Bean scanning for Spring Boot in IntelliJ
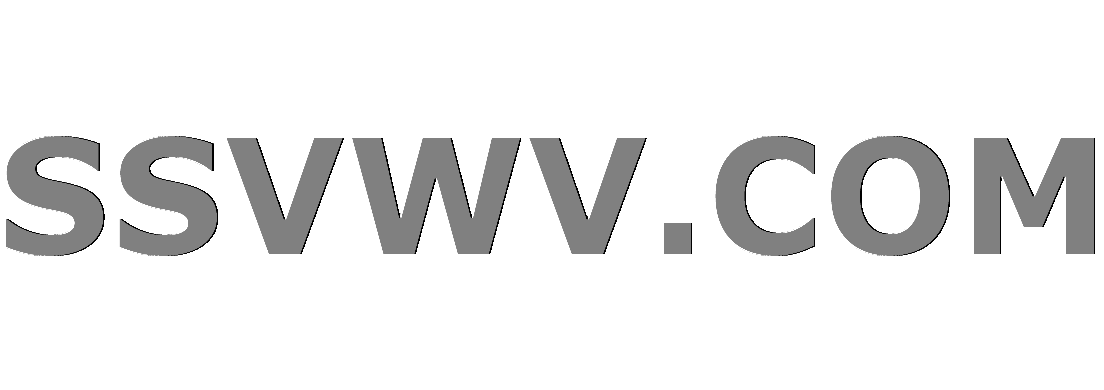
Multi tool use
I am using Spring Boot to create a micro service, and all is well, except for a couple of minor annoyances.
Autowiring in my test classes, I am getting the following warnings on @Autowired annotations:
Autowired members must be defined in the valid spring
bean(@Component/@Service,etc.) Checks that auto wired members are
defined in valid Spring bean (@Component|@Service|...).
this is using the following test class
@RunWith(SpringJUnit4ClassRunner.class)
@SpringApplicationConfiguration(classes = Application.class)
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
where my Application configuration class is defined as
@SpringBootApplication
public class Application {
public static void main(String args) {
SpringApplication.run(Application.class, args);
}
@Bean
public Marshaller getMarshaller() {
Jaxb2Marshaller marshaller = new Jaxb2Marshaller();
marshaller.setPackagesToScan("com.my.classes");
return marshaller;
}
}
and MyFactory has the @Component annotation:
@Component
public class MyFactory {
...
}
How can I resolve this?
Also, standing out like a sore thumb, the only uncovered line in my application is the SpringApplication.run line in the public static void main method:
public static void main(String args) {
SpringApplication.run(Application.class, args);
}
How can I get this covered or ignored?
unit-testing annotations spring-boot autowired
add a comment |
I am using Spring Boot to create a micro service, and all is well, except for a couple of minor annoyances.
Autowiring in my test classes, I am getting the following warnings on @Autowired annotations:
Autowired members must be defined in the valid spring
bean(@Component/@Service,etc.) Checks that auto wired members are
defined in valid Spring bean (@Component|@Service|...).
this is using the following test class
@RunWith(SpringJUnit4ClassRunner.class)
@SpringApplicationConfiguration(classes = Application.class)
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
where my Application configuration class is defined as
@SpringBootApplication
public class Application {
public static void main(String args) {
SpringApplication.run(Application.class, args);
}
@Bean
public Marshaller getMarshaller() {
Jaxb2Marshaller marshaller = new Jaxb2Marshaller();
marshaller.setPackagesToScan("com.my.classes");
return marshaller;
}
}
and MyFactory has the @Component annotation:
@Component
public class MyFactory {
...
}
How can I resolve this?
Also, standing out like a sore thumb, the only uncovered line in my application is the SpringApplication.run line in the public static void main method:
public static void main(String args) {
SpringApplication.run(Application.class, args);
}
How can I get this covered or ignored?
unit-testing annotations spring-boot autowired
I believe it's because:@SpringApplicationConfiguration
or@RunWith
doesnt have @Component inside the annotation. You could use@SpringBootTest
– Anand Varkey Philips
Sep 16 '18 at 18:59
add a comment |
I am using Spring Boot to create a micro service, and all is well, except for a couple of minor annoyances.
Autowiring in my test classes, I am getting the following warnings on @Autowired annotations:
Autowired members must be defined in the valid spring
bean(@Component/@Service,etc.) Checks that auto wired members are
defined in valid Spring bean (@Component|@Service|...).
this is using the following test class
@RunWith(SpringJUnit4ClassRunner.class)
@SpringApplicationConfiguration(classes = Application.class)
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
where my Application configuration class is defined as
@SpringBootApplication
public class Application {
public static void main(String args) {
SpringApplication.run(Application.class, args);
}
@Bean
public Marshaller getMarshaller() {
Jaxb2Marshaller marshaller = new Jaxb2Marshaller();
marshaller.setPackagesToScan("com.my.classes");
return marshaller;
}
}
and MyFactory has the @Component annotation:
@Component
public class MyFactory {
...
}
How can I resolve this?
Also, standing out like a sore thumb, the only uncovered line in my application is the SpringApplication.run line in the public static void main method:
public static void main(String args) {
SpringApplication.run(Application.class, args);
}
How can I get this covered or ignored?
unit-testing annotations spring-boot autowired
I am using Spring Boot to create a micro service, and all is well, except for a couple of minor annoyances.
Autowiring in my test classes, I am getting the following warnings on @Autowired annotations:
Autowired members must be defined in the valid spring
bean(@Component/@Service,etc.) Checks that auto wired members are
defined in valid Spring bean (@Component|@Service|...).
this is using the following test class
@RunWith(SpringJUnit4ClassRunner.class)
@SpringApplicationConfiguration(classes = Application.class)
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
where my Application configuration class is defined as
@SpringBootApplication
public class Application {
public static void main(String args) {
SpringApplication.run(Application.class, args);
}
@Bean
public Marshaller getMarshaller() {
Jaxb2Marshaller marshaller = new Jaxb2Marshaller();
marshaller.setPackagesToScan("com.my.classes");
return marshaller;
}
}
and MyFactory has the @Component annotation:
@Component
public class MyFactory {
...
}
How can I resolve this?
Also, standing out like a sore thumb, the only uncovered line in my application is the SpringApplication.run line in the public static void main method:
public static void main(String args) {
SpringApplication.run(Application.class, args);
}
How can I get this covered or ignored?
unit-testing annotations spring-boot autowired
unit-testing annotations spring-boot autowired
asked Sep 28 '15 at 11:26
Xetius
29.1k2477114
29.1k2477114
I believe it's because:@SpringApplicationConfiguration
or@RunWith
doesnt have @Component inside the annotation. You could use@SpringBootTest
– Anand Varkey Philips
Sep 16 '18 at 18:59
add a comment |
I believe it's because:@SpringApplicationConfiguration
or@RunWith
doesnt have @Component inside the annotation. You could use@SpringBootTest
– Anand Varkey Philips
Sep 16 '18 at 18:59
I believe it's because:
@SpringApplicationConfiguration
or @RunWith
doesnt have @Component inside the annotation. You could use @SpringBootTest
– Anand Varkey Philips
Sep 16 '18 at 18:59
I believe it's because:
@SpringApplicationConfiguration
or @RunWith
doesnt have @Component inside the annotation. You could use @SpringBootTest
– Anand Varkey Philips
Sep 16 '18 at 18:59
add a comment |
1 Answer
1
active
oldest
votes
As Anand stated, you need the following on your test for the dependencies to be autowired in tests:
@RunWith(SpringRunner.class)
@SpringBootTest
Like this:
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
@Xetius did this answer your question?
– skylerl
yesterday
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f32821839%2fbean-scanning-for-spring-boot-in-intellij%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
As Anand stated, you need the following on your test for the dependencies to be autowired in tests:
@RunWith(SpringRunner.class)
@SpringBootTest
Like this:
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
@Xetius did this answer your question?
– skylerl
yesterday
add a comment |
As Anand stated, you need the following on your test for the dependencies to be autowired in tests:
@RunWith(SpringRunner.class)
@SpringBootTest
Like this:
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
@Xetius did this answer your question?
– skylerl
yesterday
add a comment |
As Anand stated, you need the following on your test for the dependencies to be autowired in tests:
@RunWith(SpringRunner.class)
@SpringBootTest
Like this:
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
As Anand stated, you need the following on your test for the dependencies to be autowired in tests:
@RunWith(SpringRunner.class)
@SpringBootTest
Like this:
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyRecordTest {
public static final String NUMBER = "ABC/123456";
@Autowired
private MyFactory factory;
@Autowired
private Marshaller marshaller;
...
}
answered Dec 27 '18 at 22:09


skylerl
1,456102950
1,456102950
@Xetius did this answer your question?
– skylerl
yesterday
add a comment |
@Xetius did this answer your question?
– skylerl
yesterday
@Xetius did this answer your question?
– skylerl
yesterday
@Xetius did this answer your question?
– skylerl
yesterday
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f32821839%2fbean-scanning-for-spring-boot-in-intellij%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
V7kWOYUt xt3M4oDTUwHyG2B,9W,9EYJ RFmSM5cm2b,TEUxRbAwh
I believe it's because:
@SpringApplicationConfiguration
or@RunWith
doesnt have @Component inside the annotation. You could use@SpringBootTest
– Anand Varkey Philips
Sep 16 '18 at 18:59