Declaration for Module Exporting Object
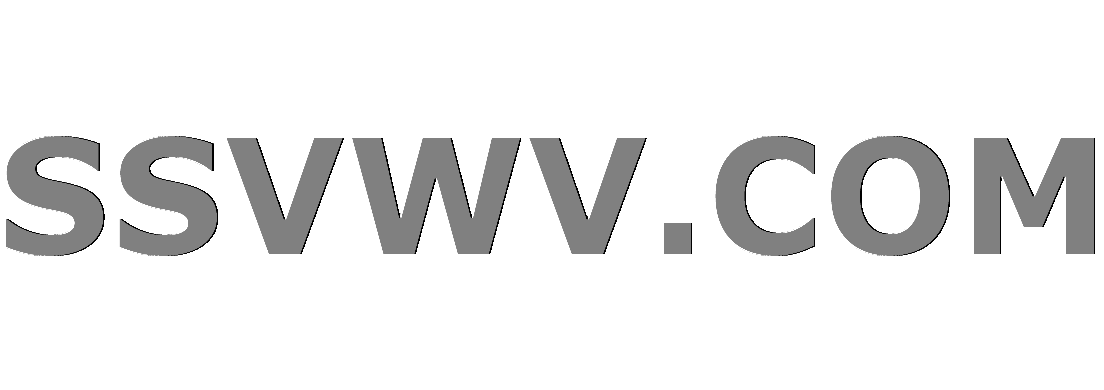
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have a project that compiles and exposes two CommonJS modules; each of them an object. Then, I have another project that consumes them.
The project structures are as follows:
multi-mod-package
|-mod1
| |-index.js
|-mod2
| |-index.js
|-package.json
multi-mod-consumer
|-index.ts
|-package.json
|-tsconfig.json
And the file contents:
multi-mod-package/mod1/index.js
module.exports.default = {
"count": "One"
};
multi-mod-package/mod2/index.js
module.exports.default = {
"count": "Two"
};
multi-mod-consumer/index.ts
import one from "multi-mod-package/mod1";
import two from "multi-mod-package/mod2";
console.log(`${one.count}, ${two.count}, Buckle My Shoes`);
multi-mod-consumer/tsconfig.json
{
"compilerOptions": {
"module": "commonjs",
"noImplicitAny": true
},
"include": [
"index.ts"
]
}
Without the noImplicitAny
, this works fine. The project compiles, and One, Two, Buckle My Shoes
is output as expected. However, the modules have no typings. I would like to enable noImplicitAny
and have proper typings for these modules, so I am trying to write declaration files for each. I have tried a number of iterations of declare module
and declare namespace
, but I keep getting "... is not a module" errors when attempting to consume the modules.
How do I write a declaration file for a module that exports an object?
UPDATE
Adding two definition files - multi-mod-package/mod1/index.d.ts
and multi-mod-package/mod1/index.d.ts
- with the contents declare module "multi-mod-package/mod1";
and declare module "multi-mod-package/mod1";
- respectively - gets rid of the "... is not a module" errors. However, there is still no proper typings; one.count
still comes up as type any
.
When I try to give the module a body (even just an empty one), TypeScript complains that the module has no default export. So, I still need a solution for telling TypeScript the module is an object containing the count
property.
typescript declaration-files
add a comment |
I have a project that compiles and exposes two CommonJS modules; each of them an object. Then, I have another project that consumes them.
The project structures are as follows:
multi-mod-package
|-mod1
| |-index.js
|-mod2
| |-index.js
|-package.json
multi-mod-consumer
|-index.ts
|-package.json
|-tsconfig.json
And the file contents:
multi-mod-package/mod1/index.js
module.exports.default = {
"count": "One"
};
multi-mod-package/mod2/index.js
module.exports.default = {
"count": "Two"
};
multi-mod-consumer/index.ts
import one from "multi-mod-package/mod1";
import two from "multi-mod-package/mod2";
console.log(`${one.count}, ${two.count}, Buckle My Shoes`);
multi-mod-consumer/tsconfig.json
{
"compilerOptions": {
"module": "commonjs",
"noImplicitAny": true
},
"include": [
"index.ts"
]
}
Without the noImplicitAny
, this works fine. The project compiles, and One, Two, Buckle My Shoes
is output as expected. However, the modules have no typings. I would like to enable noImplicitAny
and have proper typings for these modules, so I am trying to write declaration files for each. I have tried a number of iterations of declare module
and declare namespace
, but I keep getting "... is not a module" errors when attempting to consume the modules.
How do I write a declaration file for a module that exports an object?
UPDATE
Adding two definition files - multi-mod-package/mod1/index.d.ts
and multi-mod-package/mod1/index.d.ts
- with the contents declare module "multi-mod-package/mod1";
and declare module "multi-mod-package/mod1";
- respectively - gets rid of the "... is not a module" errors. However, there is still no proper typings; one.count
still comes up as type any
.
When I try to give the module a body (even just an empty one), TypeScript complains that the module has no default export. So, I still need a solution for telling TypeScript the module is an object containing the count
property.
typescript declaration-files
You need to provide an example. And what does it mean by exposing two CommonJS modules? You only have one "main" field in package.json.
– unional
Jan 3 at 22:12
@unional I added a basic code example. There is nomain
field; the modules are intended to be consumed as shown in the example.
– dawsonc623
Jan 4 at 19:51
trydeclare module 'multi-mod-packages/mod1'
and.../mod2'
directly.
– unional
Jan 5 at 2:01
Addingdeclare module "multi-mod-package/mod1";
helps TypeScript recognize the file as a module which is a huge step in the right direction. The original issue still stands though: now I need to let TypeScript know the module is an object. Updating the question with this new information. Thanks for the help so far!
– dawsonc623
Jan 7 at 14:32
add a comment |
I have a project that compiles and exposes two CommonJS modules; each of them an object. Then, I have another project that consumes them.
The project structures are as follows:
multi-mod-package
|-mod1
| |-index.js
|-mod2
| |-index.js
|-package.json
multi-mod-consumer
|-index.ts
|-package.json
|-tsconfig.json
And the file contents:
multi-mod-package/mod1/index.js
module.exports.default = {
"count": "One"
};
multi-mod-package/mod2/index.js
module.exports.default = {
"count": "Two"
};
multi-mod-consumer/index.ts
import one from "multi-mod-package/mod1";
import two from "multi-mod-package/mod2";
console.log(`${one.count}, ${two.count}, Buckle My Shoes`);
multi-mod-consumer/tsconfig.json
{
"compilerOptions": {
"module": "commonjs",
"noImplicitAny": true
},
"include": [
"index.ts"
]
}
Without the noImplicitAny
, this works fine. The project compiles, and One, Two, Buckle My Shoes
is output as expected. However, the modules have no typings. I would like to enable noImplicitAny
and have proper typings for these modules, so I am trying to write declaration files for each. I have tried a number of iterations of declare module
and declare namespace
, but I keep getting "... is not a module" errors when attempting to consume the modules.
How do I write a declaration file for a module that exports an object?
UPDATE
Adding two definition files - multi-mod-package/mod1/index.d.ts
and multi-mod-package/mod1/index.d.ts
- with the contents declare module "multi-mod-package/mod1";
and declare module "multi-mod-package/mod1";
- respectively - gets rid of the "... is not a module" errors. However, there is still no proper typings; one.count
still comes up as type any
.
When I try to give the module a body (even just an empty one), TypeScript complains that the module has no default export. So, I still need a solution for telling TypeScript the module is an object containing the count
property.
typescript declaration-files
I have a project that compiles and exposes two CommonJS modules; each of them an object. Then, I have another project that consumes them.
The project structures are as follows:
multi-mod-package
|-mod1
| |-index.js
|-mod2
| |-index.js
|-package.json
multi-mod-consumer
|-index.ts
|-package.json
|-tsconfig.json
And the file contents:
multi-mod-package/mod1/index.js
module.exports.default = {
"count": "One"
};
multi-mod-package/mod2/index.js
module.exports.default = {
"count": "Two"
};
multi-mod-consumer/index.ts
import one from "multi-mod-package/mod1";
import two from "multi-mod-package/mod2";
console.log(`${one.count}, ${two.count}, Buckle My Shoes`);
multi-mod-consumer/tsconfig.json
{
"compilerOptions": {
"module": "commonjs",
"noImplicitAny": true
},
"include": [
"index.ts"
]
}
Without the noImplicitAny
, this works fine. The project compiles, and One, Two, Buckle My Shoes
is output as expected. However, the modules have no typings. I would like to enable noImplicitAny
and have proper typings for these modules, so I am trying to write declaration files for each. I have tried a number of iterations of declare module
and declare namespace
, but I keep getting "... is not a module" errors when attempting to consume the modules.
How do I write a declaration file for a module that exports an object?
UPDATE
Adding two definition files - multi-mod-package/mod1/index.d.ts
and multi-mod-package/mod1/index.d.ts
- with the contents declare module "multi-mod-package/mod1";
and declare module "multi-mod-package/mod1";
- respectively - gets rid of the "... is not a module" errors. However, there is still no proper typings; one.count
still comes up as type any
.
When I try to give the module a body (even just an empty one), TypeScript complains that the module has no default export. So, I still need a solution for telling TypeScript the module is an object containing the count
property.
typescript declaration-files
typescript declaration-files
edited Jan 7 at 14:39
dawsonc623
asked Jan 3 at 21:44
dawsonc623dawsonc623
183315
183315
You need to provide an example. And what does it mean by exposing two CommonJS modules? You only have one "main" field in package.json.
– unional
Jan 3 at 22:12
@unional I added a basic code example. There is nomain
field; the modules are intended to be consumed as shown in the example.
– dawsonc623
Jan 4 at 19:51
trydeclare module 'multi-mod-packages/mod1'
and.../mod2'
directly.
– unional
Jan 5 at 2:01
Addingdeclare module "multi-mod-package/mod1";
helps TypeScript recognize the file as a module which is a huge step in the right direction. The original issue still stands though: now I need to let TypeScript know the module is an object. Updating the question with this new information. Thanks for the help so far!
– dawsonc623
Jan 7 at 14:32
add a comment |
You need to provide an example. And what does it mean by exposing two CommonJS modules? You only have one "main" field in package.json.
– unional
Jan 3 at 22:12
@unional I added a basic code example. There is nomain
field; the modules are intended to be consumed as shown in the example.
– dawsonc623
Jan 4 at 19:51
trydeclare module 'multi-mod-packages/mod1'
and.../mod2'
directly.
– unional
Jan 5 at 2:01
Addingdeclare module "multi-mod-package/mod1";
helps TypeScript recognize the file as a module which is a huge step in the right direction. The original issue still stands though: now I need to let TypeScript know the module is an object. Updating the question with this new information. Thanks for the help so far!
– dawsonc623
Jan 7 at 14:32
You need to provide an example. And what does it mean by exposing two CommonJS modules? You only have one "main" field in package.json.
– unional
Jan 3 at 22:12
You need to provide an example. And what does it mean by exposing two CommonJS modules? You only have one "main" field in package.json.
– unional
Jan 3 at 22:12
@unional I added a basic code example. There is no
main
field; the modules are intended to be consumed as shown in the example.– dawsonc623
Jan 4 at 19:51
@unional I added a basic code example. There is no
main
field; the modules are intended to be consumed as shown in the example.– dawsonc623
Jan 4 at 19:51
try
declare module 'multi-mod-packages/mod1'
and .../mod2'
directly.– unional
Jan 5 at 2:01
try
declare module 'multi-mod-packages/mod1'
and .../mod2'
directly.– unional
Jan 5 at 2:01
Adding
declare module "multi-mod-package/mod1";
helps TypeScript recognize the file as a module which is a huge step in the right direction. The original issue still stands though: now I need to let TypeScript know the module is an object. Updating the question with this new information. Thanks for the help so far!– dawsonc623
Jan 7 at 14:32
Adding
declare module "multi-mod-package/mod1";
helps TypeScript recognize the file as a module which is a huge step in the right direction. The original issue still stands though: now I need to let TypeScript know the module is an object. Updating the question with this new information. Thanks for the help so far!– dawsonc623
Jan 7 at 14:32
add a comment |
1 Answer
1
active
oldest
votes
You need to declare the sub-module as individual modules.
In your typings (e.g. typings/multi-mod-package.d.ts
):
declare module 'multi-mod-package/mod1' {
// typings for mod1
}
declare module 'multi-mod-package/mod2' {
// typings for mod2
}
For how to write your typings, you can check out the handbook:
https://www.typescriptlang.org/docs/handbook/declaration-files/introduction.html
TypeScript does not do automatic deeplinking because node resolution for deep linking can be different than the typings structure.
Node deep linking is resolved based on the location of package.json.
When trying this, I kept running into an error when trying to doimport mod1 from "multi-mod-package/mod1";
(andmod2
), but it turns out I needed to doimport * as mod1 from "multi-mod-package/mod1"
(andmod2
again). Once I imported that way everything worked as expected. Marking this as correct.
– dawsonc623
Jan 9 at 21:46
1
Turn onesModuleInterop
. It wil save you from so much troubles in the future.
– unional
Jan 9 at 21:49
Oh, I had no idea that existed. Thanks for the tip!
– dawsonc623
Jan 9 at 21:51
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030228%2fdeclaration-for-module-exporting-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to declare the sub-module as individual modules.
In your typings (e.g. typings/multi-mod-package.d.ts
):
declare module 'multi-mod-package/mod1' {
// typings for mod1
}
declare module 'multi-mod-package/mod2' {
// typings for mod2
}
For how to write your typings, you can check out the handbook:
https://www.typescriptlang.org/docs/handbook/declaration-files/introduction.html
TypeScript does not do automatic deeplinking because node resolution for deep linking can be different than the typings structure.
Node deep linking is resolved based on the location of package.json.
When trying this, I kept running into an error when trying to doimport mod1 from "multi-mod-package/mod1";
(andmod2
), but it turns out I needed to doimport * as mod1 from "multi-mod-package/mod1"
(andmod2
again). Once I imported that way everything worked as expected. Marking this as correct.
– dawsonc623
Jan 9 at 21:46
1
Turn onesModuleInterop
. It wil save you from so much troubles in the future.
– unional
Jan 9 at 21:49
Oh, I had no idea that existed. Thanks for the tip!
– dawsonc623
Jan 9 at 21:51
add a comment |
You need to declare the sub-module as individual modules.
In your typings (e.g. typings/multi-mod-package.d.ts
):
declare module 'multi-mod-package/mod1' {
// typings for mod1
}
declare module 'multi-mod-package/mod2' {
// typings for mod2
}
For how to write your typings, you can check out the handbook:
https://www.typescriptlang.org/docs/handbook/declaration-files/introduction.html
TypeScript does not do automatic deeplinking because node resolution for deep linking can be different than the typings structure.
Node deep linking is resolved based on the location of package.json.
When trying this, I kept running into an error when trying to doimport mod1 from "multi-mod-package/mod1";
(andmod2
), but it turns out I needed to doimport * as mod1 from "multi-mod-package/mod1"
(andmod2
again). Once I imported that way everything worked as expected. Marking this as correct.
– dawsonc623
Jan 9 at 21:46
1
Turn onesModuleInterop
. It wil save you from so much troubles in the future.
– unional
Jan 9 at 21:49
Oh, I had no idea that existed. Thanks for the tip!
– dawsonc623
Jan 9 at 21:51
add a comment |
You need to declare the sub-module as individual modules.
In your typings (e.g. typings/multi-mod-package.d.ts
):
declare module 'multi-mod-package/mod1' {
// typings for mod1
}
declare module 'multi-mod-package/mod2' {
// typings for mod2
}
For how to write your typings, you can check out the handbook:
https://www.typescriptlang.org/docs/handbook/declaration-files/introduction.html
TypeScript does not do automatic deeplinking because node resolution for deep linking can be different than the typings structure.
Node deep linking is resolved based on the location of package.json.
You need to declare the sub-module as individual modules.
In your typings (e.g. typings/multi-mod-package.d.ts
):
declare module 'multi-mod-package/mod1' {
// typings for mod1
}
declare module 'multi-mod-package/mod2' {
// typings for mod2
}
For how to write your typings, you can check out the handbook:
https://www.typescriptlang.org/docs/handbook/declaration-files/introduction.html
TypeScript does not do automatic deeplinking because node resolution for deep linking can be different than the typings structure.
Node deep linking is resolved based on the location of package.json.
answered Jan 9 at 4:06
unionalunional
6,12121531
6,12121531
When trying this, I kept running into an error when trying to doimport mod1 from "multi-mod-package/mod1";
(andmod2
), but it turns out I needed to doimport * as mod1 from "multi-mod-package/mod1"
(andmod2
again). Once I imported that way everything worked as expected. Marking this as correct.
– dawsonc623
Jan 9 at 21:46
1
Turn onesModuleInterop
. It wil save you from so much troubles in the future.
– unional
Jan 9 at 21:49
Oh, I had no idea that existed. Thanks for the tip!
– dawsonc623
Jan 9 at 21:51
add a comment |
When trying this, I kept running into an error when trying to doimport mod1 from "multi-mod-package/mod1";
(andmod2
), but it turns out I needed to doimport * as mod1 from "multi-mod-package/mod1"
(andmod2
again). Once I imported that way everything worked as expected. Marking this as correct.
– dawsonc623
Jan 9 at 21:46
1
Turn onesModuleInterop
. It wil save you from so much troubles in the future.
– unional
Jan 9 at 21:49
Oh, I had no idea that existed. Thanks for the tip!
– dawsonc623
Jan 9 at 21:51
When trying this, I kept running into an error when trying to do
import mod1 from "multi-mod-package/mod1";
(and mod2
), but it turns out I needed to do import * as mod1 from "multi-mod-package/mod1"
(and mod2
again). Once I imported that way everything worked as expected. Marking this as correct.– dawsonc623
Jan 9 at 21:46
When trying this, I kept running into an error when trying to do
import mod1 from "multi-mod-package/mod1";
(and mod2
), but it turns out I needed to do import * as mod1 from "multi-mod-package/mod1"
(and mod2
again). Once I imported that way everything worked as expected. Marking this as correct.– dawsonc623
Jan 9 at 21:46
1
1
Turn on
esModuleInterop
. It wil save you from so much troubles in the future.– unional
Jan 9 at 21:49
Turn on
esModuleInterop
. It wil save you from so much troubles in the future.– unional
Jan 9 at 21:49
Oh, I had no idea that existed. Thanks for the tip!
– dawsonc623
Jan 9 at 21:51
Oh, I had no idea that existed. Thanks for the tip!
– dawsonc623
Jan 9 at 21:51
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030228%2fdeclaration-for-module-exporting-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Z3LZj,hd4pJcDK2B6e1oGcspc,t
You need to provide an example. And what does it mean by exposing two CommonJS modules? You only have one "main" field in package.json.
– unional
Jan 3 at 22:12
@unional I added a basic code example. There is no
main
field; the modules are intended to be consumed as shown in the example.– dawsonc623
Jan 4 at 19:51
try
declare module 'multi-mod-packages/mod1'
and.../mod2'
directly.– unional
Jan 5 at 2:01
Adding
declare module "multi-mod-package/mod1";
helps TypeScript recognize the file as a module which is a huge step in the right direction. The original issue still stands though: now I need to let TypeScript know the module is an object. Updating the question with this new information. Thanks for the help so far!– dawsonc623
Jan 7 at 14:32