How can I prevent form fields from being reset on button click? (typescript/angular)
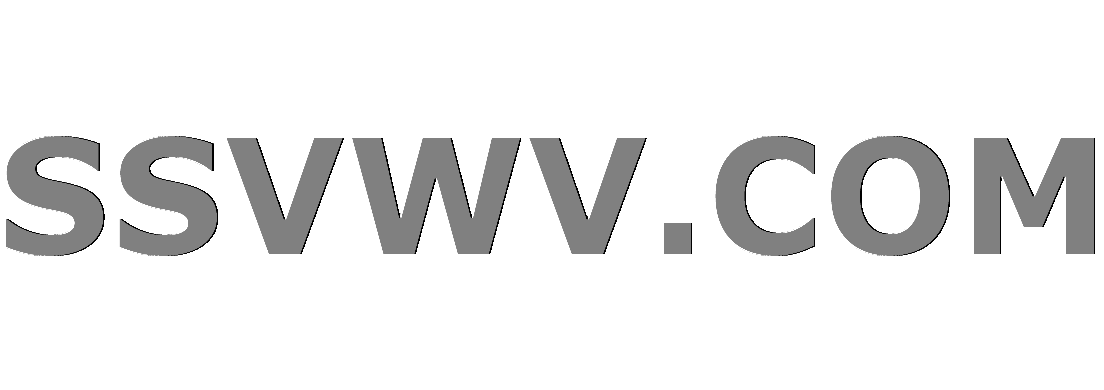
Multi tool use
There is a button that users can click which will add more address form fields. If users fill out their address information in the fields and then click the button to add more addresses, the previous inputs get cleared. How can I prevent the button from clearing previous entries?
Snippets from my component.ts file:
ngOnInit() {
this.addresses = ;
this.addresses.push(new Address('', undefined ,'','Canada',''));
}
addAddress(){
this.addresses.push(new Address('', undefined ,'','Canada',''));
}
My address.ts file:
export class Address {
constructor(
public streetAddress: string,
public province: string,
public city: string,
public country: string,
public postalCode: string
) { }
}
Snippets from my component.html file:
<div class="card-body" *ngFor="let address of addresses">
<div class="row">
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control"
[(ngModel)]="address.streetAddress" #streetAddress="ngModel"
name="streetAddress" placeholder="Apartment/House Number and Street
Address">
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" [(ngModel)]="address.city"
#city="ngModel" name="city" placeholder="City/Town">
</div>
</div>
</div>
</div>
<button type="button" class="btn btn-warning btn-block" id="addAddress"
(click)="addAddress()">More Addresses</button>

add a comment |
There is a button that users can click which will add more address form fields. If users fill out their address information in the fields and then click the button to add more addresses, the previous inputs get cleared. How can I prevent the button from clearing previous entries?
Snippets from my component.ts file:
ngOnInit() {
this.addresses = ;
this.addresses.push(new Address('', undefined ,'','Canada',''));
}
addAddress(){
this.addresses.push(new Address('', undefined ,'','Canada',''));
}
My address.ts file:
export class Address {
constructor(
public streetAddress: string,
public province: string,
public city: string,
public country: string,
public postalCode: string
) { }
}
Snippets from my component.html file:
<div class="card-body" *ngFor="let address of addresses">
<div class="row">
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control"
[(ngModel)]="address.streetAddress" #streetAddress="ngModel"
name="streetAddress" placeholder="Apartment/House Number and Street
Address">
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" [(ngModel)]="address.city"
#city="ngModel" name="city" placeholder="City/Town">
</div>
</div>
</div>
</div>
<button type="button" class="btn btn-warning btn-block" id="addAddress"
(click)="addAddress()">More Addresses</button>

add a comment |
There is a button that users can click which will add more address form fields. If users fill out their address information in the fields and then click the button to add more addresses, the previous inputs get cleared. How can I prevent the button from clearing previous entries?
Snippets from my component.ts file:
ngOnInit() {
this.addresses = ;
this.addresses.push(new Address('', undefined ,'','Canada',''));
}
addAddress(){
this.addresses.push(new Address('', undefined ,'','Canada',''));
}
My address.ts file:
export class Address {
constructor(
public streetAddress: string,
public province: string,
public city: string,
public country: string,
public postalCode: string
) { }
}
Snippets from my component.html file:
<div class="card-body" *ngFor="let address of addresses">
<div class="row">
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control"
[(ngModel)]="address.streetAddress" #streetAddress="ngModel"
name="streetAddress" placeholder="Apartment/House Number and Street
Address">
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" [(ngModel)]="address.city"
#city="ngModel" name="city" placeholder="City/Town">
</div>
</div>
</div>
</div>
<button type="button" class="btn btn-warning btn-block" id="addAddress"
(click)="addAddress()">More Addresses</button>

There is a button that users can click which will add more address form fields. If users fill out their address information in the fields and then click the button to add more addresses, the previous inputs get cleared. How can I prevent the button from clearing previous entries?
Snippets from my component.ts file:
ngOnInit() {
this.addresses = ;
this.addresses.push(new Address('', undefined ,'','Canada',''));
}
addAddress(){
this.addresses.push(new Address('', undefined ,'','Canada',''));
}
My address.ts file:
export class Address {
constructor(
public streetAddress: string,
public province: string,
public city: string,
public country: string,
public postalCode: string
) { }
}
Snippets from my component.html file:
<div class="card-body" *ngFor="let address of addresses">
<div class="row">
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control"
[(ngModel)]="address.streetAddress" #streetAddress="ngModel"
name="streetAddress" placeholder="Apartment/House Number and Street
Address">
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" [(ngModel)]="address.city"
#city="ngModel" name="city" placeholder="City/Town">
</div>
</div>
</div>
</div>
<button type="button" class="btn btn-warning btn-block" id="addAddress"
(click)="addAddress()">More Addresses</button>


asked Jan 3 at 18:33
HoobsHoobs
135
135
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Better practice to store and operate data in services
address.service.ts
import { Injectable } from '@angular/core';
import { Address } from './Address';
@Injectable()
export class AddressService {
constructor() { }
addresses = ;
addAddress(address: Address) {
this.addresses.push(Address);
}
}
In addAddresses method as argument you can give an Address from submitted form
Also read about how to correctly manage your form here
Your component.ts file can be like:
export class AppComponent implements OnInit {
addresses: Address;
form = new FormGroup({
streetAddress: new FormControl(''),
province: new FormControl(''),
city: new FormControl(''),
country: new FormControl(''),
postalCode: new FormControl('')
});
constructor(private addrs: AddressService) {}
ngOnInit() {
this.addresses = this.addrs.addresses;
}
onSubmit() {
this.addrs.addAddress(this.form.value);
this.form.reset();
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54027917%2fhow-can-i-prevent-form-fields-from-being-reset-on-button-click-typescript-angu%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Better practice to store and operate data in services
address.service.ts
import { Injectable } from '@angular/core';
import { Address } from './Address';
@Injectable()
export class AddressService {
constructor() { }
addresses = ;
addAddress(address: Address) {
this.addresses.push(Address);
}
}
In addAddresses method as argument you can give an Address from submitted form
Also read about how to correctly manage your form here
Your component.ts file can be like:
export class AppComponent implements OnInit {
addresses: Address;
form = new FormGroup({
streetAddress: new FormControl(''),
province: new FormControl(''),
city: new FormControl(''),
country: new FormControl(''),
postalCode: new FormControl('')
});
constructor(private addrs: AddressService) {}
ngOnInit() {
this.addresses = this.addrs.addresses;
}
onSubmit() {
this.addrs.addAddress(this.form.value);
this.form.reset();
}
}
add a comment |
Better practice to store and operate data in services
address.service.ts
import { Injectable } from '@angular/core';
import { Address } from './Address';
@Injectable()
export class AddressService {
constructor() { }
addresses = ;
addAddress(address: Address) {
this.addresses.push(Address);
}
}
In addAddresses method as argument you can give an Address from submitted form
Also read about how to correctly manage your form here
Your component.ts file can be like:
export class AppComponent implements OnInit {
addresses: Address;
form = new FormGroup({
streetAddress: new FormControl(''),
province: new FormControl(''),
city: new FormControl(''),
country: new FormControl(''),
postalCode: new FormControl('')
});
constructor(private addrs: AddressService) {}
ngOnInit() {
this.addresses = this.addrs.addresses;
}
onSubmit() {
this.addrs.addAddress(this.form.value);
this.form.reset();
}
}
add a comment |
Better practice to store and operate data in services
address.service.ts
import { Injectable } from '@angular/core';
import { Address } from './Address';
@Injectable()
export class AddressService {
constructor() { }
addresses = ;
addAddress(address: Address) {
this.addresses.push(Address);
}
}
In addAddresses method as argument you can give an Address from submitted form
Also read about how to correctly manage your form here
Your component.ts file can be like:
export class AppComponent implements OnInit {
addresses: Address;
form = new FormGroup({
streetAddress: new FormControl(''),
province: new FormControl(''),
city: new FormControl(''),
country: new FormControl(''),
postalCode: new FormControl('')
});
constructor(private addrs: AddressService) {}
ngOnInit() {
this.addresses = this.addrs.addresses;
}
onSubmit() {
this.addrs.addAddress(this.form.value);
this.form.reset();
}
}
Better practice to store and operate data in services
address.service.ts
import { Injectable } from '@angular/core';
import { Address } from './Address';
@Injectable()
export class AddressService {
constructor() { }
addresses = ;
addAddress(address: Address) {
this.addresses.push(Address);
}
}
In addAddresses method as argument you can give an Address from submitted form
Also read about how to correctly manage your form here
Your component.ts file can be like:
export class AppComponent implements OnInit {
addresses: Address;
form = new FormGroup({
streetAddress: new FormControl(''),
province: new FormControl(''),
city: new FormControl(''),
country: new FormControl(''),
postalCode: new FormControl('')
});
constructor(private addrs: AddressService) {}
ngOnInit() {
this.addresses = this.addrs.addresses;
}
onSubmit() {
this.addrs.addAddress(this.form.value);
this.form.reset();
}
}
answered Jan 3 at 19:12


Roman LitvinovRoman Litvinov
1819
1819
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54027917%2fhow-can-i-prevent-form-fields-from-being-reset-on-button-click-typescript-angu%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EClwlj qV,C,KqCasUYHlV,V