May 18 '16 at 22:12
That's no longer an explicit interface at that point. If you try and add the virtual keyword in front an explicit interface you get
error CS0106: The modifier
virtual' is not valid for this item`– James McMahon
May 18 '16 at 22:12
@JamesMcMahon There's no reason your example needs to be an explicit interface; if that's a requirement you should change your example/question.
– Casey
May 18 '16 at 22:13
@JamesMcMahon There's no reason your example needs to be an explicit interface; if that's a requirement you should change your example/question.
– Casey
May 18 '16 at 22:13
Updated the question to clarify things
– James McMahon
May 18 '16 at 22:14
Updated the question to clarify things
– James McMahon
May 18 '16 at 22:14
@JamesMcMahon Explicit interface is only needed when you have to implement multiple interfaces with methods with the same signatures, and in this case the answer is to create methods that don't have those names and call them in the explicit interface implementations
– Casey
May 18 '16 at 22:15
@JamesMcMahon Explicit interface is only needed when you have to implement multiple interfaces with methods with the same signatures, and in this case the answer is to create methods that don't have those names and call them in the explicit interface implementations
– Casey
May 18 '16 at 22:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f37310896%2foverriding-explicit-interface-implementations%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
endOU,EEr4effNW o,VZG6L
2
What's wrong with implicit interface implementation? What are you trying to accomplish?
– chomba
May 18 '16 at 22:23
@chomba, The use of explicit interfaces has been a style choice in my codebase. I just think it's a lot cleaner to look at a class that implements 4 or 5 different interfaces and know what methods are associated with what interface. It's been fine for the majority of my use cases, this is the first time I've run into a limitation with them.
– James McMahon
May 18 '16 at 22:27
@JamesMcMahon As far as I know the main use case for explicit is multiple interfaces with members with identical signatures. I very rarely see them used and if I did I'd feel like whoever wrote the code didn't write C# very often.
– Casey
May 18 '16 at 22:28
Yeah that gets into programmer preference, there is a bunch of answers at stackoverflow.com/questions/143405 that go into the pros / cons of each approach.
– James McMahon
May 18 '16 at 22:32
@JamesMcMahon Yes, I agree, but I feel like this is one of those things that's mostly agreed upon by people who use the language often. It's the same way I'd feel weird about seeing C# code that said
var some_object = new my_class()
; there's nothing wrong with snake case but it feels out of place in C#.– Casey
May 18 '16 at 23:50