how to update quantity in shopping cart in flask?
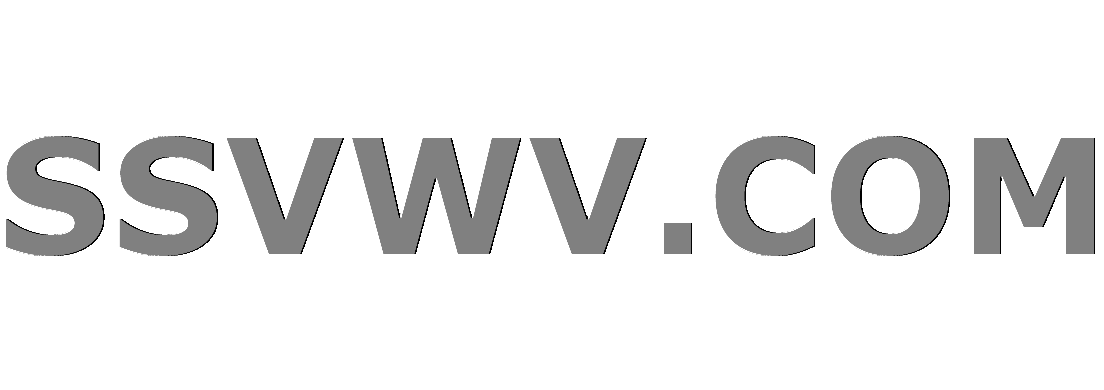
Multi tool use
I'm working on a shopping cart and I'm coding from scratch to try to figure out how it actually works. I ran into a hiccup. the items in the shopping cart are retrieved from MySQL database and are displayed in a table on a website. I want the user to change the quantity automatically when they select the quantity.
I've tried adding tag around and inside the option tag but it does nothing. I also tried adding it around the select tag but once i click on the element the link is run. I came across something like this: onchange="location = this.value;" but I don't know how to implement it.
html select code.
<select id="quantity" name="quantity" class="form-control" style="background-color:white ; border-color:white">
{% for number in quantity_data %}
<option value="{{ number.quantity }}"><a href="/confirm_cart">{{ number.quantity }}</a></option>
<!--<input name="description" type="text" placeholder="Enter description..." class="form-control">-->
{% endfor %}
</select>
flask code - app.py
if request.method == 'POST':
customer_name = request.form['customer_name']
item = request.form['item_']
cost = request.form['cost']
quantity = request.form['quantity']
con = pymysql.connect("localhost", "root", "", "sampledb")
# update main database
cursor = con.cursor()
sql = "UPDATE `shopping_cart_tbl` "
"SET `customer_name`=%s, `item`=%s, `cost`=%s, `quantity`=%s "
"WHERE `customer_name`=%s"
cursor.execute(sql, (customer_name, item, cost, quantity, session['userkey']))
con.commit()
Simply put, i want the select options to act as a link. I already have the update database SQL code. Here's the code
python mysql flask
add a comment |
I'm working on a shopping cart and I'm coding from scratch to try to figure out how it actually works. I ran into a hiccup. the items in the shopping cart are retrieved from MySQL database and are displayed in a table on a website. I want the user to change the quantity automatically when they select the quantity.
I've tried adding tag around and inside the option tag but it does nothing. I also tried adding it around the select tag but once i click on the element the link is run. I came across something like this: onchange="location = this.value;" but I don't know how to implement it.
html select code.
<select id="quantity" name="quantity" class="form-control" style="background-color:white ; border-color:white">
{% for number in quantity_data %}
<option value="{{ number.quantity }}"><a href="/confirm_cart">{{ number.quantity }}</a></option>
<!--<input name="description" type="text" placeholder="Enter description..." class="form-control">-->
{% endfor %}
</select>
flask code - app.py
if request.method == 'POST':
customer_name = request.form['customer_name']
item = request.form['item_']
cost = request.form['cost']
quantity = request.form['quantity']
con = pymysql.connect("localhost", "root", "", "sampledb")
# update main database
cursor = con.cursor()
sql = "UPDATE `shopping_cart_tbl` "
"SET `customer_name`=%s, `item`=%s, `cost`=%s, `quantity`=%s "
"WHERE `customer_name`=%s"
cursor.execute(sql, (customer_name, item, cost, quantity, session['userkey']))
con.commit()
Simply put, i want the select options to act as a link. I already have the update database SQL code. Here's the code
python mysql flask
Two things, if I understand correctly; first, you're not updating any quantity after the insert into the table (either you need to update a value in the session or re-count the items after insert) and secondly, you're not feeding back to the template which probably requires AJAX/JQuery to update that value alone rather than refresh the page.
– roganjosh
Jan 3 at 18:31
in the database the column quantity already has a default 1. when the user selects an option in the drop-down menu in the shopping cart page, it should be updated by running a route that way the change is recorded.. I'm updating in the database. I'm only using session to keep track of the logged in user's cart items so that they are displayed on the shopping cart table. You can replace session['userkey'] with 'peter' pr someone's name.
– High Roller
Jan 3 at 18:52
Thats not what I'm saying. You update it in the database - fine - But why should that automatically update the front end without you actually doing it? You're not reloading anything on the front end.
– roganjosh
Jan 3 at 18:59
i see what you're saying. I hadn't gotten to that point yet honestly. My first concern was updating the DB. I hadn't considered retrieving the database value and displaying it at the front end. IAny suggestion for doing both
– High Roller
Jan 3 at 19:11
add a comment |
I'm working on a shopping cart and I'm coding from scratch to try to figure out how it actually works. I ran into a hiccup. the items in the shopping cart are retrieved from MySQL database and are displayed in a table on a website. I want the user to change the quantity automatically when they select the quantity.
I've tried adding tag around and inside the option tag but it does nothing. I also tried adding it around the select tag but once i click on the element the link is run. I came across something like this: onchange="location = this.value;" but I don't know how to implement it.
html select code.
<select id="quantity" name="quantity" class="form-control" style="background-color:white ; border-color:white">
{% for number in quantity_data %}
<option value="{{ number.quantity }}"><a href="/confirm_cart">{{ number.quantity }}</a></option>
<!--<input name="description" type="text" placeholder="Enter description..." class="form-control">-->
{% endfor %}
</select>
flask code - app.py
if request.method == 'POST':
customer_name = request.form['customer_name']
item = request.form['item_']
cost = request.form['cost']
quantity = request.form['quantity']
con = pymysql.connect("localhost", "root", "", "sampledb")
# update main database
cursor = con.cursor()
sql = "UPDATE `shopping_cart_tbl` "
"SET `customer_name`=%s, `item`=%s, `cost`=%s, `quantity`=%s "
"WHERE `customer_name`=%s"
cursor.execute(sql, (customer_name, item, cost, quantity, session['userkey']))
con.commit()
Simply put, i want the select options to act as a link. I already have the update database SQL code. Here's the code
python mysql flask
I'm working on a shopping cart and I'm coding from scratch to try to figure out how it actually works. I ran into a hiccup. the items in the shopping cart are retrieved from MySQL database and are displayed in a table on a website. I want the user to change the quantity automatically when they select the quantity.
I've tried adding tag around and inside the option tag but it does nothing. I also tried adding it around the select tag but once i click on the element the link is run. I came across something like this: onchange="location = this.value;" but I don't know how to implement it.
html select code.
<select id="quantity" name="quantity" class="form-control" style="background-color:white ; border-color:white">
{% for number in quantity_data %}
<option value="{{ number.quantity }}"><a href="/confirm_cart">{{ number.quantity }}</a></option>
<!--<input name="description" type="text" placeholder="Enter description..." class="form-control">-->
{% endfor %}
</select>
flask code - app.py
if request.method == 'POST':
customer_name = request.form['customer_name']
item = request.form['item_']
cost = request.form['cost']
quantity = request.form['quantity']
con = pymysql.connect("localhost", "root", "", "sampledb")
# update main database
cursor = con.cursor()
sql = "UPDATE `shopping_cart_tbl` "
"SET `customer_name`=%s, `item`=%s, `cost`=%s, `quantity`=%s "
"WHERE `customer_name`=%s"
cursor.execute(sql, (customer_name, item, cost, quantity, session['userkey']))
con.commit()
Simply put, i want the select options to act as a link. I already have the update database SQL code. Here's the code
python mysql flask
python mysql flask
asked Jan 3 at 18:20


High RollerHigh Roller
12
12
Two things, if I understand correctly; first, you're not updating any quantity after the insert into the table (either you need to update a value in the session or re-count the items after insert) and secondly, you're not feeding back to the template which probably requires AJAX/JQuery to update that value alone rather than refresh the page.
– roganjosh
Jan 3 at 18:31
in the database the column quantity already has a default 1. when the user selects an option in the drop-down menu in the shopping cart page, it should be updated by running a route that way the change is recorded.. I'm updating in the database. I'm only using session to keep track of the logged in user's cart items so that they are displayed on the shopping cart table. You can replace session['userkey'] with 'peter' pr someone's name.
– High Roller
Jan 3 at 18:52
Thats not what I'm saying. You update it in the database - fine - But why should that automatically update the front end without you actually doing it? You're not reloading anything on the front end.
– roganjosh
Jan 3 at 18:59
i see what you're saying. I hadn't gotten to that point yet honestly. My first concern was updating the DB. I hadn't considered retrieving the database value and displaying it at the front end. IAny suggestion for doing both
– High Roller
Jan 3 at 19:11
add a comment |
Two things, if I understand correctly; first, you're not updating any quantity after the insert into the table (either you need to update a value in the session or re-count the items after insert) and secondly, you're not feeding back to the template which probably requires AJAX/JQuery to update that value alone rather than refresh the page.
– roganjosh
Jan 3 at 18:31
in the database the column quantity already has a default 1. when the user selects an option in the drop-down menu in the shopping cart page, it should be updated by running a route that way the change is recorded.. I'm updating in the database. I'm only using session to keep track of the logged in user's cart items so that they are displayed on the shopping cart table. You can replace session['userkey'] with 'peter' pr someone's name.
– High Roller
Jan 3 at 18:52
Thats not what I'm saying. You update it in the database - fine - But why should that automatically update the front end without you actually doing it? You're not reloading anything on the front end.
– roganjosh
Jan 3 at 18:59
i see what you're saying. I hadn't gotten to that point yet honestly. My first concern was updating the DB. I hadn't considered retrieving the database value and displaying it at the front end. IAny suggestion for doing both
– High Roller
Jan 3 at 19:11
Two things, if I understand correctly; first, you're not updating any quantity after the insert into the table (either you need to update a value in the session or re-count the items after insert) and secondly, you're not feeding back to the template which probably requires AJAX/JQuery to update that value alone rather than refresh the page.
– roganjosh
Jan 3 at 18:31
Two things, if I understand correctly; first, you're not updating any quantity after the insert into the table (either you need to update a value in the session or re-count the items after insert) and secondly, you're not feeding back to the template which probably requires AJAX/JQuery to update that value alone rather than refresh the page.
– roganjosh
Jan 3 at 18:31
in the database the column quantity already has a default 1. when the user selects an option in the drop-down menu in the shopping cart page, it should be updated by running a route that way the change is recorded.. I'm updating in the database. I'm only using session to keep track of the logged in user's cart items so that they are displayed on the shopping cart table. You can replace session['userkey'] with 'peter' pr someone's name.
– High Roller
Jan 3 at 18:52
in the database the column quantity already has a default 1. when the user selects an option in the drop-down menu in the shopping cart page, it should be updated by running a route that way the change is recorded.. I'm updating in the database. I'm only using session to keep track of the logged in user's cart items so that they are displayed on the shopping cart table. You can replace session['userkey'] with 'peter' pr someone's name.
– High Roller
Jan 3 at 18:52
Thats not what I'm saying. You update it in the database - fine - But why should that automatically update the front end without you actually doing it? You're not reloading anything on the front end.
– roganjosh
Jan 3 at 18:59
Thats not what I'm saying. You update it in the database - fine - But why should that automatically update the front end without you actually doing it? You're not reloading anything on the front end.
– roganjosh
Jan 3 at 18:59
i see what you're saying. I hadn't gotten to that point yet honestly. My first concern was updating the DB. I hadn't considered retrieving the database value and displaying it at the front end. IAny suggestion for doing both
– High Roller
Jan 3 at 19:11
i see what you're saying. I hadn't gotten to that point yet honestly. My first concern was updating the DB. I hadn't considered retrieving the database value and displaying it at the front end. IAny suggestion for doing both
– High Roller
Jan 3 at 19:11
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54027738%2fhow-to-update-quantity-in-shopping-cart-in-flask%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54027738%2fhow-to-update-quantity-in-shopping-cart-in-flask%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GwsQbhf5VBESQnkIAfm1NV,hn X M IZZWl9ba2 ekhBLT,t9gg5QKE,qJ4IQSp
Two things, if I understand correctly; first, you're not updating any quantity after the insert into the table (either you need to update a value in the session or re-count the items after insert) and secondly, you're not feeding back to the template which probably requires AJAX/JQuery to update that value alone rather than refresh the page.
– roganjosh
Jan 3 at 18:31
in the database the column quantity already has a default 1. when the user selects an option in the drop-down menu in the shopping cart page, it should be updated by running a route that way the change is recorded.. I'm updating in the database. I'm only using session to keep track of the logged in user's cart items so that they are displayed on the shopping cart table. You can replace session['userkey'] with 'peter' pr someone's name.
– High Roller
Jan 3 at 18:52
Thats not what I'm saying. You update it in the database - fine - But why should that automatically update the front end without you actually doing it? You're not reloading anything on the front end.
– roganjosh
Jan 3 at 18:59
i see what you're saying. I hadn't gotten to that point yet honestly. My first concern was updating the DB. I hadn't considered retrieving the database value and displaying it at the front end. IAny suggestion for doing both
– High Roller
Jan 3 at 19:11