iOS Swift Floating Action Button Moves When Changing Screens
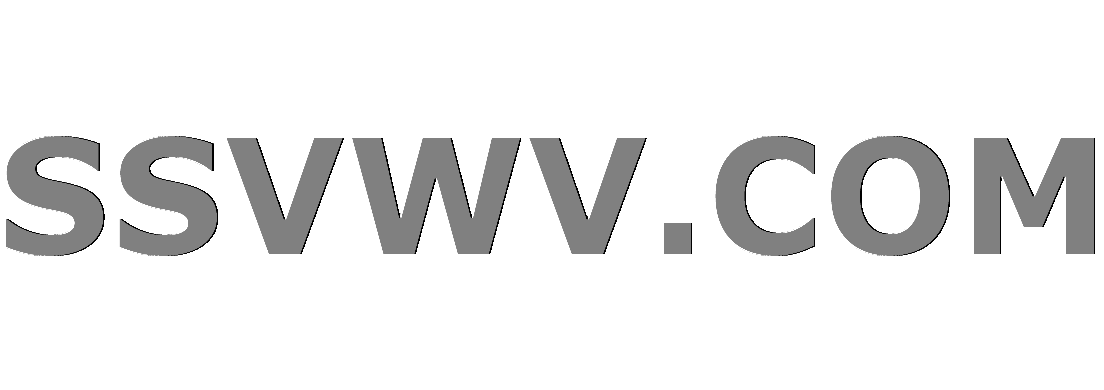
Multi tool use
I am having some trouble with my Floating Action Button in Swift. I am using Floaty found here: https://github.com/kciter/Floaty
. So it works well on the first time I click the "Calls" screen, but when I switch to another tab, it goes behind the tab bar. The code I use to set up the FAB can be found below.
What I think is going on is that whenever the tabs are switched, the view alternates between recognizing the tabBar and Safe Inset Areas, but sometimes it doesn't and only the paddingX and paddingY values I supplied in the beginning are supported.
I have tried switching between a TableViewController and a ViewController with a tableView in it, but that doesn't really seem to be the problem. I have also tried resetting the padding on every viewWillAppear() just to make sure it is at the right height, but that did not work either. Lastly, I tried to add constraints to the FAB. That was a complex process, that I don't think I did correctly, so it didn't end up working either. The code I used can be seen below.
Does anyone have any suggestions as to why this might be happening, or what I can do to make it work? Right now, my other option is to either get rid of the FAB entirely, or switch to some other library like Google's Material Design Components and use that FAB.
import UIKit
import Floaty
import Material
class CallCreationViewController: UIViewController, UISearchResultsUpdating, UISearchBarDelegate {
@IBOutlet weak var tableView: UITableView!
let floaty : Floaty = Floaty()
var connections: [User] =
var filteredConnections : [User] =
var selectedIds: [String] =
let inset : CGFloat = 34
let blue : Color = Color(red:0.09, green:0.04, blue:0.35, alpha:1.0)
override func viewDidLoad() {
super.viewDidLoad()
floaty.buttonColor = blue
floaty.plusColor = .white
let voiceItem : FloatyItem = FloatyItem()
voiceItem.title = "Start Voice Call"
voiceItem.icon = UIImage(imageLiteralResourceName: "baseline_phone_white_24pt")
voiceItem.iconTintColor = .white
voiceItem.buttonColor = blue
voiceItem.handler = { item in
self.performSegue(withIdentifier: "startVoiceCall", sender: self)
self.floaty.close()
}
let videoItem : FloatyItem = FloatyItem()
videoItem.title = "Start Video Call"
videoItem.icon = UIImage(imageLiteralResourceName: "baseline_video_call_white_24pt")
videoItem.iconTintColor = .white
videoItem.buttonColor = blue
videoItem.handler = { item in
self.performSegue(withIdentifier: "startVideoCall", sender: self)
self.floaty.close()
}
let broadcastItem : FloatyItem = FloatyItem()
broadcastItem.title = "Start Broadcast"
broadcastItem.icon = UIImage(imageLiteralResourceName: "baseline_settings_input_antenna_white_24pt")
broadcastItem.iconTintColor = .white
broadcastItem.buttonColor = blue
broadcastItem.handler = { item in
self.performSegue(withIdentifier: "startBroadcast", sender: self)
self.floaty.close()
}
floaty.addItem(item: voiceItem)
floaty.addItem(item: videoItem)
floaty.addItem(item: broadcastItem)
floaty.respondsToKeyboard = false
//let constraint = NSLayoutConstraint(item: floaty, attribute: NSLayoutConstraint.Attribute.bottomMargin, relatedBy: NSLayoutConstraint.Relation.equal, toItem: self.tabBarController?.tabBar, attribute: NSLayoutConstraint.Attribute.notAnAttribute, multiplier: 1, constant: 100)
floaty.sticky = true
floaty.paddingX = inset
floaty.paddingY = inset
self.view.addSubview(floaty)
//let constraint = NSLayoutConstraint(item: floaty, attribute: .bottomMargin, relatedBy: .equal, toItem: self.navigationController?.navigationBar, attribute: .top, multiplier: 1, constant: 100)
//floaty.addConstraint(constraint)
self.tableView.dataSource = self
// Do any additional setup after loading the view.
}
}
ios swift uitableview uitabbarcontroller
add a comment |
I am having some trouble with my Floating Action Button in Swift. I am using Floaty found here: https://github.com/kciter/Floaty
. So it works well on the first time I click the "Calls" screen, but when I switch to another tab, it goes behind the tab bar. The code I use to set up the FAB can be found below.
What I think is going on is that whenever the tabs are switched, the view alternates between recognizing the tabBar and Safe Inset Areas, but sometimes it doesn't and only the paddingX and paddingY values I supplied in the beginning are supported.
I have tried switching between a TableViewController and a ViewController with a tableView in it, but that doesn't really seem to be the problem. I have also tried resetting the padding on every viewWillAppear() just to make sure it is at the right height, but that did not work either. Lastly, I tried to add constraints to the FAB. That was a complex process, that I don't think I did correctly, so it didn't end up working either. The code I used can be seen below.
Does anyone have any suggestions as to why this might be happening, or what I can do to make it work? Right now, my other option is to either get rid of the FAB entirely, or switch to some other library like Google's Material Design Components and use that FAB.
import UIKit
import Floaty
import Material
class CallCreationViewController: UIViewController, UISearchResultsUpdating, UISearchBarDelegate {
@IBOutlet weak var tableView: UITableView!
let floaty : Floaty = Floaty()
var connections: [User] =
var filteredConnections : [User] =
var selectedIds: [String] =
let inset : CGFloat = 34
let blue : Color = Color(red:0.09, green:0.04, blue:0.35, alpha:1.0)
override func viewDidLoad() {
super.viewDidLoad()
floaty.buttonColor = blue
floaty.plusColor = .white
let voiceItem : FloatyItem = FloatyItem()
voiceItem.title = "Start Voice Call"
voiceItem.icon = UIImage(imageLiteralResourceName: "baseline_phone_white_24pt")
voiceItem.iconTintColor = .white
voiceItem.buttonColor = blue
voiceItem.handler = { item in
self.performSegue(withIdentifier: "startVoiceCall", sender: self)
self.floaty.close()
}
let videoItem : FloatyItem = FloatyItem()
videoItem.title = "Start Video Call"
videoItem.icon = UIImage(imageLiteralResourceName: "baseline_video_call_white_24pt")
videoItem.iconTintColor = .white
videoItem.buttonColor = blue
videoItem.handler = { item in
self.performSegue(withIdentifier: "startVideoCall", sender: self)
self.floaty.close()
}
let broadcastItem : FloatyItem = FloatyItem()
broadcastItem.title = "Start Broadcast"
broadcastItem.icon = UIImage(imageLiteralResourceName: "baseline_settings_input_antenna_white_24pt")
broadcastItem.iconTintColor = .white
broadcastItem.buttonColor = blue
broadcastItem.handler = { item in
self.performSegue(withIdentifier: "startBroadcast", sender: self)
self.floaty.close()
}
floaty.addItem(item: voiceItem)
floaty.addItem(item: videoItem)
floaty.addItem(item: broadcastItem)
floaty.respondsToKeyboard = false
//let constraint = NSLayoutConstraint(item: floaty, attribute: NSLayoutConstraint.Attribute.bottomMargin, relatedBy: NSLayoutConstraint.Relation.equal, toItem: self.tabBarController?.tabBar, attribute: NSLayoutConstraint.Attribute.notAnAttribute, multiplier: 1, constant: 100)
floaty.sticky = true
floaty.paddingX = inset
floaty.paddingY = inset
self.view.addSubview(floaty)
//let constraint = NSLayoutConstraint(item: floaty, attribute: .bottomMargin, relatedBy: .equal, toItem: self.navigationController?.navigationBar, attribute: .top, multiplier: 1, constant: 100)
//floaty.addConstraint(constraint)
self.tableView.dataSource = self
// Do any additional setup after loading the view.
}
}
ios swift uitableview uitabbarcontroller
add a comment |
I am having some trouble with my Floating Action Button in Swift. I am using Floaty found here: https://github.com/kciter/Floaty
. So it works well on the first time I click the "Calls" screen, but when I switch to another tab, it goes behind the tab bar. The code I use to set up the FAB can be found below.
What I think is going on is that whenever the tabs are switched, the view alternates between recognizing the tabBar and Safe Inset Areas, but sometimes it doesn't and only the paddingX and paddingY values I supplied in the beginning are supported.
I have tried switching between a TableViewController and a ViewController with a tableView in it, but that doesn't really seem to be the problem. I have also tried resetting the padding on every viewWillAppear() just to make sure it is at the right height, but that did not work either. Lastly, I tried to add constraints to the FAB. That was a complex process, that I don't think I did correctly, so it didn't end up working either. The code I used can be seen below.
Does anyone have any suggestions as to why this might be happening, or what I can do to make it work? Right now, my other option is to either get rid of the FAB entirely, or switch to some other library like Google's Material Design Components and use that FAB.
import UIKit
import Floaty
import Material
class CallCreationViewController: UIViewController, UISearchResultsUpdating, UISearchBarDelegate {
@IBOutlet weak var tableView: UITableView!
let floaty : Floaty = Floaty()
var connections: [User] =
var filteredConnections : [User] =
var selectedIds: [String] =
let inset : CGFloat = 34
let blue : Color = Color(red:0.09, green:0.04, blue:0.35, alpha:1.0)
override func viewDidLoad() {
super.viewDidLoad()
floaty.buttonColor = blue
floaty.plusColor = .white
let voiceItem : FloatyItem = FloatyItem()
voiceItem.title = "Start Voice Call"
voiceItem.icon = UIImage(imageLiteralResourceName: "baseline_phone_white_24pt")
voiceItem.iconTintColor = .white
voiceItem.buttonColor = blue
voiceItem.handler = { item in
self.performSegue(withIdentifier: "startVoiceCall", sender: self)
self.floaty.close()
}
let videoItem : FloatyItem = FloatyItem()
videoItem.title = "Start Video Call"
videoItem.icon = UIImage(imageLiteralResourceName: "baseline_video_call_white_24pt")
videoItem.iconTintColor = .white
videoItem.buttonColor = blue
videoItem.handler = { item in
self.performSegue(withIdentifier: "startVideoCall", sender: self)
self.floaty.close()
}
let broadcastItem : FloatyItem = FloatyItem()
broadcastItem.title = "Start Broadcast"
broadcastItem.icon = UIImage(imageLiteralResourceName: "baseline_settings_input_antenna_white_24pt")
broadcastItem.iconTintColor = .white
broadcastItem.buttonColor = blue
broadcastItem.handler = { item in
self.performSegue(withIdentifier: "startBroadcast", sender: self)
self.floaty.close()
}
floaty.addItem(item: voiceItem)
floaty.addItem(item: videoItem)
floaty.addItem(item: broadcastItem)
floaty.respondsToKeyboard = false
//let constraint = NSLayoutConstraint(item: floaty, attribute: NSLayoutConstraint.Attribute.bottomMargin, relatedBy: NSLayoutConstraint.Relation.equal, toItem: self.tabBarController?.tabBar, attribute: NSLayoutConstraint.Attribute.notAnAttribute, multiplier: 1, constant: 100)
floaty.sticky = true
floaty.paddingX = inset
floaty.paddingY = inset
self.view.addSubview(floaty)
//let constraint = NSLayoutConstraint(item: floaty, attribute: .bottomMargin, relatedBy: .equal, toItem: self.navigationController?.navigationBar, attribute: .top, multiplier: 1, constant: 100)
//floaty.addConstraint(constraint)
self.tableView.dataSource = self
// Do any additional setup after loading the view.
}
}
ios swift uitableview uitabbarcontroller
I am having some trouble with my Floating Action Button in Swift. I am using Floaty found here: https://github.com/kciter/Floaty
. So it works well on the first time I click the "Calls" screen, but when I switch to another tab, it goes behind the tab bar. The code I use to set up the FAB can be found below.
What I think is going on is that whenever the tabs are switched, the view alternates between recognizing the tabBar and Safe Inset Areas, but sometimes it doesn't and only the paddingX and paddingY values I supplied in the beginning are supported.
I have tried switching between a TableViewController and a ViewController with a tableView in it, but that doesn't really seem to be the problem. I have also tried resetting the padding on every viewWillAppear() just to make sure it is at the right height, but that did not work either. Lastly, I tried to add constraints to the FAB. That was a complex process, that I don't think I did correctly, so it didn't end up working either. The code I used can be seen below.
Does anyone have any suggestions as to why this might be happening, or what I can do to make it work? Right now, my other option is to either get rid of the FAB entirely, or switch to some other library like Google's Material Design Components and use that FAB.
import UIKit
import Floaty
import Material
class CallCreationViewController: UIViewController, UISearchResultsUpdating, UISearchBarDelegate {
@IBOutlet weak var tableView: UITableView!
let floaty : Floaty = Floaty()
var connections: [User] =
var filteredConnections : [User] =
var selectedIds: [String] =
let inset : CGFloat = 34
let blue : Color = Color(red:0.09, green:0.04, blue:0.35, alpha:1.0)
override func viewDidLoad() {
super.viewDidLoad()
floaty.buttonColor = blue
floaty.plusColor = .white
let voiceItem : FloatyItem = FloatyItem()
voiceItem.title = "Start Voice Call"
voiceItem.icon = UIImage(imageLiteralResourceName: "baseline_phone_white_24pt")
voiceItem.iconTintColor = .white
voiceItem.buttonColor = blue
voiceItem.handler = { item in
self.performSegue(withIdentifier: "startVoiceCall", sender: self)
self.floaty.close()
}
let videoItem : FloatyItem = FloatyItem()
videoItem.title = "Start Video Call"
videoItem.icon = UIImage(imageLiteralResourceName: "baseline_video_call_white_24pt")
videoItem.iconTintColor = .white
videoItem.buttonColor = blue
videoItem.handler = { item in
self.performSegue(withIdentifier: "startVideoCall", sender: self)
self.floaty.close()
}
let broadcastItem : FloatyItem = FloatyItem()
broadcastItem.title = "Start Broadcast"
broadcastItem.icon = UIImage(imageLiteralResourceName: "baseline_settings_input_antenna_white_24pt")
broadcastItem.iconTintColor = .white
broadcastItem.buttonColor = blue
broadcastItem.handler = { item in
self.performSegue(withIdentifier: "startBroadcast", sender: self)
self.floaty.close()
}
floaty.addItem(item: voiceItem)
floaty.addItem(item: videoItem)
floaty.addItem(item: broadcastItem)
floaty.respondsToKeyboard = false
//let constraint = NSLayoutConstraint(item: floaty, attribute: NSLayoutConstraint.Attribute.bottomMargin, relatedBy: NSLayoutConstraint.Relation.equal, toItem: self.tabBarController?.tabBar, attribute: NSLayoutConstraint.Attribute.notAnAttribute, multiplier: 1, constant: 100)
floaty.sticky = true
floaty.paddingX = inset
floaty.paddingY = inset
self.view.addSubview(floaty)
//let constraint = NSLayoutConstraint(item: floaty, attribute: .bottomMargin, relatedBy: .equal, toItem: self.navigationController?.navigationBar, attribute: .top, multiplier: 1, constant: 100)
//floaty.addConstraint(constraint)
self.tableView.dataSource = self
// Do any additional setup after loading the view.
}
}
ios swift uitableview uitabbarcontroller
ios swift uitableview uitabbarcontroller
asked Dec 27 '18 at 22:12
Brad Hoffman
427
427
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
While I'm not familiar with Floaty, my guess would be that it's your tableview being underneath the tab bar that's causing it to fluctuate in it's location.
For example if I added a generic UIView like this:
let greenView : UIView = UIView(frame: CGRect(x: self.view.frame.size.width - 100, y: self.view.frame.size.height - 100, width: 100, height: 100))
greenView.backgroundColor = UIColor.green
self.view.addSubview(greenView)
It would be underneath the tab bar.
If you're looking for a quick fix, and don't necessarily need your tableview to extend behind your tab bar, you should just be able to add this line:
self.edgesForExtendedLayout.remove(UIRectEdge.bottom)
before setting up the Floaty in viewDidLoad and your tableview won't extend below the bottom tab bar.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53951389%2fios-swift-floating-action-button-moves-when-changing-screens%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
While I'm not familiar with Floaty, my guess would be that it's your tableview being underneath the tab bar that's causing it to fluctuate in it's location.
For example if I added a generic UIView like this:
let greenView : UIView = UIView(frame: CGRect(x: self.view.frame.size.width - 100, y: self.view.frame.size.height - 100, width: 100, height: 100))
greenView.backgroundColor = UIColor.green
self.view.addSubview(greenView)
It would be underneath the tab bar.
If you're looking for a quick fix, and don't necessarily need your tableview to extend behind your tab bar, you should just be able to add this line:
self.edgesForExtendedLayout.remove(UIRectEdge.bottom)
before setting up the Floaty in viewDidLoad and your tableview won't extend below the bottom tab bar.
add a comment |
While I'm not familiar with Floaty, my guess would be that it's your tableview being underneath the tab bar that's causing it to fluctuate in it's location.
For example if I added a generic UIView like this:
let greenView : UIView = UIView(frame: CGRect(x: self.view.frame.size.width - 100, y: self.view.frame.size.height - 100, width: 100, height: 100))
greenView.backgroundColor = UIColor.green
self.view.addSubview(greenView)
It would be underneath the tab bar.
If you're looking for a quick fix, and don't necessarily need your tableview to extend behind your tab bar, you should just be able to add this line:
self.edgesForExtendedLayout.remove(UIRectEdge.bottom)
before setting up the Floaty in viewDidLoad and your tableview won't extend below the bottom tab bar.
add a comment |
While I'm not familiar with Floaty, my guess would be that it's your tableview being underneath the tab bar that's causing it to fluctuate in it's location.
For example if I added a generic UIView like this:
let greenView : UIView = UIView(frame: CGRect(x: self.view.frame.size.width - 100, y: self.view.frame.size.height - 100, width: 100, height: 100))
greenView.backgroundColor = UIColor.green
self.view.addSubview(greenView)
It would be underneath the tab bar.
If you're looking for a quick fix, and don't necessarily need your tableview to extend behind your tab bar, you should just be able to add this line:
self.edgesForExtendedLayout.remove(UIRectEdge.bottom)
before setting up the Floaty in viewDidLoad and your tableview won't extend below the bottom tab bar.
While I'm not familiar with Floaty, my guess would be that it's your tableview being underneath the tab bar that's causing it to fluctuate in it's location.
For example if I added a generic UIView like this:
let greenView : UIView = UIView(frame: CGRect(x: self.view.frame.size.width - 100, y: self.view.frame.size.height - 100, width: 100, height: 100))
greenView.backgroundColor = UIColor.green
self.view.addSubview(greenView)
It would be underneath the tab bar.
If you're looking for a quick fix, and don't necessarily need your tableview to extend behind your tab bar, you should just be able to add this line:
self.edgesForExtendedLayout.remove(UIRectEdge.bottom)
before setting up the Floaty in viewDidLoad and your tableview won't extend below the bottom tab bar.
answered Dec 27 '18 at 23:37
R4N
81724
81724
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53951389%2fios-swift-floating-action-button-moves-when-changing-screens%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cI28kpaL5qCwbU2,Lx3 uyI2unamgdaQ0u8,lFZP