AutocompleteTextView suggestions always selecting the first item in suggestions
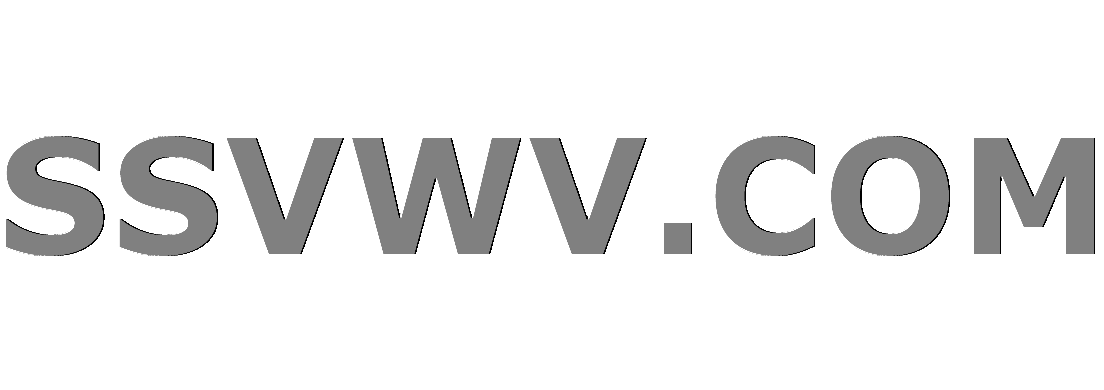
Multi tool use
I'm facing an issue that I cannot solve. I have created an AutoCompleteTextView that displays filtered suggestions from remote JSON data. The suggestions box pops up fine and filters properly. I have two issues which I cannot understand.
- When selecting the suggestion item the text in the
AutoCompleteTextView it's always set to the first item in the original
suggestions array, whether the data is filtered or not. - When deleting characters in the AutocompleteTextview, if it becomes
empty a null pointer exception is thrown
The code of my custom adapter:
package tz.co.fsm.fas;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.Filter;
import android.widget.Filterable;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class office_locations_adapter extends ArrayAdapter<office_location_data> implements Filterable {
private Context context;
private List<office_location_data> items, tempItems, suggestions;
int row_layout;
public office_locations_adapter(Context context, int row_layout, List<office_location_data> items) {
super(context, row_layout, items);
this.context = context;
this.row_layout = row_layout;
this.items = items;
tempItems = new ArrayList<>(items);
suggestions = new ArrayList<>();
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = convertView;
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = inflater.inflate(row_layout, parent, false);
}
office_location_data office = items.get(position);
if (office != null) {
TextView lbloffice = view.findViewById(R.id.rowtxtOfficeLocation);
if (lbloffice != null) {
lbloffice.setText(office.getOffice_location());
}
}
return view;
}
// This is important as it returns the count of the new arraylist when we filter it.
@Override
public int getCount() {
return items.size();
}
// Method to return the filter
@Override
public Filter getFilter() {
return performFiletring;
}
// Create a new filter. Here we perform the filtering results and use this in the getFilter() method
Filter performFiletring = new Filter() {
@Override
public CharSequence convertResultToString(Object resultValue) {
String str = ((office_location_data) resultValue).getOffice_location();
return str;
}
@Override
protected FilterResults performFiltering(CharSequence constraint) {
// We have some text to search
if (constraint != null) {
// CLear the suggestions array
suggestions.clear();
// Find the rows which match and add them to suggestions
for (office_location_data offices : tempItems) {
if (offices.getOffice_location().toLowerCase().contains(constraint.toString().toLowerCase())) {
suggestions.add(offices);
}
}
// Pass the filter results to the next step publish results
FilterResults filterResults = new FilterResults();
filterResults.values = suggestions;
filterResults.count = suggestions.size();
// System.out.println(filterResults.count);
return filterResults;
} else {
return new FilterResults();
}
}
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
items = (List) results.values;
if (results.count > 0) {
//suggestions = (office_location_data) results.values;
notifyDataSetChanged();
} else {
notifyDataSetInvalidated();
}
}
};
}

add a comment |
I'm facing an issue that I cannot solve. I have created an AutoCompleteTextView that displays filtered suggestions from remote JSON data. The suggestions box pops up fine and filters properly. I have two issues which I cannot understand.
- When selecting the suggestion item the text in the
AutoCompleteTextView it's always set to the first item in the original
suggestions array, whether the data is filtered or not. - When deleting characters in the AutocompleteTextview, if it becomes
empty a null pointer exception is thrown
The code of my custom adapter:
package tz.co.fsm.fas;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.Filter;
import android.widget.Filterable;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class office_locations_adapter extends ArrayAdapter<office_location_data> implements Filterable {
private Context context;
private List<office_location_data> items, tempItems, suggestions;
int row_layout;
public office_locations_adapter(Context context, int row_layout, List<office_location_data> items) {
super(context, row_layout, items);
this.context = context;
this.row_layout = row_layout;
this.items = items;
tempItems = new ArrayList<>(items);
suggestions = new ArrayList<>();
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = convertView;
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = inflater.inflate(row_layout, parent, false);
}
office_location_data office = items.get(position);
if (office != null) {
TextView lbloffice = view.findViewById(R.id.rowtxtOfficeLocation);
if (lbloffice != null) {
lbloffice.setText(office.getOffice_location());
}
}
return view;
}
// This is important as it returns the count of the new arraylist when we filter it.
@Override
public int getCount() {
return items.size();
}
// Method to return the filter
@Override
public Filter getFilter() {
return performFiletring;
}
// Create a new filter. Here we perform the filtering results and use this in the getFilter() method
Filter performFiletring = new Filter() {
@Override
public CharSequence convertResultToString(Object resultValue) {
String str = ((office_location_data) resultValue).getOffice_location();
return str;
}
@Override
protected FilterResults performFiltering(CharSequence constraint) {
// We have some text to search
if (constraint != null) {
// CLear the suggestions array
suggestions.clear();
// Find the rows which match and add them to suggestions
for (office_location_data offices : tempItems) {
if (offices.getOffice_location().toLowerCase().contains(constraint.toString().toLowerCase())) {
suggestions.add(offices);
}
}
// Pass the filter results to the next step publish results
FilterResults filterResults = new FilterResults();
filterResults.values = suggestions;
filterResults.count = suggestions.size();
// System.out.println(filterResults.count);
return filterResults;
} else {
return new FilterResults();
}
}
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
items = (List) results.values;
if (results.count > 0) {
//suggestions = (office_location_data) results.values;
notifyDataSetChanged();
} else {
notifyDataSetInvalidated();
}
}
};
}

add a comment |
I'm facing an issue that I cannot solve. I have created an AutoCompleteTextView that displays filtered suggestions from remote JSON data. The suggestions box pops up fine and filters properly. I have two issues which I cannot understand.
- When selecting the suggestion item the text in the
AutoCompleteTextView it's always set to the first item in the original
suggestions array, whether the data is filtered or not. - When deleting characters in the AutocompleteTextview, if it becomes
empty a null pointer exception is thrown
The code of my custom adapter:
package tz.co.fsm.fas;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.Filter;
import android.widget.Filterable;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class office_locations_adapter extends ArrayAdapter<office_location_data> implements Filterable {
private Context context;
private List<office_location_data> items, tempItems, suggestions;
int row_layout;
public office_locations_adapter(Context context, int row_layout, List<office_location_data> items) {
super(context, row_layout, items);
this.context = context;
this.row_layout = row_layout;
this.items = items;
tempItems = new ArrayList<>(items);
suggestions = new ArrayList<>();
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = convertView;
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = inflater.inflate(row_layout, parent, false);
}
office_location_data office = items.get(position);
if (office != null) {
TextView lbloffice = view.findViewById(R.id.rowtxtOfficeLocation);
if (lbloffice != null) {
lbloffice.setText(office.getOffice_location());
}
}
return view;
}
// This is important as it returns the count of the new arraylist when we filter it.
@Override
public int getCount() {
return items.size();
}
// Method to return the filter
@Override
public Filter getFilter() {
return performFiletring;
}
// Create a new filter. Here we perform the filtering results and use this in the getFilter() method
Filter performFiletring = new Filter() {
@Override
public CharSequence convertResultToString(Object resultValue) {
String str = ((office_location_data) resultValue).getOffice_location();
return str;
}
@Override
protected FilterResults performFiltering(CharSequence constraint) {
// We have some text to search
if (constraint != null) {
// CLear the suggestions array
suggestions.clear();
// Find the rows which match and add them to suggestions
for (office_location_data offices : tempItems) {
if (offices.getOffice_location().toLowerCase().contains(constraint.toString().toLowerCase())) {
suggestions.add(offices);
}
}
// Pass the filter results to the next step publish results
FilterResults filterResults = new FilterResults();
filterResults.values = suggestions;
filterResults.count = suggestions.size();
// System.out.println(filterResults.count);
return filterResults;
} else {
return new FilterResults();
}
}
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
items = (List) results.values;
if (results.count > 0) {
//suggestions = (office_location_data) results.values;
notifyDataSetChanged();
} else {
notifyDataSetInvalidated();
}
}
};
}

I'm facing an issue that I cannot solve. I have created an AutoCompleteTextView that displays filtered suggestions from remote JSON data. The suggestions box pops up fine and filters properly. I have two issues which I cannot understand.
- When selecting the suggestion item the text in the
AutoCompleteTextView it's always set to the first item in the original
suggestions array, whether the data is filtered or not. - When deleting characters in the AutocompleteTextview, if it becomes
empty a null pointer exception is thrown
The code of my custom adapter:
package tz.co.fsm.fas;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.Filter;
import android.widget.Filterable;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class office_locations_adapter extends ArrayAdapter<office_location_data> implements Filterable {
private Context context;
private List<office_location_data> items, tempItems, suggestions;
int row_layout;
public office_locations_adapter(Context context, int row_layout, List<office_location_data> items) {
super(context, row_layout, items);
this.context = context;
this.row_layout = row_layout;
this.items = items;
tempItems = new ArrayList<>(items);
suggestions = new ArrayList<>();
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = convertView;
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = inflater.inflate(row_layout, parent, false);
}
office_location_data office = items.get(position);
if (office != null) {
TextView lbloffice = view.findViewById(R.id.rowtxtOfficeLocation);
if (lbloffice != null) {
lbloffice.setText(office.getOffice_location());
}
}
return view;
}
// This is important as it returns the count of the new arraylist when we filter it.
@Override
public int getCount() {
return items.size();
}
// Method to return the filter
@Override
public Filter getFilter() {
return performFiletring;
}
// Create a new filter. Here we perform the filtering results and use this in the getFilter() method
Filter performFiletring = new Filter() {
@Override
public CharSequence convertResultToString(Object resultValue) {
String str = ((office_location_data) resultValue).getOffice_location();
return str;
}
@Override
protected FilterResults performFiltering(CharSequence constraint) {
// We have some text to search
if (constraint != null) {
// CLear the suggestions array
suggestions.clear();
// Find the rows which match and add them to suggestions
for (office_location_data offices : tempItems) {
if (offices.getOffice_location().toLowerCase().contains(constraint.toString().toLowerCase())) {
suggestions.add(offices);
}
}
// Pass the filter results to the next step publish results
FilterResults filterResults = new FilterResults();
filterResults.values = suggestions;
filterResults.count = suggestions.size();
// System.out.println(filterResults.count);
return filterResults;
} else {
return new FilterResults();
}
}
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
items = (List) results.values;
if (results.count > 0) {
//suggestions = (office_location_data) results.values;
notifyDataSetChanged();
} else {
notifyDataSetInvalidated();
}
}
};
}


asked Dec 30 '18 at 8:51


Fabrizio MazzoniFabrizio Mazzoni
853925
853925
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Found the issue. The publishResults()
method was wrong:
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
List<office_location_data> items = (ArrayList<office_location_data>) results.values;
if (results != null && results.count > 0) {
clear();
for (office_location_data offices : suggestions) {
add(offices);
notifyDataSetChanged();
}
} else {
notifyDataSetInvalidated();
}
}
This is how I did it.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53976319%2fautocompletetextview-suggestions-always-selecting-the-first-item-in-suggestions%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Found the issue. The publishResults()
method was wrong:
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
List<office_location_data> items = (ArrayList<office_location_data>) results.values;
if (results != null && results.count > 0) {
clear();
for (office_location_data offices : suggestions) {
add(offices);
notifyDataSetChanged();
}
} else {
notifyDataSetInvalidated();
}
}
This is how I did it.
add a comment |
Found the issue. The publishResults()
method was wrong:
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
List<office_location_data> items = (ArrayList<office_location_data>) results.values;
if (results != null && results.count > 0) {
clear();
for (office_location_data offices : suggestions) {
add(offices);
notifyDataSetChanged();
}
} else {
notifyDataSetInvalidated();
}
}
This is how I did it.
add a comment |
Found the issue. The publishResults()
method was wrong:
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
List<office_location_data> items = (ArrayList<office_location_data>) results.values;
if (results != null && results.count > 0) {
clear();
for (office_location_data offices : suggestions) {
add(offices);
notifyDataSetChanged();
}
} else {
notifyDataSetInvalidated();
}
}
This is how I did it.
Found the issue. The publishResults()
method was wrong:
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
List<office_location_data> items = (ArrayList<office_location_data>) results.values;
if (results != null && results.count > 0) {
clear();
for (office_location_data offices : suggestions) {
add(offices);
notifyDataSetChanged();
}
} else {
notifyDataSetInvalidated();
}
}
This is how I did it.
edited Dec 30 '18 at 13:20
answered Dec 30 '18 at 13:12


Fabrizio MazzoniFabrizio Mazzoni
853925
853925
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53976319%2fautocompletetextview-suggestions-always-selecting-the-first-item-in-suggestions%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0,CatAgesn1XT8Jsbb1q6brIaAL4UY4ccozTmLot