Converting a D object pointer to void* and passing to a callback
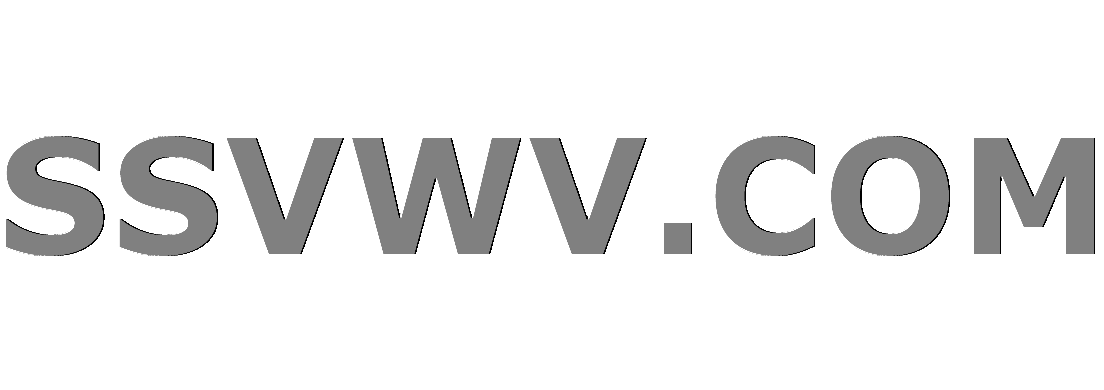
Multi tool use
I want to convert a D class pointer into void*
, pass this void*
pointer together with the pointer to my callback extern(C)
function to a C library routine.
The C library routine will call my callback extern(C)
function, which will convert void*
back to the class pointer and use the object of this class.
The trouble: I heard that GC objects may be moved to other locations (maybe not in current D version but in a future). Does this mean that my void*
pointer may become invalid (no more point to my object)?
If the problem really exists, how to solve it?
pointers garbage-collection d void-pointers
add a comment |
I want to convert a D class pointer into void*
, pass this void*
pointer together with the pointer to my callback extern(C)
function to a C library routine.
The C library routine will call my callback extern(C)
function, which will convert void*
back to the class pointer and use the object of this class.
The trouble: I heard that GC objects may be moved to other locations (maybe not in current D version but in a future). Does this mean that my void*
pointer may become invalid (no more point to my object)?
If the problem really exists, how to solve it?
pointers garbage-collection d void-pointers
add a comment |
I want to convert a D class pointer into void*
, pass this void*
pointer together with the pointer to my callback extern(C)
function to a C library routine.
The C library routine will call my callback extern(C)
function, which will convert void*
back to the class pointer and use the object of this class.
The trouble: I heard that GC objects may be moved to other locations (maybe not in current D version but in a future). Does this mean that my void*
pointer may become invalid (no more point to my object)?
If the problem really exists, how to solve it?
pointers garbage-collection d void-pointers
I want to convert a D class pointer into void*
, pass this void*
pointer together with the pointer to my callback extern(C)
function to a C library routine.
The C library routine will call my callback extern(C)
function, which will convert void*
back to the class pointer and use the object of this class.
The trouble: I heard that GC objects may be moved to other locations (maybe not in current D version but in a future). Does this mean that my void*
pointer may become invalid (no more point to my object)?
If the problem really exists, how to solve it?
pointers garbage-collection d void-pointers
pointers garbage-collection d void-pointers
asked Dec 31 '18 at 14:56
portonporton
1,81532242
1,81532242
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You can tell the GC to hold on to the pointer as a root and furthermore, tell it not to move it on you with the import core.memory; GC.addRoot(ptr);
function. This example shows it in full:
http://dpldocs.info/experimental-docs/core.memory.GC.addRoot.html#examples
// Typical C-style callback mechanism; the passed function
// is invoked with the user-supplied context pointer at a
// later point.
extern(C) void addCallback(void function(void*), void*);
// Allocate an object on the GC heap (this would usually be
// some application-specific context data).
auto context = new Object;
// Make sure that it is not collected even if it is no
// longer referenced from D code (stack, GC heap, …).
GC.addRoot(cast(void*)context);
// Also ensure that a moving collector does not relocate
// the object.
GC.setAttr(cast(void*)context, GC.BlkAttr.NO_MOVE);
// Now context can be safely passed to the C library.
addCallback(&myHandler, cast(void*)context);
extern(C) void myHandler(void* ctx)
{
// Assuming that the callback is invoked only once, the
// added root can be removed again now to allow the GC
// to collect it later.
GC.removeRoot(ctx);
GC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
auto context = cast(Object)ctx;
// Use context here…
}
I'd exchangeGC.removeRoot(ctx);
andGC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
, because in OOP resource should be freed in reverse order of their acquision.
– porton
Dec 31 '18 at 15:11
Will your code work if instead:scope auto context = new Object;
(that is Object allocated on the stack rather than on the heap)?
– porton
Dec 31 '18 at 15:36
Not in general - the danger of the callback is that it will outlive the scope of the caller, which means the stack allocated object is definitely dead. If the callback only happens in scope, it doesn't matter either way - the stack reference to the GC'd object will also be live anyway. (And if it just runs inside the C function, the D GC will never run anyway, unless there is another thread triggering it.)
– Adam D. Ruppe
Dec 31 '18 at 15:39
I wanted to ask whetherGC.addRoot(cast(void*)context);
will work ifcontext
is pointing onto the stack. I suspect it may segfault, throw an exception, or lead to undefined behavior. Is my fear founded?
– porton
Dec 31 '18 at 15:49
1
It is undefined; the documentation only says what it does if it points to a GC block or null.
– Adam D. Ruppe
Dec 31 '18 at 16:35
add a comment |
Based on Adam D. Ruppe's answer but reorganized.
Even better OO code:
import core.memory : GC;
class UnmovableObject {
this() {
//GC.addRoot(cast(void*)this); // prevents finalization
GC.setAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
~this() {
//GC.removeRoot(cast(void*)this);
GC.clrAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
}
1
That destructor will never run unless called manually (with the.destroy(obj)
function) since while the object is a GC root, it will never be collected and thus never destroyed.
– Adam D. Ruppe
Dec 31 '18 at 15:34
@AdamD.Ruppe That it cannot be done with a destructor is really bad. RAI idiom is broken here. I suggest to devise a new D language feature to solve this problem
– porton
Dec 31 '18 at 15:53
Hm, well: Why toaddRoot
andremoveRoot
?setAttr
/clrAttr
seems to work for any GC pointer, not only for a root. So I propose to removeC.addRoot(cast(void*)this);
andGC.removeRoot(cast(void*)this);
. I think after this my OO code will work. Right?
– porton
Dec 31 '18 at 15:57
Destructors are only triggered when the object is destroyed. A GC root is never destroyed (unless you manually do it). That's the whole point of that function! The original problem was the GC might free the block while C was holding on to it, because D's GC can't see C's internal memory. By adding it as a root, it tell's D's GC not to try to automatically free it until you tell it it is OK (via the removeRoot) function. This protects it from being freed while C is still holding on to it.
– Adam D. Ruppe
Dec 31 '18 at 16:43
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53988772%2fconverting-a-d-object-pointer-to-void-and-passing-to-a-callback%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can tell the GC to hold on to the pointer as a root and furthermore, tell it not to move it on you with the import core.memory; GC.addRoot(ptr);
function. This example shows it in full:
http://dpldocs.info/experimental-docs/core.memory.GC.addRoot.html#examples
// Typical C-style callback mechanism; the passed function
// is invoked with the user-supplied context pointer at a
// later point.
extern(C) void addCallback(void function(void*), void*);
// Allocate an object on the GC heap (this would usually be
// some application-specific context data).
auto context = new Object;
// Make sure that it is not collected even if it is no
// longer referenced from D code (stack, GC heap, …).
GC.addRoot(cast(void*)context);
// Also ensure that a moving collector does not relocate
// the object.
GC.setAttr(cast(void*)context, GC.BlkAttr.NO_MOVE);
// Now context can be safely passed to the C library.
addCallback(&myHandler, cast(void*)context);
extern(C) void myHandler(void* ctx)
{
// Assuming that the callback is invoked only once, the
// added root can be removed again now to allow the GC
// to collect it later.
GC.removeRoot(ctx);
GC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
auto context = cast(Object)ctx;
// Use context here…
}
I'd exchangeGC.removeRoot(ctx);
andGC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
, because in OOP resource should be freed in reverse order of their acquision.
– porton
Dec 31 '18 at 15:11
Will your code work if instead:scope auto context = new Object;
(that is Object allocated on the stack rather than on the heap)?
– porton
Dec 31 '18 at 15:36
Not in general - the danger of the callback is that it will outlive the scope of the caller, which means the stack allocated object is definitely dead. If the callback only happens in scope, it doesn't matter either way - the stack reference to the GC'd object will also be live anyway. (And if it just runs inside the C function, the D GC will never run anyway, unless there is another thread triggering it.)
– Adam D. Ruppe
Dec 31 '18 at 15:39
I wanted to ask whetherGC.addRoot(cast(void*)context);
will work ifcontext
is pointing onto the stack. I suspect it may segfault, throw an exception, or lead to undefined behavior. Is my fear founded?
– porton
Dec 31 '18 at 15:49
1
It is undefined; the documentation only says what it does if it points to a GC block or null.
– Adam D. Ruppe
Dec 31 '18 at 16:35
add a comment |
You can tell the GC to hold on to the pointer as a root and furthermore, tell it not to move it on you with the import core.memory; GC.addRoot(ptr);
function. This example shows it in full:
http://dpldocs.info/experimental-docs/core.memory.GC.addRoot.html#examples
// Typical C-style callback mechanism; the passed function
// is invoked with the user-supplied context pointer at a
// later point.
extern(C) void addCallback(void function(void*), void*);
// Allocate an object on the GC heap (this would usually be
// some application-specific context data).
auto context = new Object;
// Make sure that it is not collected even if it is no
// longer referenced from D code (stack, GC heap, …).
GC.addRoot(cast(void*)context);
// Also ensure that a moving collector does not relocate
// the object.
GC.setAttr(cast(void*)context, GC.BlkAttr.NO_MOVE);
// Now context can be safely passed to the C library.
addCallback(&myHandler, cast(void*)context);
extern(C) void myHandler(void* ctx)
{
// Assuming that the callback is invoked only once, the
// added root can be removed again now to allow the GC
// to collect it later.
GC.removeRoot(ctx);
GC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
auto context = cast(Object)ctx;
// Use context here…
}
I'd exchangeGC.removeRoot(ctx);
andGC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
, because in OOP resource should be freed in reverse order of their acquision.
– porton
Dec 31 '18 at 15:11
Will your code work if instead:scope auto context = new Object;
(that is Object allocated on the stack rather than on the heap)?
– porton
Dec 31 '18 at 15:36
Not in general - the danger of the callback is that it will outlive the scope of the caller, which means the stack allocated object is definitely dead. If the callback only happens in scope, it doesn't matter either way - the stack reference to the GC'd object will also be live anyway. (And if it just runs inside the C function, the D GC will never run anyway, unless there is another thread triggering it.)
– Adam D. Ruppe
Dec 31 '18 at 15:39
I wanted to ask whetherGC.addRoot(cast(void*)context);
will work ifcontext
is pointing onto the stack. I suspect it may segfault, throw an exception, or lead to undefined behavior. Is my fear founded?
– porton
Dec 31 '18 at 15:49
1
It is undefined; the documentation only says what it does if it points to a GC block or null.
– Adam D. Ruppe
Dec 31 '18 at 16:35
add a comment |
You can tell the GC to hold on to the pointer as a root and furthermore, tell it not to move it on you with the import core.memory; GC.addRoot(ptr);
function. This example shows it in full:
http://dpldocs.info/experimental-docs/core.memory.GC.addRoot.html#examples
// Typical C-style callback mechanism; the passed function
// is invoked with the user-supplied context pointer at a
// later point.
extern(C) void addCallback(void function(void*), void*);
// Allocate an object on the GC heap (this would usually be
// some application-specific context data).
auto context = new Object;
// Make sure that it is not collected even if it is no
// longer referenced from D code (stack, GC heap, …).
GC.addRoot(cast(void*)context);
// Also ensure that a moving collector does not relocate
// the object.
GC.setAttr(cast(void*)context, GC.BlkAttr.NO_MOVE);
// Now context can be safely passed to the C library.
addCallback(&myHandler, cast(void*)context);
extern(C) void myHandler(void* ctx)
{
// Assuming that the callback is invoked only once, the
// added root can be removed again now to allow the GC
// to collect it later.
GC.removeRoot(ctx);
GC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
auto context = cast(Object)ctx;
// Use context here…
}
You can tell the GC to hold on to the pointer as a root and furthermore, tell it not to move it on you with the import core.memory; GC.addRoot(ptr);
function. This example shows it in full:
http://dpldocs.info/experimental-docs/core.memory.GC.addRoot.html#examples
// Typical C-style callback mechanism; the passed function
// is invoked with the user-supplied context pointer at a
// later point.
extern(C) void addCallback(void function(void*), void*);
// Allocate an object on the GC heap (this would usually be
// some application-specific context data).
auto context = new Object;
// Make sure that it is not collected even if it is no
// longer referenced from D code (stack, GC heap, …).
GC.addRoot(cast(void*)context);
// Also ensure that a moving collector does not relocate
// the object.
GC.setAttr(cast(void*)context, GC.BlkAttr.NO_MOVE);
// Now context can be safely passed to the C library.
addCallback(&myHandler, cast(void*)context);
extern(C) void myHandler(void* ctx)
{
// Assuming that the callback is invoked only once, the
// added root can be removed again now to allow the GC
// to collect it later.
GC.removeRoot(ctx);
GC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
auto context = cast(Object)ctx;
// Use context here…
}
answered Dec 31 '18 at 15:05


Adam D. RuppeAdam D. Ruppe
22.8k43354
22.8k43354
I'd exchangeGC.removeRoot(ctx);
andGC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
, because in OOP resource should be freed in reverse order of their acquision.
– porton
Dec 31 '18 at 15:11
Will your code work if instead:scope auto context = new Object;
(that is Object allocated on the stack rather than on the heap)?
– porton
Dec 31 '18 at 15:36
Not in general - the danger of the callback is that it will outlive the scope of the caller, which means the stack allocated object is definitely dead. If the callback only happens in scope, it doesn't matter either way - the stack reference to the GC'd object will also be live anyway. (And if it just runs inside the C function, the D GC will never run anyway, unless there is another thread triggering it.)
– Adam D. Ruppe
Dec 31 '18 at 15:39
I wanted to ask whetherGC.addRoot(cast(void*)context);
will work ifcontext
is pointing onto the stack. I suspect it may segfault, throw an exception, or lead to undefined behavior. Is my fear founded?
– porton
Dec 31 '18 at 15:49
1
It is undefined; the documentation only says what it does if it points to a GC block or null.
– Adam D. Ruppe
Dec 31 '18 at 16:35
add a comment |
I'd exchangeGC.removeRoot(ctx);
andGC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
, because in OOP resource should be freed in reverse order of their acquision.
– porton
Dec 31 '18 at 15:11
Will your code work if instead:scope auto context = new Object;
(that is Object allocated on the stack rather than on the heap)?
– porton
Dec 31 '18 at 15:36
Not in general - the danger of the callback is that it will outlive the scope of the caller, which means the stack allocated object is definitely dead. If the callback only happens in scope, it doesn't matter either way - the stack reference to the GC'd object will also be live anyway. (And if it just runs inside the C function, the D GC will never run anyway, unless there is another thread triggering it.)
– Adam D. Ruppe
Dec 31 '18 at 15:39
I wanted to ask whetherGC.addRoot(cast(void*)context);
will work ifcontext
is pointing onto the stack. I suspect it may segfault, throw an exception, or lead to undefined behavior. Is my fear founded?
– porton
Dec 31 '18 at 15:49
1
It is undefined; the documentation only says what it does if it points to a GC block or null.
– Adam D. Ruppe
Dec 31 '18 at 16:35
I'd exchange
GC.removeRoot(ctx);
and GC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
, because in OOP resource should be freed in reverse order of their acquision.– porton
Dec 31 '18 at 15:11
I'd exchange
GC.removeRoot(ctx);
and GC.clrAttr(ctx, GC.BlkAttr.NO_MOVE);
, because in OOP resource should be freed in reverse order of their acquision.– porton
Dec 31 '18 at 15:11
Will your code work if instead:
scope auto context = new Object;
(that is Object allocated on the stack rather than on the heap)?– porton
Dec 31 '18 at 15:36
Will your code work if instead:
scope auto context = new Object;
(that is Object allocated on the stack rather than on the heap)?– porton
Dec 31 '18 at 15:36
Not in general - the danger of the callback is that it will outlive the scope of the caller, which means the stack allocated object is definitely dead. If the callback only happens in scope, it doesn't matter either way - the stack reference to the GC'd object will also be live anyway. (And if it just runs inside the C function, the D GC will never run anyway, unless there is another thread triggering it.)
– Adam D. Ruppe
Dec 31 '18 at 15:39
Not in general - the danger of the callback is that it will outlive the scope of the caller, which means the stack allocated object is definitely dead. If the callback only happens in scope, it doesn't matter either way - the stack reference to the GC'd object will also be live anyway. (And if it just runs inside the C function, the D GC will never run anyway, unless there is another thread triggering it.)
– Adam D. Ruppe
Dec 31 '18 at 15:39
I wanted to ask whether
GC.addRoot(cast(void*)context);
will work if context
is pointing onto the stack. I suspect it may segfault, throw an exception, or lead to undefined behavior. Is my fear founded?– porton
Dec 31 '18 at 15:49
I wanted to ask whether
GC.addRoot(cast(void*)context);
will work if context
is pointing onto the stack. I suspect it may segfault, throw an exception, or lead to undefined behavior. Is my fear founded?– porton
Dec 31 '18 at 15:49
1
1
It is undefined; the documentation only says what it does if it points to a GC block or null.
– Adam D. Ruppe
Dec 31 '18 at 16:35
It is undefined; the documentation only says what it does if it points to a GC block or null.
– Adam D. Ruppe
Dec 31 '18 at 16:35
add a comment |
Based on Adam D. Ruppe's answer but reorganized.
Even better OO code:
import core.memory : GC;
class UnmovableObject {
this() {
//GC.addRoot(cast(void*)this); // prevents finalization
GC.setAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
~this() {
//GC.removeRoot(cast(void*)this);
GC.clrAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
}
1
That destructor will never run unless called manually (with the.destroy(obj)
function) since while the object is a GC root, it will never be collected and thus never destroyed.
– Adam D. Ruppe
Dec 31 '18 at 15:34
@AdamD.Ruppe That it cannot be done with a destructor is really bad. RAI idiom is broken here. I suggest to devise a new D language feature to solve this problem
– porton
Dec 31 '18 at 15:53
Hm, well: Why toaddRoot
andremoveRoot
?setAttr
/clrAttr
seems to work for any GC pointer, not only for a root. So I propose to removeC.addRoot(cast(void*)this);
andGC.removeRoot(cast(void*)this);
. I think after this my OO code will work. Right?
– porton
Dec 31 '18 at 15:57
Destructors are only triggered when the object is destroyed. A GC root is never destroyed (unless you manually do it). That's the whole point of that function! The original problem was the GC might free the block while C was holding on to it, because D's GC can't see C's internal memory. By adding it as a root, it tell's D's GC not to try to automatically free it until you tell it it is OK (via the removeRoot) function. This protects it from being freed while C is still holding on to it.
– Adam D. Ruppe
Dec 31 '18 at 16:43
add a comment |
Based on Adam D. Ruppe's answer but reorganized.
Even better OO code:
import core.memory : GC;
class UnmovableObject {
this() {
//GC.addRoot(cast(void*)this); // prevents finalization
GC.setAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
~this() {
//GC.removeRoot(cast(void*)this);
GC.clrAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
}
1
That destructor will never run unless called manually (with the.destroy(obj)
function) since while the object is a GC root, it will never be collected and thus never destroyed.
– Adam D. Ruppe
Dec 31 '18 at 15:34
@AdamD.Ruppe That it cannot be done with a destructor is really bad. RAI idiom is broken here. I suggest to devise a new D language feature to solve this problem
– porton
Dec 31 '18 at 15:53
Hm, well: Why toaddRoot
andremoveRoot
?setAttr
/clrAttr
seems to work for any GC pointer, not only for a root. So I propose to removeC.addRoot(cast(void*)this);
andGC.removeRoot(cast(void*)this);
. I think after this my OO code will work. Right?
– porton
Dec 31 '18 at 15:57
Destructors are only triggered when the object is destroyed. A GC root is never destroyed (unless you manually do it). That's the whole point of that function! The original problem was the GC might free the block while C was holding on to it, because D's GC can't see C's internal memory. By adding it as a root, it tell's D's GC not to try to automatically free it until you tell it it is OK (via the removeRoot) function. This protects it from being freed while C is still holding on to it.
– Adam D. Ruppe
Dec 31 '18 at 16:43
add a comment |
Based on Adam D. Ruppe's answer but reorganized.
Even better OO code:
import core.memory : GC;
class UnmovableObject {
this() {
//GC.addRoot(cast(void*)this); // prevents finalization
GC.setAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
~this() {
//GC.removeRoot(cast(void*)this);
GC.clrAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
}
Based on Adam D. Ruppe's answer but reorganized.
Even better OO code:
import core.memory : GC;
class UnmovableObject {
this() {
//GC.addRoot(cast(void*)this); // prevents finalization
GC.setAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
~this() {
//GC.removeRoot(cast(void*)this);
GC.clrAttr(cast(void*)this, GC.BlkAttr.NO_MOVE);
}
}
edited Dec 31 '18 at 18:54
answered Dec 31 '18 at 15:28
portonporton
1,81532242
1,81532242
1
That destructor will never run unless called manually (with the.destroy(obj)
function) since while the object is a GC root, it will never be collected and thus never destroyed.
– Adam D. Ruppe
Dec 31 '18 at 15:34
@AdamD.Ruppe That it cannot be done with a destructor is really bad. RAI idiom is broken here. I suggest to devise a new D language feature to solve this problem
– porton
Dec 31 '18 at 15:53
Hm, well: Why toaddRoot
andremoveRoot
?setAttr
/clrAttr
seems to work for any GC pointer, not only for a root. So I propose to removeC.addRoot(cast(void*)this);
andGC.removeRoot(cast(void*)this);
. I think after this my OO code will work. Right?
– porton
Dec 31 '18 at 15:57
Destructors are only triggered when the object is destroyed. A GC root is never destroyed (unless you manually do it). That's the whole point of that function! The original problem was the GC might free the block while C was holding on to it, because D's GC can't see C's internal memory. By adding it as a root, it tell's D's GC not to try to automatically free it until you tell it it is OK (via the removeRoot) function. This protects it from being freed while C is still holding on to it.
– Adam D. Ruppe
Dec 31 '18 at 16:43
add a comment |
1
That destructor will never run unless called manually (with the.destroy(obj)
function) since while the object is a GC root, it will never be collected and thus never destroyed.
– Adam D. Ruppe
Dec 31 '18 at 15:34
@AdamD.Ruppe That it cannot be done with a destructor is really bad. RAI idiom is broken here. I suggest to devise a new D language feature to solve this problem
– porton
Dec 31 '18 at 15:53
Hm, well: Why toaddRoot
andremoveRoot
?setAttr
/clrAttr
seems to work for any GC pointer, not only for a root. So I propose to removeC.addRoot(cast(void*)this);
andGC.removeRoot(cast(void*)this);
. I think after this my OO code will work. Right?
– porton
Dec 31 '18 at 15:57
Destructors are only triggered when the object is destroyed. A GC root is never destroyed (unless you manually do it). That's the whole point of that function! The original problem was the GC might free the block while C was holding on to it, because D's GC can't see C's internal memory. By adding it as a root, it tell's D's GC not to try to automatically free it until you tell it it is OK (via the removeRoot) function. This protects it from being freed while C is still holding on to it.
– Adam D. Ruppe
Dec 31 '18 at 16:43
1
1
That destructor will never run unless called manually (with the
.destroy(obj)
function) since while the object is a GC root, it will never be collected and thus never destroyed.– Adam D. Ruppe
Dec 31 '18 at 15:34
That destructor will never run unless called manually (with the
.destroy(obj)
function) since while the object is a GC root, it will never be collected and thus never destroyed.– Adam D. Ruppe
Dec 31 '18 at 15:34
@AdamD.Ruppe That it cannot be done with a destructor is really bad. RAI idiom is broken here. I suggest to devise a new D language feature to solve this problem
– porton
Dec 31 '18 at 15:53
@AdamD.Ruppe That it cannot be done with a destructor is really bad. RAI idiom is broken here. I suggest to devise a new D language feature to solve this problem
– porton
Dec 31 '18 at 15:53
Hm, well: Why to
addRoot
and removeRoot
? setAttr
/clrAttr
seems to work for any GC pointer, not only for a root. So I propose to remove C.addRoot(cast(void*)this);
and GC.removeRoot(cast(void*)this);
. I think after this my OO code will work. Right?– porton
Dec 31 '18 at 15:57
Hm, well: Why to
addRoot
and removeRoot
? setAttr
/clrAttr
seems to work for any GC pointer, not only for a root. So I propose to remove C.addRoot(cast(void*)this);
and GC.removeRoot(cast(void*)this);
. I think after this my OO code will work. Right?– porton
Dec 31 '18 at 15:57
Destructors are only triggered when the object is destroyed. A GC root is never destroyed (unless you manually do it). That's the whole point of that function! The original problem was the GC might free the block while C was holding on to it, because D's GC can't see C's internal memory. By adding it as a root, it tell's D's GC not to try to automatically free it until you tell it it is OK (via the removeRoot) function. This protects it from being freed while C is still holding on to it.
– Adam D. Ruppe
Dec 31 '18 at 16:43
Destructors are only triggered when the object is destroyed. A GC root is never destroyed (unless you manually do it). That's the whole point of that function! The original problem was the GC might free the block while C was holding on to it, because D's GC can't see C's internal memory. By adding it as a root, it tell's D's GC not to try to automatically free it until you tell it it is OK (via the removeRoot) function. This protects it from being freed while C is still holding on to it.
– Adam D. Ruppe
Dec 31 '18 at 16:43
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53988772%2fconverting-a-d-object-pointer-to-void-and-passing-to-a-callback%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VCwO3dHO,ImktwONVAgvnNT7FLPLaA,q ideWVHS3iIMV 78flbqSBkEqblJ0p3fAHL yTFOSguP